Test-Driven Development with Twisted
Introduction
Here we'll take the tutorial "Test-Driven Development with Twisted", located at this address, and try to repeat it using PyCharm.
Creating project, packages and Python files
Go to New Project dialog, choose to create a pure Python project. Let's call it Calculus
.
Create an empty directory called calculus containing an empty __init__.py file. To do that, go to in the main menu, and then choose Python Package from the New popup menu.
Next, create a Python file. To do that, again go to Python File in the popup. This file is called base_1.py and it opens for editing immediately. Enter the following code:
in the main menu, and chooseNext, right-click the package calculus and create a Python package again – this time it should be called test.
Installing packages
You must install the Twisted package to execute tests. Besides that, if you are working on Windows, also install the package pypiwin32.
It's most easy to install the required packages in PyCharm: to do it, go to Settings dialog and open the Packages toolbar section, click and select the required packages in the Available Packages dialog. For more information, refer to Install, uninstall, and upgrade packages.
Selecting the required test runner
Again In the Settings dialog (Ctrl+Alt+S) , under the Tools node, click the Python Integrated Tools page.
On this page, select the test runner Twisted Trial from the list in the Default test runner field.
Apply the changes and close the dialog.
Creating a failing test
Right-click the file base_1.py next to the class declaration, and press Ctrl+Shift+T or choose from the context menu. In the Create Test dialog you'll have to make some changes to the default settings:
First, click the browse button next to the Target directory field, and choose test package.
Second, in the Test filename field, enter the name test_base_1.py, and in the Test class name field, enter the name TestBase_1
. Finally, select all methods – thus the tests for all the methods of the tested class will be included.
You should get the following code:
This is a working class, but we are writing tests for Twisted... Let's import the package. You'll end up with the following code:
As the idea of TDD (Test-Driven Development) is writing tests before the code, let's make the actual tests:
By the way, look at the Project tool window – it shows the structure of the project. At the moment, you have 4 files:
calculus/__init__.py
calculus/base_1.py
calculus/test/__init__.py
calculus/test/test_base_1.py
Running the failing tests
Now let's run this test. To do that, open for editing the test file test_base_1.py, right-click the editor background and from the context menu choose Run 'Twisted Trial for test_base_1.CalculationTestCase'.
As expected, all tests failed:
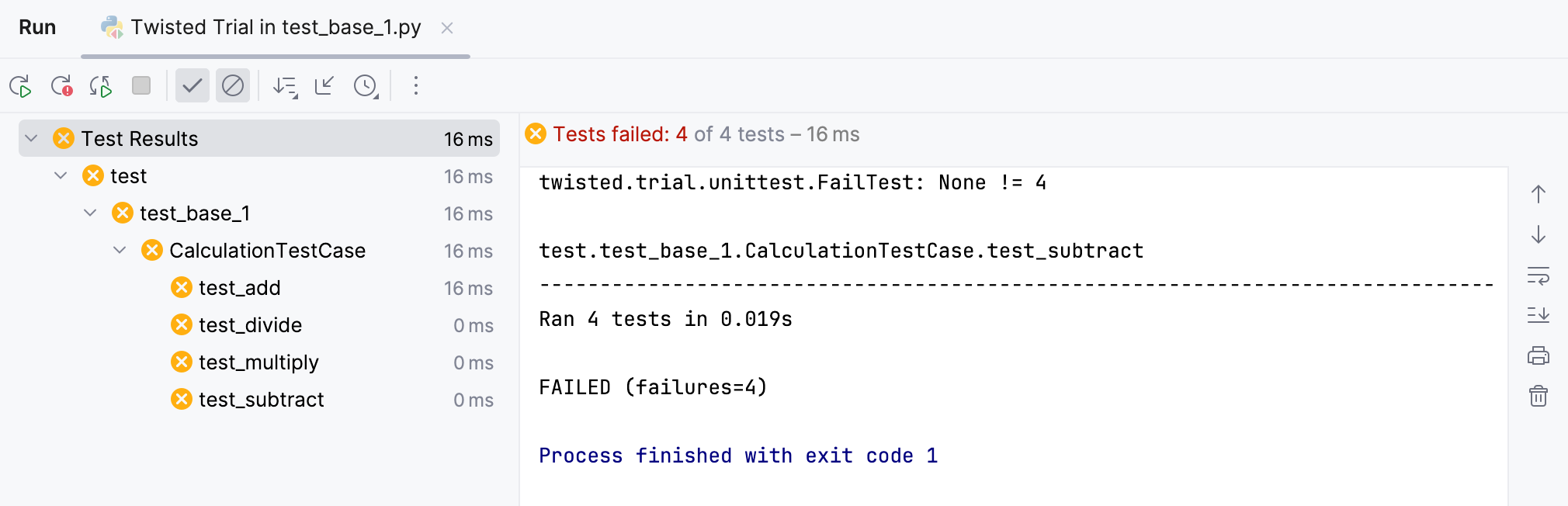
Note that in the Project tool window appears one more directory - _trial_temp. It contains the log file:
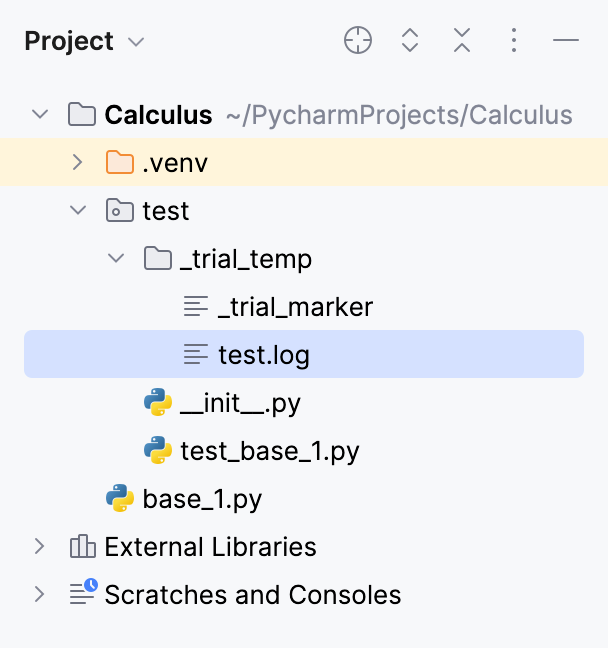
Making tests pass
Let's change the class being tested and make the tests pass. To do that, we'll change the base_1.py script. The code of the file will look as follows:
Let's run test_base_1.py again. To do it, right-click the editor background and choose Run 'Twisted Trial for test_base_1.CalculationTestCase'. This time the tests pass:
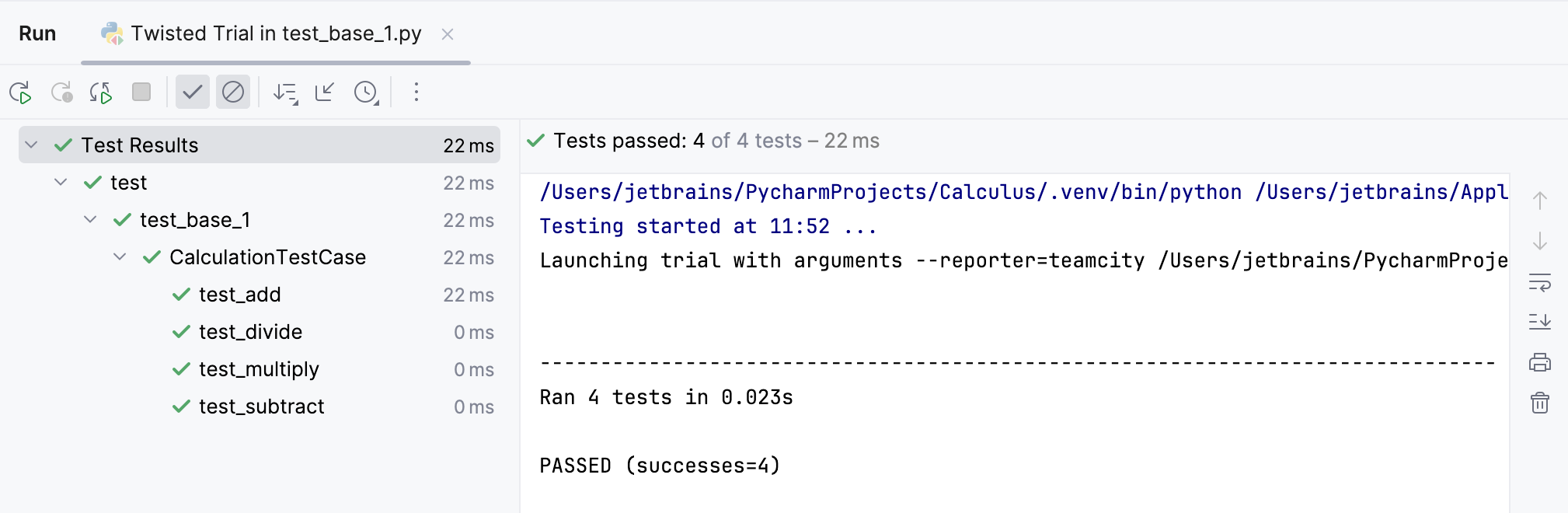