Using extract and inline refactorings
With extract and inline refactorings, you can quickly clean up your code and make it more streamlined or explicit.
Extract a variable
Select and right-click the following code fragment:
&User{ ID: 1, Name: "Smith",}
.Navigate to
.Type u as a name for a new variable.
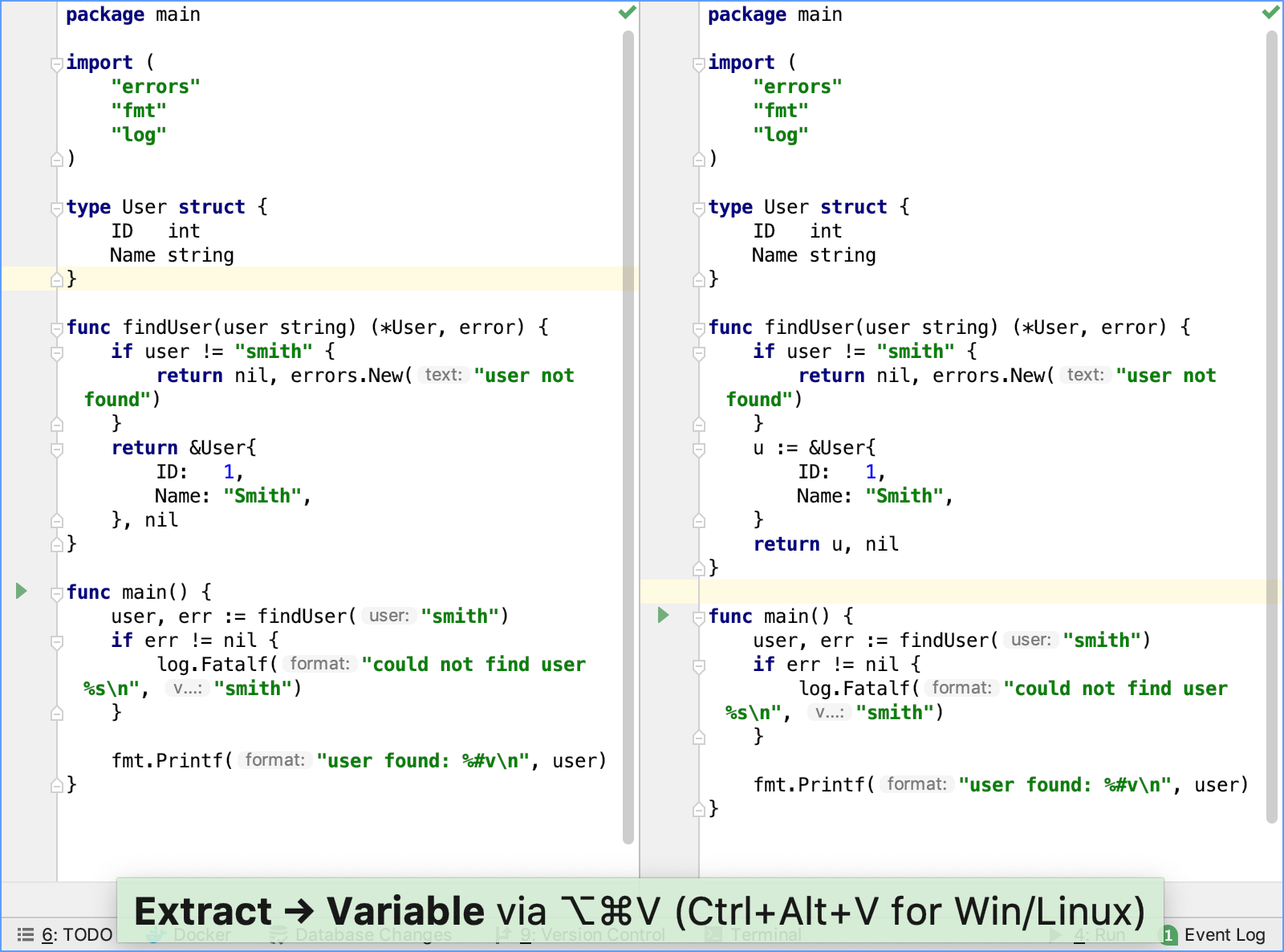
Code snippet:
Extract methods
Select and right-click the following code fragment:
&User{ ID: 1, Name: "Smith",}
.Navigate to
.
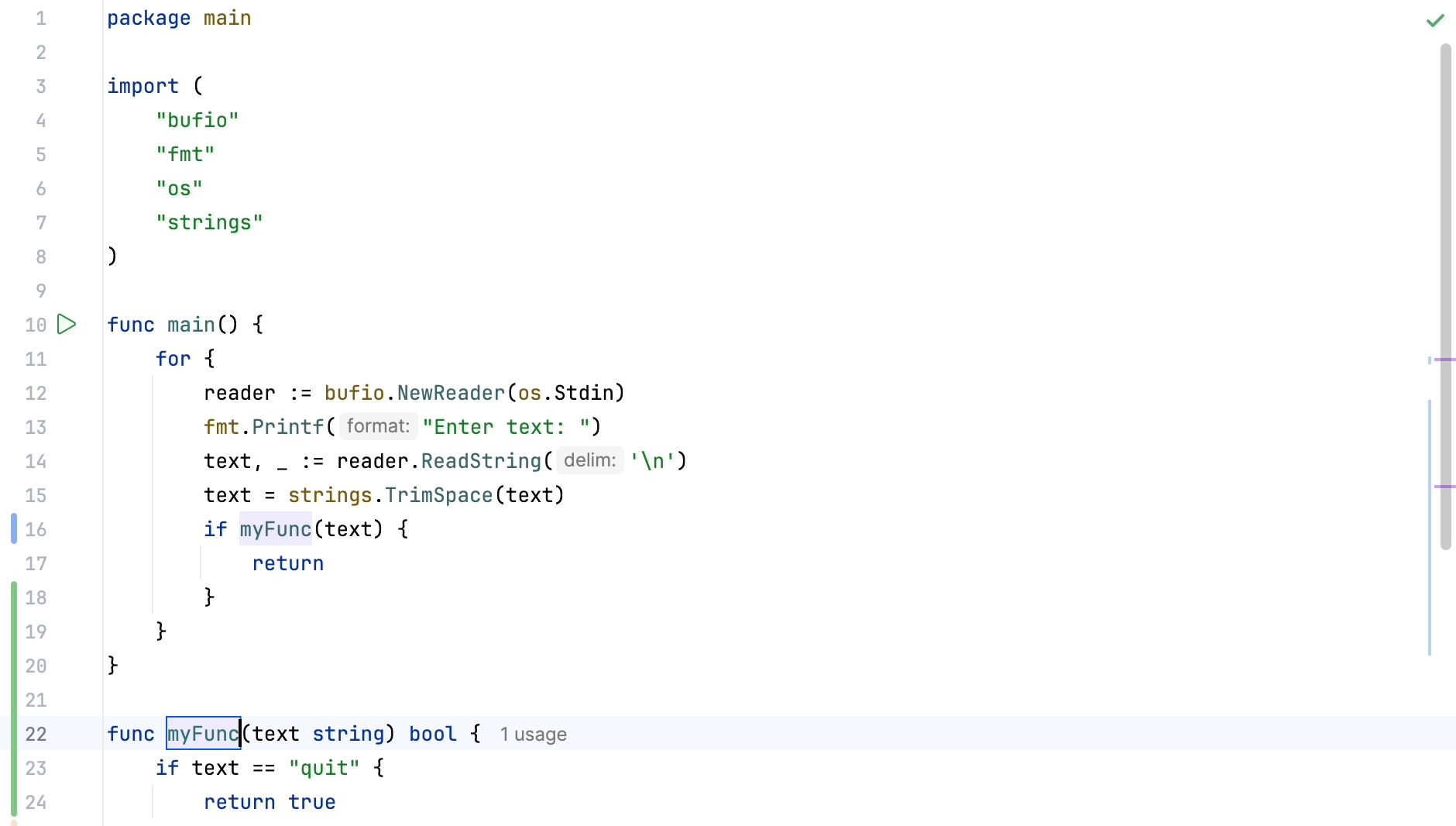
Code snippet:
Extract a constant
In the editor, select an expression or declaration of a variable you want to replace with a constant.
Press Ctrl+Alt+C to introduce a constant or select
.Select an expression you want to extract as constant and press Enter. Select the number of occurrences you want to replace and a name you want to use.
Extract functions and methods
In the editor, select an expression or its part that you want to extract. You can also place the caret within the expression, in this case GoLand offers you a list of potential code selections.
Press Ctrl+Alt+M or go to
in the main menu.Type a method name and press Enter.