Integration with the UIKit framework
Compose Multiplatform is interoperable with the UIKit framework. You can embed Compose Multiplatform within an UIKit application as well as embed native UIKit components within Compose Multiplatform. This page provides examples both for using Compose Multiplatform inside a UIKit application and for embedding UIKit components inside Compose Multiplatform UI.
Use Compose Multiplatform inside a UIKit application
To use Compose Multiplatform inside a UIKit application, add your Compose Multiplatform code to any container view controller. This example uses Compose Multiplatform inside the UITabBarController
class:
With this code, your application should look like this:
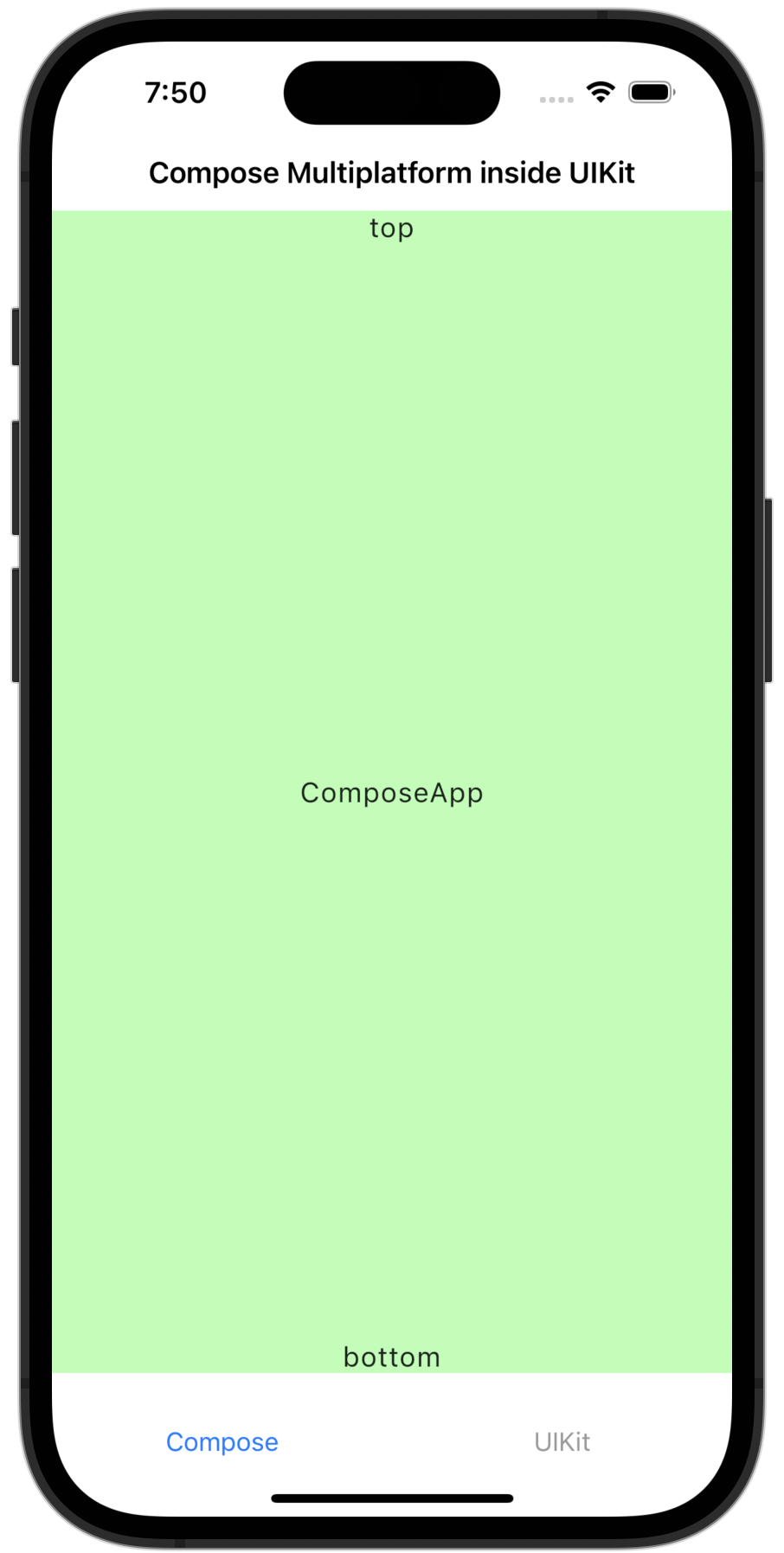
Explore this code in the sample project.
Use UIKit inside Compose Multiplatform
To use UIKit elements inside Compose Multiplatform, add the UIKit elements that you want to use to a UIKitView from Compose Multiplatform. You can write this code purely in Kotlin or use Swift as well.
In this example, UIKit's MKMapView
component is displayed in Compose Multiplatform. Set the component size by using the Modifier.size()
or Modifier.fillMaxSize()
functions from Compose Multiplatform:
With this code, your application should look like this:
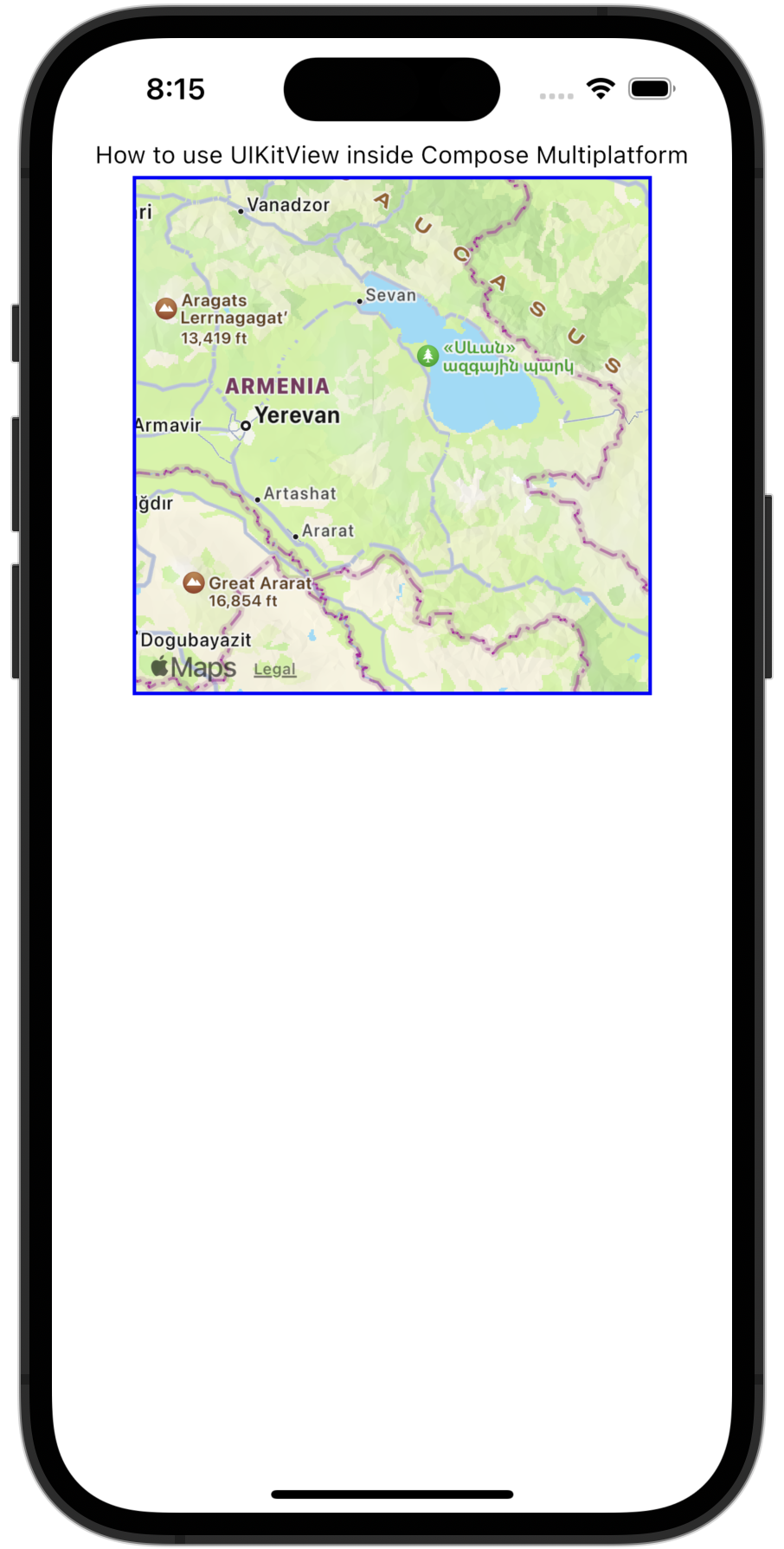
Now, let's look at an advanced example. This code wraps UIKit's UITextField
in Compose Multiplatform:
The factory parameter contains the editingChanged()
function and the textField.addTarget()
listener to detect any changes to UITextField
. The editingChanged()
function is annotated with @ObjCAction
so that it can interoperate with Objective-C code. The action
parameter of the addTarget()
function later on passes the name of the editingChanged()
function, so it would be called in response to a UIControlEventEditingChanged
event.
The update
parameter of UIKitView()
is called when the observable message state changes its value:
The function updates the text
attribute of the UITextField
so that the user sees the updated value.
Explore the code for this example in this sample project.
What's next
You can also explore the way Compose Multiplatform can be integrated with the SwiftUI framework.