GraphQL
With WebStorm, you can use a GraphQL plugin that supports the GraphQL language. The following functionality is available:
Full language support for GraphQL Specification including the Schema Definition Language (SDL).
Schema-aware completion, error highlighting, and documentation.
Syntax highlighting, code-formatting, folding, commenter, and brace-matching.
Detecting your local schema on the fly. Remote schemas are easily fetched using introspection. You can introspect GraphQL endpoints to generate schema declaration files using the GraphQL Type System Definition Language.
Support for multi-schema projects using configurable project scopes or graphql-config files. Schema discovery is configured using graphql-config files, which includes support for multi-schema projects.
Built-in support for Apollo GraphQL and Relay projects:
graphql
andgql
tagged template literals in JavaScript and TypeScript are automatically recognized as GraphQL.Executing queries using variables against configurable endpoints, including support for custom headers and environment variables.
Find Usages and Go to Declaration for schema types, fields, and fragments.
Structure view to navigate GraphQL files.
Loading variables from shell, .env files or setup them manually per configuration file.
Built-in
Relay
,Federation
, andApollo Kotlin
type definitions (you need to enable them in Settings | Languages & Frameworks | GraphQL).
GraphQL is supported through a dedicated GraphQL plugin, which is available from the JetBrains Marketplace.
Install the GraphQL plugin
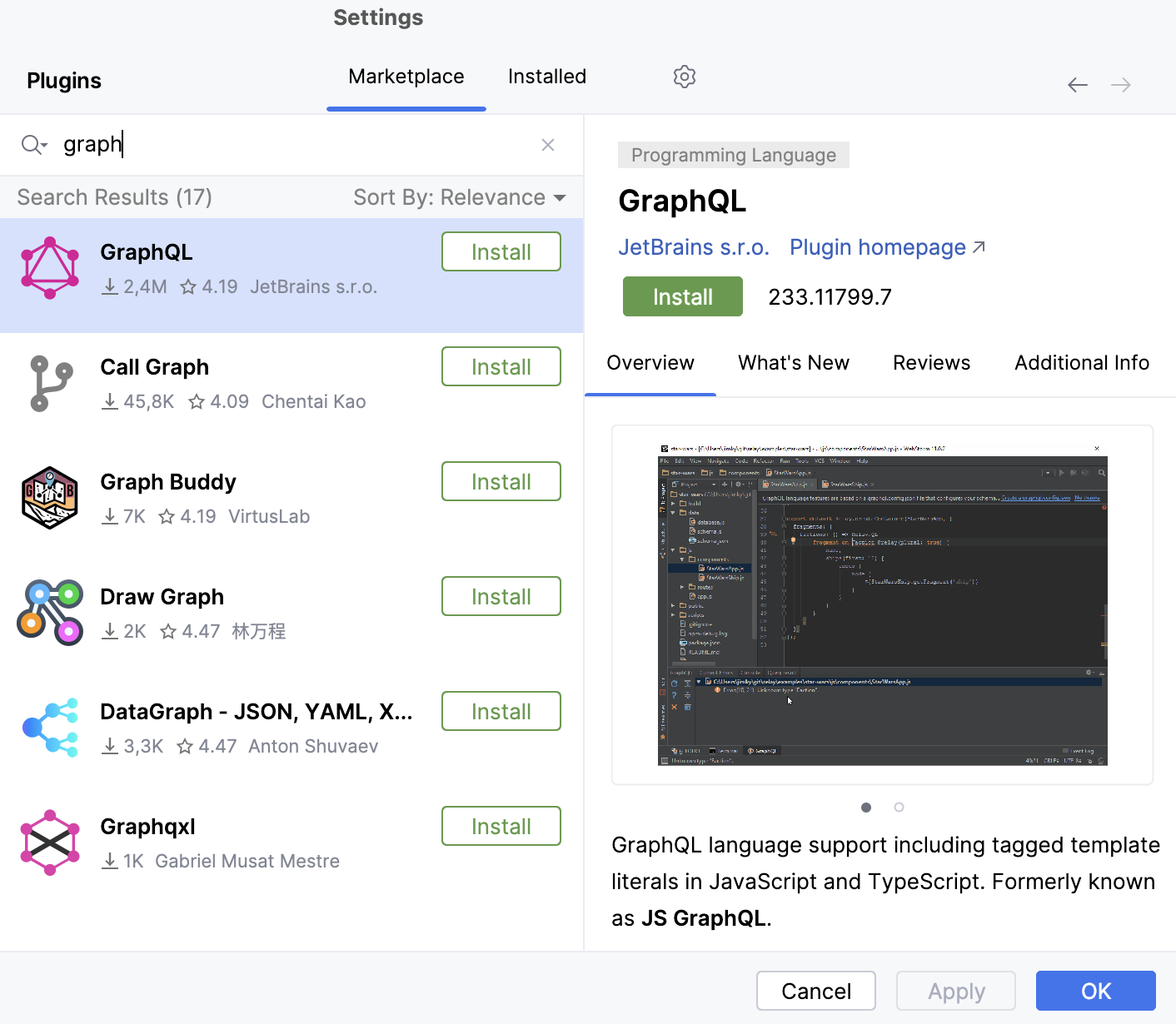
Press Ctrl+Alt+S to open the IDE settings and then select
.On the Marketplace tab, start typing
graph
in the search field, select GraphQL from the list, and click Install.
Configure your application to use GraphQL
It is important that you configure how the schema types are discovered. Based on the information on the schema type WebStorm provides coding assistance, such as code completion and error highlighting.
Schemas and their types are declared using GraphQL Type System Definition Language, which is also widely known as GraphQL Schema Definition Language (often abbreviated as SDL). If you're authoring your schemas in SDL, the plugin provides the following features:
Completion for types when defining fields, arguments, implemented interfaces, and so on.
Error highlighting of schema errors such as unknown types, wrong use of types, and missing fields when implementing interfaces.
Find usages in SDL and refactoring such as rename, which will update the relevant queries, mutations, and so on.
For single-schema projects, schema types are discovered automatically without any additional configuration from your side. WebStorm processes all .graphql files in the Project files scope for type definitions and adds the detected type definitions to a singleton type registry. Injected GraphQL strings in the Project files scope are processed for all JavaScript file types - .js
, .jsx
, .ts
, .tsx
, .html
and html
-based files like .vue
.
For projects with multiple schemas, a scope for each schema should be configured. A schema-specific scope ensures that each type is declared only once and picked up in only one GraphQL type registry, which prevents validation errors. In addition, the scopes prevent non-conflicting types from showing up in completions and ensure that validation only recognizes the types that belong to the current schema.
Learn more from Introduction to GraphQL configuration.
Basic configuration
In the Project tool window, place the cursor at the folder where you want a simple configuration file created and select .
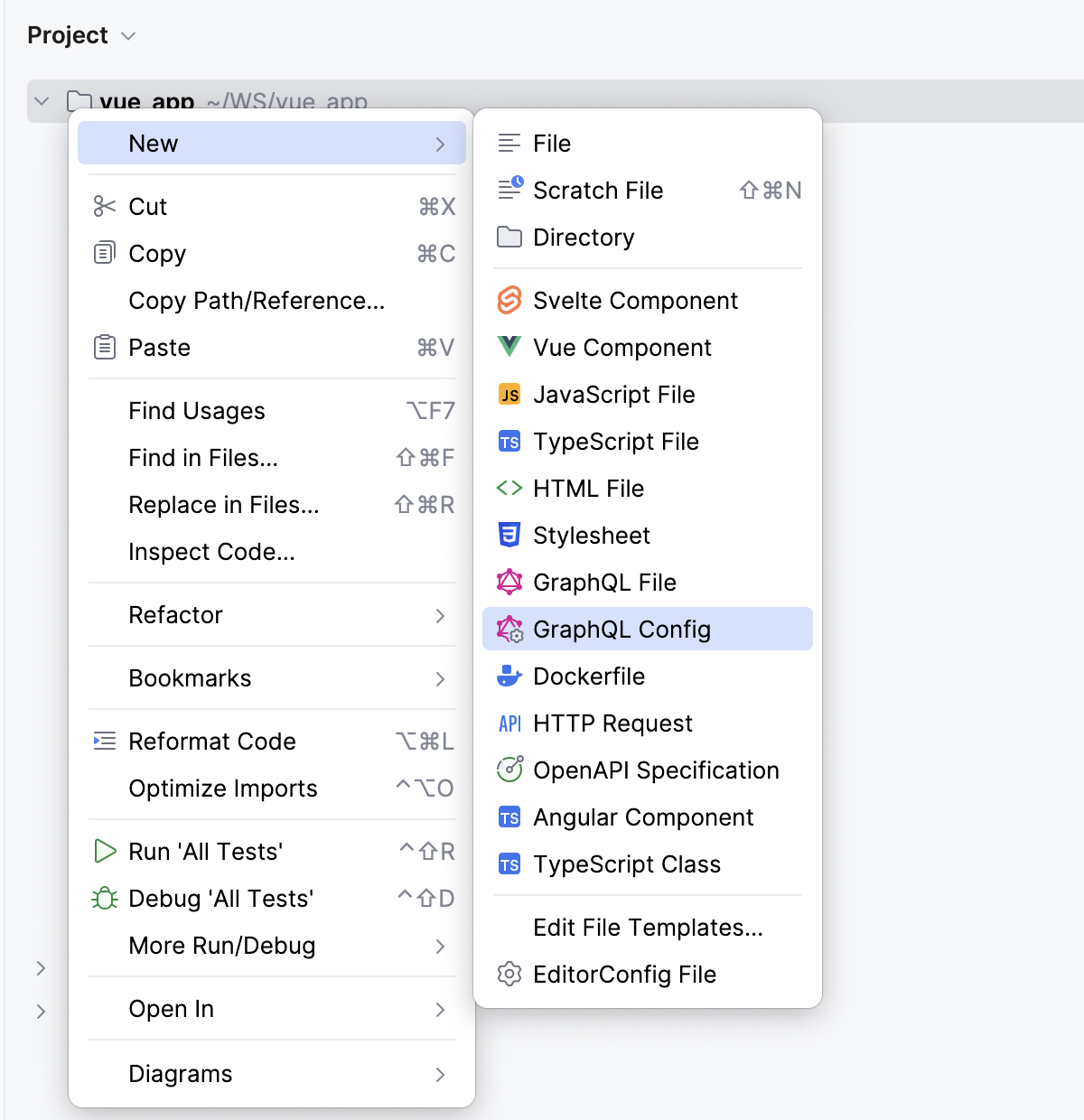
WebStorm creates a file graphql.config.yml and opens it in the editor:
This configuration file states that the schema definition should be located in a schema .graphql file. The documents
key is defined using a glob pattern that will include any GraphQL operation in the current or nested directory. The term operation only refers to GraphQL queries and fragments, but not to type definitions.
Please note that paths are specified relative to the config directory, unless they are explicitly defined as absolute. Therefore, you do not need to prefix them with ./
. Just schema.graphql is sufficient. The same applies to glob patterns.
Configuration for node_modules
To use a schema from the node_modules folder, create a GraphQL configuration file, for example, graphql.config.yml, and specify an exact path to a schema there as follows:
Composite schema
GraphQL Config can also assemble multiple modularized schemas into a single GraphQL schema object by specifying a list of schema files as follows:
Alternatively, you can use a glob pattern to find and include pieces of schema:
GraphQL Config looks for the specified files, reads these files, and merges them to produce a GraphQL schema object.
Remote schemas
If you have a GraphQL endpoint and do not have the local schema file yet, you can define one or more endpoints and make an introspection query. This will load a schema from the server, convert it to a GraphQL file, and save it in the IDE's cache directory.
Depending on whether you need additional configuration for an endpoint, you can specify it as a string or an object with supplementary keys containing data such as headers.
Local introspection schemas
To store an introspection result locally, configure an endpoint as it was done in the legacy configuration format. Define one or multiple endpoints in the endpoints extension, and then make an introspection query. A file will be saved at the first path in the corresponding schema section, for example, a local.graphql file in the example below.
Advanced configuration
To have more fine-grained control over which files should be included in the schema, use the optional include
and exclude
keys. First, a candidate file is checked for exclusion. If the file is not excluded, the file path is matched against the include
pattern.
In the example below, schema.graphql and every file inside the src
directory except files in the __tests__
directory will be included in that project.
Multiple projects
Config per project
A config file defines a GraphQL "module"
root, just like package .json or similar files do. Even if the config file is empty, all files in its directory and in the nested directories use the schema that is associated with that configuration.
Therefore, the simplest way to separate different schemas is to create a configuration file inside each subdirectory, if they are completely independent, which is usually the case with monorepos. With this approach, the location of each config file creates a separate scope for each subtree.
Single config
If you prefer to have a single configuration file, you can specify multiple projects in the same file.
The configuration for such case should be as follows:
Files are matched against projects in the order in which the projects are defined. Therefore, if a file matches several projects, the first project will be chosen.
GraphQL operations are matched non-strictly when no include
or exclude
keys are defined for a specific project. This means that if a query or a fragment does not match any project explicitly, the file will be associated with the first project that does not have include
or exclude
keys. In example above, there is an additional root directory called queries
, in addition to backend
and frontend
. If queries
contains some GraphQL documents that do not match any of the provided patterns, the first project, frontend
, will be associated with those queries.
To achieve this, you can add an exclude
pattern to the frontend
project configuration. This will associate the files in the queries
folder with the backend
project.
This does not apply to type definitions, as mentioned previously. WebStorm only uses type definitions from files that strictly match the schema
or include
keys.
Configuration files
WebStorm recognizes the following formats of configuration files:
graphql.config.json
graphql.config.js
graphql.config.cjs
graphql.config.ts
graphql.config.yaml
graphql.config.yml
.graphqlrc
(YAML and JSON).graphqlrc.json
.graphqlrc.yaml
.graphqlrc.yml
.graphqlrc.js
.graphqlrc.ts
YAML
Make sure the YAML bundled plugin is enabled in the Installed tab of the Settings | Plugins page as described in Install plugins.
JavaScript
Make sure you have Node.js on your computer. Node.js is used to download JavaScript configuration files.
WebStorm does not transpile configuration files, so make sure they are written using the appropriate for Node.js module system. In the example below, the
module.exports
syntax is used instead ofexport default
.module.exports = { schema: 'https://localhost:8000' }To use ESM in your configuration, add
"type": "module"
to your package.json file.
TypeScript
Make sure you have Node.js on your computer. Node.js is used to download TypeScript configuration files.
To load a TypeScript config, download and install the
ts-node
package by typing one of the following commands in the built-in Terminal tool window Alt+F12:npm install --save-dev ts-node
to install the package in your project.npm install --g ts-node
to install the package globally on your. computer
Learn more from Typestrong ts-node.
To use ESM modules in your project:
Configure ESM modules as
"type": "module"
in your package .json file.Set up an ESM loader for
ts-node
by adding the following to your tsconfig.json file:{ "compilerOptions": { // or ES2015, ES2020 "module": "ESNext" }, "ts-node": { "esm": true } }
Learn more from Native ECMAScript modules.
Migration
If you are using a legacy configuration file, such as .graphqlconfig, it is strongly recommended that you convert it to a modern format of your choice, for example, YAML which is the most convenient for this purpose. WebStorm incorporates an automatic conversion tool and shows a notification panel at the top of the editor as soon as you open a legacy file. Just click Migrate in the notification panel to have the config keys and the environment variable syntax updated automatically.
It is recommended that you migrate existing includes
patterns that were previously used to configure compound schemas to the new schema
key. Please note that environment variables now have a different syntax, for example, support specifying a default value.
Legacy configuration
If you still prefer to use a legacy configuration format, make sure to explicitly specify the paths to schema files in the includes
property. Otherwise, only the types from schemaPath
will be used for schema construction.
Environment variables
You can use environment variables in your configuration files to specify a schema path, URL, or a header value. The syntax for using environment variables in configuration files is as follows:
You can load definitions from environment variables, with or without fallback values.
If you want to define a fallback endpoint, you probably need to wrap your value with quotation marks.
.env files
There are several ways to provide environment variables. The most recommended method is to create a dedicated file with the variable values. To avoid exposing your credentials, please refrain from committing this file.
The following filenames are supported in order of priority from top to bottom:
.env.local
.env.development.local
.env.development
.env.dev.local
.env.dev
.env
WebStorm searches for the specified file starting from the directory that contains the corresponding configuration file and going up to the project root.
In such files, environment variables are represented as simple key-value pairs separated by the =
sign. Values can optionally be enclosed in quotes.
Manual configuration
To use .env files, provide environment variables manually for each configuration file by doing one of the following:
To edit GraphQL environment variables in a config file, open the file in the editor, right-click anywhere to open the context menu, and select
. This will open a modal dialog where you can provide values for each environment variable in the file.Open any GraphQL file and click the toolbar. The dialog will automatically find a matching configuration file.
Otherwise, only missing variables would be requested on the first introspection query or GraphQL request.
System
If no variables are found in the .env files or set manually, WebStorm attempts to retrieve them from your system environment.
Frameworks
Gatsby
Create a graphql.config.js at the root of your project with the following contents:
Introspection
To provide resolution, completion, and validation, WebStorm requires a schema. This can be achieved by performing an introspection query, which should be configured beforehand.
If you are using YAML or JSON config files, the easiest way to make an introspection query is to click
in the gutter. This will make an introspection query and save a file locally, either to the IDE's cache or to the project sources, depending on the configuration.
Select Run Introspection Query from the toolbar.
In the GraphQL tool window, right-click an end-point and select from the context menu.
To inspect the schema structure of an introspection file in the editor, use the Open Introspection Schema action on the toolbar. This will open a file for the selected endpoint.
Rerun latest introspection
If your schema is constantly changing, and you find yourself repeatedly running the same introspection action against the same endpoint, it may be more convenient to use the Rerun Introspection Query action. Note that this option only becomes available after you have already performed an introspection query.
Press Ctrl+Shift+A to open the Actions tab of the Search Everywhere popup, start typing
Rerun Introspection...
, and select Rerun Introspection Query from the list. Learn more from Search for actions.To assign a shortcut to this action, press Alt+Enter and specify a keystroke in the dialog that opens. Learn more from Add a keyboard shortcut.
Queries
To execute a query directly from the editor, place the caret on the query definition, and click Execute GraphQL on the toolbar or press Ctrl+Enter. The query will be sent to a selected endpoint in the toolbar.
If your query has variables, click
on the toolbar to open a dedicated variables editor and then provide the variables in the
JSON
format.Alternatively, create a GraphQL scratch file and use it as a playground for sending queries. In the GraphQL tool window, double-click the endpoint node and select New GraphQL Scratch File.
Scratch files
If the leading comment in a GraphQL scratch file contains a string that follows the # config=<path>[!<optionalProjectName>]
pattern, the specified config and project will be used for resolving and type validation. Comments that follow this pattern are considered valid:
Toolbar
Each GraphQL file has a toolbar with the most commonly used actions.
Open Configuration File | Open the corresponding configuration file, or create a new one if it does not exist. | |
Edit Environment Variables | This opens a dialog that allows you to provide values for environment variables that are defined in the associated configuration file. | |
Toggle Variables Editor | This opens an editor window where you can provide query variables in JSON format. | |
Endpoints list | A list of known URLs is defined in a config file, which you can use to select a URL where the GraphQL queries should go or from where the introspection should be fetched. | |
Execute GraphQL | Run a selected GraphQL query from the editor below. | |
Run Introspection Query | This action refreshes an introspected schema from the selected endpoint. | |
Open Introspection Schema | This command opens a local file that corresponding to a selected endpoint. |
Tool window
The GraphQL tool window provides an overview of your GraphQL projects. It can show validation errors, perform introspection queries, create scratches, and more.
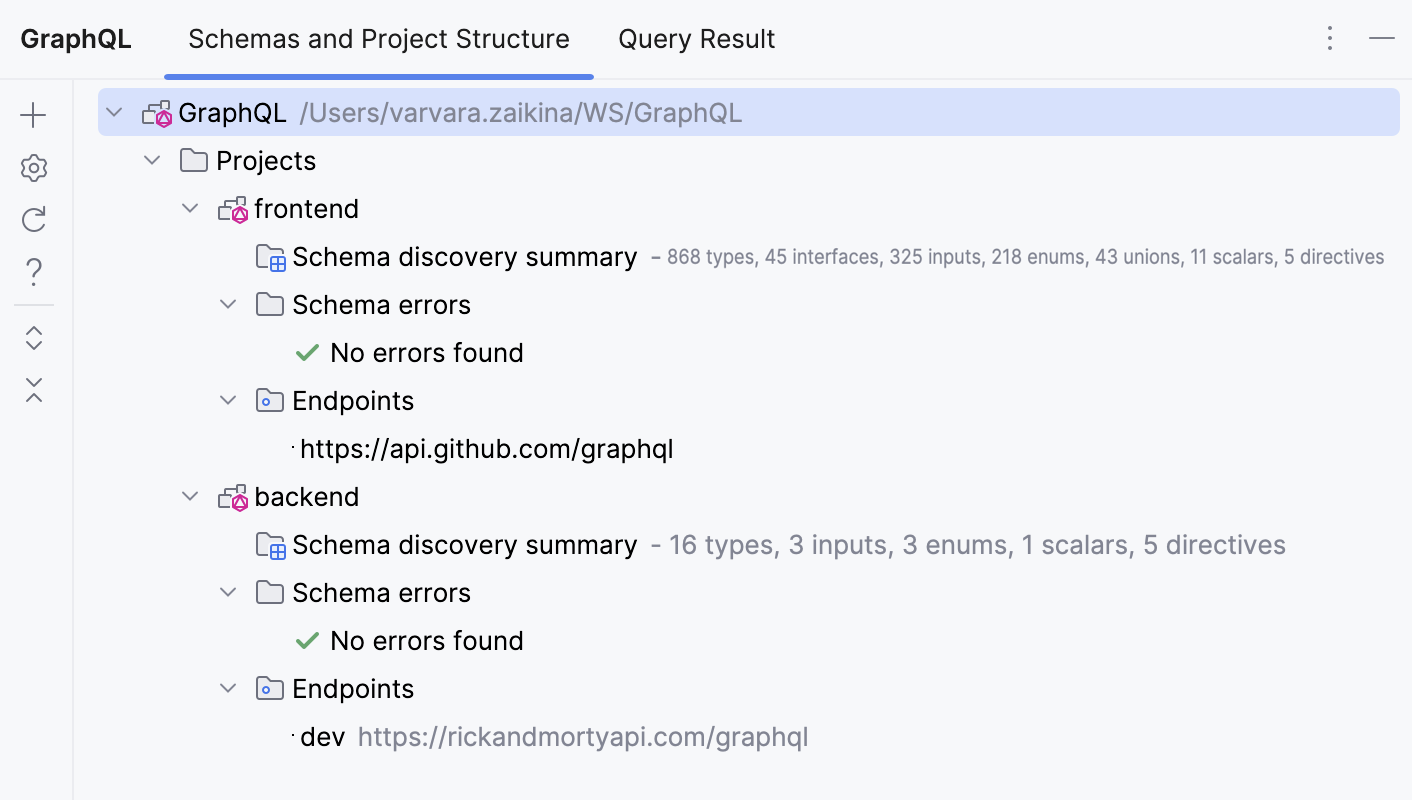
The Schemas and Project Structure tab provides an overview of detected configuration files and the GraphQL schema associated with each of them. The following useful actions for multiple nodes are available in this tree view.
Schema discovery summary - To search through the types discovered for each project, double-click on the corresponding node. This will open a dialog window that enables you to search through every type and navigate to the place where they are defined.
Schema errors - Click an error node to navigate to the source of the error.
Endpoints - Right-click an endpoint to o make an introspection query or to create a Scratch file associated with the selected configuration file and project.
Tool window toolbar
The toolbar in the left-hand side of the GraphQL tool window provides the following handy actions:
Add Schema Configuration | Create a configuration file in a selected directory. Alternatively, you can create a configuration file from the Project tool window as described in Basic configuration above. | |
Edit Selected Schema Configuration | Opens a configuration file for the selected node in the tool window. | |
Restart Schema Discovery | This action clears all loaded schemas and starts the discovery process from scratch. It can be useful in cases where cached data is causing issues. |
Injections
Tagged template literals
WebStorm supports graphql
, gql
, Relay.QL
, and Apollo.gql
tags.