Docker toolchain
For the purpose of development in Docker containers, CLion provides full Docker integration via the dedicated Docker toolchain. Watch this video to learn more:
Sample Dockerfile
To help you get started with Docker development in CLion, we created an example Dockerfile for the case of Ubuntu base image. You can copy this file to your project and adjust for your needs or just use it as a reference.
The example file includes the following lines and sections:
In the comments at the top, you can find the commands for building the container.
The
FROM ubuntu:20.04
line refers to the container's base image.The
apt-get
part installs all the toolchain dependencies into the container. Here you can adjust the tools and their versions.
Build the container
Run the
docker build
command from the top of the Dockerfile:docker build -t clion/ubuntu/cpp-env:1.0 -f Dockerfile.cpp-env-ubuntu .Depending on your platform and your Docker setup, you may need to run it using
sudo
.This command will build the Ubuntu base image with proper toolchain dependencies.
Create a Docker toolchain
Go to
.Click
and select Docker:
Click the gear button to the Server field to add a Docker image:
You can also configure a Docker server in
and then select it in the toolchain settings.Select the Docker image and wait until the tools detection finishes.
Use the Container Settings field to provide additional container settings, such as port and volume bindings:
Build, run, debug with a Docker toolchain
After configuring a Docker toolchain, you can select it in CMake profiles or in Makefile settings. Alternatively, move the toolchain to the top of the list to make it default.
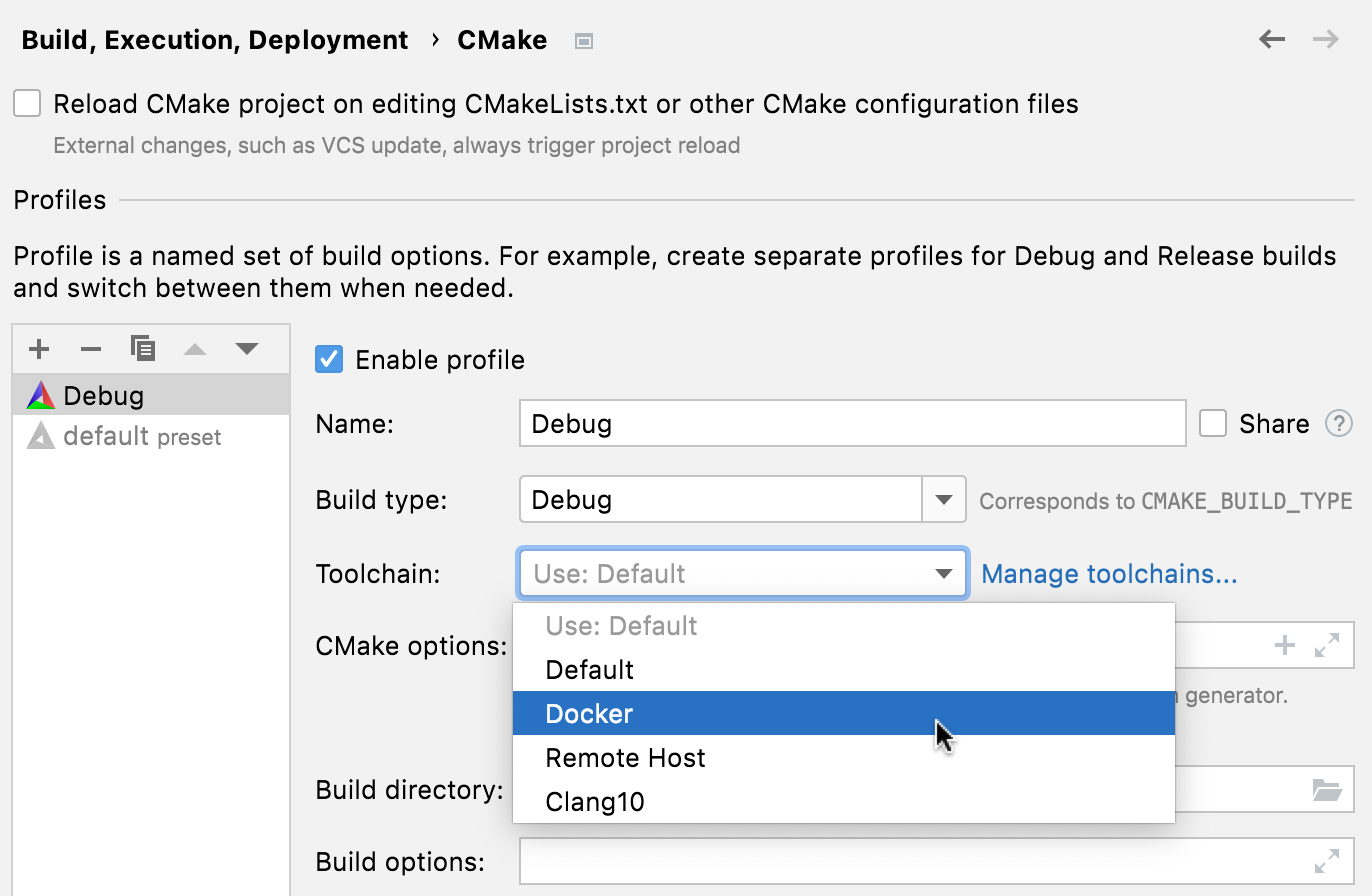
The project folder will be mounted to the Docker container, and build/run/debug will be performed inside it.
By default, the project folder is mounted into the /tmp folder in the container. However, if there are path mappings specified in the toolchain, CLion will use them instead.
For example, if the project root is /data/code/project and toolchain path mapping is /data/code-> /code, then CLion will reuse this mapping and will not mount the project into /tmp/project.
CLion will start the container and shut it down after the command is executed.
Bind mounts on SELinux
You can add :z
configuration flags to Docker bind mount to connect files and folders to containers running on workstations with SELinux enabled. This allows CMake and other tools to access your project files and other files on the system.
Go to
.Set the corresponding checkbox:
Improve Docker toolchain performance on Windows
To get better performance on Windows, we recommend using Docker with the WSL 2 backend.
In the Docker desktop application, navigate to
and enable integration with your WSL distribution (for example,ubuntu-20.04
).Place the project sources into the WSL filesystem (for example, \\wsl$\ubuntu-20.04\tmp\llvm), then open it in CLion and configure a Docker toolchain.
Alternative workflow: develop in Docker using remote with local sources
In this case, Docker-based toolchains are configured via remote with local sources. The container should be running with an SSH daemon.
Sample Dockerfile for remote scenario
Use the remote-cpp-env example file. It includes two additional sections:
The
ssh
section sets up the SSH for CLion to connect into.The
user
section creates a user into the container.
1. Build the container
Use the
docker build
line from the top of the Dockerfile:docker build -t clion/remote-cpp-env:0.5 -f Dockerfile.remote-cpp-env .Depending on your platform and your Docker setup, you may need to run it using
sudo
.This command will build the Ubuntu base image with proper toolchain dependencies, set up SSH, and create the user.
2. Run the container
Use the next command,
docker run
:docker run -d --cap-add sys_ptrace -p127.0.0.1:2222:22 --name clion_remote_env clion/remote-cpp-env:0.5In this line,
-d
runs the container as a daemon and--cap-add sys_ptrace
adds theptrace
capability, which is necessary for debugging.The
-p
part specifies a port mapping. It exposes the default SSH port inside the container (22) as port 2222 on the host environment. You can specify any available port numbers here.(Optional) You can create mapped volumes using the
-v
flag:-v /local/path/to/project:/remote/path/to/project
After that, go to Local or mounted folder, and set the path mappings. See Remote with local sources: Check and adjust the deployment configuration.
, change the connection type to
3. Clear cached SSH keys
Last step of building and running the container is the
ssh-keygen
command, which clears any cached SSH keys. This is important since localhost ports are only temporarily mapped and can be reused by different containers.ssh-keygen -f "$HOME/.ssh/known_hosts" -R [localhost]:2222"
4. Create a Remote Host toolchain
At this point, the container is running with an SSH server daemon, and you can connect into it using CLion’s standard Remote Development features.
Follow the general instructions on creating a remote toolchain.
In the Credentials field, set up the SSH configuration:
Host - localhost
Port - 2222
User name / Password - as specified in the Dockerfile
After establishing the connection, CLion attempts to detect the toolchain. Since the tools were installed into default locations, they will be detected automatically.
If you change the
apt-get
part of the Dockefile to install the tools into other locations, provide the paths in the Make, C Compiler, C++ Compiler, and Debugger fields.Create a CMake profile that uses the remote toolchain. Wait for the project to reload.
After the files get transferred into the container, you will be able to select the profile in the Run/Debug configuration switcher to build, run, or debug your code inside the container using the specified toolchain.