Tutorial: Create your first Kotlin application
In this tutorial, you will learn how to:
Create a Kotlin project.
Write code using the basic coding assistance features.
Run your code from IntelliJ IDEA.
Build and package the application.
Run the packaged application.
You can choose to build your app with one of the four supported build tools.
Create a new project
In IntelliJ IDEA, a project helps you organize everything that is necessary for developing your application in a single unit.
From the main menu, select
.In the left-hand menu, select Kotlin.
Give your project a name and select Console Application as Project Template.
For Build System, select Gradle Groovy IntelliJ Maven.
In Project JDK select a JDK (version 8 or later is recommended).
Click Next, then Finish.
Write code
Source code is the central part of your project. In source code, you define what your application will be doing. Real projects may be very big and contain hundreds of thousands lines of code. In this tutorial we are going to start with a simple three-line application that asks the user their name and greets them.
In the Project tool window on the left, expand the node named after your project and open the /src/main/kotlin/main.kt file.
The file only contains the
main()
function with a single print statement. This function is the entry point of your program.Replace
Hello world
withWhat is your name?
.Now that the program asks the user for input, we must provide them with a way to give it. Also, the program needs to store the input somewhere.
Move the caret to the next line and type
val name = rl
. IntelliJ IDEA will suggest to convertrl
toreadLine()
. Hit Enter to accept the suggestion.Move the caret to the next line, type
sout
, and hit Enter.Place the caret inside the parentheses of the
println
statement and type"Hello, $"
. Press Ctrl+Space to invoke code completion and select thename
variable from the list.
Now we have a working code that reads the user name from the console, stores it in a read-only variable, and outputs a greeting using the stored value.
Run code from IntelliJ IDEA
Let's verify that our program works as expected.
IntelliJ IDEA allows you to run applications right from the editor. You don't need to worry about the technical aspect because IntelliJ IDEA automatically does all the necessary preparations behind the scenes.
Click the Run icon in the gutter and select Run 'MainKt' or press Ctrl+Shift+F10.
When the program has started, the Run tool window opens, where you can review the output and interact with the program.
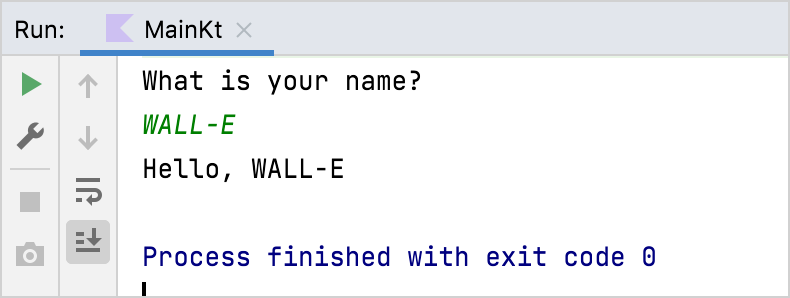
Package as JAR
At this point, you know how to write code and run it from IntelliJ IDEA, which is convenient in the development process. However, this is not the way the users are supposed to run applications. For the users to run it on their computers, we are going to build and package the application as a jar file.
Building the app includes the following steps:
Compiling the sources – in this step, you translate the code you've just written into JVM bytecode. The compiled sources have the .class extension.
Bundling the dependencies – for the application to function correctly, we need to provide the libraries it depends on. The only required library for this project is Kotlin runtime, but still, it needs to be included. Otherwise, the users will have to provide it themselves every time they run the program, which is not very convenient.
Both the compiled sources and the dependencies end up in the resulting .jar file. The result of the build process such as .jar file is called an artifact.
Open the
build.gradle.kts
build.gradle
script.In the build script, add the following task definition:
tasks.jar { manifest { attributes["Main-Class"] = "MainKt" } configurations["compileClasspath"].forEach { file: File -> from(zipTree(file.absoluteFile)) } duplicatesStrategy = DuplicatesStrategy.INCLUDE }tasks.jar { manifest { attributes 'Main-Class': 'MainKt' } from { configurations.compile.collect { it.isDirectory() ? it : zipTree(it) } } }The
manifest
section specifies the entry point of the program, and the rest tells the build tool to recursively scan the project for dependencies and include them in the build.In the right-hand sidebar, open Gradle and run the
jar
task ( ). If the sidebar is not present, go to and toggle the Tool Window Bars menu item.
The resulting JAR appears in the build/libs directory.
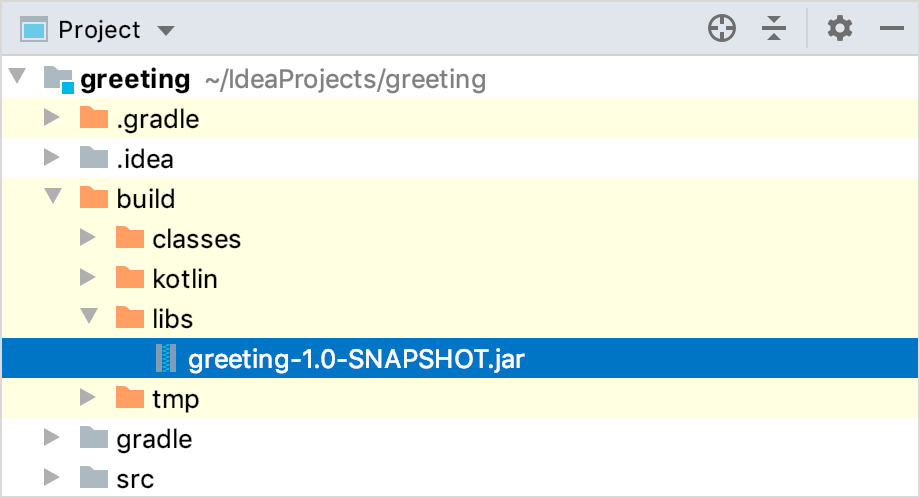
Go to Artifacts tab.
Ctrl+Alt+Shift+S and open theClick Add
, then JAR, then From modules with dependencies.
In the Main Class field, click the Browse button, and select Main.kt as the main class.
Specify the absolute path to
/src/main/resources
as the manifest directory, for example:/Users/me.user/IdeaProjects/greeting/src/main/resources
Click OK in the Create JAR from Modules dialog.
Click OK in the Project Structure dialog.
From the main menu, select Build.
, then click
The resulting JAR appears in the out/artifacts directory.
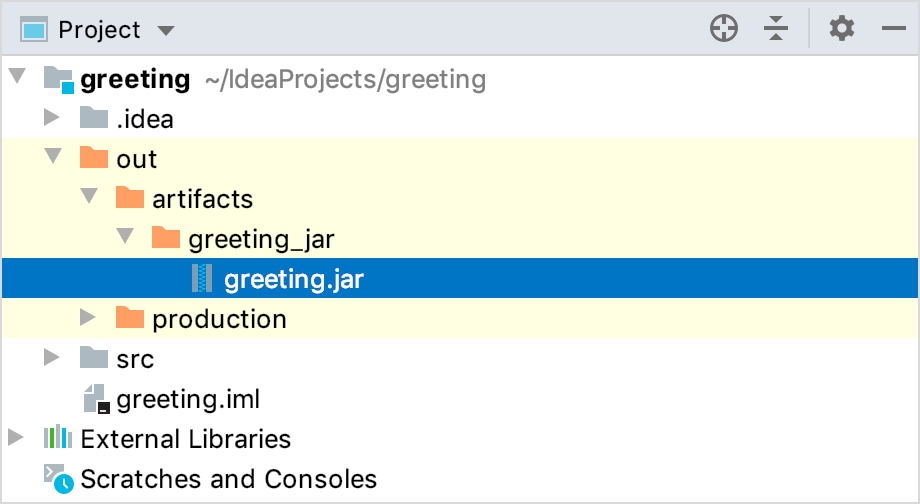
Run the JAR
Run from IntelliJ IDEA
In the Project tool window, right-click the .jar file and select Run.
This is a quick way to run a .jar file from IntelliJ IDEA.
Run from CLI
Open the terminal and from the containing folder, run:
java -jar consoleApp.jar
java -jar greeting.jar
java -jar greeting-1.0-SNAPSHOT.jar
This is how the users of your application would run it.