Spring Boot
Spring Boot is an extension of the Spring framework that simplifies the initial configuration of Spring applications. It enables you to quickly create a working standalone Spring application with minimum default configuration.
Spring Initializr is a web application that can generate a Spring Boot project. You can select the necessary configuration, including the build tool, language, version of the Spring Boot framework, and any dependencies for your project. IntelliJ IDEA provides the Spring Initializr project wizard that integrates with the Spring Initializr API to generate and import your project directly from the IDE.
Create a new Spring Boot project via the Spring Boot wizard
In the main menu, go to
.In the left pane of the New Project wizard, select Spring Boot.
Go through the steps of the Spring Boot project wizard.
For an example, refer to Tutorial: Create your first Spring application.
Spring Initializr generates a valid project structure with the following files:
A build configuration file, for example, build.gradle for Gradle or pom.xml for Maven.
A class with the
main()
method to bootstrap the application.An empty JUnit test class.
An empty Spring application configuration file: application.properties
By default, IntelliJ IDEA applies code formatting to the generated files. If you want the files to remain formatted as they are generated by Spring Initializr, open the IDE settings with Ctrl+Alt+S, select and disable the Reformat code when creating a new project option.
Run a Spring Boot application
Open the class with the
main()
method (it is usually also designated with the@SpringBootApplication
annotation), clickin the gutter, and select to run the class.
Alternatively, you can press Ctrl+Shift+F10 with the class file open in the editor.
IntelliJ IDEA creates and executes the Spring Boot run configuration. For more information, refer to Spring Boot run configuration.
Use Gradle to run Spring Boot
By default, for Spring Boot Gradle-based applications, IntelliJ IDEA uses Gradle to build the project and IntelliJ IDEA to run it. You can configure the IDE to use Gradle instead of IntelliJ IDEA to run Spring Boot applications.
Go to the Advanced Settings page of settings Ctrl+Alt+S.
Under Frameworks. Spring Boot select Run using Gradle.
The Run using Gradle checkbox has no effect if IntelliJ IDEA is selected in . In this case, IntelliJ IDEA will be used to both build and run your Spring Boot application.
Custom configuration files
Spring Initializr creates one default configuration file that may not always be sufficient for development. If you do not want to use the default configuration file, or if you want to run your code in different environments, you can use custom configuration files defined in your project.
Let IntelliJ IDEA know which files are configuration files in your project to enable relevant highlighting and coding assistance:
Define project configuration files
In the main menu, go to Project Structure dialog.
or press Ctrl+Alt+Shift+S to open theAdd the Spring facet: from the left-hand list, select Facets, click
, and select Spring.
In the right-hand section, select Configuration Files and click
(Customize Spring Boot) in the toolbar.
If you want to use a custom configuration file instead of the default one, type its name in the
spring.config.name
field.If you want to use multiple configuration files, click
and select files from the project tree.
Valid configuration files are marked with
.
Click OK and apply the changes.
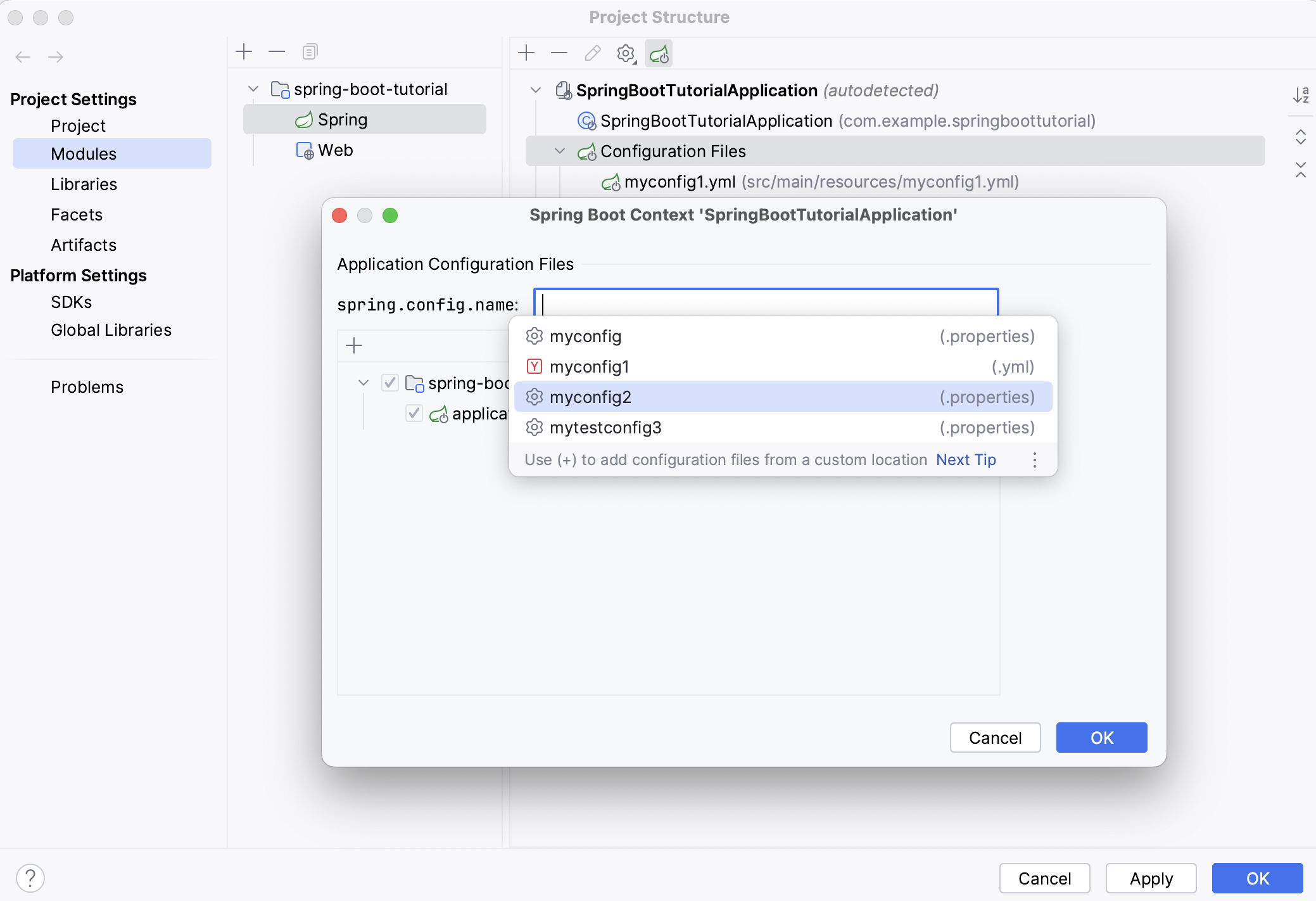
Runtime endpoints
Spring Boot includes additional features for monitoring and managing the state of your application in the production environment through HTTP endpoints or with Java Management Extensions (JMX). For more information, refer to Spring Boot Actuator: Production-ready Features.
Enable the Spring Boot actuator endpoints
In your pom.xml or build.gradle file, click the Edit Starters inlay hint next to the
dependencies
list.Alternatively, press Alt+Insert anywhere in the file and select Edit Starters.
In the window that opens, select Spring Boot Actuator.
Or you can add the spring-boot-starter-actuator
dependency manually:
Open the pom.xml file and add the following dependency under dependencies
:
Open the build.gradle file and add the following dependency under dependencies
:
Open the build.gradle.kts file and add the following dependency under dependencies
:
When you run your application with this dependency, you will be able to access the exposed actuator endpoints via HTTP. For example, if the application is running on localhost port number 8080, the default URL for the health
endpoint will be http://localhost:8080/actuator/health.
View the Spring Boot actuator endpoints
Run your Spring Boot application and open the Services tool window: select or press Alt+8.
Select your running Spring Boot application and open the Actuator tab.
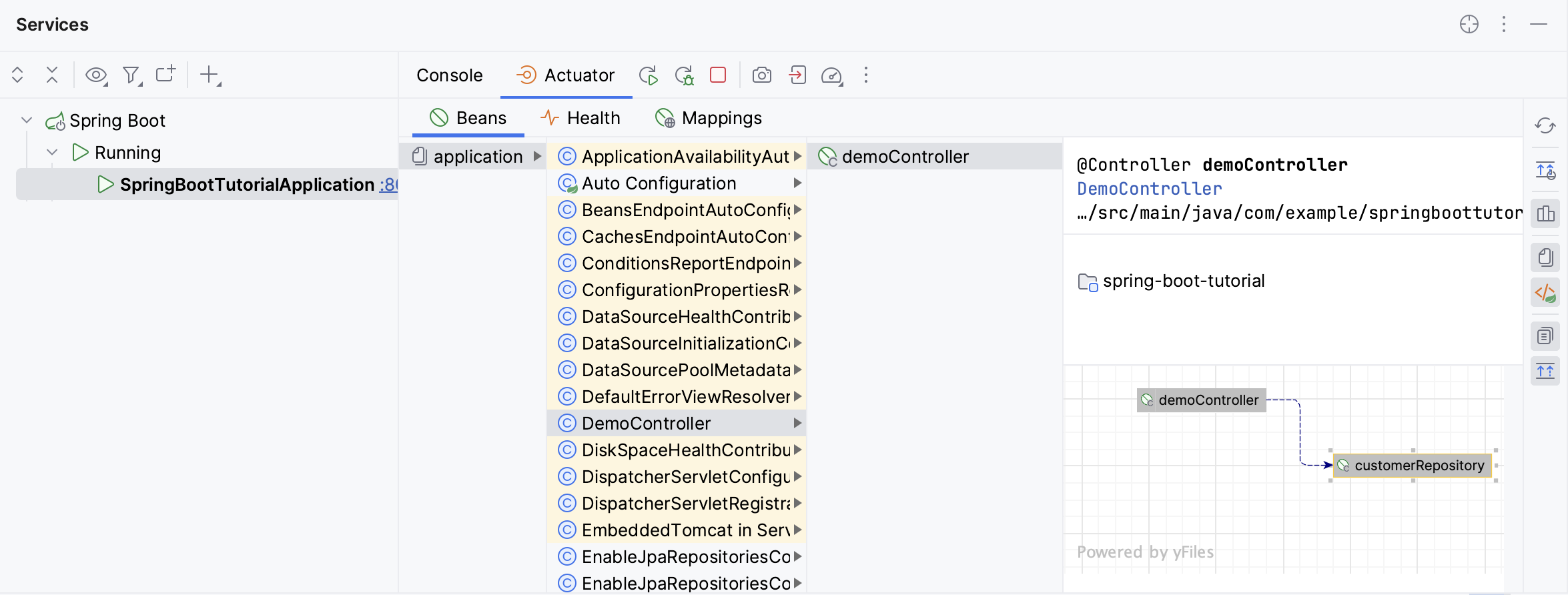
You can use tabs to view endpoints of the following types: runtime beans, health information, and request mappings.
Beans
The Beans tab under Actuator shows the runtime beans of your Spring Boot application. Double-click any bean to open its declaration in the editor. These beans are indicated using the icon in the gutter. Click this icon to view dependent and injected beans.
The Beans tab includes the following toolbar actions:
Action | Description |
---|---|
| Refresh the runtime beans information collected by the JMX agent. |
| Show the complete graph for all your runtime beans instead of a list. Required plugin: Diagrams (bundled). |
| Show beans from libraries. |
| Show available Spring application contexts. |
| Show available configuration files. |
| Show the documentation for the selected bean. |
| Show the direct dependencies for the selected bean. Required plugin: Diagrams (bundled). |
Health
The Health tab under Actuator shows the status of your application. There are some auto-configured health indicators and you can also write custom health indicators.
For more information, refer to Health.
Mappings
The Mappings tab under Actuator shows the request mappings of your application. It lists all methods with the @RequestMapping
annotation or its shortcuts, such as @GetMapping
.
If you click the path mapping URI, you can select to run the corresponding HTTP request, open an HTTP requests file with the request, or open the request URL in the web browser (if it's a GET
request). For more information, refer to HTTP Client.
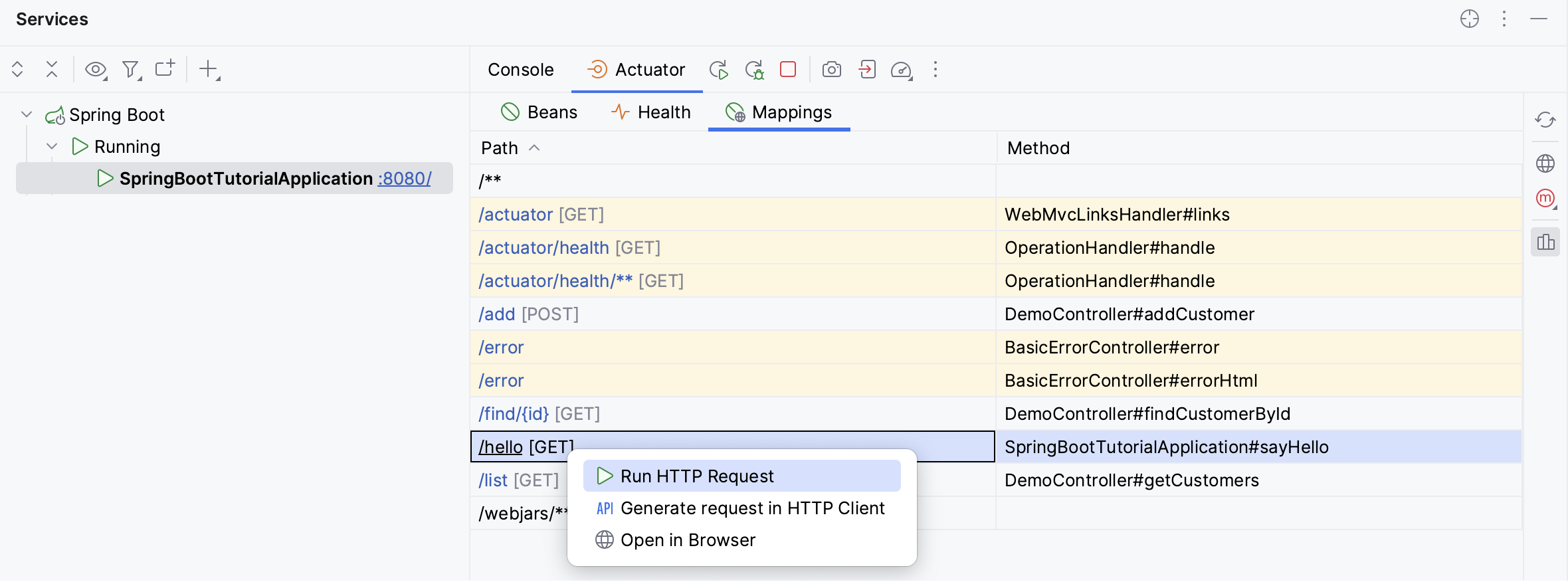
Double-click a method to open its declaration in the editor. Spring registers such methods as handlers and IntelliJ IDEA indicates them with the icon in the gutter. Click this icon to run the corresponding HTTP request, open it in a requests files, or in the web browser (if it's a
GET
request).
The Mappings tab includes the following toolbar actions:
Action | Description |
---|---|
| Refresh the request mappings collected by the JMX agent. |
| Open the root application URL in a web browser. |
| Select which request methods to show. |
| Show request mappings from libraries. |
Application update policies
With the spring-boot-devtools
module, your application will restart every time files on the classpath change. If IntelliJ IDEA is configured to continuously compile changed files, you can set a trigger file. In this case, your application will restart only after you modify the trigger file. For more information, refer to Automatic Restart.
Enable automatic restart
Add the
spring-boot-devtools
module dependency for your project.Open the pom.xml file and add the following dependency under
dependencies
:<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <optional>true</optional> </dependency>Setting the
spring-boot-devtools
dependency asoptional
prevents it from being used in other modules that use your project.Open the build.gradle file and add the following dependency under
dependencies
:developmentOnly("org.springframework.boot:spring-boot-devtools")Setting the
spring-boot-devtools
dependency asdevelopmentOnly
prevents it from being used in other modules that use your project.
To update a running application, go to Services tool window and click . Depending on your needs, you can configure what the IDE will do when you execute this action.
Configure the application update policy
In the main menu, go to
.Select the necessary Spring Boot run configuration to open its settings. Click Modify options.
In the list that opens, point to On 'Update' action. You can choose to update only the resources, update both the classes and the resources (build your application), update the trigger file (which will trigger a restart), or try to perform a class hot swap, and if it fails, update the trigger file.
In the Modify options list, point to On frame deactivation and select an action that the IDE will do after you switch to another application: update the resources, or build your application.