Start and Cancel Builds
In this article, we explore common use cases concerning starting and canceling builds via TeamCity REST API:
Starting a TeamCity build from an external software.
Implementing a complex build logic by invoking REST API from the Command Line build runner.
Start Regular Build
To be able to start a build, you need to access the build queue via this endpoint:
Queueing a new build requires two steps:
Prepare the request payload (that is, the
Build
entity) which will represent the target build.Send a
POST
request with the payload.
Step 1:
The payload of this Build
entity must include a reference to a build configuration (or, in REST API terms, BuildType
). We will include it as a sub-entity of Build
.
Step 2:
To add a build to the queue, send a POST
request with the body specified in Step 1:
The request headers should include an authorization header (unless the guest authorization scheme is used) and, optionally, Content-Type
/Accept
headers.
The endpoint will respond with a short description of the launched build. It will also include the TCSESSIONID
cookie: you can reuse it to skip authorization in the following runs.
Start Custom Build
A more complex example is when you need to run a build with settings that differ from those specified in its build configuration. In this case, you need to send extra data about the build in your request.
From the fields available for the Build
entity, we will pass the following:
personal
: to define that the build is personalbranchName
: to launch a build in a non-default branchagent
: to select a specific agent for this build by sending anAgent
sub-entitycomment
: to supply a build with a descriptionproperties
: to set custom build parameters (or create them, if no such parameters are present) by sending a compositeProperties
entity.
Most of the data should be available locally. However, in this example we request a specific agent which requires knowing its ID. If you know an agent by name, you can find its ID by sending the following request:
Here, the ?locator=...
part specifies an AgentLocator
entity which is used to find the agent by name. The request will return the respective Agent
entity from which you can parse out an ID.
The eventual payload for our POST
request will look as follows:
Send it via a POST
request as in the basic case. As a result, a personal build will be run in the myBranch
branch on the specified agent and with two new build parameter values.
Advanced Build Run
In this example, we will show how to compose a payload to rerun a build, clean its sources, and select a specific revision.
Inside the Build
entity, we will supply:
triggeringOptions
: to specify extra parameters by sending theBuildTriggeringOptions
sub-entity.lastChanges
: to select a specific revision for the build by sending theChange
entity. The element also supports a locator field which expectsChangeLocator
to identify a specific revision.
In triggeringOptions
, we want to enable clean checkout, rebuild all dependencies before the current build, and put the build at the top of the queue.
In lastChanges
, we will use the locator as follows:
version
: SHA of the specific revision already detected by TeamCitybuildType
:BuildTypeLocator
which will point to the sameBuiltType
as the one we run the build on.
The expected payload:
Get Queued Build
After queueing a build, you can check its status:
where BuildQueueLocator
represents a queued build — you can supply the build ID you received on sending the POST
request.
This request returns a Build
entity. By default, TeamCity will return basic fields of the entity. For polling purposes, you might want to receive only the build status. You can limit the scope of fields available in the response by using the ?fields
parameter in the request path (read more):
As a result of the call, TeamCity will return the Build
entity with only two fields populated: id
and state
.
Cancel Queued Build
To cancel a just queued build, send:
where a BuildCancelRequest
entity is used as a payload.
This entity comprises two fields:
comment
: to provide an optional comment on why the build was canceledreaddIntoQueue
: a boolean property which declares if this is a request to restore a previously canceled build; as we are aiming to cancel the build, not to restore it, we will set it tofalse
The resulting payload:
To cancel a build by its ID, send:
Alternatively, you may choose to simply delete a queued build (if you don't need its metadata). To do so, run a DELETE
request on the same endpoint:
Cancel Started Build
To cancel a build that has already started, send:
with the same BuildCancelRequest
entity as in the above example:
To cancel a build by its ID, send:
Trigger Chain Builds
In TeamCity, you can organize builds from different configurations to run in a single chain. For example, you can have a chain of three build configurations that ends with a composite build to aggregate build results: ConfigA → ConfigB → ConfigC → ChainABC.
To start an entire chain, trigger a new build for the last build configuration:
If you trigger a new build for a configuration that is not the last one in a chain, only this configuration and those on which it depends will run. For example, the following payload triggers only the ConfigA → ConfigB sequence (ConfigB depends on ConfigA).
Reusing Builds with Direct Dependencies
When triggering a dependent build, you can specify a finished build of a preceding configuration which this new build should reuse. For example, the following payload triggers a new ConfigB build that should reuse an existing ConfigA build with the "4976" ID.
You can specify multiple direct dependencies in the same request. For instance, if ConfigA and ConfigB are independent configurations and ConfigC depends on both of them, you can send the following payload:
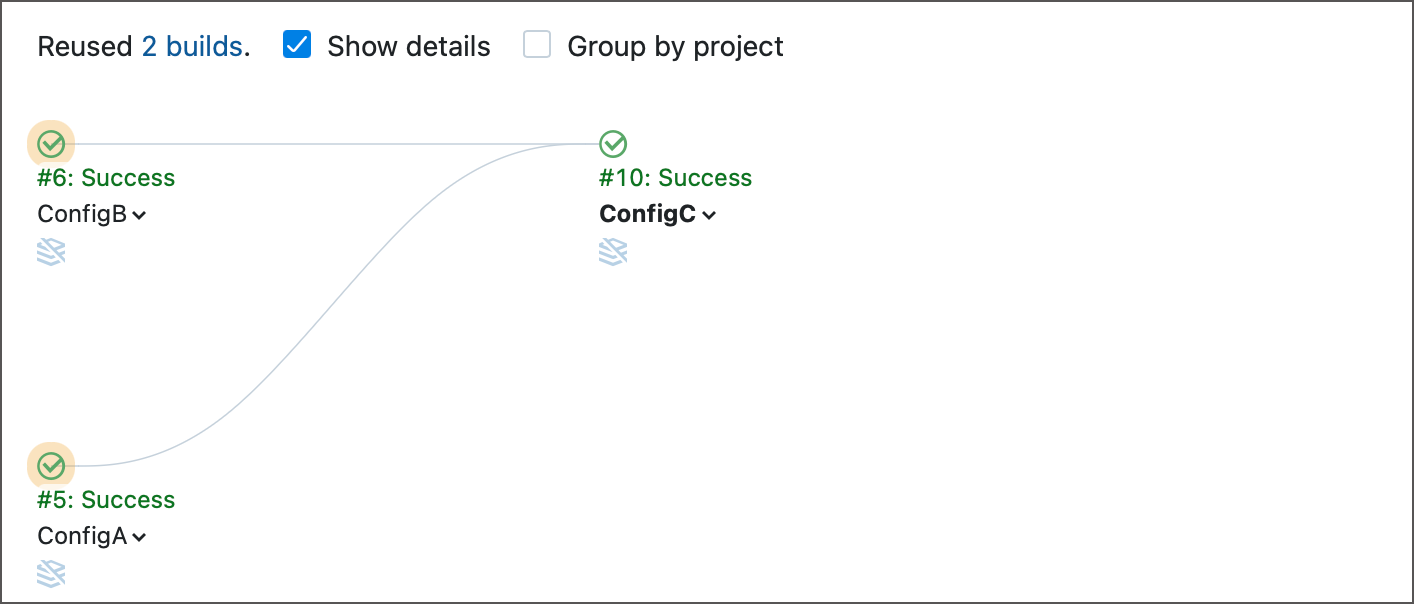
Reusing Builds with Indirect Dependencies
In the previous section, requests reused builds of a configuration on which triggered builds directly depend. However, you can also reuse builds in indirect dependencies.
For example, for the same ConfigA → ConfigB → ConfigC chain you can trigger ConfigC build that should reuse an existing ConfigA build.
A new ConfigB build will or will not run depending on ConfigC's dependency settings. If ConfigC allows reusing suitable builds, both ConfigA and ConfigB builds will be reused.
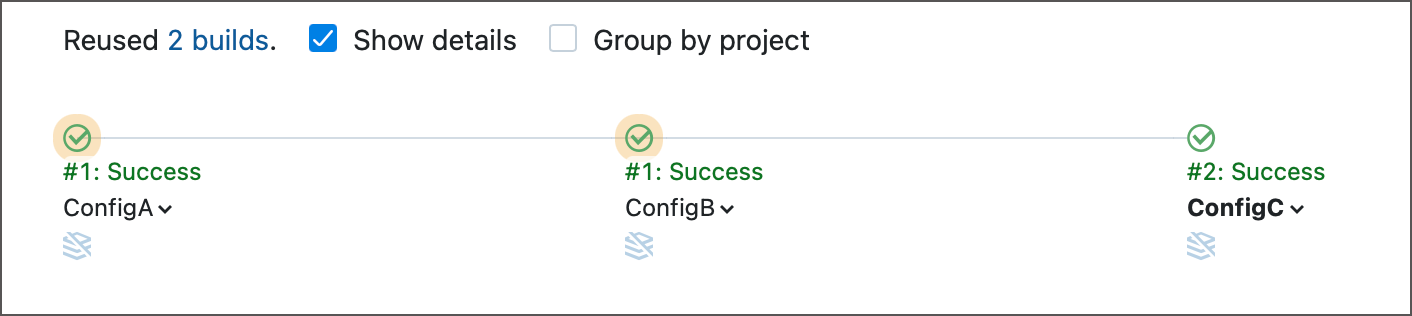
Otherwise, if ConfigC always requires a fresh build, a new ConfigB build will run (using results from an existing ConfigA build).
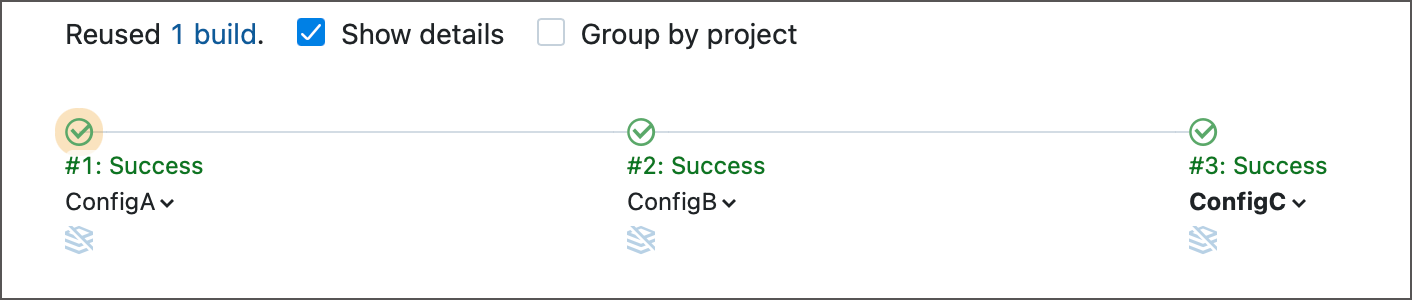