Extract Superclass refactoring
This refactoring allows you to extract certain members from a selected class into a new base class. The original class will extend the created base class.
If the current type already implements any interfaces, those interfaces can also be extracted into the new base class.
If you want to create an abstract class, mark at least one of the extracted method abstract.
In the example below, we extract an abstract class Shape
from the existing class Circle
:
Extract a base class
Select a class in one of the following ways:
In the editor, place the caret at the name of a class.
Select a class in the Solution Explorer.
Select a class in the File Structure window window.
Select a class in the Class View.
Select a class in the Object Browser.
Select a class in the type dependency diagram.
Do one of the following:
Press Control+Shift+R and then choose Extract Superclass.
Right-click and choose Refactor | Extract Superclass from the context menu.
Choose
from the main menu.
The Extract Superclass dialog will open.
Specify a name for the new base class and where it should be placed — in a new file or in the same file as the original type.
Select members that you want to transfer to the new base class. The list of members can contain:
Members of the current type
Interfaces that the selected type currently implements
To make/keep individual members abstract, tick the checkbox in the Make Abstract column next to them. If there is at least one member marked abstract, the base class will be created abstract too.
To quickly select all public members, click All Public.
If you select members that reference other members, there will be undefined symbols in the extracted class. Click Dependent to resolve the conflict by selecting all members that depend on the currently selected members.
To apply the refactoring, click Next.
If no conflicts are found, ReSharper performs the refactoring immediately. Otherwise, it prompts you to resolve conflicts.
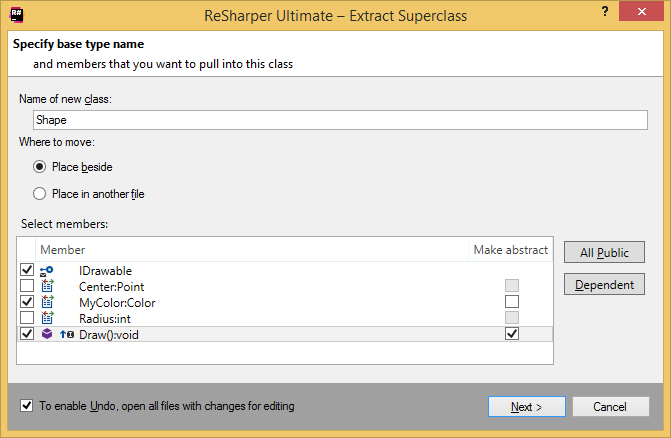
This feature is supported in the following languages and technologies:
The instructions and examples given here address the use of the feature in C#. For more information about other languages, refer to corresponding topics in the ReSharper by language section.