Debug window
When you start a debugger session, the Debug tool window appears. Use this window to control the debugger session, display and analyze the program data (frames, threads, variables, and so on), and perform various debugger actions.
This is a brief overview of the Debug tool window. For general instructions on using tool windows, refer to Tool windows.
To cycle through the tool window tabs, press Alt+Right and Alt+Left.
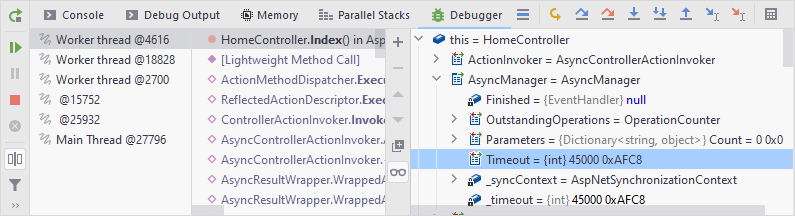
Sessions
If you run several debug sessions simultaneously, they are separated into tabs in the top part of the Debug tool window.
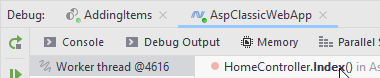
If you enable the Services window for specific run/debug configurations, the entire view of the Debug window will be displayed inside the Services window when you debug any of these configurations.
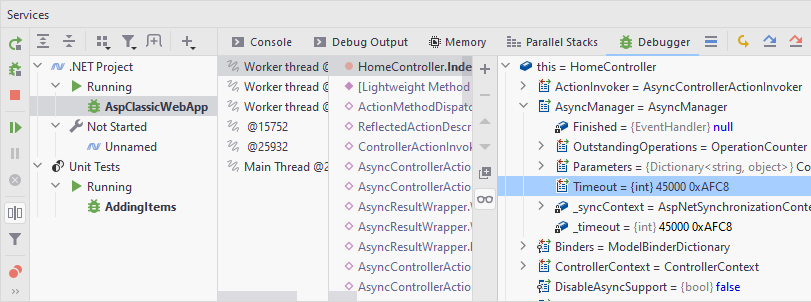
All the information like inline variable values and execution point is shown for the selected session tab. This is important if you are running several debug sessions in parallel that use the same code.
Tabs
The Debug tool window shows the following tabs for each session:
Console: displays the program output.
For local sessions, the tab works the same as when you just run the program without the debugger attached.
When you attach to a process, the program output is not redirected and only the debugger output is shown in the debugger console.
Debug Output: shows messages sent by your application to the debug output, for example with the
Debug.WriteLine()
method.Parallel Stacks: shows threads and their stack frames on the diagram.
Debugger: lets you explore states of objects in the current execution point of the suspended program.
The tab is divided into the following panes:
Threads and frames on the left let you choose the thread and one of its frames that you want to examine.
Variables: displays local variables in the scope of the selected frame. By default, watches are integrated with variables, but you can show watches in a separate pane by clicking Show watches in the variables tab
.
Memory: provides the information on the currently available objects on the heap and lets you monitor and analyze their lifetime.
Show/hide tabs
Click
and select which tabs you want to see.
Restore default layout
If you changed the layout of the Debug tool window and don't like the new arrangement, you can revert it to the default state.
Click
in the top-right corner of the Debug tool window, then click Restore Default Layout.
Debug toolbar
Regardless of the selected tab, you can always use the following toolbar controls in the left part of the window:
Item | Tooltip and Shortcut | Description |
---|---|---|
Rerun Ctrl+F5 | Click this button to stop the current application and run it again. | |
Resume Program F9 | When an application is paused, click this button to resume program execution. | |
Pause Program Ctrl+Pause | Click this button to pause program execution. | |
Stop Ctrl+F2 | Click this button to terminate the current process . | |
View Breakpoints Ctrl+Shift+F8 | Click this button to open the Breakpoints dialog where you can configure breakpoints behavior. | |
Mute Breakpoints | Use this button to toggle breakpoints status. You can temporarily mute all breakpoints in the project to execute the program without stopping at breakpoints. | |
Debugger Settings | Opens the menu with the following options:
| |
Pin Tab | Click this button to pin or unpin the current tab. You may need to pin a tab to prevent it from closing automatically when the maximum number of tabs is reached in this window. |
Item | Tooltip and Shortcut | Description |
---|---|---|
Rerun Ctrl+F5 | Click this button to stop the current application and run it again. | |
Resume Program F9 | When an application is paused, click this button to resume program execution. | |
Pause Program Ctrl+Pause | Click this button to pause program execution. | |
Stop Ctrl+F2 | Click this button to terminate the current process . | |
View Breakpoints Ctrl+Shift+F8 | Click this button to open the Breakpoints dialog where you can configure breakpoints behavior. | |
Mute Breakpoints | Use this button to toggle breakpoints status. You can temporarily mute all the breakpoints in a project to execute the program without stopping at breakpoints. | |
Settings | Click this button to open the menu with the following options:
| |
Pin Tab | Click this button to pin or unpin the current tab. You may need to pin a tab to prevent it from closing automatically when the maximum number of tabs is reached in this window. |
Stepping toolbar
Item | Tooltip and Shortcut | Description |
---|---|---|
Show Execution Point Alt+F10 | Click this button to highlight the current execution point in the editor and show the corresponding stack frame in the Frames pane. | |
Skip to Cursor Ctrl+Alt+Shift+F9 | Instead of running the program up to the target statement, you may want to skip a part of code without actually executing it (for example, to avoid a known bug or not to repeat some routine). You can do this with the Skip to Cursor You can also skip a part of the program by dragging the execution pointer ![]() You can also hover over the target statement and then pick the corresponding action: ![]() Remember the following when you jump to another statement:
| |
Step Over F8 | Click this button to execute the program until the next line in the current method or file, skipping the methods referenced at the current execution point (if any). If the current line is the last one in the method, execution steps to the line executed right after this method. | |
Force Step Over Alt+Shift+F8 | Click this button to have the debugger step over the method even if this method has breakpoints inside. | |
Step Into F7 | Click this button to have the debugger step into the method called at the current execution point. The debugger will set the execution pointer at the first statement of the first function called from the current line. If no function is called, then the debugger will step to the next statement. By default, JetBrains Rider enables external-source debugging, which means that when you step into the library code it will be automatically decompiled and the debugger will move the execution point there. If you want the debugger to ignore the library code, you can disable external-source debugging — clear Enable external source debug on the page of JetBrains Rider settings Ctrl+Alt+S. | |
Smart Step Into Shift+F7 | If the current line contains several nested calls, JetBrains Rider lets you choose a call to step into. Consider the following code line: Console.WriteLine(Foo(Bar("input") + Baz("input"))); The line contains several calls and if you use Step Into, the debugger will first step into the implementation of If you want to choose which of the calls should be stepped into, for example, ![]() When you choose the function to step into, the debugger will set the execution pointer at the first statement of the selected function. The selector also shows you which of the calls were already executed and allows you to execute these calls again. You can enable the 'smart step into' behavior when you invoke Step Into F7 in a line that contains multiple method calls. To do so, select Always do smart step into on the Build, Execution, Deployment | Debugger | Stepping page of settings Ctrl+Alt+S. | |
Step Out Shift+F8 | Click this button to have the debugger step out of the current method, to the line executed right after it. | |
Run to Cursor Alt+F9 | Click this button to resume program execution and pause until the execution point reaches the line at the current caret location in the editor. No breakpoint is required. Actually, there is a temporary breakpoint set for the current line at the caret, which is removed once program execution is paused. Thus, if the caret is positioned at the line which has already been executed, the program will be just resumed for further execution, because there is no way to roll back to previous breakpoints. This action is especially useful when you have stepped deep into the methods sequence and need to step out of several methods at once. If there are breakpoints set for the lines that should be executed before bringing you to the specified line, the debugger will pause at the first encountered breakpoint. You can also hover over the target statement and then pick the corresponding action: ![]() | |
Run to Cursor Non-Stop Ctrl+Alt+F9 | If there are breakpoints between the current execution point and the target statement, you can force the debugger to skip these breakpoints with the Run to Cursor Non-Stop | |
Evaluate Expression Alt+F8 | Click this button to evaluate expressions. |
Item | Tooltip and Shortcut | Description |
---|---|---|
Show Execution Point Alt+F10 | Click this button to highlight the current execution point in the editor and show the corresponding stack frame in the Frames pane. | |
Skip to Cursor Ctrl+Alt+Shift+F9 | Instead of running the program up to the target statement, you may want to skip a part of code without actually executing it (for example, to avoid a known bug or not to repeat some routine). You can do this with the Skip to Cursor You can also skip a part of the program by dragging the execution pointer ![]() You can also hover over the target statement and then pick the corresponding action: ![]() Remember the following when you jump to another statement:
| |
Step Over F8 | Click this button to execute the program until the next line in the current method or file, skipping the methods referenced at the current execution point (if any). If the current line is the last one in the method, execution steps to the line executed right after this method. | |
Force Step Over Alt+Shift+F8 | Click this button to have the debugger step over the method even if this method has breakpoints inside. | |
Step Into F7 | Click this button to have the debugger step into the method called at the current execution point. The debugger will set the execution pointer at the first statement of the first function called from the current line. If no function is called, then the debugger will step to the next statement. By default, JetBrains Rider enables external-source debugging, which means that when you step into the library code it will be automatically decompiled and the debugger will move the execution point there. If you want the debugger to ignore the library code, you can disable external-source debugging — clear Enable external source debug on the page of JetBrains Rider settings Ctrl+Alt+S. | |
Smart Step Into Shift+F7 | If the current line contains several nested calls, JetBrains Rider lets you choose a call to step into. Consider the following code line: Console.WriteLine(Foo(Bar("input") + Baz("input"))); The line contains several calls and if you use Step Into, the debugger will first step into the implementation of If you want to choose which of the calls should be stepped into, for example, ![]() When you choose the function to step into, the debugger will set the execution pointer at the first statement of the selected function. The selector also shows you which of the calls were already executed and allows you to execute these calls again. You can enable the 'smart step into' behavior when you invoke Step Into F7 in a line that contains multiple method calls. To do so, select Always do smart step into on the Build, Execution, Deployment | Debugger | Stepping page of settings Ctrl+Alt+S. | |
Step Out Shift+F8 | Click this button to have the debugger step out of the current method, to the line executed right after it. | |
Run to Cursor Alt+F9 | Click this button to resume program execution and pause until the execution point reaches the line at the current cursor location in the editor. No breakpoint is required. Actually, there is a temporary breakpoint set for the current line at the caret, which is removed once program execution is paused. Thus, if the caret is positioned at the line which has already been executed, the program will be just resumed for further execution, because there is no way to roll back to previous breakpoints. This action is especially useful when you have stepped deep into the methods sequence and need to step out of several methods at once. If there are breakpoints set for the lines that should be executed before bringing you to the specified line, the debugger will pause at the first encountered breakpoint. You can also hover over the target statement and then pick the corresponding action: ![]() | |
Run to Cursor Non-Stop Ctrl+Alt+F9 | If there are breakpoints between the current execution point and the target statement, you can force the debugger to skip these breakpoints with the Run to Cursor Non-Stop | |
Evaluate Expression Alt+F8 | Click this button to evaluate expressions. |