Postfix templates
Postfix templates help you transform expressions that you have already typed without jumping backwards — just type a dot after an expression and pick a template from the completion list.
Apply postfix templates
One of the simplest examples of postfix templates is negating a boolean expression. Suppose you have just typed a boolean expression and then realized that the comparison logic should be reversed. Normally, you have to move your caret back and change ==
to !=
or vice versa, and then return to where you were. With ReSharper, you can just continue typing .not
and press Enter.
There are a lot more postfix templates that you can use to speed up coding. For example, you can wrap the current expression with if
, while
, lock
, using
, add return
, yield return
, await
in front of the current expression, iterate over a collection, generate a switch statement, cast the expression to a specific type, or even introduce a field or property for the expression.
Postfix template could even change your typing routine. Consider the CheckInput
method below and imagine how you would type the null-checking clause.
Now let's see how you could do it with postfix templates.
As soon as your caret is in the method body, you can start typing input
right away because that is what you want to check. When the input
is there, just continue typing .null
- a postfix template for checking the expression for null
:
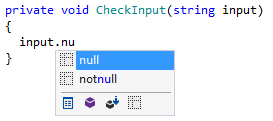
This will compare input
with null
and wrap the comparison in an if
statement, and place the caret at the position where you can continue typing:
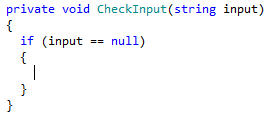
Now, instead of typing throw
, you can go ahead with typing the exception class name, and invoke the .throw
template after it:
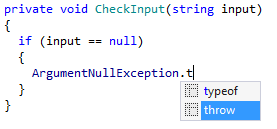
After applying this template, you have the complete throw
statement, and the caret at a place to enter arguments:
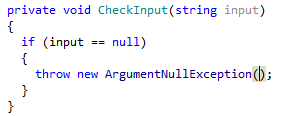
You could see that instead of typing frequently used language constructs manually, in many cases you can type just a couple of initial characters of a template shortcut and get everything in place, properly formatted and without typos.
Apply a postfix template
Type a dot after the current expression and check the completion list for the desired templates.
If you know the shortcut of the template that you are going to apply, start typing it — this will shrink the list of suggestions.
Depending on the current context, some postfix templates may not be included in the completion list. If you do not see the desired template, but still want to use it, press Control+Space twice to see all templates to the completion list.
As soon as the desired template is selected in the suggestion list, press Enter.
If the template has editable parameters (that is, requires user input), ReSharper deploys a hot spot session in the editor and sets the input position at the first parameter. Then you can do the following:
If ReSharper suggests some values for the current parameter, use Up and Down arrow keys to navigate through the list of suggested values, or just type in a desired value.
Press Tab or Enter to accept the value and move to the input position of the next parameter. If this is the last parameter, the hot spot session completes and the caret moves to the end position defined for the session.
Press Shift+Tab to move the input focus to the input position of the previous parameter.
Press Esc to exit the hot spot session. In this case, all session parameters will be initialized with default values.
Configure postfix templates
Postfix templates are enabled by default. They appear in the completion list if:
ReSharper IntelliSense is enabled on the Alt+R, O.
page of ReSharper optionsShow postfix templates is selected on the page of ReSharper options Alt+R, O.
To disable specific postfix templates, clear the corresponding check-boxes on the Alt+R, O.
page of ReSharper optionsTo hide templates in the completion list or, conversely, hide all other items, use completion filters.
List of postfix templates
Shortcut | Description | Example |
---|---|---|
.arg | Surrounds expression with invocation |
|
.await | Awaits expressions of 'Task' type |
|
.cast | Surrounds expression with cast |
|
.else | Checks boolean expression to be 'false' |
|
.field | Introduces field for expression |
|
.for | Iterates over collection with index |
|
.foreach | Iterates over enumerable collection |
|
.forr | Iterates over collection in reverse with index |
|
.if | Checks boolean expression to be 'true' |
|
.lock | Surrounds expression with lock block |
|
.new | Produces instantiation expression for type |
|
.not | Negates boolean expression |
|
.notnull | Checks expression to be not-null |
|
.null | Checks expression to be null |
|
.par | Parenthesizes current expression |
|
.parse | Parses string as value of some type |
|
.prop | Introduces property for expression |
|
.return | Returns expression from current function |
|
.sel | Selects expression in editor |
|
.switch | Produces switch statement |
|
.throw | Throws expression of 'Exception' type |
|
.to | Assigns current expression to some variable |
|
.tryparse | Parses string as value of some type |
|
.typeof | Wraps type usage with typeof() expression |
|
.using | Wraps resource with using statement |
|
.var | Introduces variable for expression |
|
.while | Iterating while boolean statement is 'true' |
|
.yield | Yields value from iterator method |
|
Shortcut | Description | Example |
---|---|---|
.beg..end | Produces iterators from range |
|
.Cast | Surrounds expression with UE cast |
|
.cbeg..cend | Produces iterators from range |
|
.co_await | Passes expression as argument to co_await |
|
.co_return | Returns expression from current coroutine |
|
.co_yield | Passes expression as argument to co_yield |
|
.const_cast | Surrounds expression with const_cast |
|
.do | Iterating until boolean expression becomes 'false' |
|
.dynamic_cast | Surrounds expression with dynamic_cast |
|
.else | Checks boolean expression to be 'false' |
|
.foreach | Iterates over range |
|
.forward | Forwards function parameter |
|
.if | Checks boolean expression to be 'true' |
|
.make_shared | Constructs an object and wraps it in a std::shared_ptr |
|
.make_unique | Constructs an object and wraps it in a std::unique_ptr |
|
.new | Produces instantiation expression for type |
|
.reinterpret_cast | Surrounds expression with reinterpret_cast |
|
.return | Returns expression from current function |
|
.safe_cast | Surrounds expression with safe_cast (C++/CLI) |
|
.static_cast | Surrounds expression with static_cast |
|
.switch | Produces switch over integral/enum type |
|
.var | Introduces variable for expression |
|
.while | Iterating while boolean expression is 'true' |
|
Shortcut | Description | Example |
---|---|---|
.else | Checks boolean expression to be 'false' |
|
.forof | Iterates over an iterable object |
|
.if | Checks boolean expression to be 'true' |
|
.notnull | Checks expression to be not-null |
|
.notundefined | Checks expression to be not-undefined |
|
.null | Checks expression to be null |
|
.return | Returns expression from current function |
|
.undefined | Checks expression to be undefined |
|
.var | Introduces variable for expression |
|
Shortcut | Description | Example |
---|---|---|
.else | Checks boolean expression to be 'false' |
|
.forof | Iterates over an iterable object |
|
.if | Checks boolean expression to be 'true' |
|
.instanceof | Checks instance of expression |
|
.notnull | Checks expression to be not-null |
|
.notundefined | Checks expression to be not-undefined |
|
.null | Checks expression to be null |
|
.return | Returns expression from current function |
|
.typeof | Checks type of expression |
|
.undefined | Checks expression to be undefined |
|
.var | Introduces variable for expression |
|
This feature is supported in the following languages and technologies: