Configure an interpreter using Docker Compose
Enable the Django plugin
This functionality relies on the Django plugin, which is bundled and enabled in PyCharm by default. If the relevant features aren't available, make sure that you didn't disable the plugin.
Press Ctrl+Alt+S to open the IDE settings and then select
.Open the Installed tab, find the Django plugin, and select the checkbox next to the plugin name.
Prerequisites
Make sure that the following prerequisites are met:
You have stable Internet connection, so that PyCharm can download and run
busybox:latest
(the latest version of the BusyBox Docker Official Image). Once you have successfully configured an interpreter using Docker, you can go offline.Docker is installed. You can install Docker on the various platforms, but here we'll use the macOS installation.
Note that you might want to repeat this tutorial on different platforms; then use Docker installations for Windows and Linux (Ubuntu, other distributions-related instructions are available as well).
Before you start working with Docker, make sure that the Docker plugin is enabled. The plugin is bundled with PyCharm and is activated by default. If the plugin is not activated, enable it on the Plugins page of the IDE settingsĀ Ctrl+Alt+S as described in Install plugins.
In the Settings dialog (Ctrl+Alt+S) , select , and select Docker for <your operating system> under Connect to Docker daemon with. For example, if you are on macOS, select Docker for Mac. See more detail in Docker settings.
Note that you cannot install any Python packages into Docker-based project interpreters.
Prepare an example
Let's use a Django application with a PostgreSQL database running in a separate container.
On the Welcome screen, click Get from VCS.
In the Get from Version Control dialog, specify the URL of the remote repository and click Clone.
PyCharm suggests creating a virtual environment based on the project requirements recorded in the
file.Click OK to create an environment.
Another hint that IDE provides is detecting a data source. Click Connect to Database to set up the database.
You might need to download missing drivers and check the rest of the configuration options:
With this done, your example is ready, and you can start configuring docker containers.
For this Django application, let's create two containers: one for the database, and one for the application itself. The Docker Compose will link the two containers together.
Add files for Docker and Docker Compose
In the Project tool window, right-click the project root and select from the context menu. Enter the filename: Dockerfile.
Copy and paste the following code in the Dockerfile file:
FROM python:3.6.7 WORKDIR /app # By copying over requirements first, we make sure that Docker will cache # our installed requirements rather than reinstall them on every build COPY requirements.txt /app/requirements.txt RUN pip install -r requirements.txt # Now copy in our code, and run it COPY . /app EXPOSE 8000 CMD ["python", "manage.py", "runserver", "0.0.0.0:8000"]Then right-click the project root again, select docker-compose.yml file.
from the context menu, and create theCopy and paste the following code into the docker-compose.yml file.
version: '2' services: web: build: . ports: - "8000:8000" volumes: - .:/app links: - db db: image: "postgres:9.6" ports: - "5432:5432" environment: POSTGRES_PASSWORD: hunter2This file defines two services:
web
anddb
, and links them together.
Configure Docker
Now that you have prepared the example, let's configure Docker.
Press Ctrl+Alt+S to open the IDE settings and then select
.Click
to create a Docker server. Accept the suggested default values:
If you installed Docker in a custom location, you may need to specify the paths to the Docker CLI executables.
Click OK to save changes.
Configure Docker Compose as a remote interpreter
Let's now define a remote interpreter based on Docker Compose.
Do one of the following:
Click the Python Interpreter selector and choose Add New Interpreter.
Press Ctrl+Alt+S to open Settings and go to . Click the Add Interpreter link next to the list of the available interpreters.
Click the Python Interpreter selector and choose Interpreter Settings. Click the Add Interpreter link next to the list of the available interpreters.
Select On Docker Compose.
Select Docker configuration in the Server dropdown.
Specify the docker-compose.yml file in Configuration files and select the service.
Optionally, specify environment variables and edit the Compose project name.
Wait until PyCharm creates and configures a new target:
Select an interpreter to use in the container. You can choose any virtualenv or conda environment that is already configured in the container, or select a system interpreter.
Click OK.
The configured remote interpreter is added to the list.
Use the Docker tool window
When you have configured Docker, the Services tool window appears at the bottom of PyCharm's main window. You can click docker-compose up in the gutter next to the
services
group to launch db
and web
services.
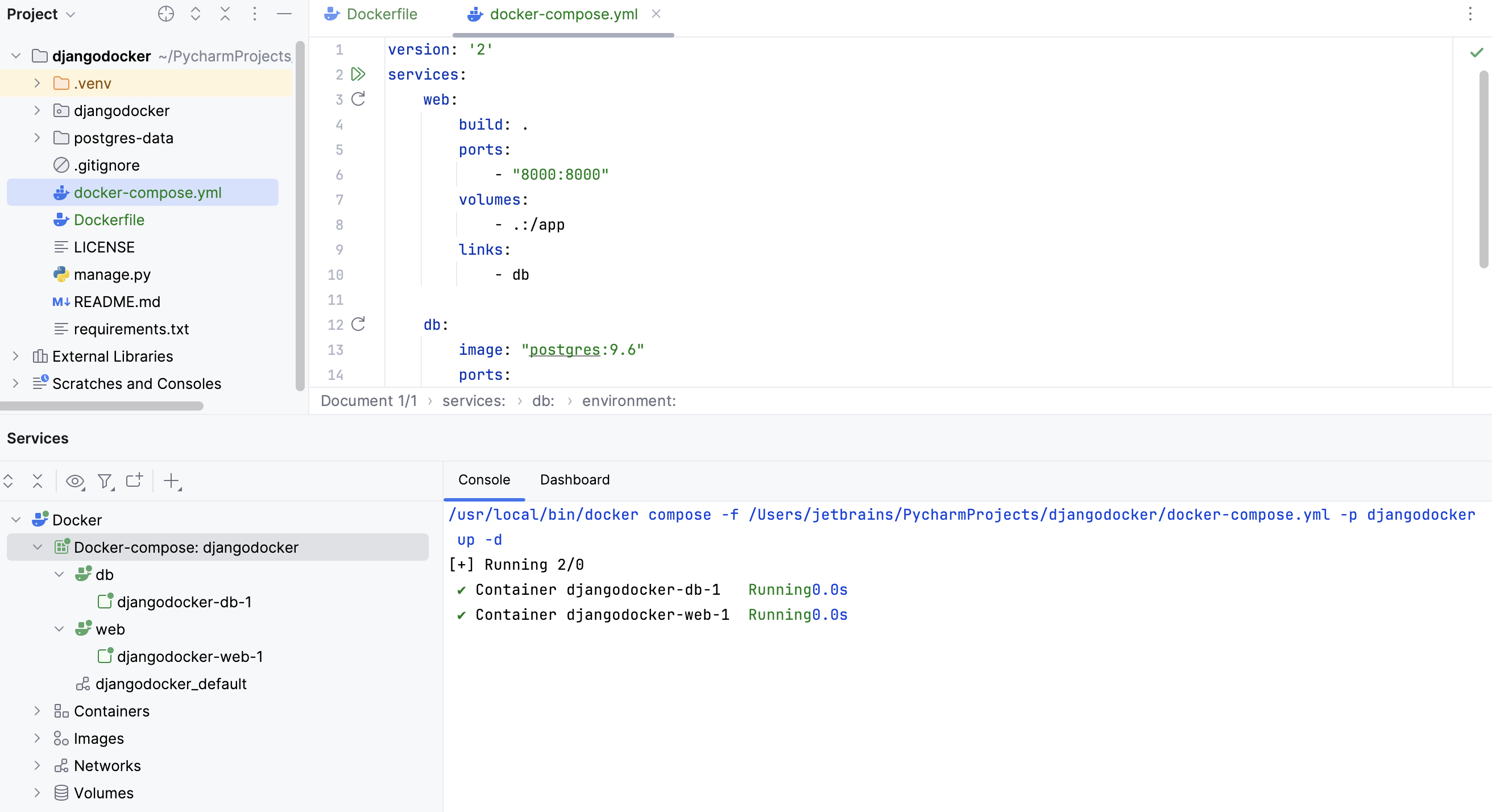
Refer to Docker for more details on managing docker containers in the Services tool windows.
Configure database credentials
Modify the DATABASES
section of the settings.py file in your Django project to add database configuration details:
Run the application
Select
from the main menu and entermigrate
to run a migration and execute a Django application.Create a Run/Debug configuration for the Django server. To do that, select
from the main menu.In the Run/Debug Configurations dialog, click
Add New Configuration and select Django Server.
Set the Host field to 0.0.0.0 to ensure that the Docker container is open to external requests. To allow the server to start at this address, add
0.0.0.0
to theALLOWED_HOSTS
list in settings.py.To run the newly created configuration, select
from the main menu.
To see the output in your web browser, go to http://localhost:8000:
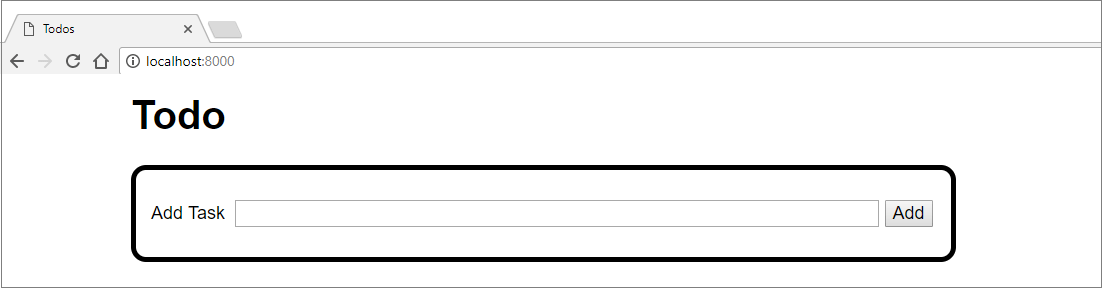
Debug the application
With the Run/Debug configuration created, you can also debug your application.
Set a breakpoint (for a Django application, you can set it in a template).
Do one of the following:
Select
from the main menu.Click
Debug next to the Run/Debug configuration drop-down with the
RunDjangoApp
configuration selected.
For more information about debugging application in a container, refer to Debugging in a Docker container.