Generate code
IntelliJ IDEA provides multiple ways to generate common code constructs and recurring elements, which helps you increase productivity. These can be either file templates used when creating a new file, custom or predefined live templates that are applied differently based on the context, various wrappers, or automatic pairing of characters.
Additionally, IntelliJ IDEA provides code completion and Emmet support.
Here is a video that demonstrates how to generate various code constructs in IntelliJ IDEA:
Generate constructors
IntelliJ IDEA can generate a constructor that initializes specific class fields using values of corresponding arguments.
Generate a constructor for a class
In the main menu, go to Code and select Generate (Alt+Insert).
In the Generate popup, click Constructor.
If the class contains fields, select the fields to be initialized by the constructor and click OK.
The following code fragment shows the result of generating a constructor for a class:
Generate delegation methods
IntelliJ IDEA can generate methods that delegate behavior to the fields or methods of your class. This approach makes it possible to give access to the data of a field or method without directly exposing this field or method.
Generate a delegation method for a class
In the main menu, go to Code and select Generate (Alt+Insert).
In the Generate popup, click Delegate Methods.
Select the target field or method, and click OK.
Select the desired methods to be delegated and click OK.
The following code fragment shows the result of delegating the get(i)
method of the Calendar
class inside another class:
Generate equals() and hashCode() methods
The Java super class java.lang.Object
provides two methods for comparing objects:
public boolean equals(Object obj)
returnstrue
if the object passed to it as the argument is equal to the object on which this method is invoked. By default, this means that two objects are stored in the same memory address.public int hashCode()
returns the hash code value of the object on which this method is invoked. The hash code must not change during one execution of the application but may change between executions.
Generate equals() and hashCode() for a class
In the main menu, go to Code and select Generate (Alt+Insert).
In the Generate popup, click equals() and hashCode().
Select a velocity template from the Template list.
You can also click
to open the Templates dialog, where you can select an existing template or create a custom template.
Select an expression to generate within the method. Hover over the question mark icon to open a tooltip with an explanation of advantages and disadvantages of using each of the expressions.
Click Next.
Select the fields that should be used to determine equality, and click Next.
Select the fields to use for calculating the hash code value. You can choose only from fields that were selected on the previous step (for determining equality). Click Next.
Select the fields that contain non-null values. This optional step helps the generated code avoid checks for null and thus improves performance. Click Finish.
The following code fragment shows the result of overriding the equals()
and hashCode()
methods:
Generate getters and setters
IntelliJ IDEA can generate accessor and mutator methods (getters and setters) for the fields in your classes. Generated methods have only one argument, as required by the JavaBeans API.
The getter and setter method names are generated by IntelliJ IDEA according to your code generation naming preferences.
In the main menu, go to Code and select Generate (Alt+Insert).
In the Generate popup, click one of the following:
Getter to generate accessor methods for getting the current values of class fields.
Setter to generate mutator methods for setting the values of class fields.
Getter and Setter to generate both accessor and mutator methods.
Select the fields to generate getters or setters for and click OK.
You can add a custom getter or setter method by clicking
and accessing the Getter/Setter Templates dialog. If a field is not in the list, then the corresponding getter and setter methods are already defined for it.
The following code fragment shows the result of generating the getter and setter methods for a class with one field var
:
Generate toString()
The toString()
method of the Java super class java.lang.Object
returns the string representation of the object. This method can be used to print any object to the standard output, for example, to quickly monitor the execution of your code. By default, toString()
returns the name of the class followed by the hash code of the object. You can override it to return the values of the object's fields, for example, which can be more informative for your needs.
Override the toString() method for a class
On the Code menu, click Generate Alt+Insert.
In the Generate popup, click toString().
Configure the following:
Select the template for generating the
toString()
method from the Template list.Select the fields that you want to return in the generated
toString()
method. By default, all the available fields are selected. Click Select None to generate atoString()
method that returns only the class name.Select the Insert @Override checkbox if necessary.
Click the Settings button to open the toString() Generation Settings dialog. where you can tune the behavior and add custom templates.
Click OK.
If the toString()
method is already defined in the class, by default, you will be prompted whether you would like to delete this method before proceeding. You can use the When method already exists group of options in the toString() Generation Settings dialog to change this behavior: either automatically replace existing method or generate a duplicating method.
The following code fragment shows the result of generating the toString()
method for a class with several fields defined:
The following code inspections are related to the toString()
method:
Class does not override 'toString()' method can be used to identify classes in which the
toString()
method is not defined. This inspection uses the exclude settings to ignore classes with fields that are not supposed to be dumped. An additional setting is to exclude certain classes using a regular expression matching their class name. As default, this is used to exclude any exception classes.Field not used in 'toString()' method can be used to identify fields that are not dumped in the
toString()
method. For example, if you added new fields to a class, but forgot to add them to thetoString()
method. Change the severity of this inspection to show errors as warnings. This will highlight any unused fields in the editor and indicate their location as yellow markers on the scroll bar.
Custom code generation templates
Templates used for generating getters and setters, as well as equals()
, hashCode()
, and toString()
methods are written in the Velocity template language. Although you can't modify predefined templates, you can add your own custom templates to implement necessary behavior.
IntelliJ IDEA provides the following variables for Velocity templates:
The following variables can be used in templates for generating getters and setters:
Variable | Description |
---|---|
| The current version of the Java Runtime Environment (JRE). |
| The current class. |
| Provides access to various code generation helper methods. |
| Provides the ability to format names according to the current code style. |
| Field for which getter or setter is generated. |
The following variables can be used in templates for generating the toString()
method:
Variable | Description |
---|---|
| The current version of the Java Runtime Environment (JRE). |
| The current class. |
| Provides access to various code generation helper methods. |
| Provides the ability to format names according to the current code style. |
| List of fields in the current class. |
The following variables can be used in templates for generating the equals()
method:
Variable | Description |
---|---|
| The current version of the Java Runtime Environment (JRE). |
| The current class. |
| Provides access to various code generation helper methods. |
| Provides the ability to format names according to the current code style. |
| List of fields in the current class. |
| Predefined name of the object on which the |
| Predefined name of the |
| The name of the parameter in the |
| Option passed from the wizard. |
| Whether the superclass has |
The following variables can be used in templates for generating the hashCode()
method:
Variable | Description |
---|---|
| The current version of the Java Runtime Environment (JRE). |
| The current class. |
| Provides access to various code generation helper methods. |
| Provides the ability to format names according to the current code style. |
| List of fields in the current class. |
| Whether the superclass has |
Productivity tips
Use code completion
Depending on the current context, IntelliJ IDEA can suggest generating relevant code constructs in the code completion popup. For example, when the caret is inside a Java class, the completion popup will contain suggestions for adding getters, setters, equals()
, hashCode()
, and toString()
methods.
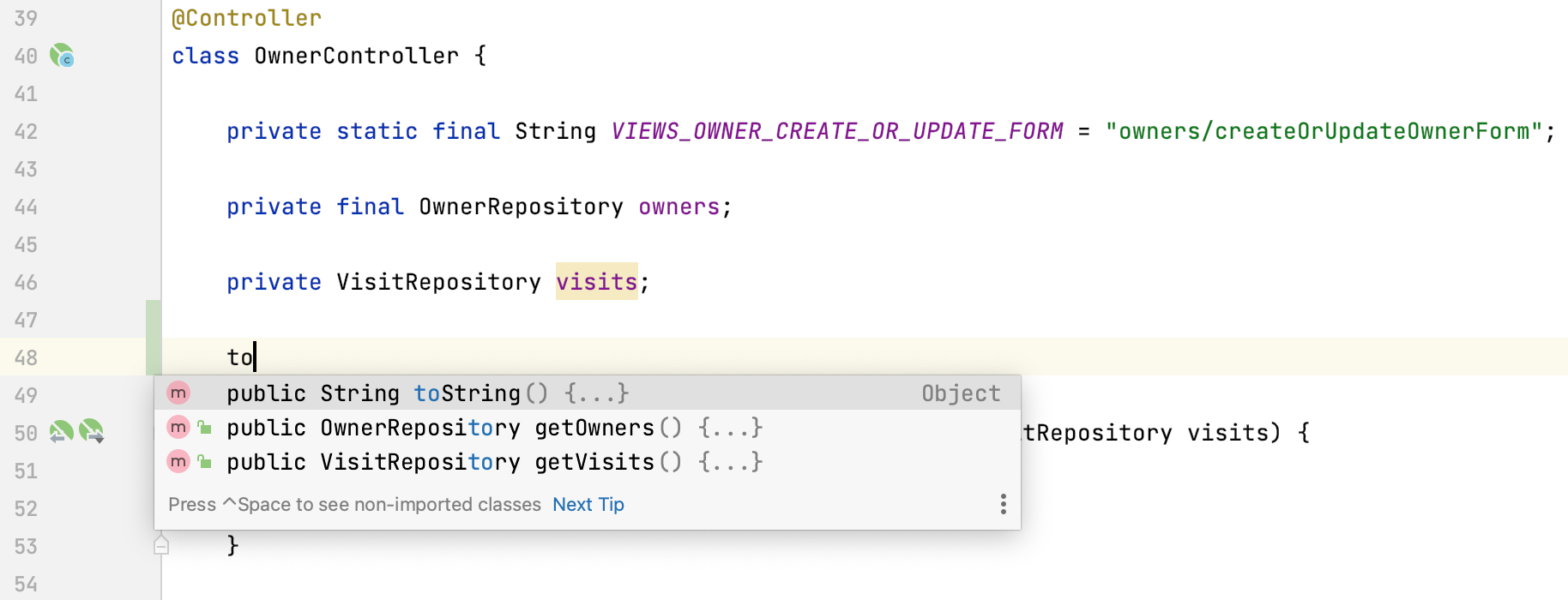