Doctest
Doctest is a single-header framework with self-registering tests. As stated in its documentation, Doctest was designed after Catch and shares some parts of the Catch's code - refer to How is doctest different from Catch.
Doctest doesn't support mocking but you can integrate it with third-party mocking libraries such as trompeloeil, googlemock, or FakeIt.
Doctest basics
If you are not familiar with Doctest, you can find a description of its main concepts below:
Sample test
A simple test written with Doctest looks like this:
In the example above, testing the factorial function is a free-form test name, which doesn't have to be unique. CHECK
is one of the Doctest's assertion macros.
Test cases, subtests, and suites
Doctest provides the mechanism of subcases for creating nested tests with shared common setup and teardown (however, class-based fixtures are also supported).
In the code above, TEST_CASE()
is executed from the start for each SUBCASE()
. Two REQUIRE
statements guarantee that size
is 5 and capacity
is at least 5 at the entry of each subcase. If one of the CHECK()
-s fails, this means the test has failed, but the execution continues. On each run of a TEST_CASE()
, Doctest executes one subcase and skips the others. Next time, the second one is executed, and so on.
Similarly to Catch's sections, subcases can be nested to create sequences of checking operations. Each leaf subcase (a subcase with no nested subcases inside) is executed once. When a parent subcase fails, it prevents child subcases from running.
You can group test cases into suites using the TEST_SUITE()
or TEST_SUITE_BEGIN()
/TEST_SUITE_END()
macros:
Doctest also supports the BDD-style syntax.
Working with Doctest in CLion
Add Doctest to your project
Download the latest version of doctest.h and copy it into your project tree.
The minimum supported version is 2.3.0.
Include the header in your test files:
#include "doctest.h"In only one header file, precede
#include
with#define DOCTEST_CONFIG_IMPLEMENT_WITH_MAIN
.See these instructions if you need to supply your own
main
.
Run/debug the automatically created Doctest configuration
CLion detects the Doctest tests in your project and creates a run/debug configuration for them.
You can use this configuration right away to run or debug all the Doctest tests in your project:
Add a new Doctest run/debug configuration
Go to Run | Edit Configurations.
Click
and select Doctest from the list of templates.
Set the configuration name in the Name field. This name will be shown in the list of the available run/debug configurations.
Select this option to run a particular test or suite.
In the Suite field, specify the suite name. Start typing, and auto-completion will suggest the available suits:
In the Test field, select the desired test. Leave this field to run the entire suite.
Select this option to specify a single test or define a set of tests:
[#*filename.cpp]
to run all tests in filename.cpp,[suite_name]
to run a suite with the corresponding name,[suite_name][test_name]
or[suite_name][test_name][subtest_name]
to run a particular test from a given suite,use
[]
([][]
) to refer to an anonymous suite, but make sure to add a test name.
You can specify several patterns in a comma-separated list, for example:
Alternatively, you can leave the Suite and Test fields empty, and provide the Doctest's command line flags via Program arguments instead.
In the Target field, select the desired target from the list.
Click Apply to save the configuration.
Select the newly created configuration in the switcher and run
or debug
it.
Run tests
The quickest way to run or debug test in CLion is by using the gutter icons:
Gutter icons also show test results (when already available): success
or failure
.
When you run a test/suite/fixture using gutter icons, CLion creates a temporary Doctest configuration, which is greyed out in the list of configurations. To save a temporary configuration, select it in the dialog and click
:
Explore test results
When you run tests, CLion shows the results and the process in the built-in test runner window. The test tree shows all the tests while they are being executed one by one. The test runner window includes:
progress bar with the percentage of tests executed so far,
tree view of all the running tests with their status and duration,
tests' output stream,
toolbar with the options to rerun failed
tests, import/export or open previous results saved automatically
, sort the tests alphabetically to easily find a particular test, or sort them by duration
to understand which test ran longer than others.
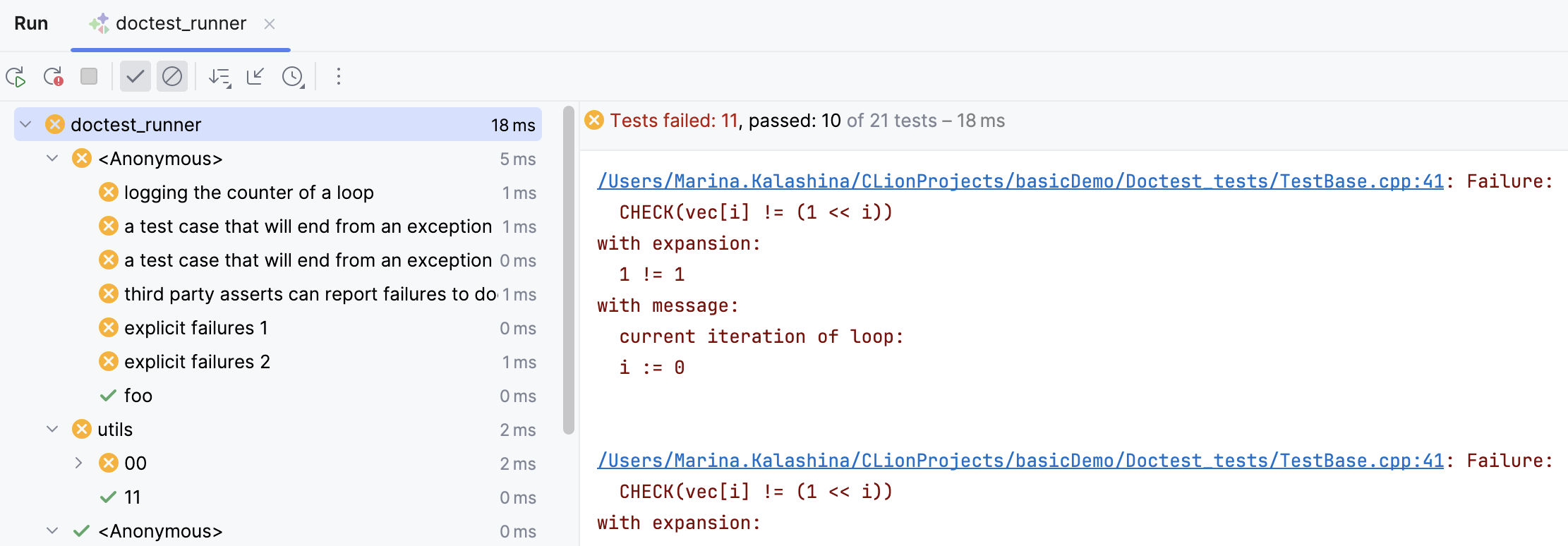