ESLint
WebStorm integrates with ESLint which brings a wide range of linting rules that can also be extended with plugins. WebStorm shows warnings and errors reported by ESLint right in the editor, as you type. With ESLint, you can also use JavaScript Standard Style as well as lint your TypeScript code.
Besides JavaScript and TypeScript, ESLint can be applied to files of other types in the entire project or in its specific parts, refer to Configure linting scope.
Before you start
Make sure you have Node.js on your computer.
Configure a Node.js interpreter in your project as described in Configuring a local Node.js interpreter, or in Using Node.js on Windows Subsystem for Linux, or in Configuring remote Node.js interpreters.
Install ESLint
In the embedded Terminal (Alt+F12) , type one of the following commands:
npm install --g eslint
for global installation.npm install --save-dev eslint
to install ESLint as a development dependency.
Optionally, install additional plugins, for example, eslint-plugin-react to lint React applications.
Activate and configure ESLint in WebStorm
By default, ESLint is disabled. You can choose to configure it automatically or specify all the configuration settings manually.
Configure ESLint automatically
With automatic configuration, WebStorm uses the ESLint package from the project node_modules folder and the .eslintrc.* configuration file from the folder where the current file is stored. If no .eslintrc.* is found in the current file folder, WebStorm will look for one in its parent folders up to the project root.
If you have several package.json files with ESLint listed as a dependency, WebStorm starts a separate process for each package.json and processes everything below it. This lets you apply a specific ESLint version or a specific set of plugins to each path in a monorepo or a project with multiple ESLint configurations.
To configure ESLint automatically in the current project, open the Settings dialog (Ctrl+Alt+S) , go to , and select the Automatic ESLint configuration option.
To configure ESLint automatically in all new projects, open the Settings for New Projects dialog ( ) , go to , and select the Automatic ESLint configuration option.
Configure ESLint manually
With manual configuration, you can use a custom ESLint package, configuration file, and working directories, as well as apply various additional rules and options.
In the Settings dialog (Ctrl+Alt+S) , go to , and select Manual ESLint configuration.
In the ESLint package field, specify the location of the eslint or standard package.
In the Working directories field, specify the working directories for the ESLint process.
By default the field is empty and WebStorm detects the working directory automatically. First it looks for a directory closest to the linted file which contains a .eslintignore or .eslintrc.* file, or a package.json file with either a
eslintIgnore
or aeslintConfig
property.If the auto-detected working directory doesn't match your project configuration, you need to specify the working directory (directories) manually. Use semicolons as separators. The acceptable values are:
Absolute paths.
Paths relative to the project base directory (the parent folder of the .idea folder where WebStorm-specific project metadata is stored). For example:
./
: use the project base directory as the ESLint process working directory.client;server
: use <project_base_dir>/client and <project_base_dir>/server as working directories. For files that are neither under the client not under the server folder, the working directory will be auto-detected as described above.packages/*
: each subfolder of the <project_base_dir>/packages directory will be used as the working directory for the corresponding linted files.
Paths relative to content roots can be used if some linted files are not under the project base directory in the folder hierarchy.
Glob patterns that define relative paths to the working directories. For example, with
**/foo -*
each directory with the name starting withfoo-
will be used as the working directory for the corresponding linted files.
Choose the configuration to use.
Automatic search: select this option if ESLint rules are configured in a package.json or in a .eslintrc.* file. This can be a .eslintrc, .eslintrc.json, or .eslintrc.yaml file, or a file in another supported format. For more information, refer to the ESLint official website.
WebStorm looks for a .eslintrc.* file or for a
eslintConfig
property in a package.json. WebStorm starts the search from the folder where the file to be checked is stored, then searches in its parent folder, and so on until the project root is reached.Configuration File - select this option to use a custom file and specify the file location in the Path field.
Learn more about configuring ESLint from the ESLint official website.
Optionally:
In the Extra eslint options field, specify additional command-line options to run ESLint with, use spaces as separators.
Learn more about ESLint CLI options from the ESLint official website.
In the Additional rules directory field, specify the location of the files with additional code verification rules. These rules will be applied after the rules from package.json, .eslintrc.*, or a custom configuration file and accordingly will override them.
See the ESLint official website for more information about ESLint configuration files and adding rules.
Configure linting scope
Open the Settings dialog (Ctrl+Alt+S) , go to , and select Automatic ESLint configuration or Manual ESLint configuration.
In the Run for files field, specify the pattern that defines the set of files to be linted. You can accept the default pattern or type a custom one.
With the default pattern,
**/*.{js,ts,jsx,tsx,html,vue}
, ESLint will wake up and process any updated JavaScript, TypeScript, JSX, TSX, HTML, or Vue file. To lint files of other types or files stored in specific folders, use glob patterns to update the default pattern.For example, to automatically reformat CoffeeScript files as well, add
coffee
to the default pattern as follows:**/*.{js,ts,jsx,tsx,html,vue,coffee}To lint files from a specific folder, including subfolders, replace
**/*
with<path to the folder>/**/*
.Suppose, you have a project with the following structure:
To lint only the files in the coffee folder, update the pattern as follows:
coffee/*.{js,ts,jsx,tsx,html,vue,coffee}As a result, the file linting.coffee is linted while no_linting .coffee is not.
Fix problems automatically on save
ESLint can fix the detected problems every time your changes are saved either manually, with Ctrl+S, or automatically, when you launch a run/debug configuration, or close WebStorm, or perform version control actions For more information, refer to Autosave.
Open the Settings dialog (Ctrl+Alt+S) , go to , and select the Run eslint --fix on save checkbox.
Lint your code
When installed and enabled, ESLint activates automatically every time you open a JavaScript file.
By default, WebStorm marks detected problems based on the severity levels from the ESLint configuration. See Configuring ESLint highlighting to learn how to override these settings.
Descriptions of the errors detected in the current file and quick-fixes for them are available from the editor and from the File tab of the Problems tool window.
Errors in all previously opened files and quick-fixes for them are shown in the Project Errors tab of the Problems tool window. To open the tool window, click the Inspection widget in the upper-right corner of the editor:
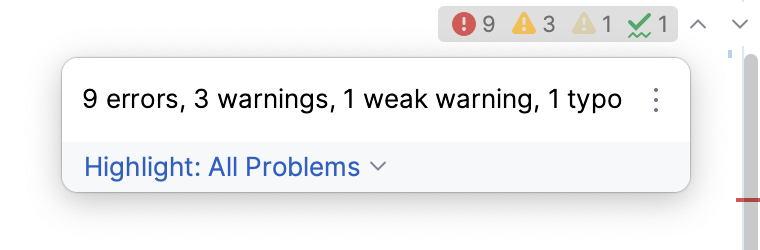
For more information, refer to View problems and apply quick-fixes in the editor and Problems tool window.
View problems and apply quick-fixes in the editor
To view the description of a problem, hover over the highlighted code.
To resolve the detected problem, click ESLint: Fix '<rule name>' or press Alt+Shift+Enter.
To resolve all the detected problems in the current file, click More actions (Alt+Enter) and select from the list.
For more information, refer to View problems and apply quick-fixes in the editor.
Alternatively open the File tab of the Problems tool window Alt+6, where you can view problem descriptions, apply quick-fixes, navigate to the fragments in the source code where errors occurred, as well as fix them in the Editor Preview pane without leaving the tool window. Learn more from Problems tool window.
You can also configure ESLint to fix all the problems in a file when this file is saved. To configure such behavior, select the Run eslint --fix on save checkbox on the ESLint page of the Settings dialog as described in Activating and configuring ESLint in WebStorm.
Lint your code in the Problems tool window
To open the Problems tool window, click the Inspections widget in the upper-right corner of the editor.
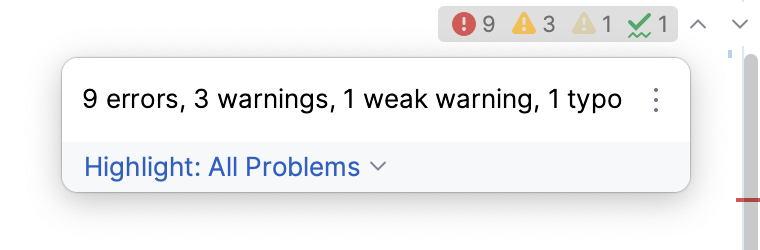
Alternatively select
from the main menu or press Alt+6.The Project Errors tab shows the errors in all files that were opened during the current session, with error messages grouped by files in which they were detected.
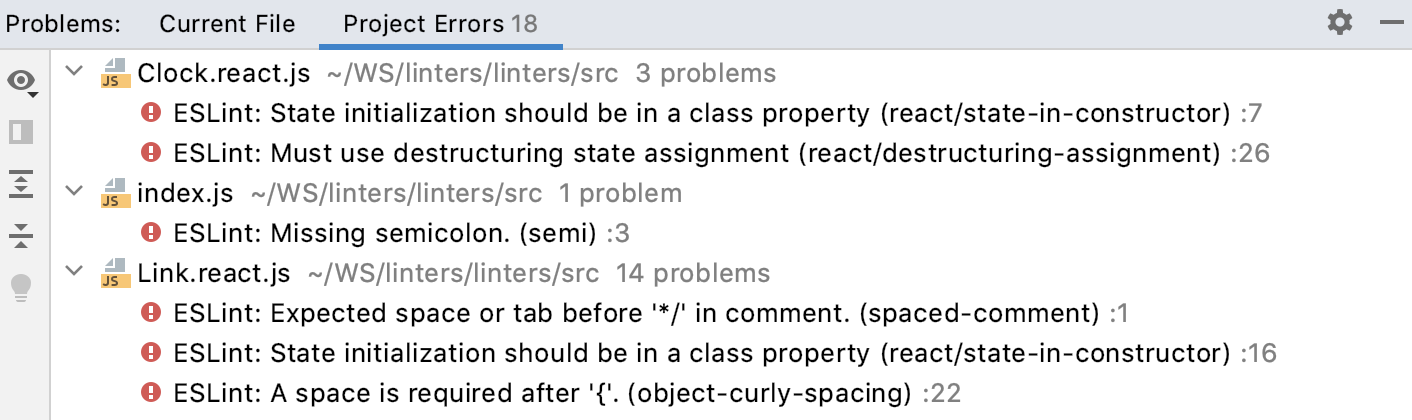
Here you can view problem descriptions, apply quick-fixes, navigate to the fragments in the source code where errors occurred, as well as fix them in the Editor Preview pane without leaving the tool window. Learn more from Problems tool window.
Configure highlighting for ESLint
By default, WebStorm marks the detected errors and warnings based on the severity levels from the ESLint configuration. For example, errors are highlighted with a red squiggly line, while warnings are marked with a yellow background. For more information, refer to Code inspections and Change inspection severity.
Change the severity level of a rule in the ESLint configuration
In .eslintrc or under
eslintConfig
in package.json, locate the rule you want to edit and set its ID to1
warn
or to2
error
.Learn more from the ESLint official website.
You can override the severities from the ESLint configuration so that WebStorm ignores them and shows everything reported by the linter as errors, warnings, or in a custom color.
Ignore the severity levels from the configuration
In the Settings dialog (Ctrl+Alt+S) , select . The Inspections page opens.
In the central pane, go to JavaScript | Code quality tools | ESLint.
In the right-hand pane, clear the Use rule severity from the configuration file checkbox and select the severity level to use instead of the default one.
Import code style from ESLint
You can import some of the ESLint code style rules to the WebStorm JavaScript code style settings. That enables WebStorm to use more accurate code style options for your project when auto-completing, generating, or refactoring the code or adding import statements. When you use the Reformat action, WebStorm will then no longer break properly formatted code from the ESLint perspective.
WebStorm understands ESLint configurations in all official formats: .eslintrc JSON files, package.json files with the eslintConfig
field, as well as JavaScript and YAML configuration files.
When you open your project for the first time, WebStorm imports the code style from the project ESLint configuration automatically.
If your ESLint configuration is updated (manually or from your version control), open it in the editor and choose Apply ESLint Code Style Rules from the context menu.
Alternatively, just answer Yes to the "Apply code style from ESLint?" question on top of the file.
The list of applied rules is shown in the Event log tool window:
Use JavaScript Standard Style
You can set JavaScript Standard Style as default JavaScript code style for your application so its main rules are applied when you type the code or reformat it. Since Standard is based on ESLint, you can also use Standard via the WebStorm ESLint integration.
Install JavaScript Standard
In the embedded Terminal (Alt+F12) , type:
npm install standard --save-dev
Learn more from the JavaScript Standard Style official website.
Enable linting with Standard via ESLint
If you open a project where standard
is listed in the project's package.json file, WebStorm enables linting with Standard automatically.
In the Settings dialog (Ctrl+Alt+S) , go to .
On the ESLint page that opens, select Manual ESLint configuration and specify the location of the
standard
package in the ESLint Package field.
Set the JavaScript Standard Style as default
In the Settings dialog (Ctrl+Alt+S) , go to .
On the JavaScript page, click Set from, and then select JavaScript Standard Style. The style will replace your current scheme.
ESLint with Docker
With WebStorm, you can run ESLint against your code inside a Docker container just in the same way as you do it locally.
Learn more from Node.js with Docker.
Make sure the Node.js, Node.js Remote Interpreter, and Docker required plugins are enabled on the Settings | Plugins page, tab Installed. For more information, refer to Managing plugins.
Download, install, and configure Docker as described in the Docker topic.
Configure a Node.js remote interpreter in Docker or via Docker Compose and set it as default in your project. Also make sure the package manager associated with this remote interpreter is set as project default.
Open your package.json and make sure ESLint is listed in the
devDependencies
section:{ "name": "node-express", "version": "0.0.0", "private": true, "dependencies": { "cookie-parser": "~1.4.4", "debug": "~2.6.9", "express": "~4.16.1", "http-errors": "~1.6.3", "morgan": "~1.9.1", "pug": "^3.0.2" }, "devDependencies": { "eslint": "^8.1.0" } }Right-click anywhere in the editor and select Run '<package manager> install' from the context menu.
After that, ESLint works in the same way as when you work with your code locally. View descriptions of detected discrepancies right in the editor or in the Problems tool window and apply suggested quick-fixes.