Code completion
This section covers various techniques of context-aware code completion that allow you to speed up your coding process.
Basic completion
Basic code completion helps you complete the names of classes, methods, and keywords within the visibility scope. When you invoke code completion, CLion analyses the context and suggests the choices that are reachable from the current caret position (suggestions also include Live templates).
If basic code completion is applied to a part of a field, parameter, or variable declaration, CLion suggests a list of possible names depending on the item type.
Invoke basic completion
By default, CLion displays the code completion popup automatically as you type. Alternatively, you can press Ctrl+Space or select
from the main menu.
Type-matching completion
Smart type-matching code completion filters the suggestions list and shows only the types applicable to the current context.
Invoke type-matching completion
To invoke type-matching completion, start typing and press Ctrl+Shift+Space or select
from the main menu.
Statement completion
You can create syntactically correct code constructs by using statement completion. It inserts the necessary syntax elements (parentheses, braces, and semicolons) and gets you in a position where you can start typing the next statement. To invoke statement completion, start typing a code construct and press Ctrl+Shift+Enter.
Complete Statement works with the following language constructs:
Type and type members: classes, namespaces, and enums.
Statements:
if/else
,while
,do
,for
,switch/case
,try/catch
.
Complete a function declaration
Start typing a function declaration and press Ctrl+Shift+Enter after the opening parenthesis.
Before Complete Statement
After Complete Statement
void myFunc(/*caret*/void myFunc();/*caret*/
Complete a code construct
Start typing a code construct and press Ctrl+Shift+Enter.
CLion automatically completes the construct and adds the required punctuation. The caret is placed at the next editing position.
Use case
Before Complete Statement
After Complete Statement
Class declaration
class NewClass /*caret*/class NewClass { /*caret*/ };switch
clauseswitch/*caret*/switch (/*caret*/) {}switch (i /*caret*/) {}switch (i) { /*caret*/ }while
statementwhile/*caret*/while (/*caret*/) {}while (n > 0/*caret*/) {}while (n > 0) { /*caret*/ }if
statementif /*caret*/if (/*caret*/) {}if (n > 0/*caret*/) {}if (n > 0) { /*caret*/ }for
statementfor /*caret*/for (/*caret*/) {}for (int i = 0; i < 10; ++i/*caret*/) {}for (int i = 0; < 10; ++i) { /*caret*/ }Try-catch statement
try /*caret*/try { /*caret*/ }catch /*caret*/catch (/*caret*/) { }catch (const std::exception/*caret*/) {}catch (const std::exception) { /*caret*/ }
Hippie completion
Hippie completion is a completion engine that analyses your text in the visible scope and generates suggestions from the current context. It helps you complete any word from any of the currently opened files.
Expand a string at caret to an existing word
Type the initial string and do one of the following:
Press Alt+/ or choose
to search for matching words before the caret.Press Alt+Shift+/ or choose
to search for matching words after the caret and in other open files.
The first suggested value appears, and the prototype is highlighted in the source code.
Accept the suggestion, or hold the Alt key and keep pressing / until the desired word is found.
Postfix code completion
Postfix code completion helps you reduce backward caret jumps as you write code. You can transform an already-typed expression to a different one based on a postfix you type after the dot, the type of expression, and its context.
In CLion, postfix code completion is available for C/C++, Python, Rust, JavaScript, TypeScript, and SQL.
For C++, postfix completion can be especially useful for the cases of wrapping with a C++-style cast, make_shared
/ make_unique
, various loops, and begin
/ end
idioms.
Enable and configure postfix completion
Go to Settings / Preferences | Editor | General | Postfix Completion and select the Enable postfix completion checkbox.
Select Tab, Space, or Enter to be used for expanding postfix templates.
Enable/disable a particular postfix template for the selected language.
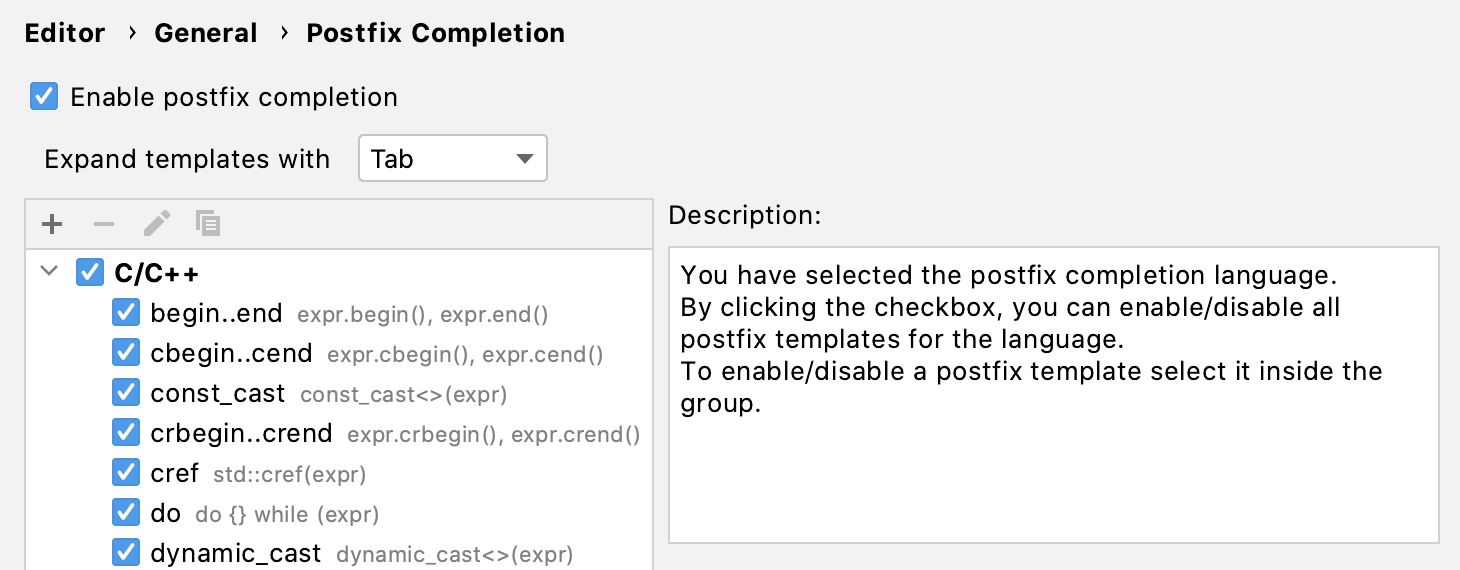
Transform a statement with a postfix
Type an expression and then type a postfix after a dot, for example,
.if:
void myfunc (int arg) { arg.if }The initial expression gets wrapped with an
if
statement:void myfunc (int arg) { if (arg) }
Postfix completion options are shown as part of the basic completion suggestions list:
You can edit the predefined postfix templates, for example, to replace a long key with a shorter one, or to expand the list of applicable expression types.
Create custom postfix templates (JavaScript, TypeScript, SQL, Rust)
Press Ctrl+Alt+S to open the IDE settings and select Editor | General | Postfix Completion.
Click the Add button (
) on the toolbar.
Specify Key that is a combination of symbols that will invoke the template.
Select which expression types the new template will be applicable to, and type the target expression in the following format:
$EXPR$ <target_expression>
, for example,$EXPR$ =!null
.
Code completion settings
To configure code completion options, go to the Editor | General | Code Completion page of the IDE settings Ctrl+Alt+S.
You can choose the following settings:
Item | Description |
---|---|
Match case | Select if you want letter case to be taken into account for completion suggestions. Choose whether you want to match case for the first letter or for all letters. |
Automatically insert single suggestions for | Select if you want to automatically complete code if there's just one suggestion for basic and/or smart type-matching completion. |
Sort suggestions alphabetically | Select if you want to sort items in the suggestions list in the alphabetical order instead of sorting them by relevance. You can change this behavior at any time by clicking |
Show suggestions as you type | Select if you want the suggestions list to be invoked automatically, without having to call completion explicitly. This option is enabled by default. |
Insert selected suggestion by pressing space, dot, or other context-dependent keys | Select if you want to insert the selected suggestion by typing certain keys that depend on the language, your context, and so on. |
Show the documentation popup in | Select to automatically show a popup for each item in the suggestions list with the documentation for the class, method, or field currently highlighted in the lookup list. In the field to the right, specify the delay (in milliseconds), after which the popup should appear. |
Sort completion suggestions based on Machine Learning | Select if you want to utilize machine learning models to rank most suitable items higher in the suggestions list. |
JavaScript | |
Only type-based completion | By default, CLion suggests completion for symbols regardless of their types. With this approach, in complicated cases the list shows multiple completion variants. To make completion more precise, select this option. The completion list will strongly depend on the CLion inference. As a result, the list may remain empty in case of poor inference. |
Suggest items with optional chaining for nullable types | By default, CLion suggests completion for symbols with the optional chaining operator (?). Clear this checkbox to suppress this behavior. |
Expand method bodies in completion for overrides | By default, when you want to override a method from the parent class or interface and select this method from the list of completion suggestions, CLion automatically adds parameters, generates a Clear this checkbox to suppress automatic generation of method bodies for overrides during completion. |
Completion of names |
|
Parameter Info | |
Show the parameter info popup (in ms) | Select this checkbox to have CLion automatically show a popup with all available method signatures when an opening bracket is typed in the editor, or a method is selected from the suggestions list. In the text field to the right, specify the delay (in milliseconds) after when the popup window should appear. If this checkbox is not selected, use Ctrl+P to show parameter info. |
Show full method signatures | If this checkbox is selected, the parameter info displays full signatures, including method name and returned type. |
SQL | |
Use aliases in completion for JOIN | Creates aliases for tables in the JOIN statement. ![]() |
Invert order of operands in auto-generated ON clause | Switches operands in the ON clause. When the checkbox is cleared, the FROM table comes the first in the JOIN condition. ![]() |
Automatically add aliases when completing table names | Creates an alias for a table name. ![]() |
Suggest alias names in completion after table names | Suggests an alias for a table name when you use code completion (Ctrl+Space). ![]() |
Custom aliases (table) | You can add a table name and the alias that you want to use for this table. To add the table-alias pair, click the Add alias button ( |
Completion tips and tricks
Narrow down the suggestions list
You can narrow down the suggestions list by typing any part of a word (even characters from somewhere in the middle) or invoking code completion after a dot separator. CLion will show suggestions that include the characters you've entered in any positions.
This makes the use of wildcards unnecessary:
In case of CamelCase or snake_case names, type the initial letters only. CLion automatically recognizes and matches the initial letters.
Accept a suggestion
You can accept a suggestion from the list in one of the following ways:
Press Enter or double-click a list item to insert it to the left of the caret.
Press Tab to replace the characters to the right from the caret.
Use Ctrl+Shift+Enter to make the current code construct syntactically correct (balance parentheses, add missing braces and semicolons, and so on).
You can also use specific keys to insert the selected completion suggestion: go to the Editor | General | Code Completion page of the IDE settings Ctrl+Alt+S and select the Insert selected suggestion by pressing space, dot, or other context-dependent keys option. These keys depend on the language, your context, and so on.
View reference
You can use the Quick Definition view by pressing Ctrl+Shift+I when you select an entry in the suggestions list:
You can use the Quick Documentation view by pressing Ctrl+Q when you select an entry in the suggestions list:
View code hierarchy
You can view code hierarchies when you've selected an entry from the suggestions list:
Ctrl+H- view type hierarchy
Ctrl+Alt+H- view call hierarchy.
Use machine-learning-assisted code completion
For JavaScript, TypeScript, Python, and Rust, you can utilize machine learning models to rank most suitable items higher in the suggestions list.
To do so, press Ctrl+Alt+S to open the IDE settings and select Editor | General | Code Completion, and then enable the Sort completion suggestions based on machine learning option under Machine Learning-Assisted Completion.
Troubleshooting
If code completion doesn't work, this may be due to one of the following reasons:
The Power Save Mode is on (File | Power Save Mode). Turning it on minimizes power consumption of your laptop by eliminating the background operations, including error highlighting, on-the-fly inspections, and code completion.
Your file doesn't reside in a content root, so it doesn't get the required class definitions and resources needed for code completion.
A file with the symbols that you want to appear in completion is excluded from indexing.