Refactorings for TypeScript
Perform a refactoring
Place the caret at a symbol, select a code fragment that you want to refactor, or select an item in a tool window.
Do one of the following:
From the main menu, choose
, and then select a desired refactoring. The list of refactorings available in this menu depends on the current context. If ReSharper cannot suggest any refactorings for the context, the entire menu is disabled.In the editor, File Structure window, or other ReSharper window, right-click the item you want to transform, choose Refactor from the context menu, and then select the required refactoring.
From the main menu, choose Refactor This in the context menu of a selection.
, or press Control+Shift+R to display the list of applicable refactorings, then select one of them. You can also chooseUse default keyboard shortcuts assigned to specific refactorings, or assign custom shortcuts to your favorite refactoring commands.
If the selected refactoring requires user input, the refactoring wizard opens. Note that the wizard's dialogs are not modal, so you can edit the code while the wizard is open.
To roll back refactoring actions, the wizard provides the option To enable Undo, open all files with changes for editing. If you select this option, ReSharper opens all modified files in new editor tabs and enables you to roll the refactoring back. In this case, you will need to save the changes yourself. If this option is not selected, ReSharper saves modified files automatically, without opening them.
If a refactoring operation would cause code conflicts (such as duplicate names, visibility conflicts, and so on), the wizard displays the list of conflicts on the last step, before you apply the refactoring. For some conflicts, the wizard can also suggest quick-fixes. For more information, refer to Resolve conflicts in refactorings.
Some refactorings are available immediately after you modify code in the editor. For more information, refer to Inplace refactorings
Copy type
This refactoring allows you to create a copy of selected type, and to place it into a specified module.
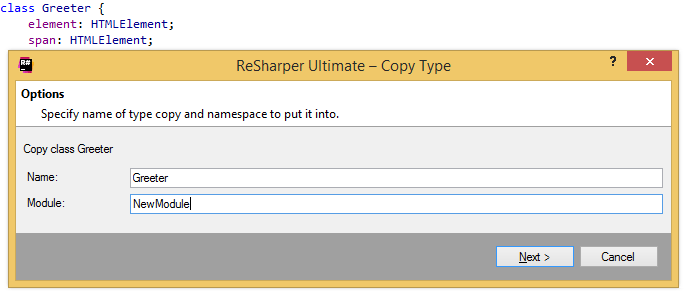
Introduce field
This refactoring allows you to create a new field based on a selected expression, initialize it with the expression or from the constructor, and replace occurrences of the expression in the current type with references to the newly introduced field.
You can also invoke this refactoring with the dedicated shortcut Control+Alt+D.
In the example below, we use this refactoring to replace two occurrences of the same string with a new constant field:
Introduce Type Alias
This refactoring helps you create a type alias for a type combination and replace the currently selected combination or all similar combinations in the current scope with the alias.
To invoke this refactoring, select a type intersection or union, a literal, or other entity that can be aliased and press Control+Shift+R or choose Introduce type alias in the Refactor This popup. If there are multiple occurrences of the selected entity in the current context, you will be able to choose whether to replace the current entity or all entities.
from the main menu , and then selectInline Type Alias
This refactoring helps you remove the selected type alias and replace all its usages with its definition.
To invoke this refactoring, select a declaration or usage of a type alias, and press Control+Shift+R or choose Inline type alias in the Refactor This popup.
from the main menu , and then selectIntroduce variable
This refactoring allows you to create a new local variable or constant based on a selected expression, initialize it with the expression, and finally replace all occurrences of the expression in the method with references to the newly introduced variable.
You can also invoke this refactoring with the dedicated shortcut Control+Alt+V.
In the example below, we use this refactoring to replace two occurrences of the same string with a variable:
Introduce variable for substring
This refactoring helps you quickly move a part of a string to a separate variable.
If TypeScript 1.4 or later is selected as a language level on the
page of ReSharper options, the refactoring will add a new template argument for the extracted substring. If the language level is TypeScript 1.3 or lower, then string concatenation is used:Before refactoring | After refactoring (TypeScript 1.4 or later) | After refactoring (TypeScript 1.3 or earlier) |
---|---|---|
var helloWorld = "Hello, World";
|
let world = "World";
var helloWorld = `Hello, ${world}`;
|
var world = "World";
var helloWorld = "Hello, " + world;
|
Inline variable
This refactoring allows you to replace all occurrences of a variable in the code with its initializer. Note that the refactoring should be only applied if the variable value stays unchanged after initialization.
You can also invoke this refactoring with the dedicated shortcut Control+Alt+N.
In the example below, we use this refactoring to inline the reversed
variable.
Move to another file
This refactoring helps you move the selected type from the current file to any existing file or to a new file. If you are moving to a new file, the new file is created automatically. If there are no more types in the current file, it can be removed. All necessary imports are moved with the type. All imports that are no longer necessary are removed from the original file.
Move to folder
This refactoring helps you move one or several types or files to another project or folder anywhere in your solution. If necessary, the refactoring will create the new target folder for you. All imports that the moved type(s) require are fixed in the new location. All imports that are no longer necessary are removed from the original file(s).
Move to resource
In TypeScript projects created from Visual Studio templates (for example, Apache Cordova), ReSharper allows moving string literals to resource files. ReSharper can optionally find all identical strings in the desired scope and replace them with the resource usage. To perform this refactoring, you need to have at least one resource file in your project (it normally has the .resjson extension).
You can also invoke this refactoring with the dedicated shortcut F6.
Move type to another module
This refactoring wraps a module declaration around the selected type.
Rename
One of the most time-consuming refactorings is supported for TypeScript. Modifying the name of a symbol can cause many problems if you try to do it manually. When you invoke the Rename refactoring (also available with the dedicated F2 shortcut), all checks are done by ReSharper. Either all modification are performed smoothly if no conflicts exist, or you get the list of conflicts that you can resolve manually to be sure that only necessary and appropriate changes are made.
