Step 1. Creating and Running Your First Python Project
Before you start
Make sure that the following prerequisites are met:
You are working with PyCharm CE or Professional .
You have installed Python itself. If you’re using macOS or Linux, your computer already has Python installed. You can get Python from python.org.
Choosing interpreter
Choosing which interpreter to use for a project is an important decision. Python is a script language, which means that your code is converted to machine code by a Python interpreter.
You can have multiple versions of Python installed on your computer, and you need to choose the one you’d like for this project. See the Configuring Python Interpreter section for details. Note that you can always change your mind later and specify another interpreter for your project.
Creating a virtual environment
When you’re using external libraries (from PyPI or elsewhere), you need to manage the versions of these as well. The Pythonic solution for this are virtualenvs (sometimes abbreviated to venv). Virtualenvs help you keep the dependencies for your different projects separate. Although in this project we’re not using any dependencies, it’s considered best practice to create a virtualenv for every new project you make, in case you’d like to add dependencies in the future.
Creating a simple Python script in PyCharm
To get started with PyCharm, let’s write a Python script.
Let’s start our project: if you’re on the Welcome screen, click Create New Project. If you’ve already got a project open, choose .
PyCharm suggests several project templates for the creation of the various types of applications (Django, Google AppEngine,, etc.). When PyCharm creates a new project from a project template, it produces the corresponding directory structure and specific files, and any needed run configurations or settings.
In this tutorial we’ll create a simple Python script, so we’ll choose Pure Python. This template will create an empty project for us.
Choose the project location. To do that, click button next to the Location field, and specify the directory for your project.
Python best practice is to create a virtualenv for each project. To do that, expand the Project Interpreter: New Virtualenv Environment node and select a tool used to create a new virtual environment. Let's choose Virtualenv tool, and specify the location and base interpreter used for the new virtual environment. Select the two check boxes below if necessary.
Then click the Create button at the bottom of the New Project dialog.
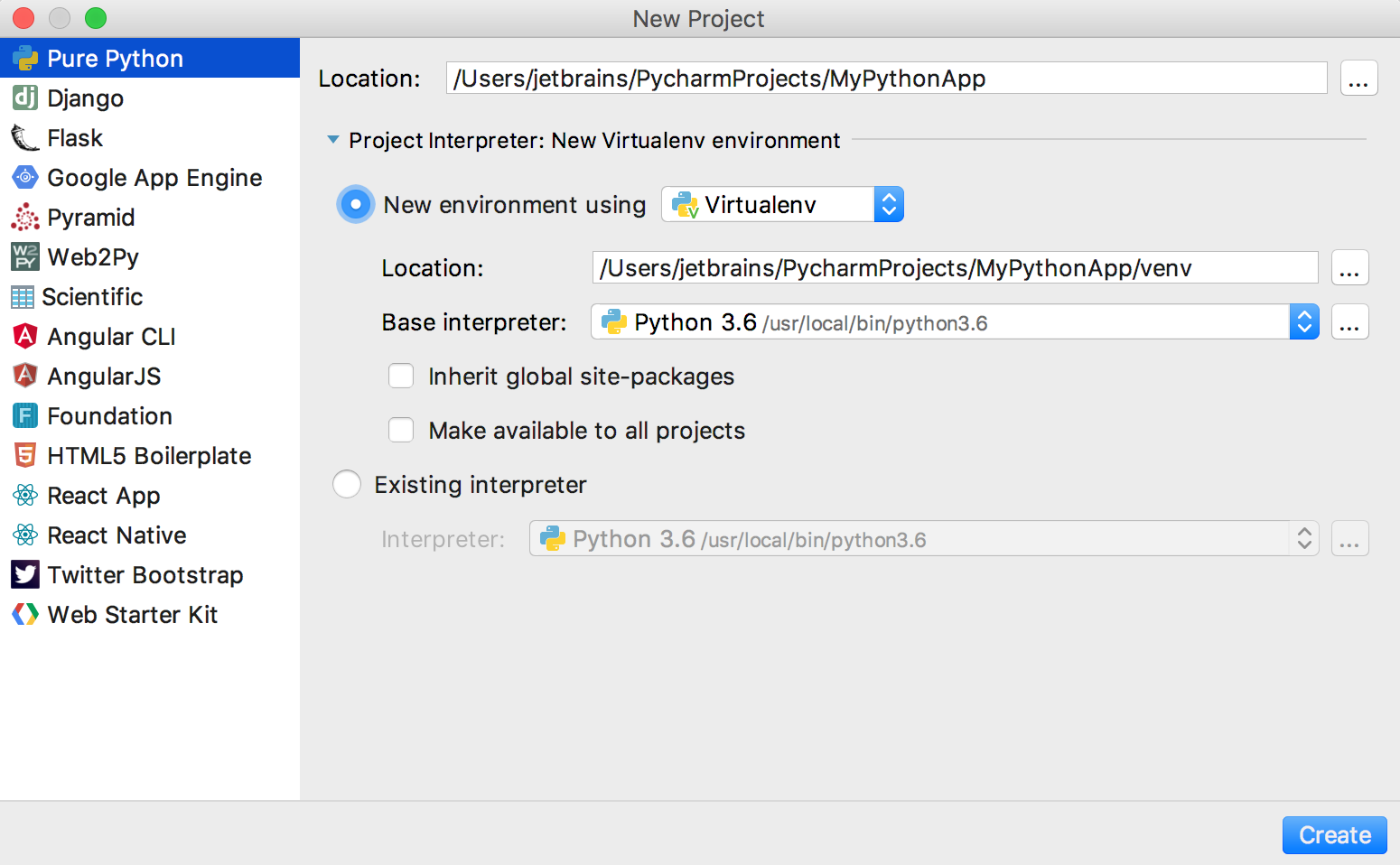
If you’ve already got a project open, after clicking Create PyCharm will ask you whether to open a new project in the current window or in a new one. Choose Open in current window - this will close the current project, but you'll be able to reopen it later. See the page Opening Multiple Projects for details.
Creating a Python file
Select the project root in the Project tool window, and press Alt+Insert.
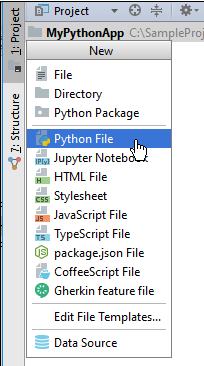
Choose the option Python file from the pop-up window, and then type the new file name Car
.
PyCharm creates a new Python file and opens it for editing.
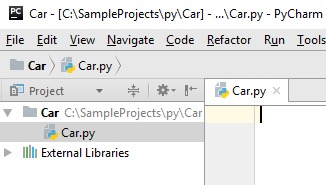
Editing source code
Let's first have a look at the Python file we've just generated.
Immediately as you start typing, you should see that PyCharm, like a pair-programmer, looks over your shoulder and suggests how to complete your line. For example, you want to create a Python class. As you just start typing the keyword, a suggestion list appears:
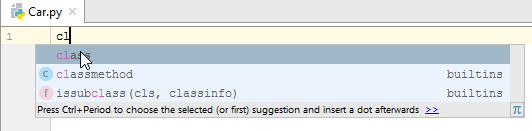
Choose the keyword class
and type the class name (Car
here).
PyCharm immediately informs you about the missing colon, then expected indentation:
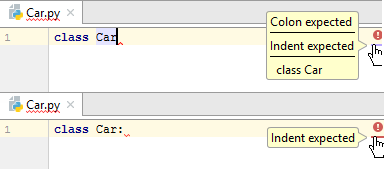
Note the stripes in the right gutter. Hover your mouse pointer over a stripe, and PyCharm shows a balloon with the detailed explanation.
Since PyCharm analyses your code on-the-fly, the results are immediately shown in the inspection indicator on top of the right gutter. This inspection indication works like a traffic light: when it is green, everything is OK, and you can go on with your code; a yellow light means some minor problems that however will not affect compilation; but when the light is red, it means that you have some serious errors.
Let's continue creating the function __init__
: when you just type the opening brace, PyCharm creates the entire code construct (mandatory parameter self
, closing brace and colon), and provides proper indentation:
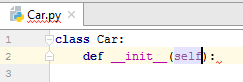
For the example, let's use this code: (you can either type it yourself, or use the copy button in the top right of the code block here in the help):
class Car:
def __init__(self):
self.speed = 0
self.odometer = 0
self.time = 0
def say_state(self):
print("I'm going {} kph!".format(self.speed))
def accelerate(self):
self.speed += 5
def brake(self):
self.speed -= 5
def step(self):
self.odometer += self.speed
self.time += 1
def average_speed(self):
return self.odometer / self.time
if __name__ == '__main__':
my_car = Car()
print("I'm a car!")
while True:
action = input("What should I do? [A]ccelerate, [B]rake, "
"show [O]dometer, or show average [S]peed?").upper()
if action not in "ABOS" or len(action) != 1:
print("I don't know how to do that")
continue
if action == 'A':
my_car.accelerate()
elif action == 'B':
my_car.brake()
elif action == 'O':
print("The car has driven {} kilometers".format(my_car.odometer))
elif action == 'S':
print("The car's average speed was {} kph".format(my_car.average_speed()))
my_car.step()
my_car.say_state()
Running your application
You can right-click the editor, and on the context menu choose to run the script (Ctrl+Shift+F10), but we suggest a better solution: since our script contains a main function, there is an icon in the left gutter. If you hover your mouse pointer over it, the available commands show up:
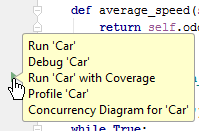
If you click this icon, you'll see the pop-up menu of the available commands. Choose Run Car:
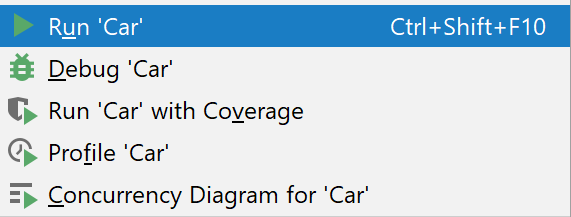
A console appears in the Run tool window.
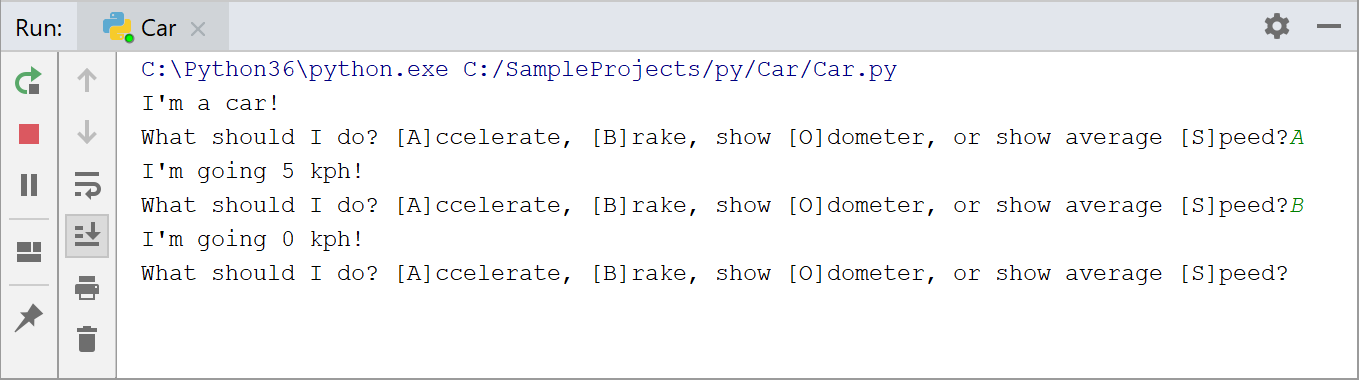
See the sections under Running node for more details about configuring how your code is executed by PyCharm.
Run/debug configuration
When we run the script just now, PyCharm created a temporary run/debug configuration for us. Let’s first save this configuration: go to the run configuration dropdown on the top-right of the editor, and choose Save configuration.
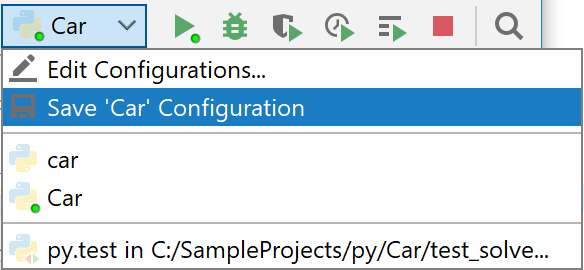
Afterwards, choose Edit Configurations to have a look at what is happening here.
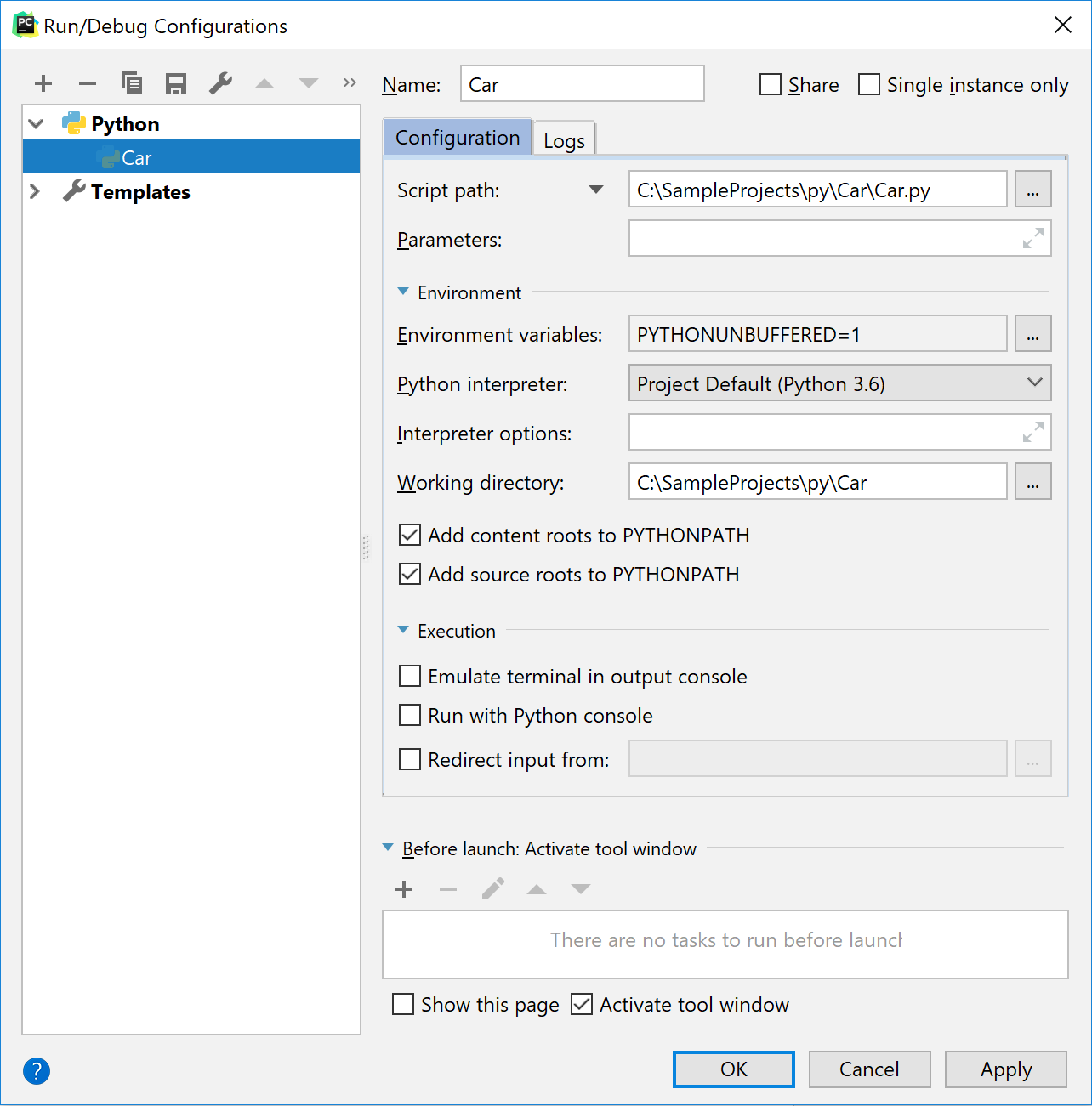
If you’d like to change how your program is executed by PyCharm, this is where you can configure various settings like: command-line parameters, work directory, and more. See Run/Debug configurations for more details.
If you’d like to start the script using this Run configuration, use the button next to the dropdown.
Summary
Congratulations on completing your first script in PyCharm! Let's repeat what you've done with the help of PyCharm:
Created a project.
Created a file in the project.
Created the source code.
Ran this source code.
Saved the run/debug configuration.
In the next step, learn how to debug your programs in PyCharm.