Google Test
Google Test and Google Mock are a pair of unit testing tools developed by Google.
Adding Google Test to your project
Download Google Test from the official repository and extract the contents of googletest-master into an empty folder in your project (for example, Google_tests/lib).
Alternatively, clone Google Test as a git submodule or use CMake to download it (instructions below will not be applicable in the latter case).
Create a CMakeLists.txt file inside the Google_tests folder: right-click it in the project tree and select .
Customize the following lines and add them into your script:
# 'Google_test' is the subproject name project(Google_tests) # 'lib' is the folder with Google Test sources add_subdirectory(lib) include_directories(${gtest_SOURCE_DIR}/include ${gtest_SOURCE_DIR}) # 'Google_Tests_run' is the target name # 'test1.cpp tests2.cpp' are source files with tests add_executable(Google_Tests_run test1.cpp tests2.cpp) target_link_libraries(Google_Tests_run gtest gtest_main)In your root CMakeLists.txt script, add the
add_subdirectory(Google_tests)
command to the end, then reload the project.When writing tests, make sure to add
#include "gtest/gtest.h"
at the beginning of every .cpp file with your tests code.
Generate menu for Google Test
In the files with gtest
included, you can generate code for tests and test fixtures using the Generate menu Alt+Insert.
When called from a fixture, this menu additionally includes SetUp Method and TearDown Method:
Google Test run/debug configuration
Although Google Test provides the main()
entry, and you can run tests as regular applications, we recommend using the dedicated Google Test run/debug configuration. It includes test-related settings and let you benefit from the built-in test runner, which is unavailable if you run tests as regular programs.
To create a Google Test configuration, go to Run | Edit Configurations, click
and select Google Test from the list of templates.
Specify the test or suite to be included in the configuration, or provide a pattern for filtering test names. Auto-completion is available in the fields to help you quickly fill them up:
Set wildcards to specify test patterns, for example:
In other fields of the configuration settings, you can set environment variables and command line options. For example, use Program arguments field to pass the
--gtest_repeat
flag and run a Google test multiple times:The output will look as follows:
Repeating all tests (iteration 1) ... Repeating all tests (iteration 2) ... Repeating all tests (iteration 3) ...Save the configuration, and it's ready for Run
or Debug
.
Running tests
In CLion, there are several ways to start a run/debug session for tests, one of which is using special gutter icons. These icons help quickly run or debug a single test or a whole suite/fixture:
Gutter icons also show test results (when already available): success or failure
.
When you run a test/suite/fixture using gutter icons, CLion creates a temporary Google Test configuration, which is greyed out in the configurations list. To save a temporary configuration, select it in the dialog and press :
Exploring results
When you run tests, the results (and the process) are shown in the test runner window. This window includes:
progress bar with the percentage of tests executed so far,
tree view of all the running tests with their status and duration,
tests' output stream,
toolbar with the options to rerun failed
tests, export
or open previous results saved automatically
, sort the tests alphabetically
to easily find a particular test, or sort them by duration
to understand which test ran longer than others.
Test tree shows all the tests while they are being executed one by one. For parameterized tests, you will see the parameters in the tree as well. Also, the tree includes disabled tests (those with the DISABLED
prefix in their names) and marks them as skipped with the corresponding icon.
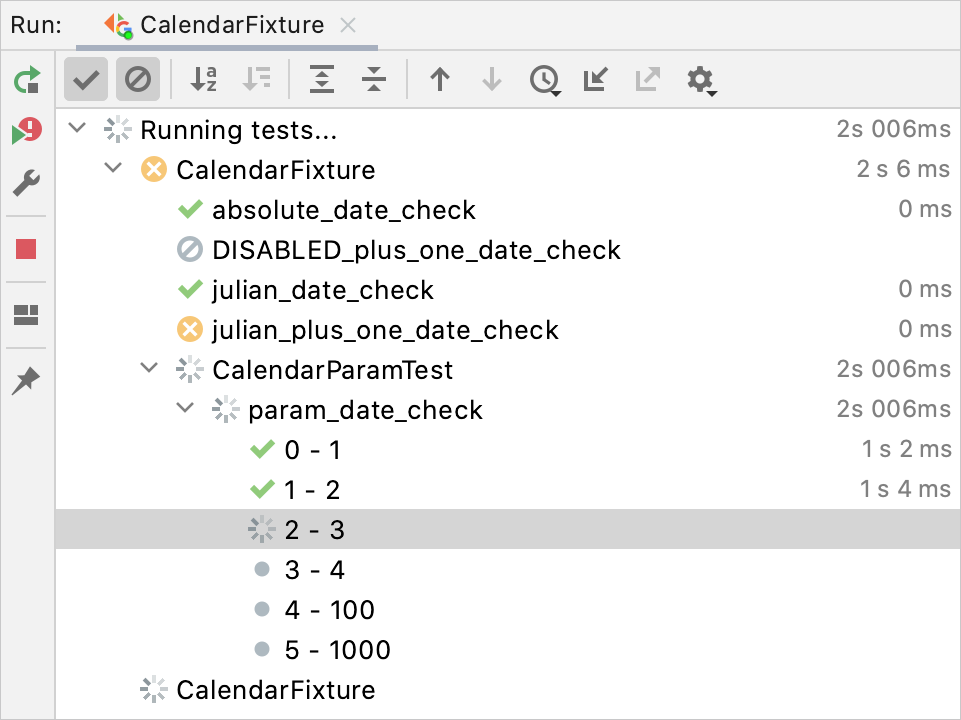
Skipping tests at runtime
You can configure some tests to be skipped based on a condition evaluated at runtime. For this, use the GTEST_SKIP()
macro.
Add the conditional statement and the GTEST_SKIP()
macro to the test you want to skip:
Use the Show ignored icon to view/hide skipped tests in the Test Runner tree:
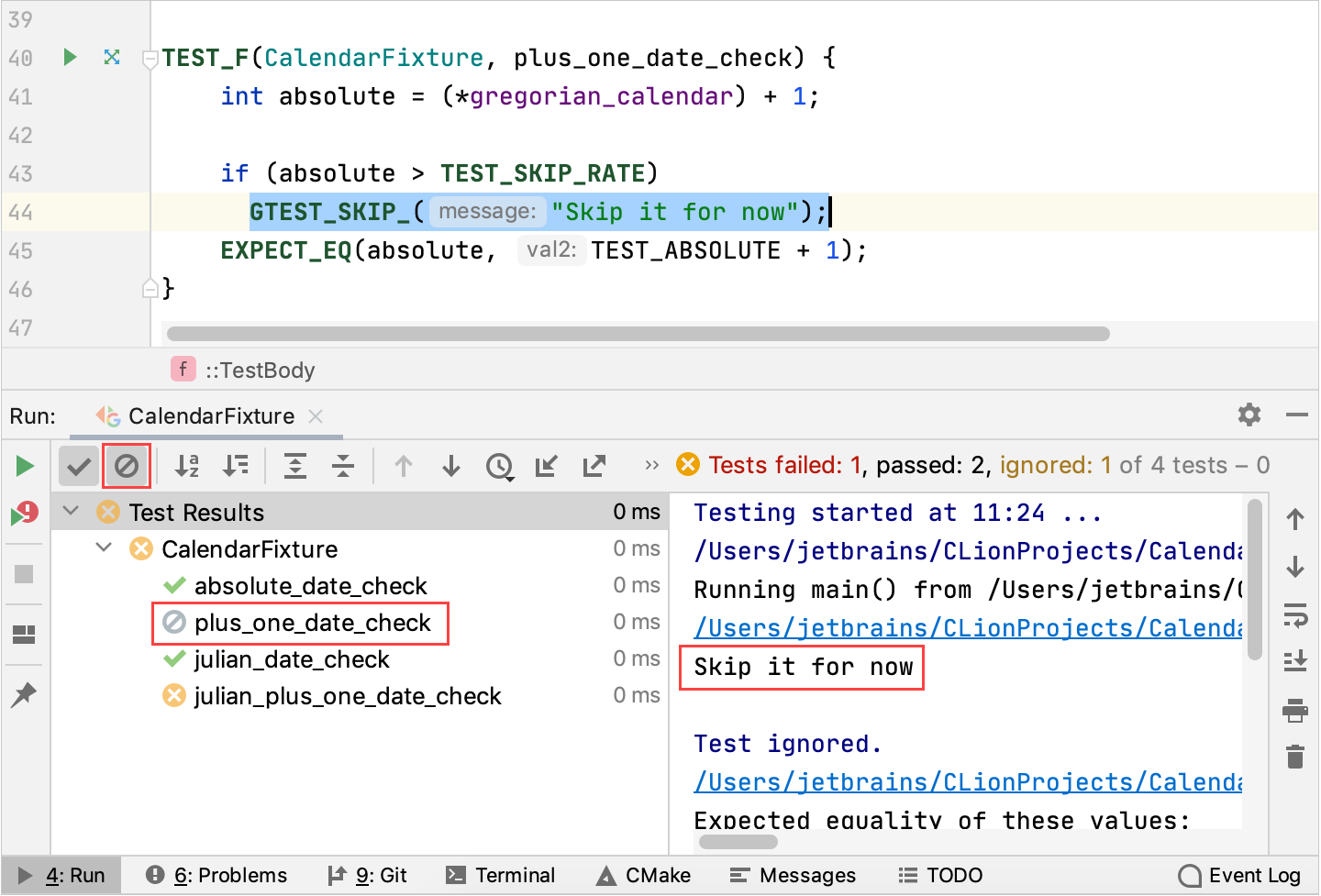