Language injections in C#
If a string literal contains some other formal language, such as regular expression, HTML, and so on, JetBrains Rider can provide code inspection, quick-fixes, code completion, context actions, and many other features specific to that language right inside this excerpt.
JetBrains Rider supports the following languages inside C# string literals:
JavaScript
HTML
CSS
JSON
XML
SQL
There are cases when language excerpts in another language file can be detected unambiguously, for example, SQL inside the SqlCommand()
constructor. In these cases, JetBrains Rider detects embedded languages automatically. If necessary, you can configure automatic language injections in specific cases on the page of JetBrains Rider settings Ctrl+Alt+S.
When a formal language inside a string literal cannot be detected automatically, JetBrains Rider allows you to manually mark the literal as containing specific language in one of the following ways:
Use context action
When your caret is within an embedded language block, you can press Alt+Enter and use the context action.
This action actually tells JetBrains Rider to mark the symbol range corresponding to the string, save this range in its internal database and keep track of it as the containing file changes. This way is very quick and straightforward, but there are two downsides: the range can be lost after an external file change, such as a VCS merge, and the injection marked this way will only be tracked locally:
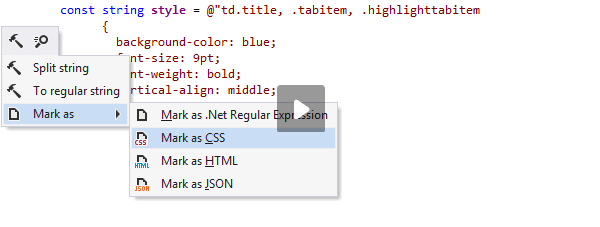
Use [StringSyntaxAttribute]
In projects targeting .NET 7 or later, you can use the [StringSyntaxAttribute] to mark method parameters, properties, and fields that contain strings. JetBrains Rider will enable its coding-assistance features inside the corresponding string literals according the attribute constructor.
For example, if you use StringSyntaxAttribute.Regex
, you can take advantage of the regular expressions assistance features in the corresponding literal:
![JetBrains Rider: Coding assistance for [StringSyntaxAttribute] JetBrains Rider: Coding assistance for [StringSyntaxAttribute]](https://resources.jetbrains.com/help/img/rider/2024.1/StringSyntaxAttribute_example.png)
Use [RegexPatternAttribute]
You can mark method parameters, properties, and fields accepting regular expressions with the [RegexPatternAttribute]
from JetBrains.Annotations. This is the recommended way for regular expressions.
Use [LanguageInjectionAttribute]
You can mark method parameters, properties, and fields that contain strings in another language with the [LanguageInjectionAttribute]
from JetBrains.Annotations.
[LanguageInjectionAttribute]
is recognized by all other JetBrains products that analyze C# code, for example, JetBrains ReSharper JetBrains Fleet and InspectCode Command-Line Tool.
![JetBrains Rider: Marking injected languages with [LanguageInjectionAttribute] JetBrains Rider: Marking injected languages with [LanguageInjectionAttribute]](https://resources.jetbrains.com/help/img/rider/2024.1/language_injection_attribute.png)
You can also use Prefix
and Suffix
parameters in the attribute for incomplete code blocks. For example, if a string only contains a list of CSS properties, you can use these parameters to process the string as a valid CSS statement:
Use comments
You can put a comment /*language=javascript|html|regexp|jsregexp|json|css|xml*/
before the string literal. This approach is similar to using the [LanguageInjectionAttribute]
but it works for variables and does not work for method parameters, and it also works outside C#. Although comments and attributes require some typing, and you can even think of them as contaminating your code, they make your intention clear to everyone who reads your code, they will not get lost, and anyone opening your code with JetBrains Rider will get the same features in the marked strings.
The format of injection comments is compatible with JetBrains ReSharper and IntelliJ Platform-based IDEs.
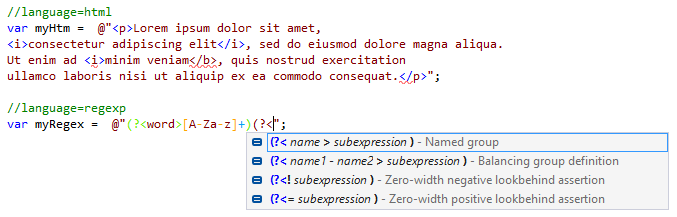
You can also use prefix=
and postfix=
parameters in the comments for incomplete code blocks. For example, if a string only contains a list of CSS properties, you can add the following comment before it: //language=css prefix=body{ postfix=}
. This will make JetBrains Rider resolve the string as valid CSS.