Dockerize a Java application
You can use Docker to pack your compiled Java application into an image along with a specific runtime environment and any other necessary dependencies. You can then run a container from that image to see how the application will run in this environment. This is called dockerizing an application.
This tutorial describes how to create a Dockerfile to build a Docker image with OpenJDK 17 and a compiled Java application. It also shows how to share this image with others and run a Docker container from it.
Create a new Java project
The sample application for this tutorial will consist of a single HelloWorld.java file, which prints Hello, World!
to the console and exits.
In the main menu, go to
.In the New Project wizard, select Java from the list on the left.
In the New Project dialog, name the project
DockerHelloWorld
.Create the main Java class file HelloWorld.java in the src directory.
To do this, in the Project tool window, right-click the src directory, point to New and click Java Class. In the New Java Class dialog, type
HelloWorld
and press Enter.Paste the following code into the new file:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }Try to compile and run the application.
Click
in the gutter and select Run 'HelloWorld.main()'.
You should see
Hello, World!
printed to the console of the Run tool window.
By default, IntelliJ IDEA compiles the output to the HelloWorld.class file in the project directory under /out/production/DockerHelloWorld/, where DockerHelloWorld
is the name of the current module.
Create a Dockerfile
In the Project tool window, right-click the project name, point to New and click File.
In the New File dialog, type Dockerfile and press Enter.
Paste the following code to the new Dockerfile:
FROM openjdk:17 COPY ./out/production/DockerHelloWorld/ /tmp WORKDIR /tmp ENTRYPOINT ["java","HelloWorld"]
This Dockerfile contains instructions for building an image based on the openjdk:17
image from Docker Hub. When you run a container from this image, Docker copies the contents of your project's output directory to the /tmp directory in the container (in this case, the output directory contains the main class HelloWorld.class). Then Docker sets the current working directory to /tmp and runs java HelloWorld
. As a result, you should see Hello, World!
printed to the container log.
Build and run the image
In the gutter inside the Dockerfile, click
and select Run on 'Docker'.
IntelliJ IDEA creates a Dockerfile run configuration, which builds an image from the Dockerfile and then runs a container based on that image. To see the whole process, open the Log tab in the Services tool window.
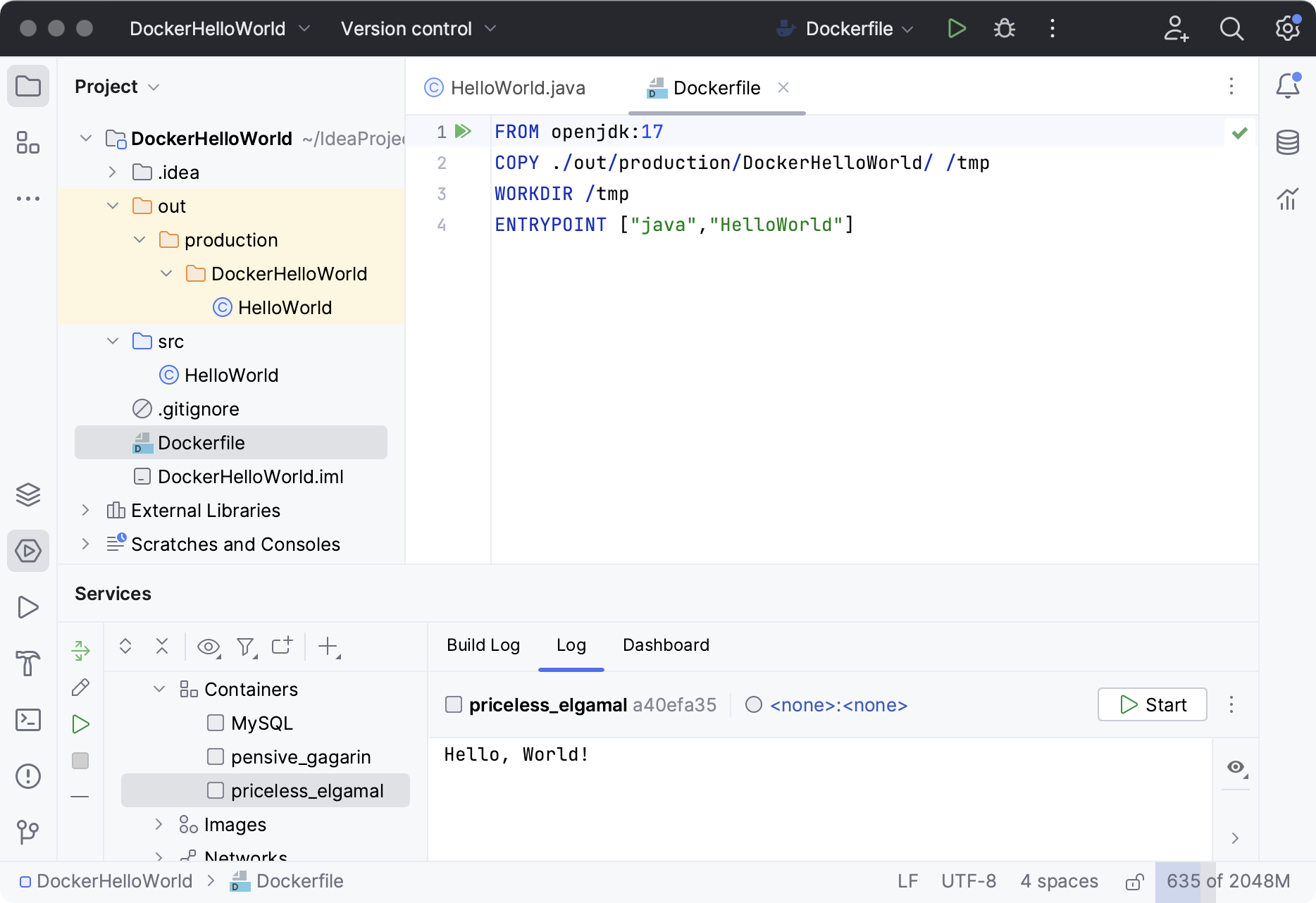
You can share the image with others, for example, to demonstrate exactly how your application is expected to run, without the need to install the necessary runtime (only Docker is required).
Share your Java application as a Docker image
In the Services tool window, find the image that was built from the Dockerfile.
By default, it is designated by a unique image ID, because no image tag was provided when you created the image. You can edit the corresponding run configuration, specify an image tag of your choice, and run the configuration again.
To find the image, right-click the running container and select
.Right-click the image with the required ID and then click Push Image in the context menu.
In the Push Image dialog, select your registry, specify the repository name and tag for the image, and click OK.
Once Docker pushed the image to the registry, anyone with access to it can pull it and run a container from this image. You can be sure that they will run the exact version of your application on the specific runtime version that you've set in the Dockerfile.