Getting started with JavaScript and TypeScript
This tutorial gets you up to speed with JavaScript development in JetBrains Fleet. It covers the installation, project setup, and working with code. The steps show vanilla JavaScript and Node.js, however you can use them for TypeScript as well.
Prerequisites
JetBrains Toolbox 1.22.10970 or later: the Download page.
Node.js is installed on your computer
Download and install Fleet
Download and install JetBrains Toolbox.
In JetBrains Toolbox, click Install near the JetBrains Fleet icon.
Set up a workspace
Workspace is the directory where your project resides. It contains the project files and settings. You can open an existing project or start a new project by opening an empty directory.
Open a workspace
Press ⌘ O or select File | Open from the menu.
In the file browser, navigate to an empty folder where you want to store your code and click Open.
When you open a directory, it becomes the root of a workspace. You can view its contents in the Files view.
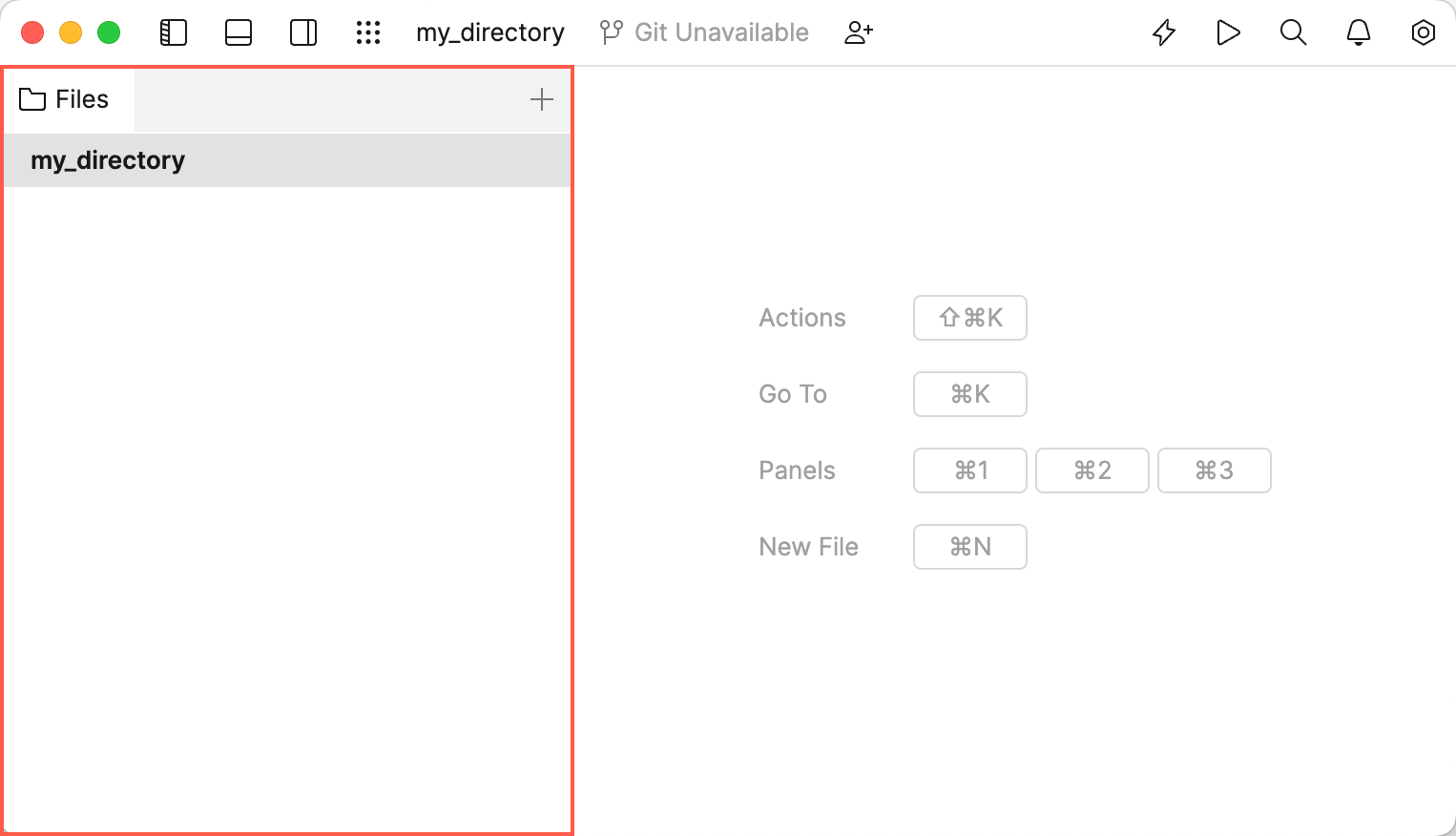
Configure a Node.js interpreter
Press ⌘ , and switch to the tab with the workspace settings.
From the Node.js list, select the path to your Node.js installation. To use the system Node.js, select node.
If you followed the standard installation procedure, Fleet detects your Node.js and adds the path to it to the list.
To use a custom Node.js installation, select Add Node.js Interpreter and select the relevant path in the dialog that opens.
Create project files
In the Files view, right-click the root folder, then select New File. Give the new file a name, for example,
app.js
.Paste the following code in the editor:
const http = require('http'); const hostname = '127.0.0.1'; const port = 3000; const server = http.createServer((req, res) => { res.statusCode = 200; res.setHeader('Content-Type', 'text/plain'); res.end('Hello World'); }); server.listen(port, hostname, () => { console.log(`Server running at http://${hostname}:${port}/`); });In the Files view, right-click the root folder, then select New File. Name the file
package.json
.Paste the following code in the editor:
{ "name": "server", "version": "1.0.0", "scripts": { "start": "node app.js" } }
Smart mode
You can use JetBrains Fleet as a smart text editor, rather than a full-fledged code editor. However, if you need code intelligence features, you can enable them by turning the Smart Mode on.
Enable smart mode
In the top-right corner of the window, click Smart Mode, then Enable.
After you click the Enable button, you may have to wait for some time, while the backend is being prepared.
Here's what you can do in Smart Mode. The below is not an exhaustive list of Smart Mode features, rather a couple of examples that'll help you to get the feel of how it works in Fleet.
Use quick-fixes and intention actions
Press ⌥ ⏎ to access actions that Fleet suggests in the current context.
Solve problems using quick-fixes:
Improve your code using intention actions:
Refactor code
Place the caret at a symbol you want to rename. Press ⌃ R and give it a new name.
The symbol gets the new name and all its usages are updated.
Navigate the codebase
Navigate to the declaration of a symbol with ⌘ B.
Use code interlines to navigate to usages.
Skim over the errors with ⌘ E and ⌘ ⇧ E.
Run code
You can run server-side JavaScript code right from Fleet provided that you have installed and configured Node.js on your computer as described in Configure a Node.js interpreter. For more information, refer to Run JavaScript code
Navigate to your package.json file and click the Run icon in the gutter next to the
start
script and select Run 'start'.The application output is shown in the console:
Reformat code with Prettier
Prettier is a tool to format .js, .ts, .css, .less, .scss, .vue, and .json code. With JetBrains Fleet, you can format selected code fragments as well as entire files.
To install Prettier, open the built-in Terminal ( ) and type one of the following commands:
npm install --save-dev --save-exact prettier
npm install --global prettier
Learn more about installation modes from the Prettier official website.
To reformat a file, select
from its context menu.To reformat a code fragment, select it and then select
from its context menu.