Make Method Non-Static refactoring
This refactoring allows you to convert a static method into an instance method of the original or another type. The target type for the new instance method can be selected from one of the types passed as parameters to the method. All usages, implementations and overrides of the method are automatically updated.
This refactoring can only be applied to static methods that have at least one parameter of a type defined in the current solution.
In the example below, we use this refactoring to convert a static method Merge
of the Info
class into an instance method of the same type:
In the following example, the static method Merge
that works with Info
objects is defined in the class Service
. We use the refactoring to make Merge
an instance method of the class Info
, where it logically belongs:
Convert a static method into an instance method
Place the caret at the declaration or a usage of a static method in the editor, or select it in the File Structure window window.
Do one of the following:
Press Control+Shift+R and then choose Make Method Non-Static.
Right-click and choose Refactor | Make Method Non-Static from the context menu.
Choose
from the main menu.
The Make Method Non-Static dialog will open.
Select a parameter from the list. The method will be converted to the instance method of the parameter type.
To apply the refactoring, click Next.
If no conflicts are found, ReSharper performs the refactoring immediately. Otherwise, it prompts you to resolve conflicts.
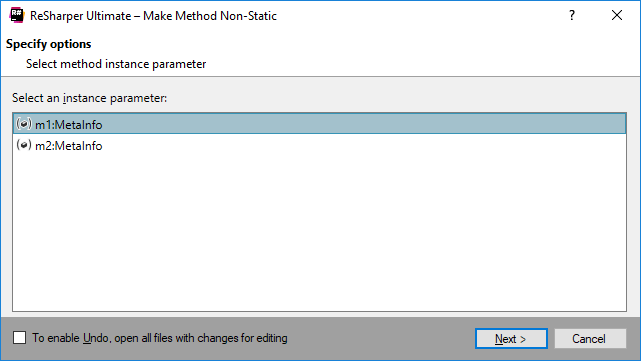
This feature is supported in the following languages and technologies:
The instructions and examples given here address the use of the feature in C#. For more information about other languages, refer to corresponding topics in the ReSharper by language section.