Code analysis in C# string literals
In system string formatting methods
JetBrains Fleet analyzes format strings and arguments of all .NET string formatting methods, such as String.Format
, Text.StringBuilder.AppendFormat
, or Console.WriteLine
.
In usages of string formatting methods, JetBrains Fleet highlights format placeholders and also synchronously highlights placeholder with the corresponding argument when your caret is at either of them:

If arguments and format placeholders mismatch (which leads to the FormatException
in runtime if arguments are missing), JetBrains Fleet generates warnings for missing or redundant arguments:

You can easily fix this problem by pressing ⌥ ⏎ over the warning. JetBrains Fleet will suggest either to automatically add the missing argument or to remove the mismatched format placeholder.
JetBrains Fleet also helps you detect and remove redundant calls of string formatting methods inside other string formatting methods. For example:

In custom string formatting methods
To enable code analysis and assistance features in custom string formatting methods, use the [StringFormatMethod]
and [StructuredMessageTemplate]
attributes from the JetBrains.Annotations namespace.
Consider a custom string formatting method ShowError
:
If the method is called incorrectly, as shown below, JetBrains Fleet has no chance to detect the missing argument:

Make JetBrains Fleet aware of a custom string formatting method
Reference the
JetBrains.Annotations
namespace as described in the Annotations in C# source code section.Annotate your custom string formatting method with the
[StringFormatMethodAttribute]
attribute, which takes a single argument — the name of the format string parameter:[StringFormatMethod("formatString")] public void ShowError(string formatString, params object[] args) { // some custom logic Console.WriteLine(formatString, args); }JetBrains Fleet will be able to warn you about missing arguments when this custom formatting method is invoked.
Alternatively, annotate the parameter that accepts a formatted string with the
[StructuredMessageTemplateAttribute]
:void LogNewUser([StructuredMessageTemplate] string message, params string[] args) { // Log new user } void Test() { // Warning: Non-existing argument in format string LogNewUser("User created: {username}"); }This second approach allows you to use custom strings as placeholders, as
username
in the example above.
In interpolated strings
Modern versions of C# provide a more elegant alternative to the String.Format
method — interpolated strings. That's why JetBrains Fleet highlights usages of String.Format
with the suggestion to convert them into interpolated strings. You can use a quick-fix to apply the conversion with a couple of keystrokes.
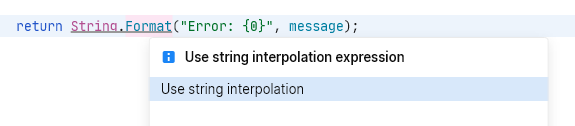
If you need to quickly add the $
at the beginning of the string when you are deep into typing out the string, you can press ⌥ ⏎ and choose To string interpolation.
