How to use type parameters for generic programming
By using type parameters, you can write functions that handle incoming parameters without depending on the type specified in the function declaration.
For example, the PrintSliceInts
function receives a slice of integers and prints it.
To apply the same functionality to a slice of strings, you need to copy and paste the code of PrintSliceInts
and make a new function called PrintSliceStrings
, where everything is the same except for the signature.
You can rewrite the function and reuse your code. All you need is to introduce the type parameter and change the function parameter in the signature.
Run code in GoLand
Ensure that you installed and use Go 1.18 beta 1 or later. You can check your current Go version by opening settings and navigating to
. To open settings, press Ctrl+Alt+S.Also, check that the go.mod file (if you use Go modules) references Go 1.18 beta 1 or later.
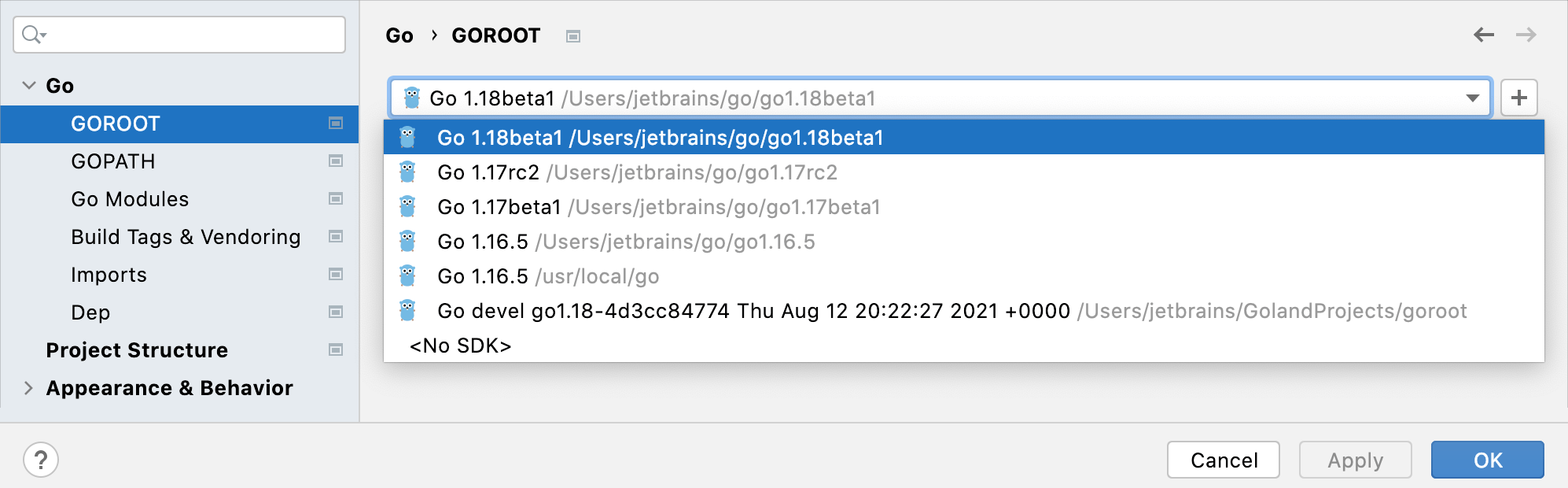
Click the Run Application icon (
) in the gutter and select Run 'go build project_name'. Alternatively, press Ctrl+Shift+F10.
Run code in the go.dev playground
You can open go.dev/play and run your code here. Note that before running you need to select Go dev branch.
Alternatively, use the Share in Playground action in GoLand.
In the editor, press Ctrl+Shift+A to search for actions.
Type Share in Playground and press Enter. Alternatively, use the following shortcut in the editor: Ctrl+Alt+Shift+S.
At go.dev, select Go dev branch, and click the Run button.