Generative completion
One of the easiest way to generate code with ReSharper is to use code completion — all you need to do is to type several characters. Code generation suggestions are included in the lists of automatic completion and basic completion.
Here are a few examples of how you can generate code with code completion.
Overriding and implementing members
In the example below, code completion helps create an override for a virtual member from a base class. Start typing the base method name in a derived type, and you will get a suggestion to override it:
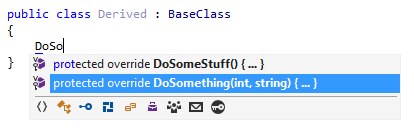
After you accept the suggestion, the method body with the default implementation expands in the editor:
Properties for fields
To generate properties for a field, start typing the name of the field. ReSharper will suggest creating a read-only or a read-write property with the corresponding name according to your naming style:
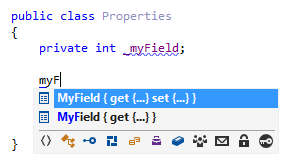
After you accept the suggestion, the property body expands in the editor:
Type constructors
Depending on the existing members of the current type, ReSharper suggests different constructors in the completion list. To create a constructor, type ctor
. In the completion list, you may see the following suggestions:
ctor
— a constructor without parametersctorf
— a constructor that initializes all fieldsctorp
— a constructor that initializes all auto-propertiesctorfp
— a constructor that initializes all fields and auto-properties
In the example below, all kinds of constructors are available.
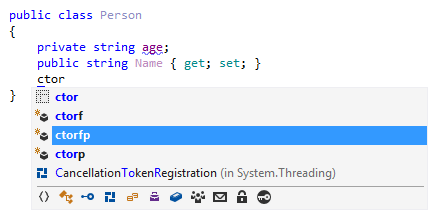
If you accept the ctorfp
suggestion, the constructor expands in the editor:
Generate equality and flag checks for enumeration types
When you need to compare a value of enum type to one of the members of this enum, just type a dot and then pick the desired enum member in the completion list:
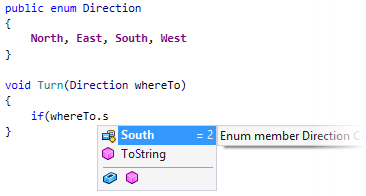
ReSharper will generate the comparison for you:
Templates in completion lists
All your live templates, postfix templates, and source templates appear in the completion list. Templates are identified by their shortcuts (here are the list of shortcuts of predefined templates). You can recognize them by the corresponding icon .
For example, to invoke the public static void Main template, type its shortcut psvm
:
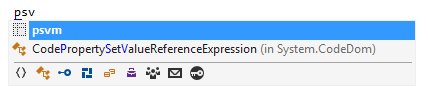
After you accept the suggestion, the Main
method expands in the editor:
When you select items in completion lists using keyboard, the selection will jump to the first item after the last item and vice versa. You can disable this behavior by clearing Loop selection around ends of a list on the page of ReSharper options Alt+R, O.
This feature is supported in the following languages and technologies:
The instructions and examples given here address the use of the feature in C#. For more information about other languages, refer to corresponding topics in the ReSharper by language section.