Compiling TypeScript into JavaScript
Because browsers and Node.js process only JavaScript, you have to compile your TypeScript code before running or debugging it.
Compilation can also produce source maps that set correspondence between your TypeScript code and the JavaScript code that is actually executed.
WebStorm comes with a built-in TypeScript compiler. By default, it outputs generated JavaScript files and sourcemaps next to the TypeScript file.
Compilation is invoked with the Compile actions from the TypeScript widget on the Status toolbar as described in Compile TypeScript code below.
Compilation errors are reported in the TypeScript Tool Window. This list is not affected by changes you make to your code and is updated only when you invoke compilation again.
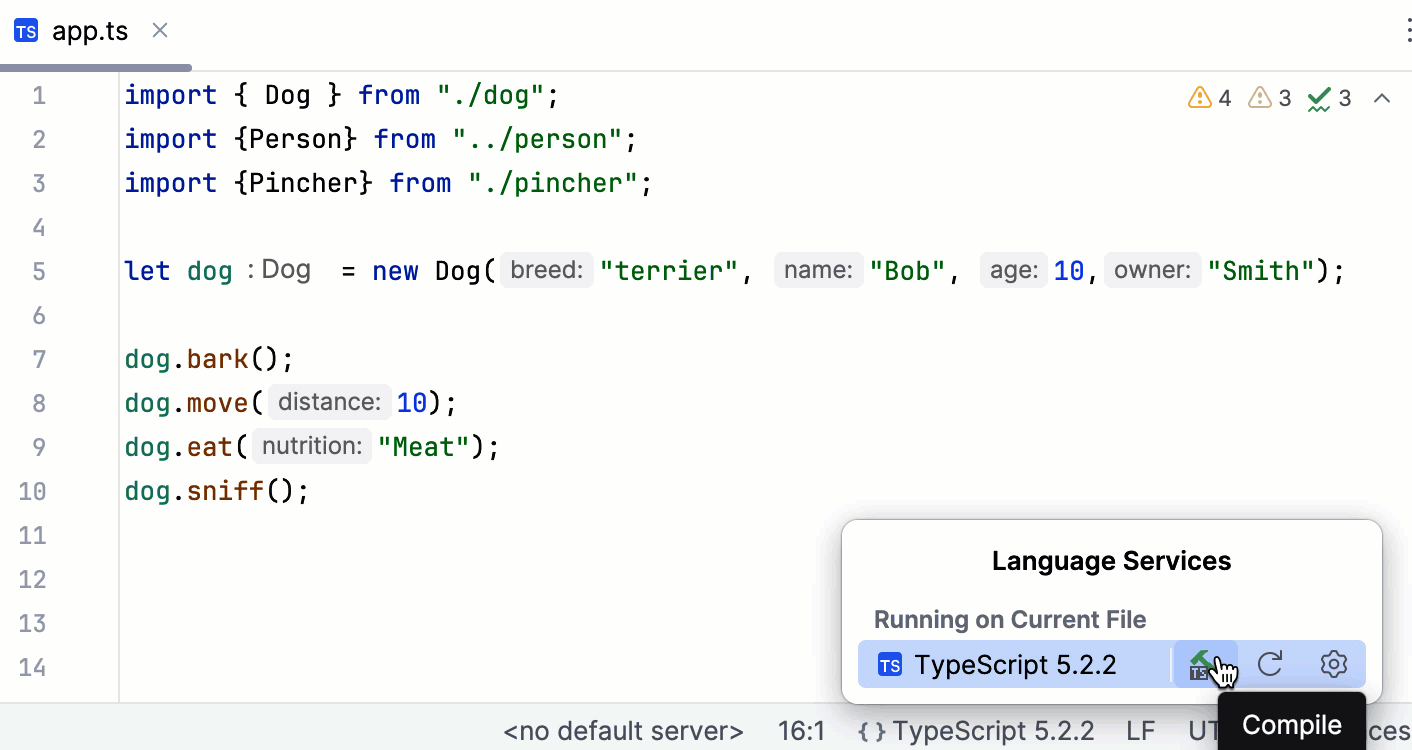
The tool window shows up only after you first compile your TypeScript code manually. After that the tool window is accessible via
in the main menu or via the tool window bar.Before you start
Press Ctrl+Alt+S to open settings and then select
.Make sure the TypeScript Language Service checkbox is selected.
Create and configure tsconfig.json files
By default, the built-in compiler does not create source maps that will let you step through your TypeScript code during a debugging session. The compiler also by default processes either the TypeScript file in the active editor tab or all TypeScript files from the current project.
With a tsconfig.json file, you can modify this default behavior to generate source maps and compile only files from a custom scope.
Create a tsconfig.json file
In the Project tool window, select the folder where your TypeScript code is (most often it is the project root folder) and then select
from the context menu.To generate source maps during compilation, make sure the
sourceMap
property is set totrue
.Optionally:
To override the default compilation scope, which is the entire project, add the
files
property and type the names of the files to process in the following format:"files" : ["<file1.ts>","<file2.ts>"],
Configure the scope for tsconfig.json
You may need to apply different TypeScript configurations to different files in your project.
It is not a problem if you arrange your sources so that all the files in each folder should be processed according to the same configuration. In such case you only have to create a separate tsconfig.json for each folder.
However if you want to apply different rules to the files that are stored in the same folder, you need to create several configuration files and configure scopes for them.
Create as many tsconfig*.json configuration files as you need.
Open the Settings dialog (Ctrl+Alt+S) , go to , and make sure the names of all these files match the patterns from the Typescript config file name pattern list.
If necessary, add patterns as described in Add file type associations.
In each *tsconfig*.json, specify the files to be processed according to its settings:
List the file names explicitly in the
files
field:"files" : ["<file1.ts>","<file2.ts>"],Learn more from TSConfig Reference: Files.
In the
include
field, specify the file names or patterns:"include" : ["<pattern1>, <pattern2>"]Learn more from TSConfig Reference: Include.
To skip some files whose names match the patterns listed in the
include
field, list their names or patterns in theexclude
field:"exclude" : ["<pattern3>, <pattern4>"]Learn more from TSConfig Reference: Exclude.
Compile TypeScript code
You can invoke compilation manually or have WebStorm compile your code automatically every time the code is changed.
Alternatively, you can configure a build process, for example, with webpack, babel, or another tool. Learn more from webpack with TypeScript and Babel with TypeScript.
Manual compilation
Click the Language Services widget on the Status bar.
Click
.
In the Compile TypeScript popup, select one of the following options:
To compile the TypeScript code of the entire application, select Compile All.
Alternatively, select Compile TypeScript from the context menu of any open TypeScript file.
To compile one file, select the path to it in the Compile TypeScript popup.
To compile files from a custom scope, make sure they are listed in the
files
property of your tsconfig.json as described above.In the Compile TypeScript popup, select the path to tsconfig.json.
Automatic compilation on changes
Open the Languages & Frameworks | TypeScript page of settings Ctrl+Alt+S and select the Recompile on changes checkbox.