Code completion in C#
JetBrains Fleet's code completion in C# suggests names of classes, methods, variables, keywords, and any other symbols that are available within the current visibility scope including extension methods that were previously imported. Many other context-specific suggestions, like live templates, code generation suggestions, unit test categories are also available in the completion list.
Completion suggestions will appear automatically as you type, but you can also invoke them explicitly by pressing ⌃ Space.
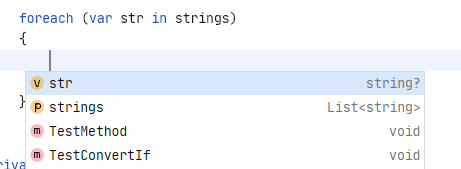
You can use CamelHumps in code completion, that is you can type the initial letters of compound name parts and the name will appear in the list of suggestions. For example, you can type tes
and then pick TextEncoderSettings
from the completion list.
When you use code completion over existing code items, you can either insert the selected completion suggestion before the existing item by pressing Enter or replace the existing identifier with the selected suggestion by pressing Tab.
Apart from suggesting types, members, and identifiers, code completion helps you generate different code constructs with a couple of keystrokes. Below are some examples.
Overriding and implementing members
In the example below, code completion helps create an override for a virtual member from a base class. Start typing the base method name in a derived type, and you will get a suggestion to override it:
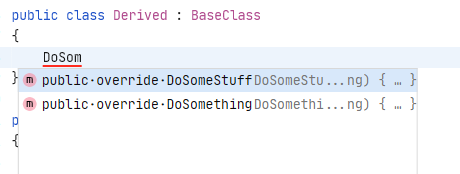
After you accept the suggestion, the method body with the default implementation expands in the editor:
Properties for fields
To generate properties for a field, start typing the name of the field. JetBrains Fleet will suggest creating a read-only or a read-write property with the corresponding name according to your naming style:
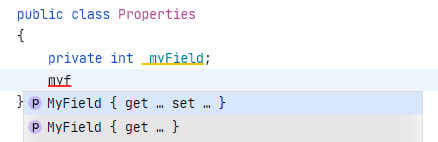
After you accept the suggestion, the property body expands in the editor:
Type constructors
Depending on the existing members of the current type, JetBrains Fleet suggests different constructors in the completion list. To create a constructor, type ctor
. In the completion list, you may see the following suggestions:
ctor
— a constructor without parametersctorf
— a constructor that initializes all fieldsctorp
— a constructor that initializes all auto-propertiesctorfp
— a constructor that initializes all fields and auto-properties
In the example below, all kinds of constructors are available.
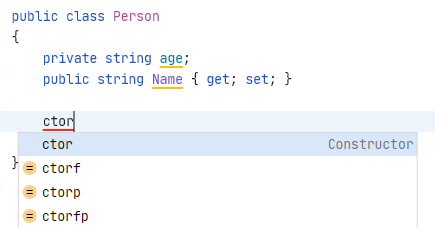
If you accept the ctorfp
suggestion, the constructor expands in the editor:
Generate equality and flag checks for enumeration types
When you need to compare a value of enum type to one of the members of this enum, just type a dot and then pick the desired enum member in the completion list:
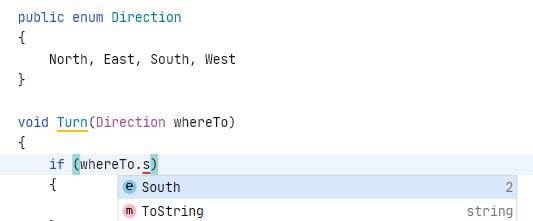
JetBrains Fleet will generate the comparison for you:
Templates in completion lists
All your live templates, postfix templates, and source templates appear in the completion list. Templates are identified by their shortcuts (here are the list of shortcuts of predefined templates).
For example, to invoke the public static void Main template, type its shortcut psvm
:
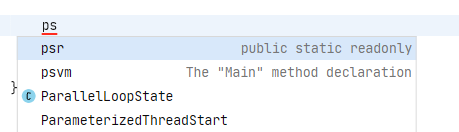
After you accept the suggestion, the Main
method expands in the editor: