Tutorial: Run a Java application
This tutorial explains how to quickly run a Java application. It also covers the setup required to run a Java application, such as creating a new project, configuring the JDK, and setting up run configurations with various options.
Create a new project
Launch IntelliJ IDEA.
If the Welcome screen opens, click New Project. Otherwise, go to in the main menu.
From the list on the left, select Java. Name the new project.
Select the following project options:
Build system: Maven
Add sample code: enabled
From the JDK list, select the latest available Oracle OpenJDK version.
If the JDK is installed on your computer, but not defined in the IDE, select Add JDK and specify the path to the JDK home directory.
If you don't have the necessary JDK on your computer, select Download JDK.
Click Create.
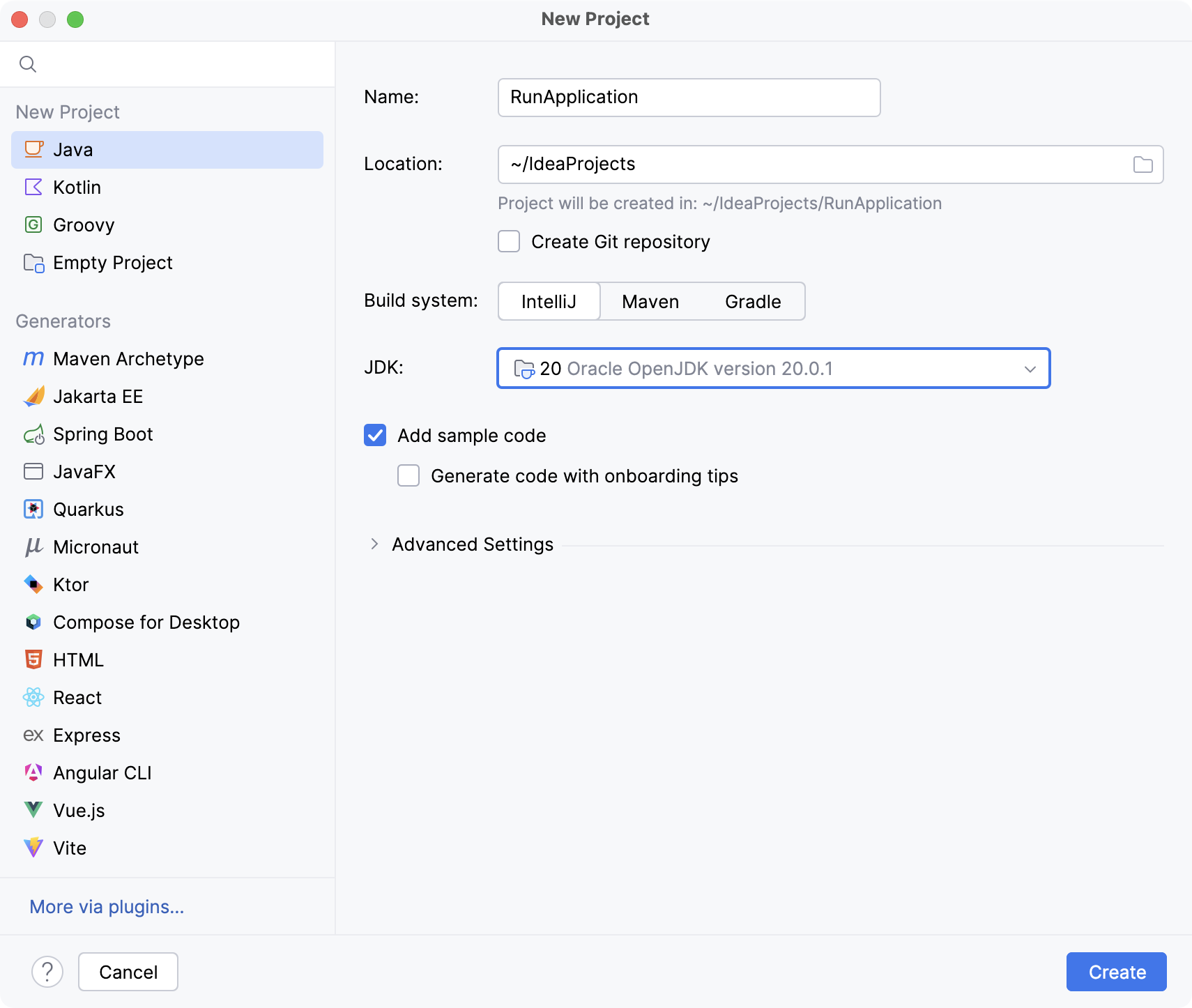
Run the application
When the project is created, in the Project tool window (Alt+1), locate the file and open it in the editor.
Replace the existing code with the following code sample:
package org.example; import java.util.stream.Stream; public class Main { public static void main(String[] args) { Stream.iterate(1, i -> i + 1) .limit(10) .forEach(System.out::println); } }In the editor, click the
gutter icon to run the application and select Run 'Main.main()'.
IntelliJ IDEA runs your code. After that, the Run tool window opens at the bottom of the screen.
The application has run successfully, that is why you will see the Process finished with exit code 0
message in the output.
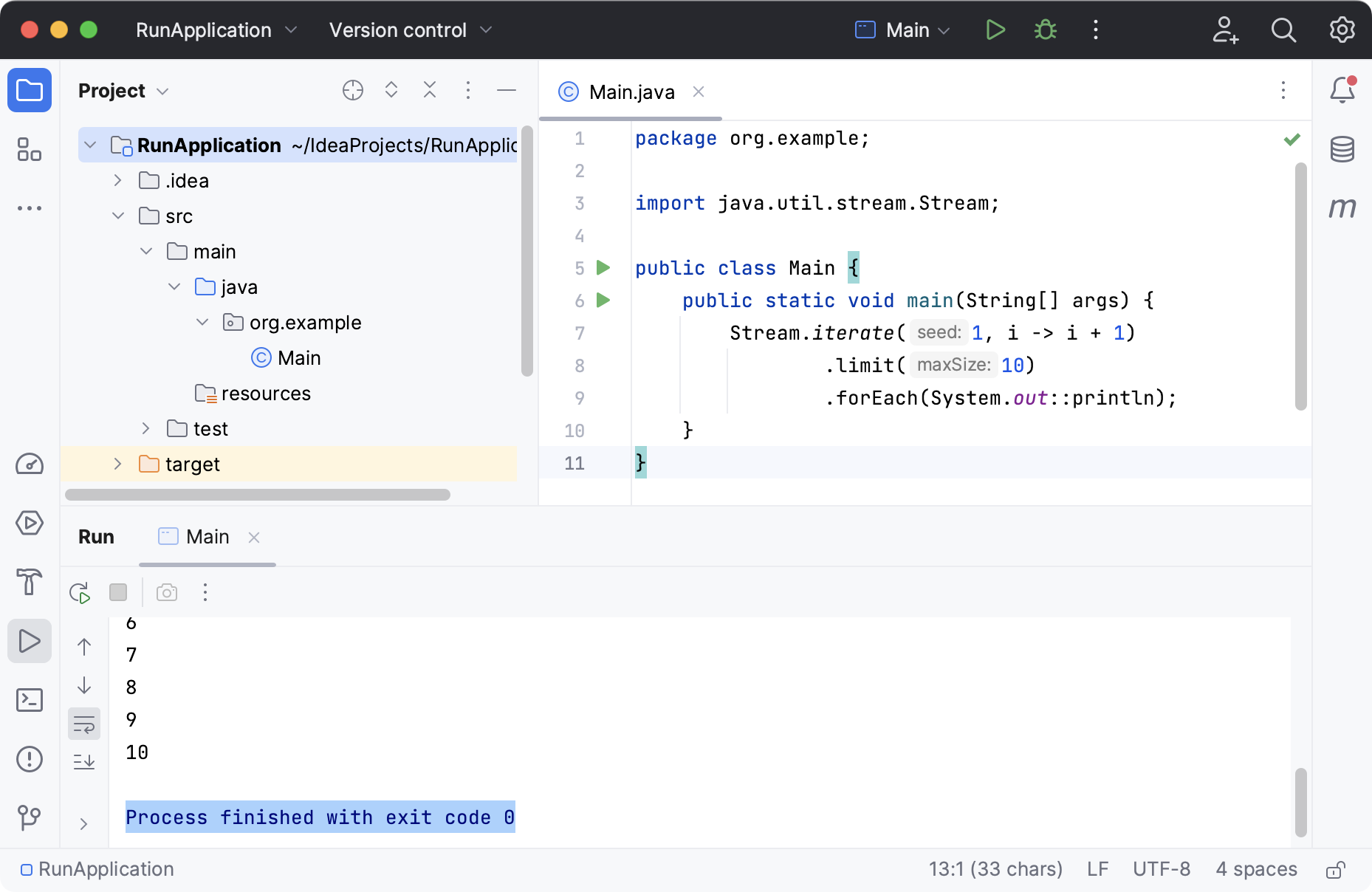
When you clicked Run, IntelliJ IDEA created a temporary run configuration named after the Main
class. The number of temporary configurations is limited to 5 by default, so the older ones are automatically deleted when new ones are added. That is why it is makes sense to save the temporary configurations that you want to keep.
Save the run configuration
In the main menu, go to
.In the area on the left, select the
Main
configuration, and then click theicon on the toolbar above.
The icons of temporary configurations are semi-transparent. So, as soon as the configuration is saved, its icon will become brighter.
Do not close the dialog yet.
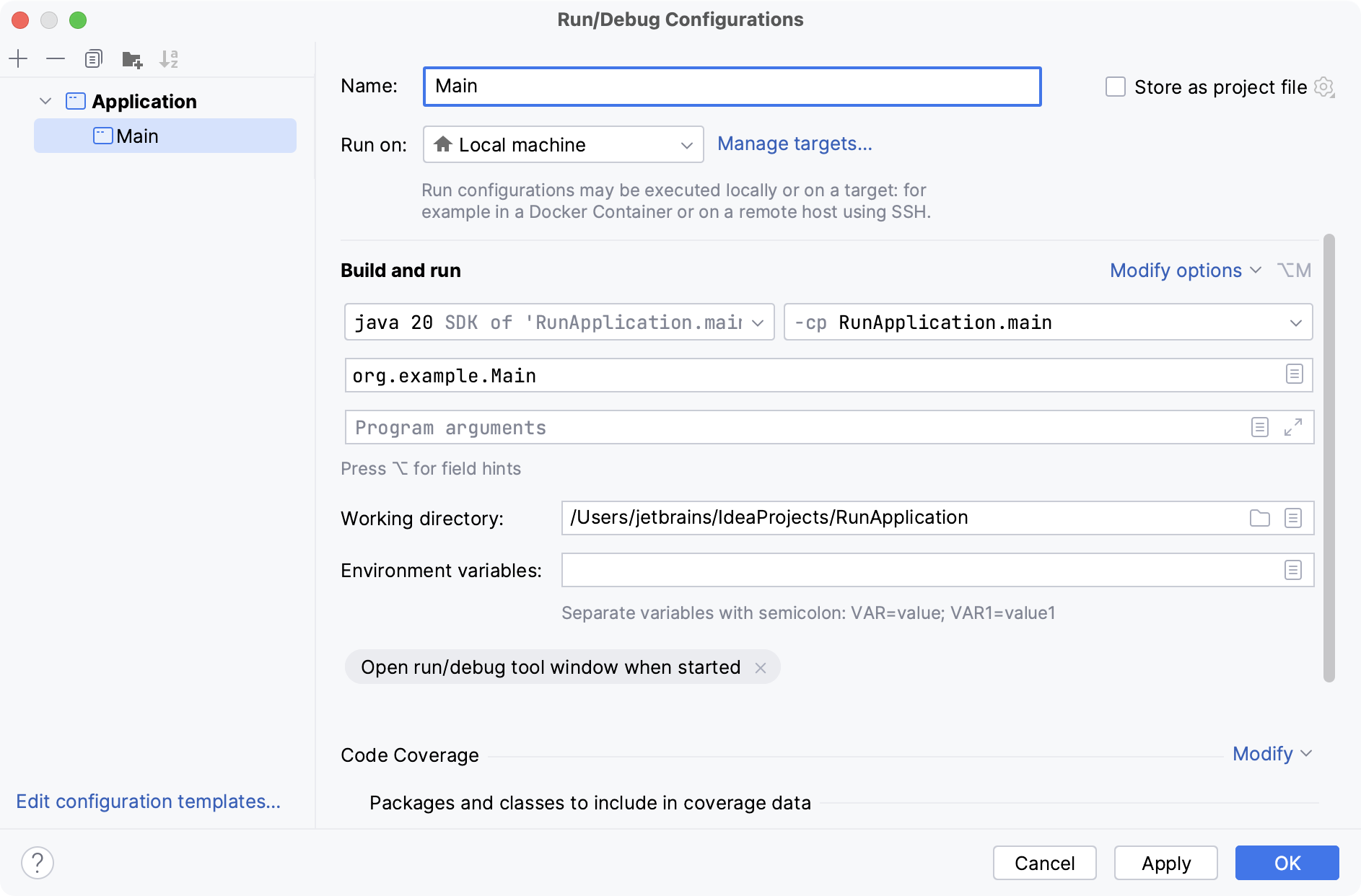
Save console output to a file
Now let's copy this configuration and modify it so that the IDE saves the console output to a file every time you run the configuration. This might be useful if you use console output for logging.
In the area on the left, click the
Main
configuration and then clickon the toolbar.
This will create a copy of the run configuration.
In the area on the right, rename the configuration to
SaveConsoleOutput
.Click Modify options and from the Logs settings group, select Save console output to file.
Specify the path to the file to which the IDE will write the output. If the file does not exist, it will be created automatically.
In our case, we will create a file in the project directory, so the path that we specify is
/Users/jetbrains/IdeaProjects/RunApplication/console.txt
.Apply the changes and close the dialog.
The new Save console output to file field appears in the dialog.
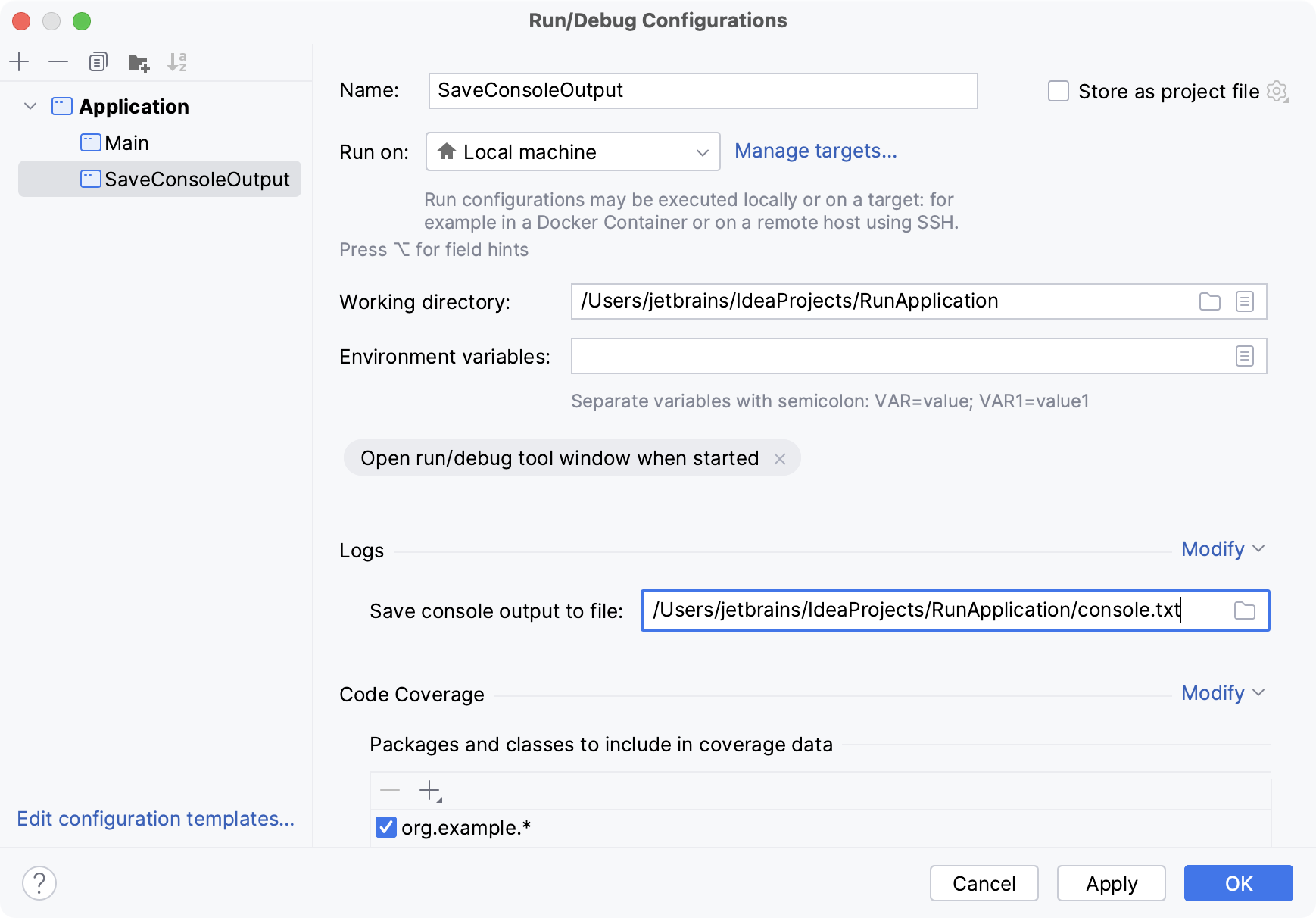
Run the saved configuration
Make sure that
SaveConsoleOutput
is selected in the Run widget in the window header and clicknext to it or press Shift+F10.
When the IDE finishes running the configuration, locate the new file with the console output in the Project tool window and make sure it has content.
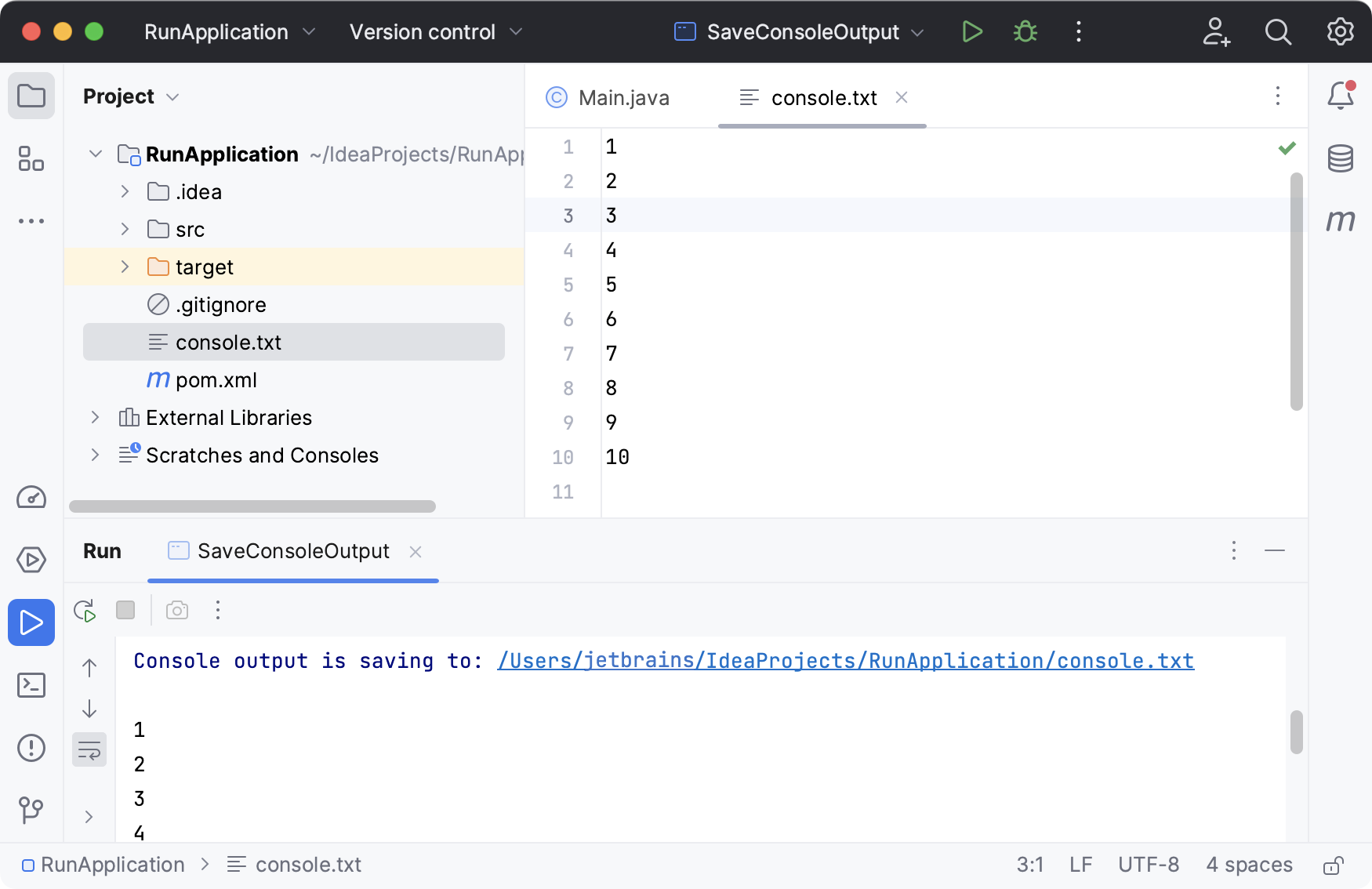
Run configurations allow you to run the same application with different parameters. Now that you have two configurations, you can choose between them according to your needs. For example, if you do not need to save the console output every single time you run the application, you can run the Main
configuration that does not have this setting.
Press Alt+Shift+F10 or use the Run widget in the window header to switch between configurations:
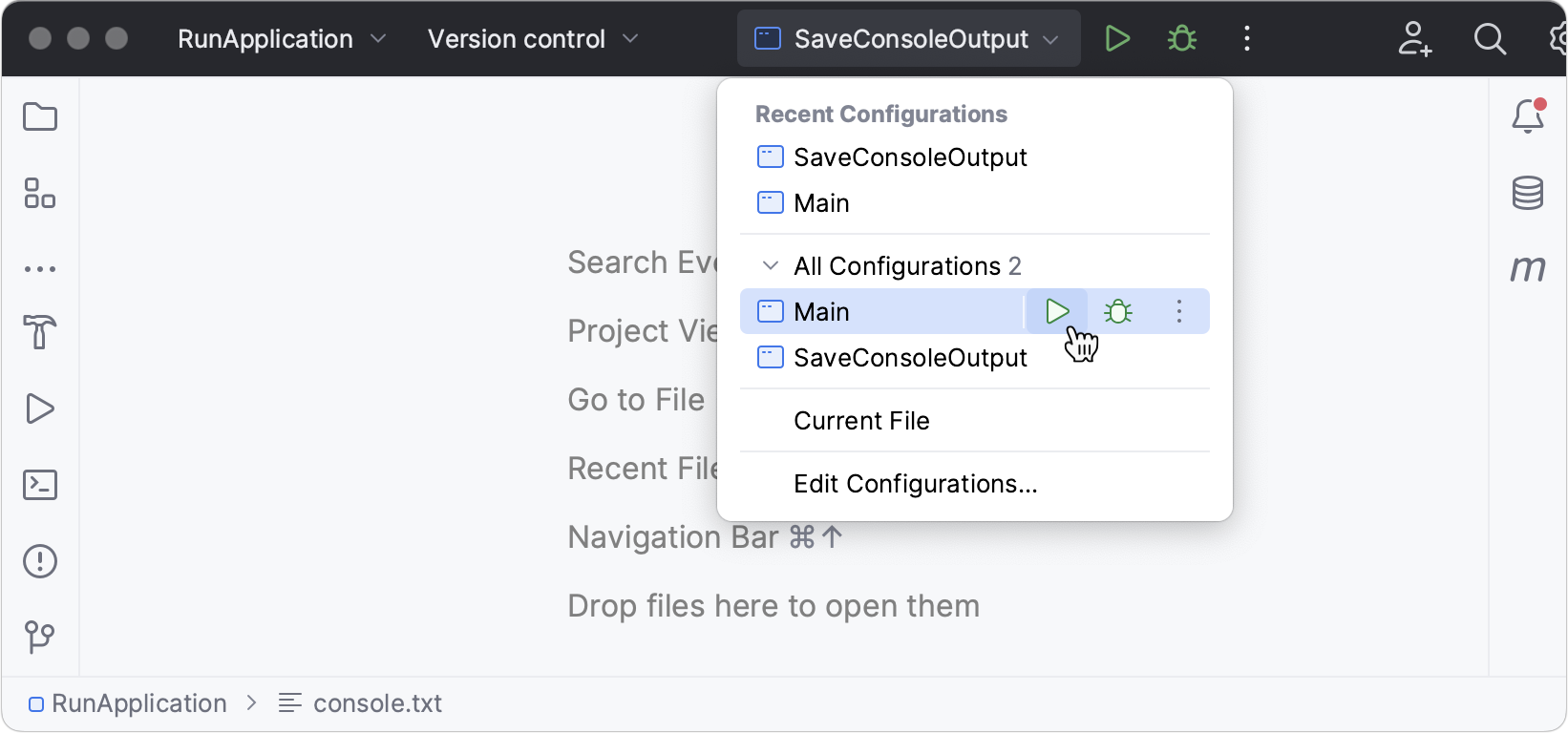
Let's take a look at another scenario.
Change the code
Imagine that our code sample has a problem.
In the Main.java file, remove:
Stream.iterate(1, i -> i + 1) .limit(10) .forEach(System.out::println);Instead, paste the following code:
var list = Stream.iterate(1, i -> i + 1) .toList(); System.out.println(list.size());In the editor, click the
gutter icon to run the application and select Run 'Main.main()'.
The application hangs for several seconds and then fails with OutOfMemoryError
. Our program declares an infinite stream of integers and then tries to convert it into a list using the toList()
method. Since the stream is infinite, the toList()
method will never return, and the program will keep running indefinitely, consuming system resources.
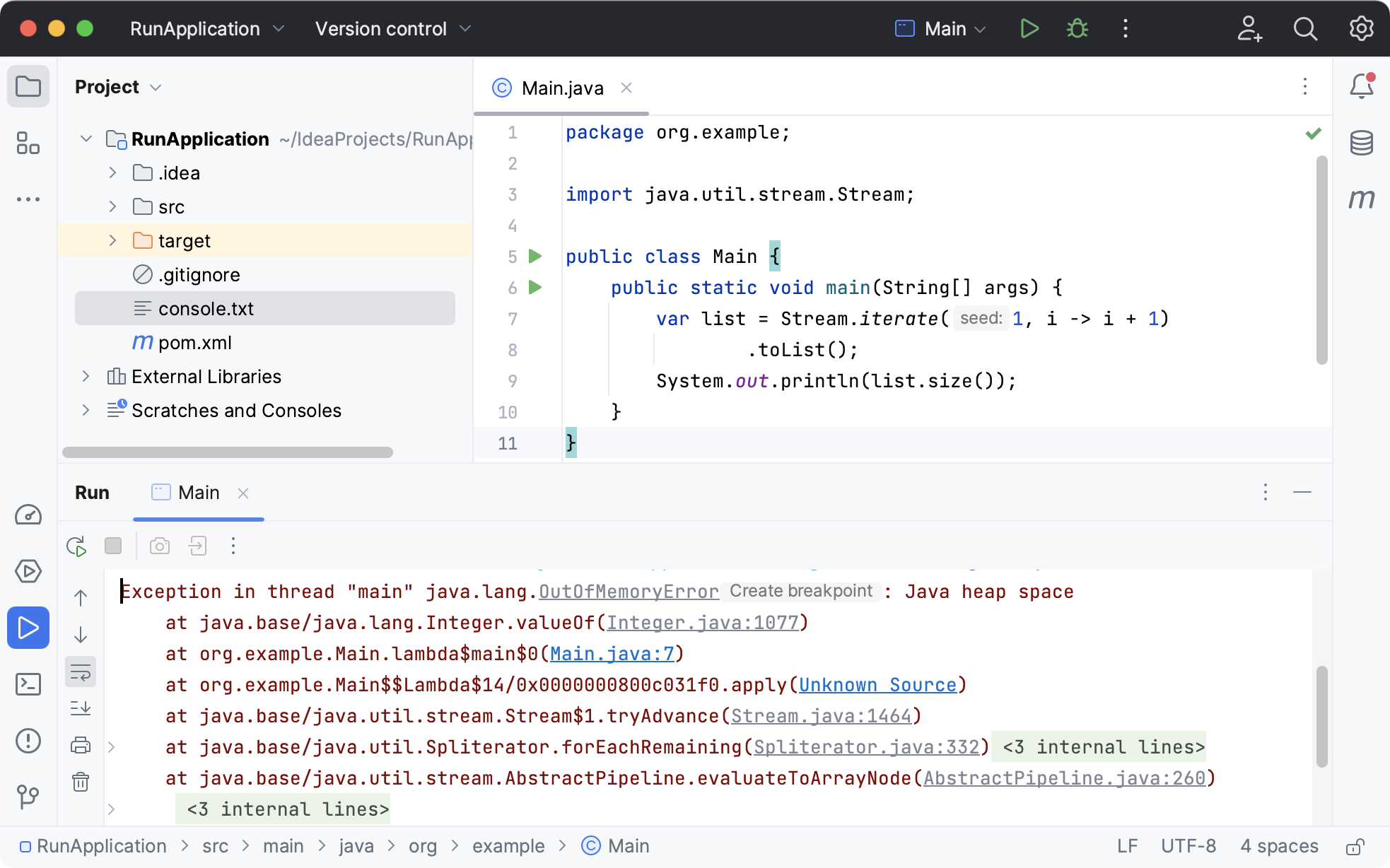
In this case, we can add a VM option that will create an .hprof file for us if our application fails with OutOfMemoryError
. Later on, we will be able to analyze this file in detail using the built-in profiler.
This is useful if you do not know the reason for OutOfMemoryError
and would like to investigate it further.
Add the VM options
In the main menu, go to
.In the area on the left, click the
Main
configuration and then clickon the toolbar to duplicate the configuration.
Rename the configuration to
OutOfMemory
.Open the Modify options list and click Add VM options.
The VM Options field appears in the dialog. In this field, add the following options with a space between them:
-Xmx512m -XX:+HeapDumpOnOutOfMemoryError
-XX:+HeapDumpOnOutOfMemoryError
creates an .hprof file, and-Xmx512m
limits the size of the dump.Apply the changes and close the dialog.
Make sure that the
OutOfMemory
configuration is selected in the Run widget and clicknext to it or press Shift+F10.
The Run tool window opens showing you that the OutOfMemoryError
exception has been thrown. Since we have configured the corresponding VM option, the IDE has created an .hprof file in your project directory.
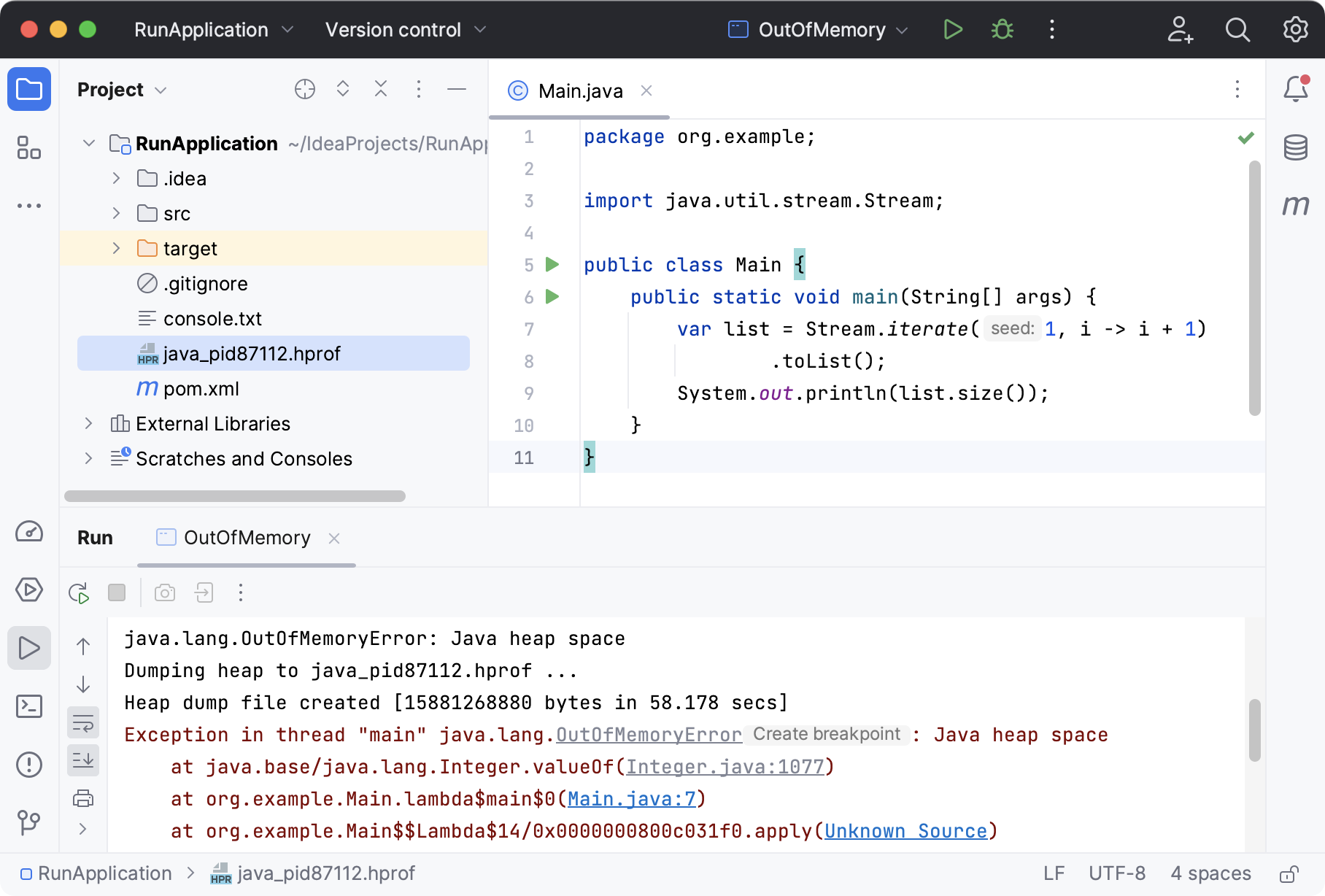