Remote Debugging with RubyMine
Introduction
What to do, if the interpreter you are going to use, is located on the other computer? Say, you are going to debug an application on Windows, but your favorite interpreter is located on Mac... With RubyMine, it's not a problem.
Before you start
Make sure that you have an SSH access to Mac computer!
Creating a project
On Windows, create a pure Ruby project, as described in the section Creating Empty Project.
In this tutorial, the project name is QuadraticEquation
.
Preparing an example
Add a Ruby file to this project (Alt+Insert - Ruby Class). Then, type the following code:
class QuadraticEquation
loop do
p "insert first quotient"
a = gets.to_f
p "insert second quotient"
b = gets.to_f
p "insert third quotient"
c = gets.to_f
discriminant = b**2 - 4 * a * c
square_root = Math.sqrt(discriminant)
x1 = ((-b + square_root) / (2 * a))
x2 = ((-b - square_root) / (2 * a))
if discriminant > 0
square_root = Math.sqrt(discriminant)
x1 = ((-b + square_root) / (2 * a))
x2 = ((-b - square_root) / (2 * a))
p "D = #{discriminant}, x1 = #{x1}, x2 = #{x2}"
elsif discriminant == 0
x = (-b / (2 * a))
p "D = #{discriminant}, x1 = x2 = #{x}"
else
p "D = #{discriminant}, no roots"
end
end
end
Creating a deployment configuration for a remote interpreter
Add a remote interpreter
Add new remote interpreter as described in Configuring Remote Interpreters via SSH.
Once you create the remote interpreter, the corresponding deployment configuration is created. To preview it, click Ctrl+Alt+S to open the Settings dialog window on the Windows machine, then click the Build, Execution, Deployment node and the Deployment node.
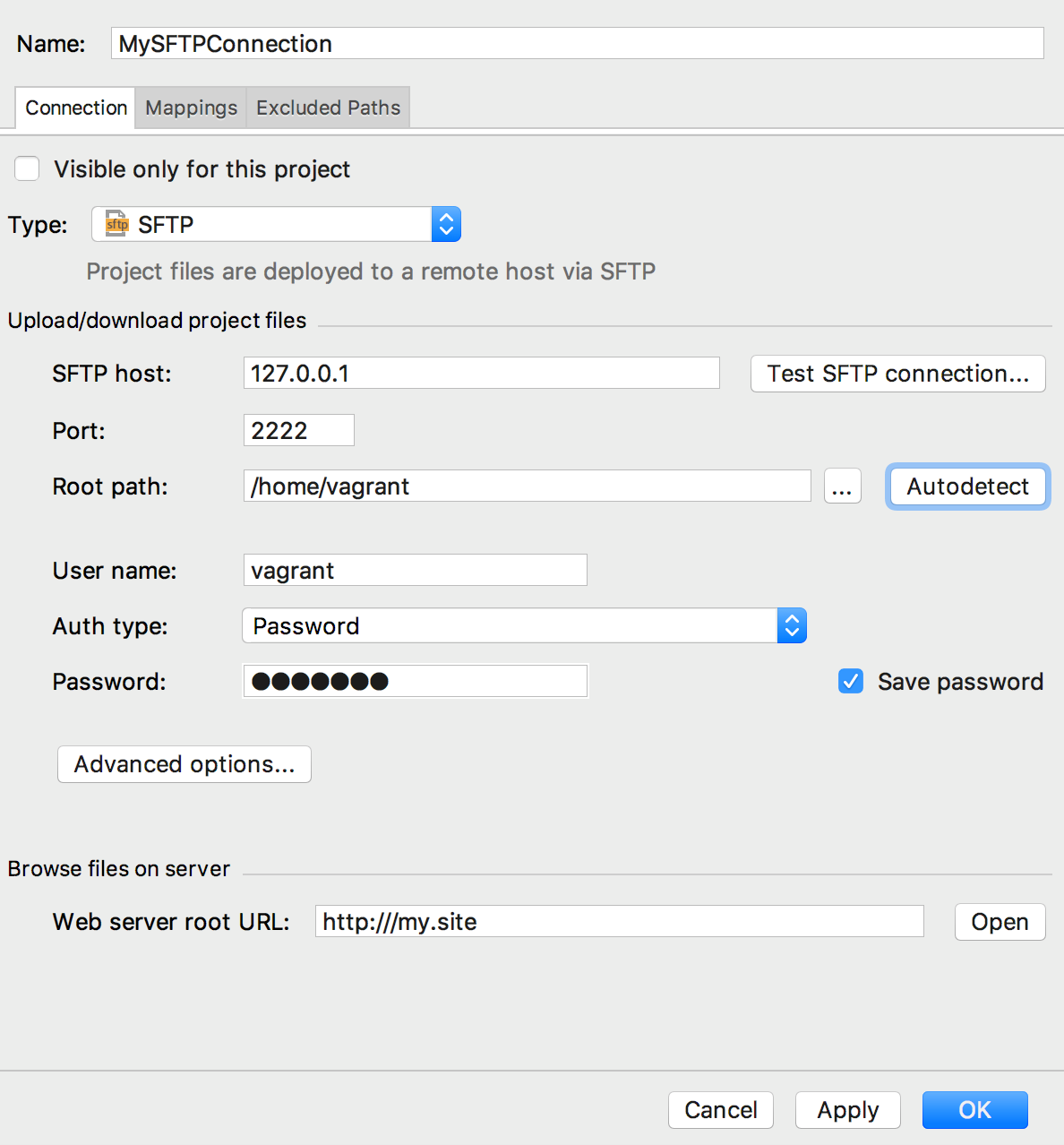
You can accept all default settings or alter them, if needed. For this example, let's use a meaningful name for your deployment configuration, for example, "MySFTPConnection". You can also click the Autodetect button near the Root path field to configure a root folder according to the user home folder on the target server.
Click the Ruby SDK and Gems node. On this page, click , and choose the remote interpreter:
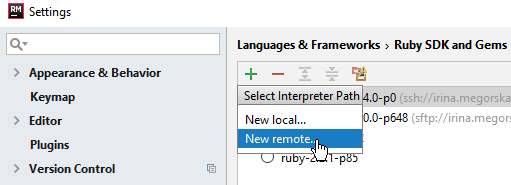
Next, in the Configure Remote Ruby Interpreter dialog box, click the SSH Credentials button. Enter the following:
- In the field Host, type the name of the Mac computer.
- In the field User name, type the user name of the Mac computer.
- As the authentication type is set to Password, choose this option and enter your Mac password.
- Type the Ruby interpreter path on Mac. Click the browse button (
) next to this field, to see the contents of the Mac computer in question:
Now your deployment configuration is ready.
Creating a remote debug configuration
On the main menu, choose and choose Ruby Remote Debug. In the same dialog box, enter the following:
- In the Name field, enter the configuration name. It's immaterial.
- In the Remote host and Remote port fields, enter the name of your Mac computer and its port.
- In the Remote root folder field, enter the path to the remote directory (on Mac).
- In the Local port field, specify the port number equal to the one specified as
dispatcher-port
. - In the Local root folder field, specify the path to the local directory (on Windows). Click the browse button (
) next to this field, to see the contents of your Windows computer.
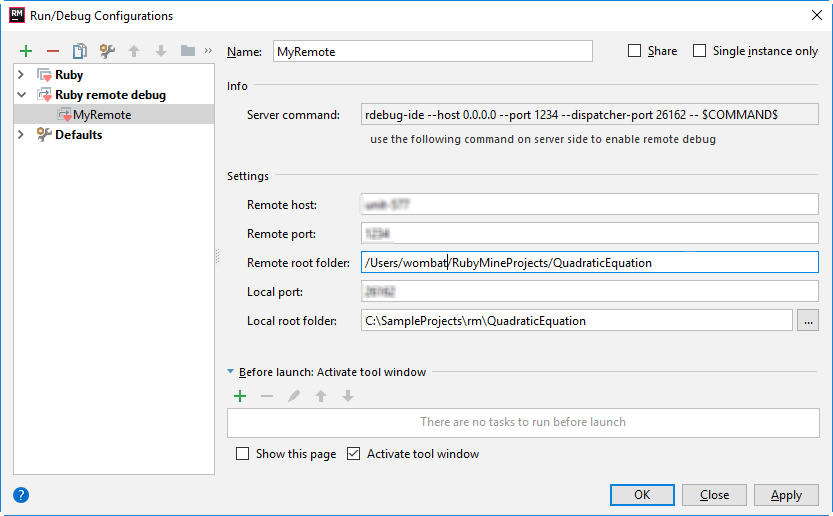
Apply changes and close the dialog.
Launching the remote debug
It's important to launch the same script on the remote computer (on Mac). Next, on Windows, press Shift+F9 or choose
on the main menu, and enjoy: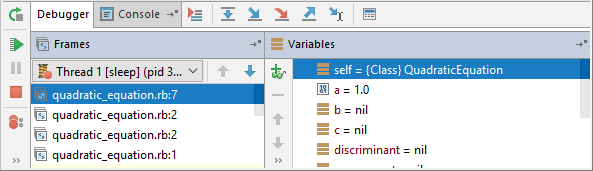