Code completion
Code completion is one of the essential features of the WebStorm editor. To show you completion suggestions, WebStorm goes through the project files with the source code and adds classes, methods, functions, and variables defined in them to a special internal index. Additional information from JSDoc comments, TypeScript type definitions, and so on can also significantly improve completion.
Completion also works for symbols from third-party code. In most cases, all you need is to add the required files to your project.
This section covers various techniques of context-aware code completion that allow you to speed up your coding process.
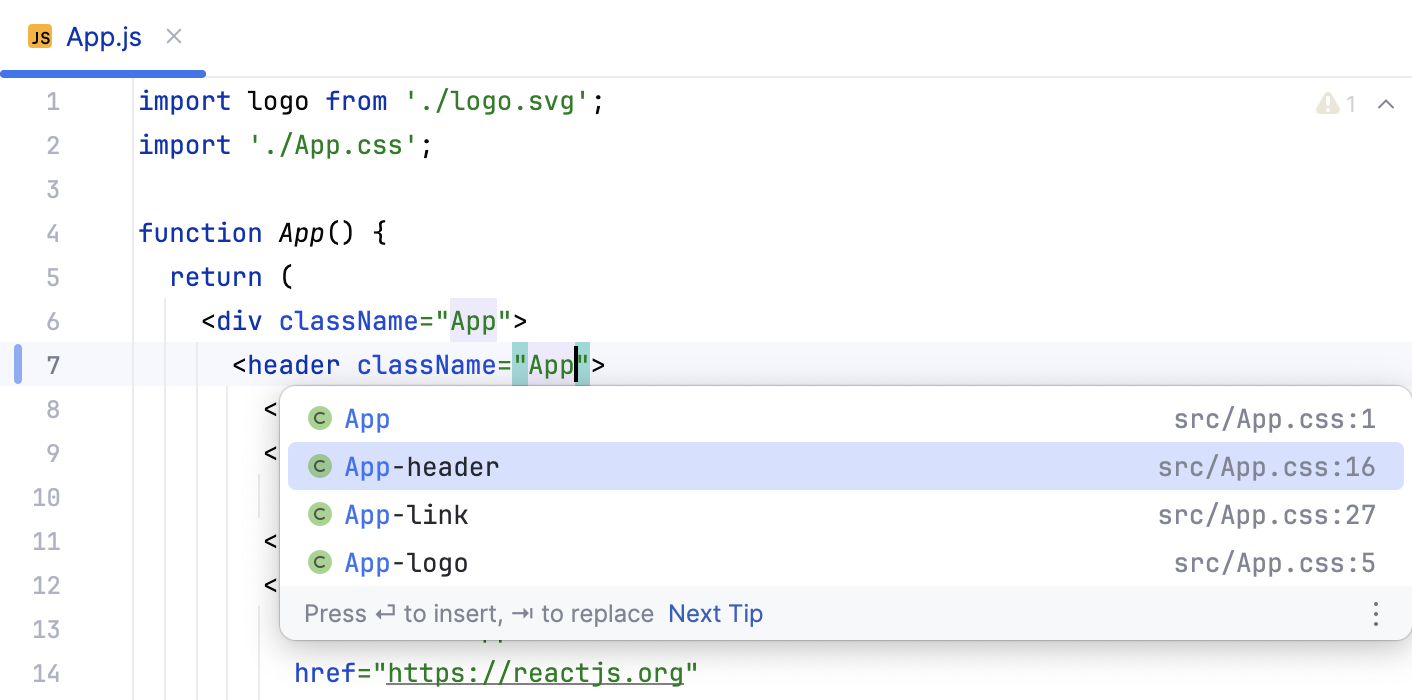
Basic completion
Basic code completion helps you complete the names of classes, methods, fields, and keywords within the visibility scope.
When you invoke code completion, WebStorm analyzes the context and suggests the choices that are reachable from the current caret position (suggestions also include Live templates) .
Invoke basic completion
Start typing a name. By default, WebStorm displays the code completion popup automatically as you type.
If automatic completion is disabled, press Ctrl+Space or choose
from the main menu.To get more suggestions, press Ctrl+Space for the second time (or press Ctrl+Alt+Space).
To narrow down the suggestion list, type any part of the expected name or keyword (even characters from somewhere in the middle are accepted) or invoke code completion after a dot separator. WebStorm shows the suggestions that include the typed characters in any positions.
Accept a suggestion from the list
Press Enter or double-click the relevant list item to insert it to the left of the caret.
Press Tab to replace the characters to the right from the caret.
Use Ctrl+Shift+Enter to make the current code construct syntactically correct (balance parentheses, add missing braces and semicolons, and so on).
Use specific language and context-dependent keys to insert the selected completion suggestion. For more information, refer to Configuring completion settings.
View reference for the selected suggestion
For quick documentation look-up, select an item in the suggestion list and press Ctrl+Q. WebStorm shows you quick documentation for it in the Documentation popup.
To view the documentation in a tool window with more controls, pin the Documentation popup. To switch between the Documentation popup and the Documentation tool window, press Ctrl+Q sequentially.
To view the definition of the selected suggestion, press Ctrl+Shift+I.
View code hierarchy
Select an entry in the suggestion list and press one of the following shortcuts:
Ctrl+H to view type hierarchy
Ctrl+Alt+H to view view call hierarchy.
Ctrl+Shift+H to view method hierarchy.
For more information, refer to Building hierarchies.
Show suggestions for the names of new class fields, variables, and parameters
Besides completing calls of existing symbols, WebStorm can suggest names for new class fields, variables, and parameters during their declaration. These suggestions are based on the names of classes, types, and interfaces that are defined in your project, in the libraries you are using, and in standard APIs.
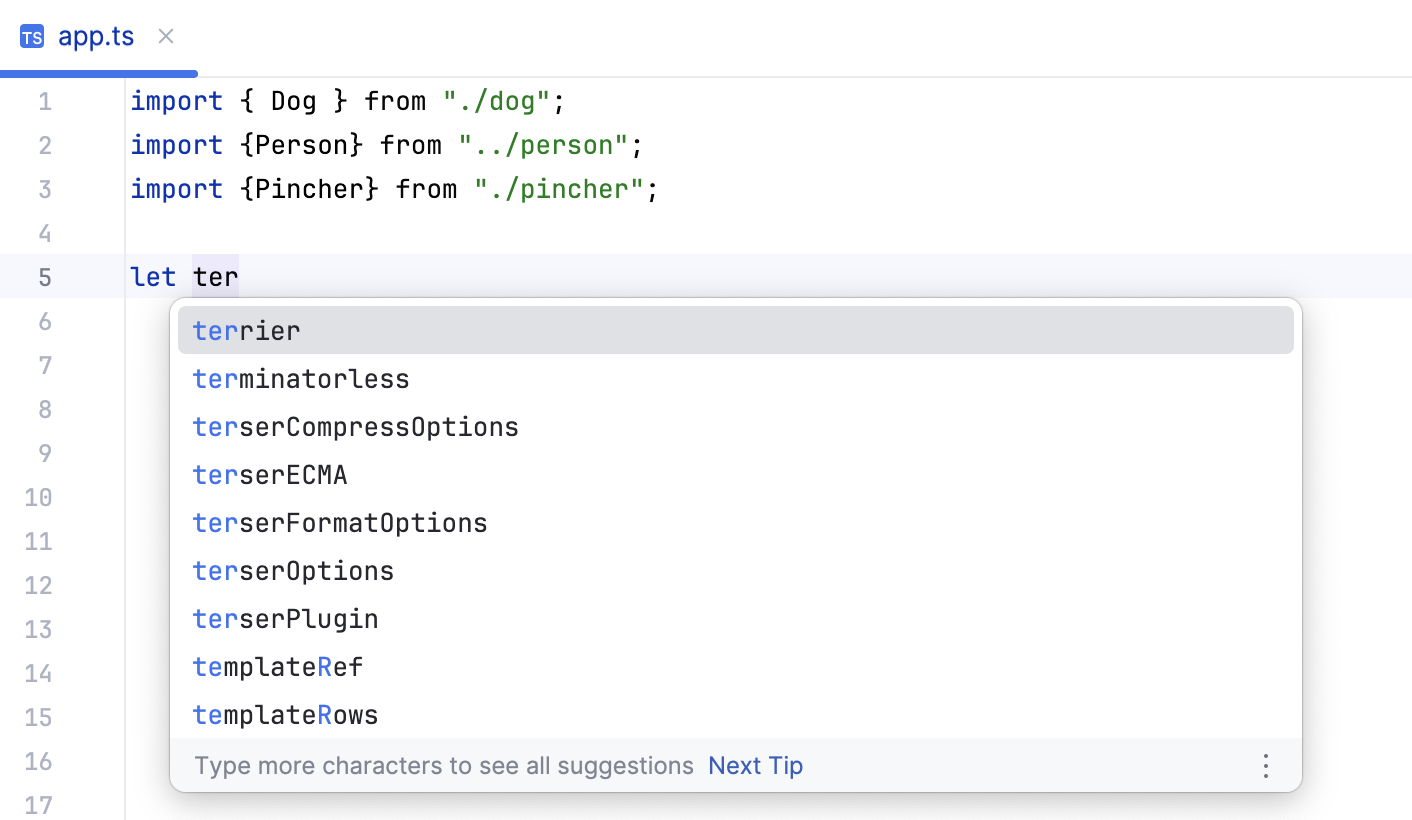
By default, this functionality is off.
Go to the Editor | General | Code Completion page of settings Ctrl+Alt+S.
In the JavaScript section, select the Suggest variable and parameter names and Suggest names for class fields checkvboxes.
If applicable, enable showing type annotations for suggested parameter names.
Completion from the TypeScript Language Service in JavaScript
You can enhance basic code completion in JavaScript files by using suggestions from the TypeScript Language Service. Learn more from Verifying TypeScript.
Open a jsconfig.json or tsconfig.json file and add
'allowJS' : true
to it.Press Ctrl+Alt+S to open settings and then select
.On the TypeScript page that opens, make sure the TypeScript Language Service checkbox is selected.
Statement completion
You can create syntactically correct code constructs by using statement completion Ctrl+Shift+Enter. It inserts the necessary syntax elements (parentheses, braces, and semicolons) and gets you in a position where you can start typing the next statement.
Complete method declarations
Start typing a method or function declaration and press Ctrl+Shift+Enter. WebStorm creates an entire construct of a method and places the caret inside the method body.
Alternatively press Ctrl+Shift+Enter later, after the opening parenthesis.
Complete code constructs
Start typing a code construct, for example, type
if
, and press Ctrl+Shift+Enter. WebStorm automatically adds the required punctuation and places the caret at the next edit point.
Hippie completion
Hippie completion is a completion engine that analyses your text in the visible scope and generates suggestions from the current context. It helps you complete any word from any of the currently opened files.
Expand a string at caret to an existing word
Type the initial string and do one of the following:
Press Alt+/ or choose
to search for matching words before the caret.Press Alt+Shift+/ or choose
to search for matching words after the caret and in other open files.
The first suggested value appears, and the prototype is highlighted in the source code.
Accept the suggestion, or hold the Alt key and keep pressing / until the desired word is found.
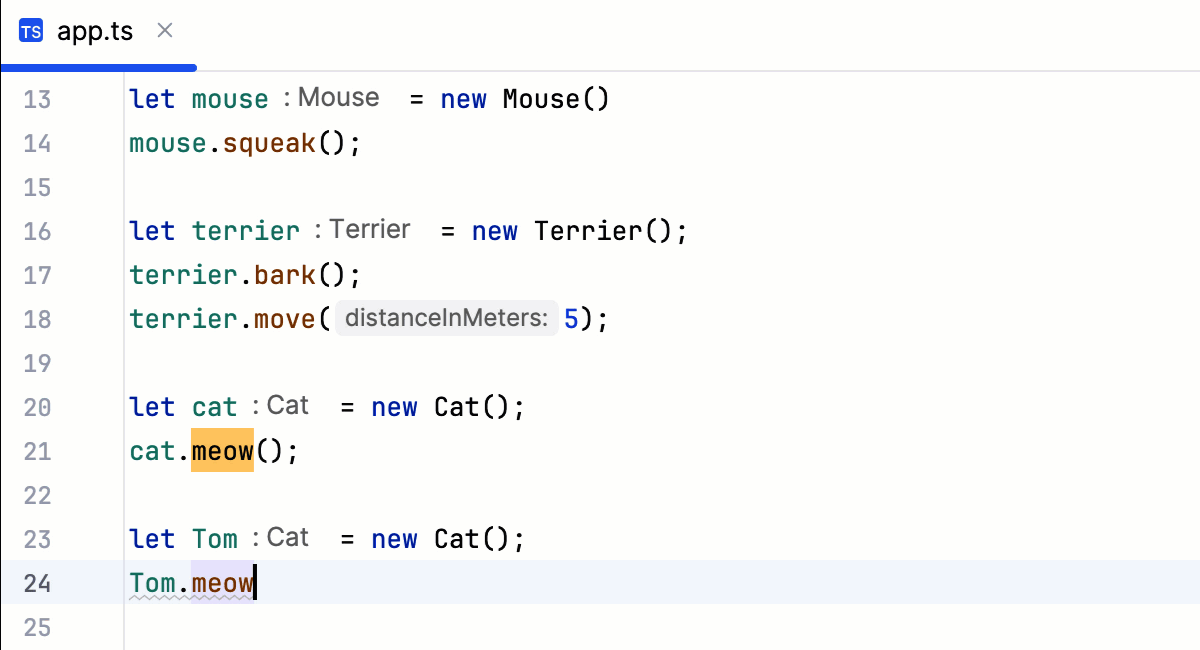
Postfix code completion
Postfix code completion helps you reduce backward caret jumps as you write code. You can transform an already-typed expression to a different one based on a postfix you type after the dot, the type of expression, and its context.
WebStorm is shipped with a set of predefined postfix templates and lets you define your own custom ones for JavaScript and TypeScript.
Custom templates can be copied, updated, and removed. For predefined templates you can only change their postfixes, for example to replace a long key with a shorter one.
Learn more from JavaScript postfix templates.
Enable and configure postfix completion
In the Settings dialog (Ctrl+Alt+S), open and select the Enable postfix completion checkbox.
Select Tab, Space, or Enter to be used for expanding postfix templates.
Enable/disable a particular postfix template for the selected language.
Learn more from Activating postfix completion.
Completion of overrides
When you want to override a method from the parent class or interface and select this method from the list of completion suggestions, WebStorm automatically adds parameters, generates a super()
call, and adds the type information, if possible.
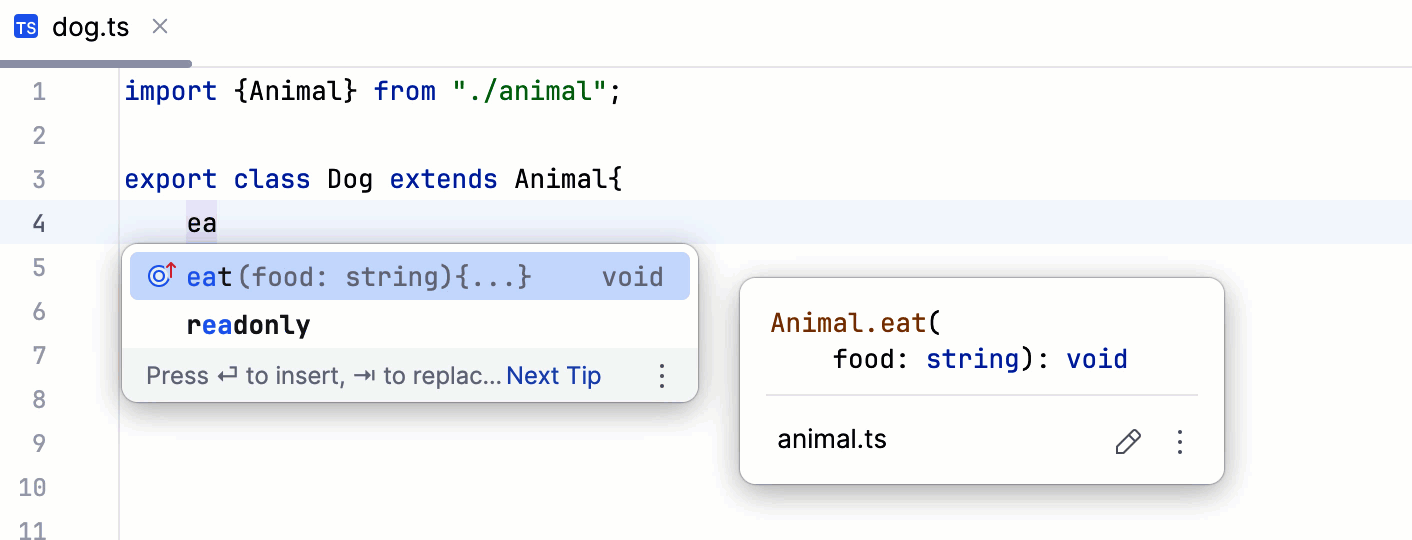
Turn off generation of method bodies for overrides
In the Settings dialog (Ctrl+Alt+S) , go to .
On the JavaScript page that opens, clear the Expand method bodies in completion for overrides checkbox.
Completion of tags, attributes, and properties
WebStorm automatically completes names and values of tags and attributes in many file types:
HTML/XHTML, including completion for CSS classes and for HTML tags inside JSX.
XML/XSL, including completion for namespaces.
JSON. For more information, refer to Editing package.json.
Completion of tags and attribute names is based on the DTD or Schema the file is associated with. If there is no schema association, WebStorm will use the file content (tag and attribute names and their values) to complete your input.
Type the opening
<
and then start typing the tag name. WebStorm displays the list of tag names appropriate in the current context.Use the Up and Down buttons to scroll through the list.
Press Enter to accept a selection from the list. If your file is associated with a schema or a DTD, WebStorm automatically inserts the mandatory attributes according to it.
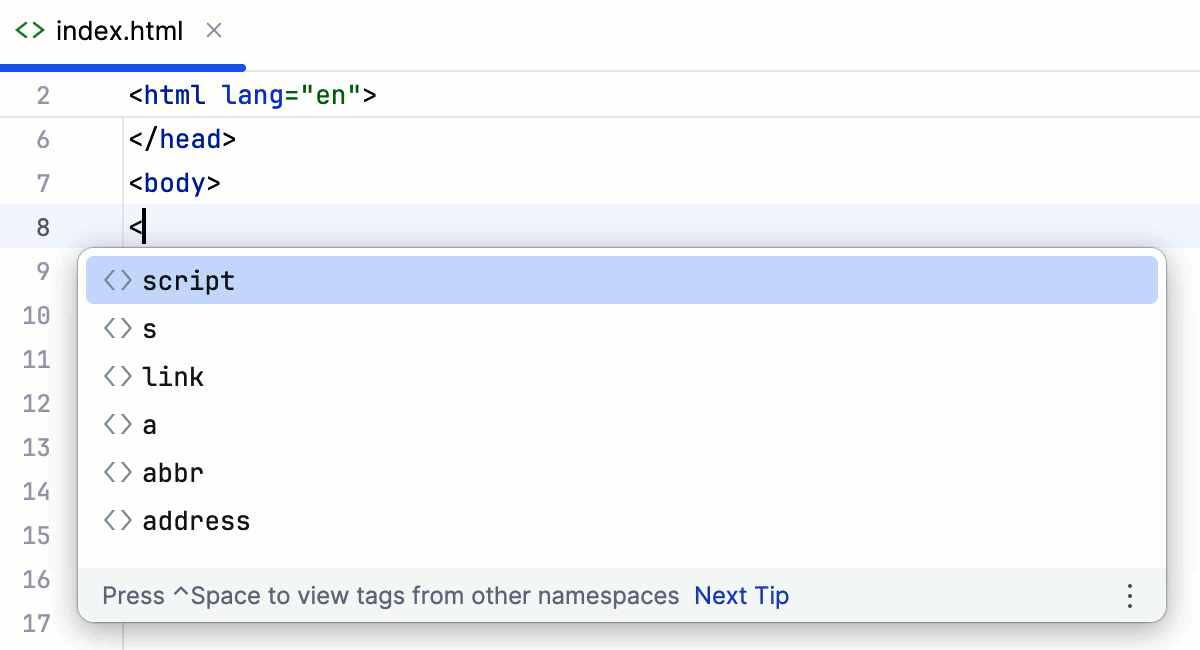
Machine-learning-assisted completion ranking
WebStorm allows you to prioritize completion suggestions based on choices that other users made in similar situations.
The ML completion mechanism doesn't add any new elements but orders the elements retrieved from code. Data is not exposed anywhere; it is collected locally.
Enable ML completion
Press Ctrl+Alt+S to open settings and select Editor | General | Code Completion.
Under Machine Learning Completion Ranking, enable the Sort completion suggestions based on machine learning option, and select the languages for which you want to use ML completion.
Enable relevance markers
Press Ctrl+Alt+S to open settings and select Editor | General | Code Completion.
Enable the following options:
Mark position changes in the completion popup: use the
and
icons to indicate whether the relevance of a suggestion is increasing or decreasing and therefore the suggestion has moved up or down the suggestion list.
Mark the most relevant item in the completion popup: use the
icon to indicate the most suitable suggestion on the list.
Full Line code completion
Full Line code completion provides suggestions as you type code in the editor. Suggestions are displayed in gray italics. To accept the suggestion, press Tab.



The IDE formats all suggestions and adds required brackets and quotes.
Each supported language has its own set of suggested code checks. The most basic ones, like unresolved reference checks, are available for most of the languages to guarantee that the IDE doesn't suggest non-existent variables and methods.
Full Line completion supports auto-import and uses smart filtering to avoid showing suggestions that tend to be canceled explicitly or deleted right after they were accepted.
Before you start working with Full Line code completion, note that:
Full Line code completion is currently not supported in Remote Development.
Full Line code completion requires a computer with a 64-bit processor or an x86 processor that supports AVX2.
Enable Full Line completion
Press Ctrl+Alt+S to open settings and select Editor | General | Code Completion.
In the Machine Learning-Assisted Completion section, select Enable Full Line suggestions and select the languages that you want to use Full Line completion with.
Models for JavaScript and TypeScript are bundled with WebStorm.
Full Line completion runs locally using the models that are downloaded to your computer. You can choose the way with which these models are updated from the Download models drop-down list. You can update the models automatically, manually, or confirm every update in a notification.
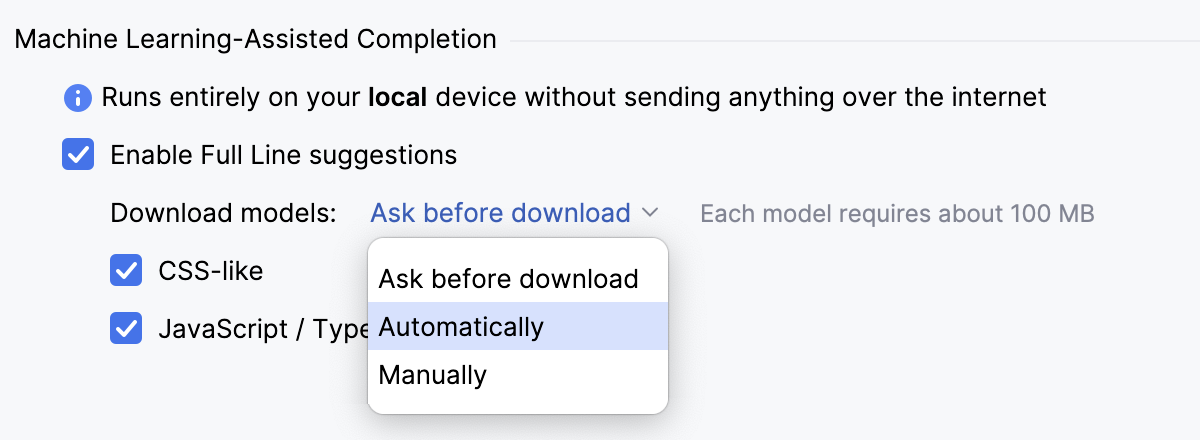
Configure Full Line completion
Hover over the suggestion.
In the popup that appears, click
and select the key that you want to use for accepting suggestions, for example, Right.
To assign your own shortcut, select Custom.
For quick access to the Full Line completion settings, click
in the popup.
Configuring code completion behavior
To customize the default completion behavior, go to the Editor | General | Code Completion page of settings Ctrl+Alt+S and update the default completion settings.
To access code completion settings right from the completion popup, click the icon and select Code Completion Settings.
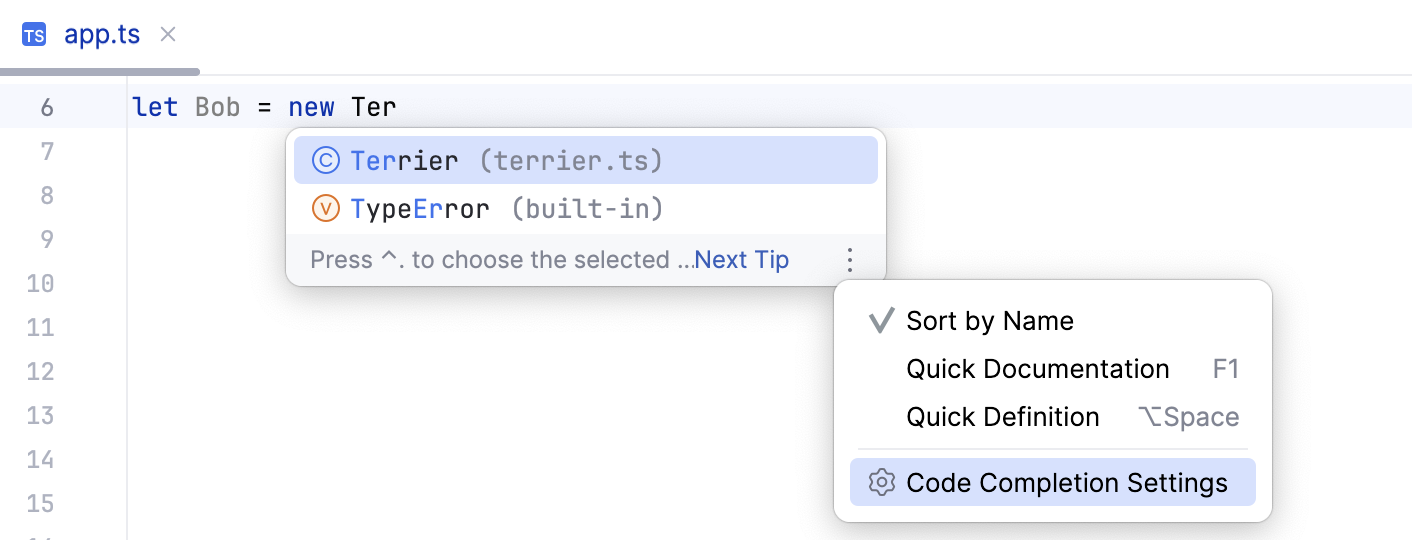
After that, the Editor | General | Code Completion page of settings Ctrl+Alt+S opens.
To tune completion in the JavaScript context, use the controls in the JavaScript area.
Display the suggestion list automatically as you type
Press Ctrl+Alt+S to open settings and then select
.Select the Show suggestions as you type checkbox. If the checkbox is cleared, you have to call code completion explicitly by pressing Ctrl+Space for basic completion or Ctrl+Shift+Space for type-matching completion.
You can also choose to automatically insert suggestions when there's just one option: select the completion type under Automatically insert single suggestions for.
Show suggestions in the alphabetic order
Press Ctrl+Alt+S to open settings and then select
.Select the Sort suggestions alphabetically checkbox. By default the checkbox is cleared and suggestions are sorted by their relevance.
Alternatively use
or
in the lower-right corner of the suggestion list to toggle these modes. Note that these icons appear are not displayed for lists containing just a few entries.
Include suggestions in the list with respect to case
Select the Match case checkbox and choose whether you want to match case for first letters only, or for all letters.
Turn on automatic documentation look-up
Press Ctrl+Alt+S to open settings and then select
.Select the Show the documentation popup checkbox.
Learn more from Basic completion: documentation look-up.
Insert parentheses automatically
Press Ctrl+Alt+S to open settings and then select
.Select the Insert parentheses automatically when applicable checkbox.
By default, this option is turned on and WebStorm automatically inserts a pair of opening and closing parentheses when you complete a function/method.
Clear the checkbox to suppress inserting parentheses automatically.
If you use an opening parentheses
(
instead of Enter to apply the selected item from a completion list, parentheses will be inserted automatically no matter whether the option is turned on or off.To use an opening parentheses
(
instead of Enter to apply the selected item from a completion list, select the Insert selected suggestion by pressing space, dot, or other context-dependent keys checkbox. For more information, refer to Use specific keys to insert suggestions.
Use specific keys to insert suggestions
Press Ctrl+Alt+S to open settings and then select
.Select the Insert selected suggestion by pressing space, dot, or other context-dependent keys checkbox. These keys depend on the language and the context where completion is invoked.
Suppress completion for plain text in HTML
In the HTML context, WebStorm by default shows completion suggestions even when you type plain text without an opening angle bracket.
To turn this annoying behavior off, open the Settings dialog (Ctrl+Alt+S) , go to , and clear the Enable the auto-popup of tag name code completion when typing in HTML text checkbox.
Sort suggestions based on Machine Learning
Press Ctrl+Alt+S to open settings and then select
.By default, completion suggestions in JavaScript and TypeScript files are sorted by their relevance based on machine-learning algorithms.
To turn off ML-based sorting, clear the Sort completion suggestions based on machine learning checkbox.
To turn off ML-based sorting in JavaScript or in TypeScript only, clear the corresponding checkbox.
WebStorm can rank suggestions by their relevance and show special markers to indicate this rank.
The most relevant suggestion is marked with an asterisk
. To show this indicator, select the Mark the most relevant item in the completion popup checkbox.
The
and
arrow icons indicate whether the relevance of a suggestion is increasing or decreasing and therefore the suggestion has moved up or down the list.
To show these indicators, select the Mark position changes in the completion popup checkbox.
Configure completion for JavaScript
Besides common settings for completion behavior, you can configure some JavaScript-specific options on the Settings dialog (Ctrl+Alt+S).
page of theWebStorm suggests completion for symbols regardless of their types. With this approach, in complicated cases the list shows multiple completion variants.
To make completion more precise, select Only type-based completion. The completion list will strongly depend on the WebStorm inference. As a result, the list may remain empty in case of poor inference.
WebStorm suggests completion for symbols with the optional chaining operator (?).
To suppress this behavior, clear the Suggest items with optional chaining for nullable types checkbox.
When you want to override a method from the parent class or interface and select this method from the list of completion suggestions, WebStorm automatically adds parameters, generates a
super()
call, and adds the type information, if possible.Clear the Expand method bodies in completion for overrides checkbox to suppress automatic generation of method bodies for overrides during completion.
Configure completion for names:
Suggest variable and parameter names: By default, the checkbox is cleared. When it is selected, WebStorm suggests names for new class fields, variables, and parameters during their declaration. These suggestions are based on the names of classes, types, and interfaces that are defined in your project, in the libraries you are using, and in standard APIs.
Suggest names for class fields
The option is turned off by default.
Add type annotations for suggested parameter names: Select this checkbox to supply each completion suggestion with information on its type.
The checkbox is available only when the Suggest variable and parameter names checkbox is selected.
Configure how parameter information is shown when you examine or accept a suggestion.
Show the parameter info popup (in ms): Select this checkbox to have WebStorm automatically show a popup with all available method signatures when an opening bracket is typed in the editor, or a method is selected from the suggestion list.
In the text field to the right, specify the delay (in milliseconds) after when the popup window should appear.
If this checkbox is not selected, select
from the main menu.Show full method signatures: If this checkbox is selected, the parameter info displays full signatures, including method name and returned type.
Completing a path
This type of completion speeds up selection of files and folders. The dialog used for that purpose is called rather often, for example, when you specify the installation folder of an external tool or a Version Control engine or configure a Node.js interpreter.
Open the Select Path dialog by choosing on the menu or by clicking Browse
next to the field where a path is required.
Make sure that the Path field is visible. If the field is hidden, click the Show path link.
Start typing a path. WebStorm suggests a list where you can quickly find the needed directory.
As you type, the suggestion list shrinks to show the matching paths only.
You can also press Ctrl+Space to show the suggestion list.
Select the relevant suggestion from the list.
Troubleshooting
If code completion doesn't work, this may be due to one of the following reasons:
The Power Save Mode is on ( ). Turning it on minimizes power consumption of your laptop by eliminating the background operations, including error highlighting, on-the-fly inspections, and code completion.
Your file doesn't reside in a content root , so it doesn't get the required class definitions and resources needed for code completion.
The file with the symbols to be used in code completion is marked as a plain text file.
The third-party file with the symbols to be used in code completion is not configured as an external JavaScript library.
Code completion popup might not appear automatically if it takes too long to gather the completion options. For example, if the computer is busy with another task. In this case, you may still activate the completion popup manually via Ctrl+Space hotkey.