PHP type checking
PhpStorm is a static code analysis tool that can parse and validate PHP data structures that involve compound data types, pseudotypes, or generics-style notations.
If you enforce the type safety of your PHP code with PHPDoc type annotations or the attribute syntax, PhpStorm will infer the defined types of keys and values and suggest code completion or quick fixes if applicable. PHP type checking is supported for:
Object and array shapes
PhpStorm parses the key-value structures declared for objects and object-like arrays in PHPDoc blocks or via attributes (#[ArrayShape]
, #[ObjectShape]
). As you type, the IDE infers the value type and invokes code completion for the keys.
If automatic code completion is disabled, press Ctrl+Space or choose
from the main menu.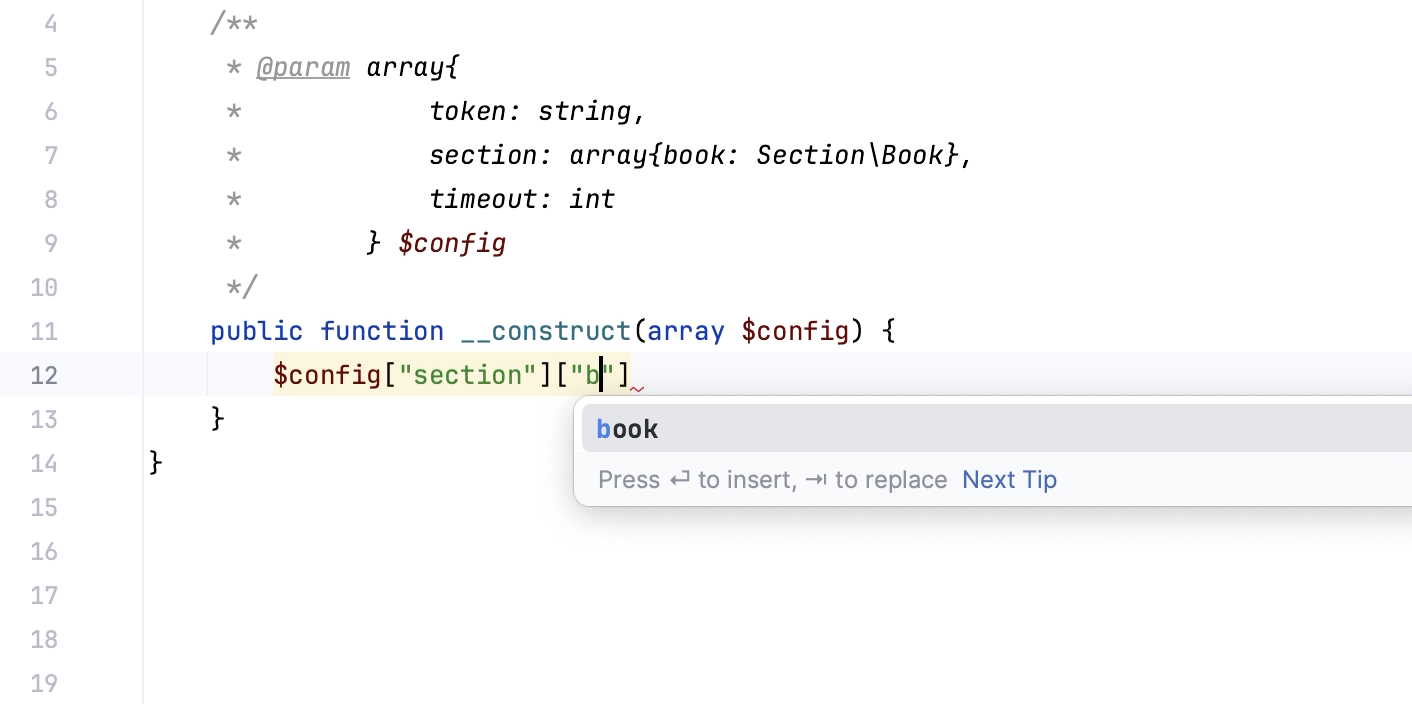
For more examples, see this blogpost.
To generate an annotation for the existing object shape, place the caret at the relevant code element, press Alt+Enter, and select the Add '#[ObjectShape]' attribute or Add ObjectShape to PHPDoc context action from the menu that opens.
In a similar manner, the context actions for array shapes are Add '#[ArrayShape]' attribute and Add ArrayShape to PHPDoc.
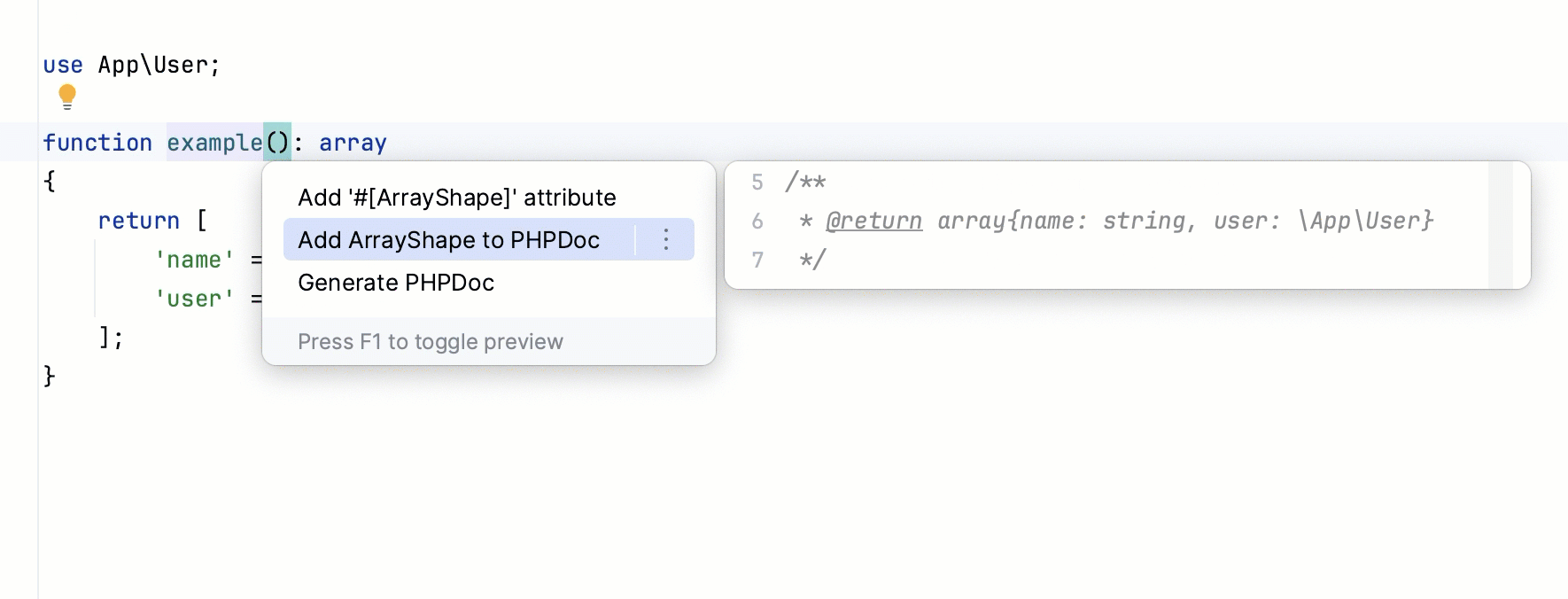
Integer ranges
To have PhpStorm check that the provided values are within the specified range of accepted integers, use the int<min, max>
syntax in the PHPDoc annotation.
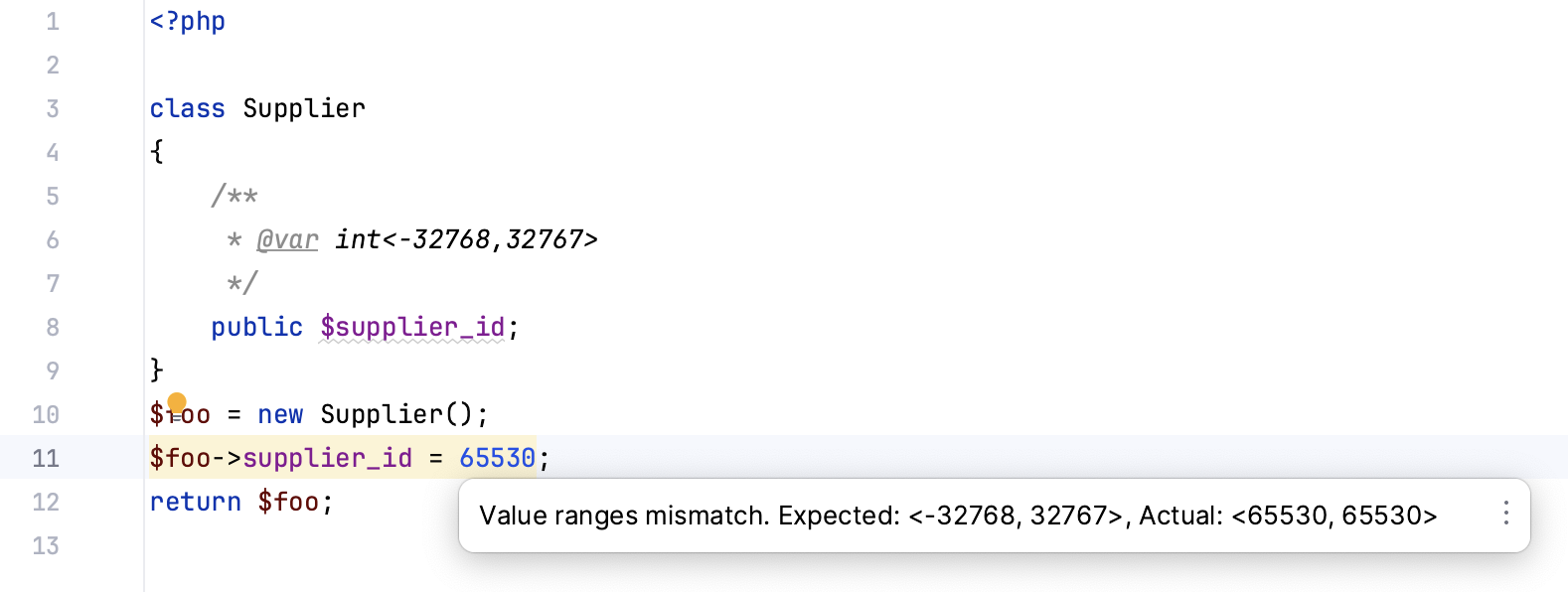
Psalm annotations
PhpStorm has built-in support for Psalm-specific annotations. When specifying an annotation, in most cases you can omit the @psalm-
part. The supported type annotations include:
Psalm type annotations. You can use these annotations for pseudotypes such as
numeric
orscalar
to provide PhpStorm with additional type information.@psalm-param
annotation, which specifies the type that a method can accept. In this case, PhpStorm starts to support all properties and methods of the type.When providing type definitions via the
@psalm-param
or@psalm-var
tags, you can use the constants' values unions (|
) and wildcards (*
) for constants sharing a common prefix.@psalm-type
annotation, which lets you create an alias to any other type in the code./** @psalm-type UserName = array{name: string} */ class Name { /** @psalm-return UserName */ function toArray(): array { return ["name" => "Matt"]; } }@psalm-import-type
annotation, which enables import of types defined with@psalm-type
.
Generic types support
PhpStorm supports generic types in PHP through the @template annotation. Initial support covers a simple case of a function returning one of its parameters.
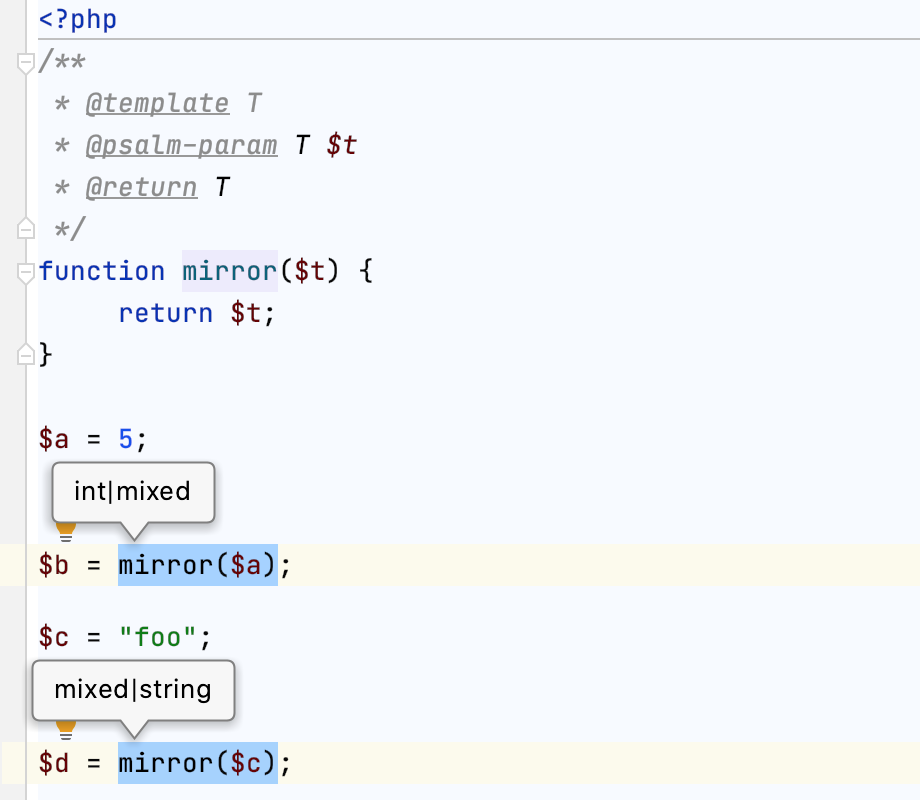