Reactive Streams
Reactive Streams is a specification for asynchronous stream processing with non-blocking back pressure. IntelliJ IDEA provides support for the following non-blocking reactive Java frameworks based on Reactive Streams:
The support for Reactive applications includes code completion, inspections, quick-fixes, and a dedicated debug mode.
Add Reactor support
There are several options for adding Reactor support to your project: you can add the necessary dependencies to the build file of Maven or Gradle projects, or you can manually download the Reactor library. For Spring Boot applications, IntelliJ IDEA allows you to add the required dependencies when you create a new project.
Add Reactor to a Maven project
For Maven projects, it's recommended that you import the Bill of Materials (BOM) together with the core, as it ensures that Reactor components work well together.
In the
pom.xml
file, add the following dependency to import the BOM:<dependencyManagement> <dependencies> <dependency> <groupId>io.projectreactor<groupId> <artifactId>reactor-bom<artifactId> <version>Dysprosium-SR1<version> <type>pom</type> <scope>import<scope> <dependency> <dependencies> <dependencyManagement>Add the following dependency to import Reactor:
<dependencies> <dependency> <groupId>io.projectreactor<groupId> <artifactId>reactor-core<artifactId> <dependency> <dependencies>
Add Reactor to Gradle (Gradle 5.0 and later)
For Gradle projects, it's recommended that you import the Bill of Materials (BOM) together with the core, as it ensures that Reactor components work well together.
In the
build.gradle
, add the following dependencies:dependencies { // import BOM implementation platform('io.projectreactor:reactor-bom:Dysprosium-SR1') // add dependencies without a version number implementation 'io.projectreactor:reactor-core' }
Add Reactor to Gradle (Gradle 4.x and earlier)
For Gradle projects, it's recommended that you import the Bill of Materials (BOM) together with the core, as it ensures that Reactor components work well together.
Previous Gradle versions don't support the BOM, however, you can use the Spring gradle-dependency-management plugin to import it to your project.
Obtain the plugin from Gradle Plugin Portal by adding the following code to
build.gradle
:plugins { id "io.spring.dependency-management" version "1.0.6.RELEASE" }Import the BOM by adding the following code:
dependencyManagement { imports { mavenBom "io.projectreactor:reactor-bom:Dysprosium-SR1" } }Add Reactor support:
dependencies { compile 'io.projectreactor:reactor-core' }
Add the Reactor library to a project
If you build your project with the native IDE builder, you can add Reactor support as a library.
In the main menu, go to
Ctrl+Alt+Shift+S or clickon the toolbar.
Select Libraries, click
, and then select From Maven.
In the dialog that opens, specify the library artifact
io.projectreactor:reactor-core:3.1.06.RELEASE
and click OK.
Create a new Spring Boot project with Reactor
Launch IntelliJ IDEA.
If the Welcome screen opens, click New Project.
Otherwise, go to
in the main menu.Select Spring Initializr from the left pane.
Click
to enter the URL of the service that you want to use, or leave the default one.
Specify a name and location for your project and configure project metadata: select a language, a build tool, and specify an artifact ID.
From the JDK list, select the JDK that you want to use in your project.
If the JDK is installed on your computer, but not defined in the IDE, select Add JDK and specify the path to the JDK home directory.
If you don't have the necessary JDK on your computer, select Download JDK.
If you want to build your project on a Java version different from your project JDK version, you can select it here.
Click Next.
On the next step of the wizard, from the Dependencies list, select Spring Reactive Web and click Create.
Reactor debug mode
Reactor includes assembly-time instrumentation that is designed for debugging asynchronous code. When you enable the Reactor debug mode, you can get a more convenient view of the stack trace after the program has been suspended. You can examine the frames and understand why particular parameters were passed to a method in a Reactive application.
IntelliJ IDEA is aware of the Reactor debug mode and shows the traceback to the failed operation on the Frame tab of the Debug tool window. Moreover, IntelliJ IDEA can enable the debug mode without any changes in your code, making the necessary calls as you run the debugging session.
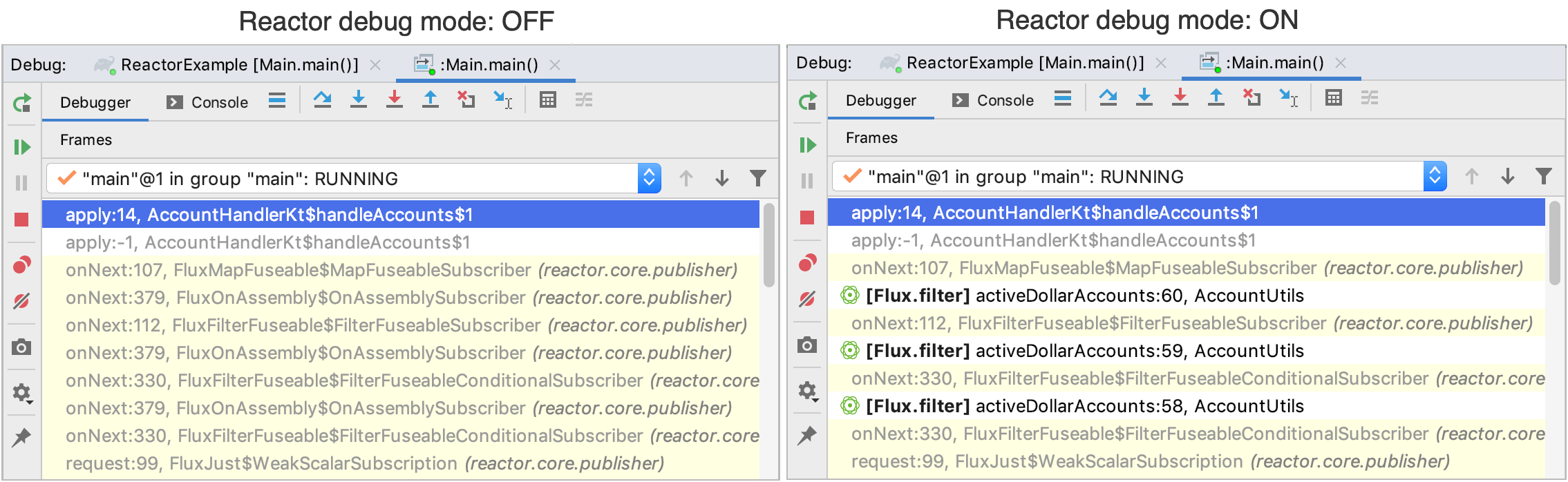
By default, the Reactor debug mode is enabled. This is convenient during development, testing, and debugging, but generally impacts the performance of the application.
Configure the Reactor debug mode
Press Ctrl+Alt+S to open settings and then select
.Enable the Enable Reactor Debug mode option and select one of the debug initialization methods:
Hooks.onOperatorDebug(): This method captures the stack trace on every operator, which is slow and requires a lot of resources. Do not use this method in production. For more information, refer to Activating Debug Mode.
ReactorDebugAgent.init(): Use this Java agent to debug exceptions in your application without much runtime impact. For more information, refer to Production-ready Global Debugging.
Additionally select Notify if unable to call ReactorDebugAgent.init() if you want to show a notification when the agent is not available.
None: Select this option if you don't have global debugging enabled and use the
checkpoint()
operator in your code for a more fine-grained approach. For more information, refer to The checkpoint() Alternative.
Enable the Render Mono/Flux instances as lazy computed value option to evaluate Mono and Flux values in the debugging session. This will enable you to configure the following parameters:
Maximum number of Flux elements to fetch at once: specify the maximum number of elements to retrieve in a single get operation from a Flux (when clicking
collectList()
in the debugging session).Stop Mono/Flux evaluation after timeout: the maximum time to wait for the future to complete.
Apply the changes and close the dialog.
Subscribe to Mono or Flux in Debug mode
When debugging your reactive application, you can evaluate values of Mono and Flux by subscribing to the corresponding publisher in the debugging session.
Set a breakpoint at a method, which returns Mono or Flux.
Start the debugging session: click
on the toolbar or press Shift+F9.
In the Debug tool window that opens, select the Debugger tab.
If you have a Mono object, click
get()
next to it. This will subscribe you to the publisher and fetch the Mono value using thetoFuture().get()
method.If you have a Flux object, click
collectList()
next to it. This will subscribe you to the publisher and fetch the stream elements using thecollectList().toFuture().get()
method. The number of fetched elements is limited to 100. Once the first 100 elements are displayed, you can double-click the end of the list to get more. This limit can be configured in .
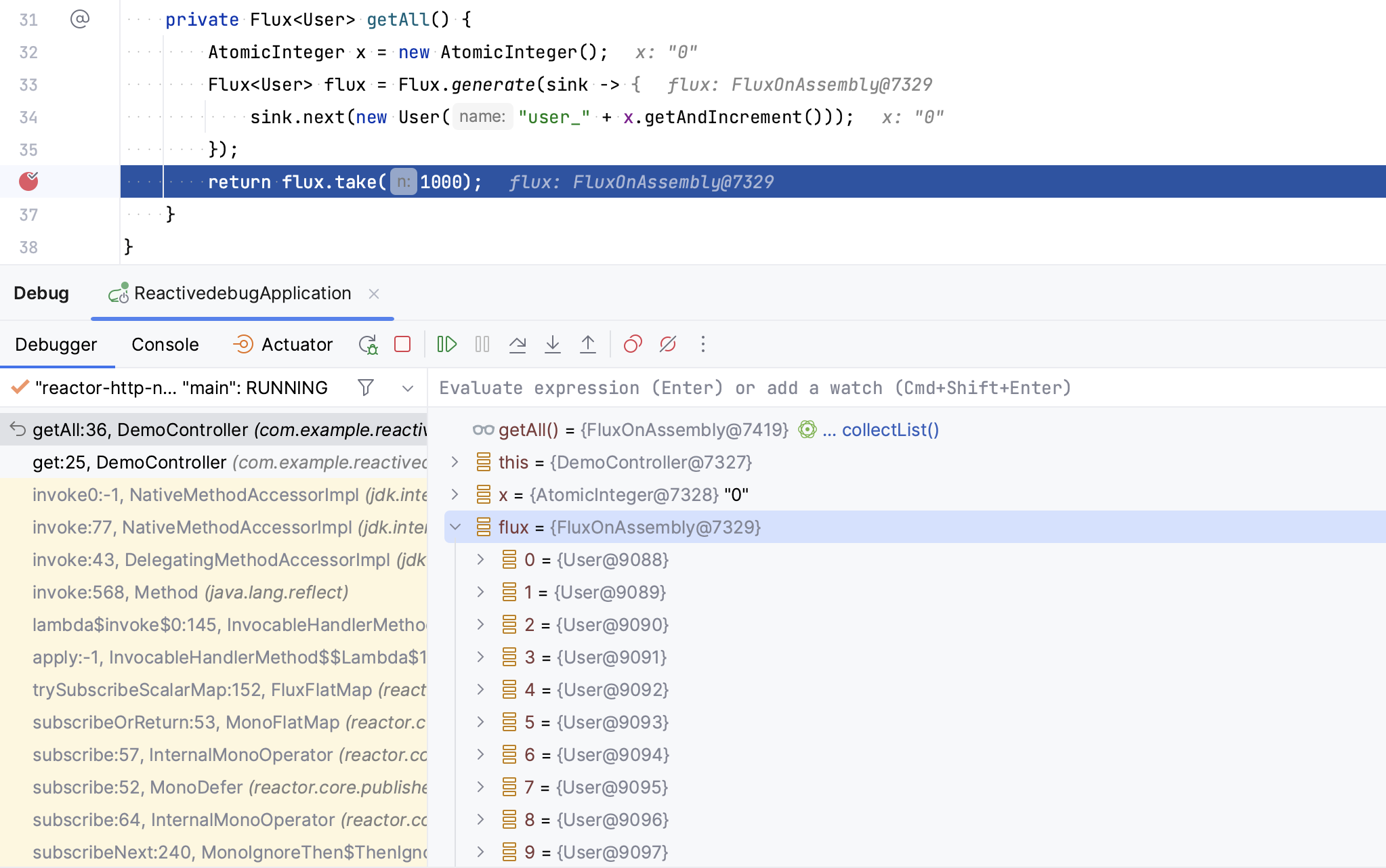
Advanced Reactor inspection
IntelliJ IDEA includes the Possibly blocking call in non-blocking context inspection that detects inappropriate thread-blocking method calls in code fragments where a thread should not be blocked. Reactor support also adds an option that enables IntelliJ IDEA to locally understand on which thread the operator will be executed by processing the subscribeOn()
and publishIn()
operators.
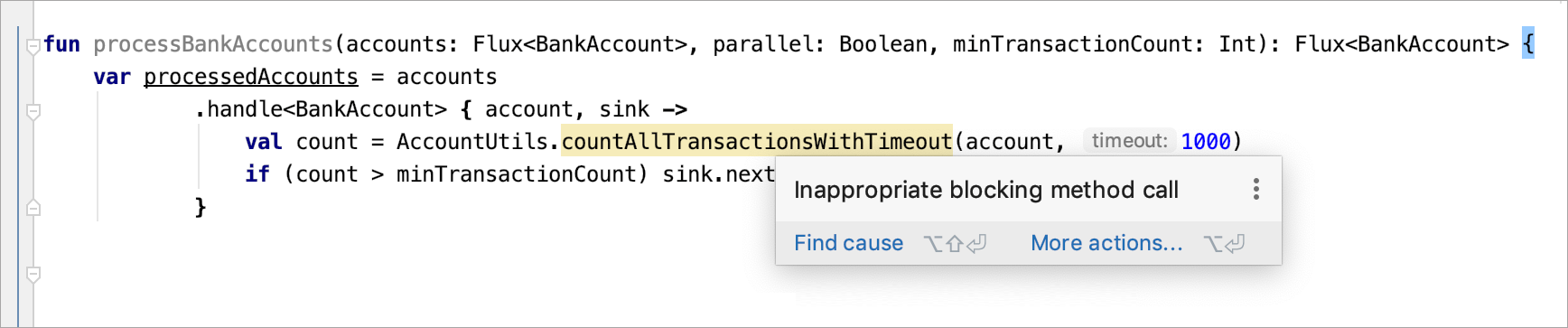
The option is enabled by default, and if you want to disable it, do the following:
Press Ctrl+Alt+S to open settings and then select
.Clear the Use advanced analysis to detect non-blocking scopes checkbox and click OK to apply the new settings.
@Blocking and @NonBlocking annotations
The JetBrains annotations collection includes the @Blocking
and @NonBlocking
annotations that help IntelliJ IDEA detect blocking calls in non-blocking contexts, such as Kotlin coroutines or reactive code with Project Reactor or RxJava.
Add org.jetbrains:annotations
version 22.0.0 or later to your project's dependencies. IntelliJ IDEA also supports the corresponding annotations from Micronaut and SmallRye Mutiny.
Postfix completion in Reactor
IntelliJ IDEA provides postfix code completion for projects that use Reactor. Postfix completion can transform an already-typed expression to a different one based on what you have typed.
In projects with Reactor support, the IDE can wrap an expression with a suitable reactor.core.publisher.Flux
factory or a reactor.core.publisher.Mono
factory.
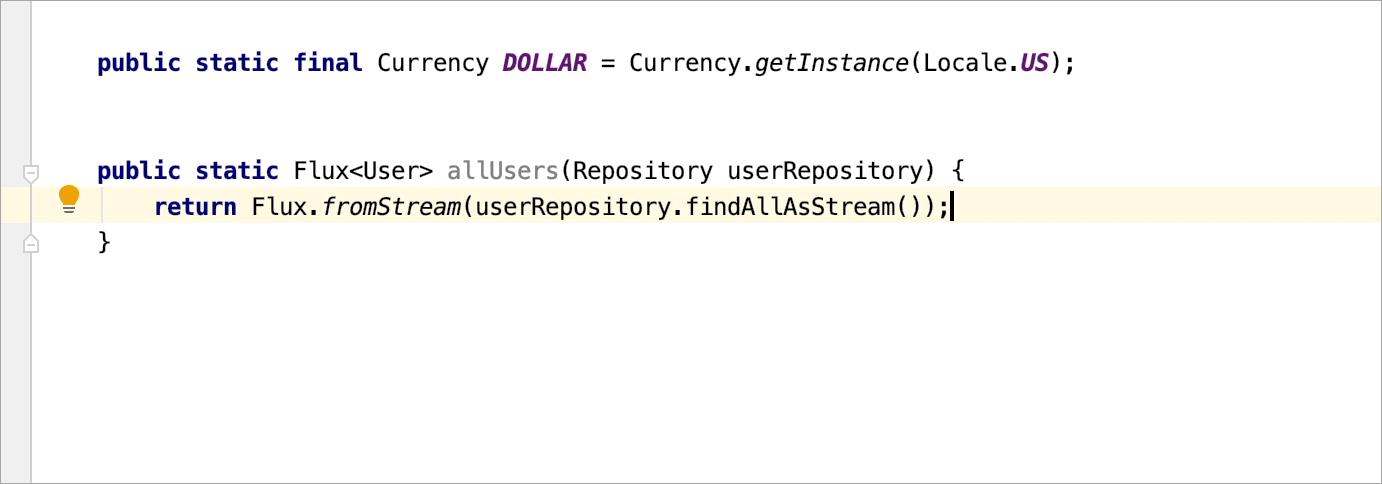
Video tutorial
The following video demonstrates how to create a working Reactive Spring Boot application in IntelliJ IDEA: