Trigger Job Run
Automation supports the following job triggers:
Trigger | Description |
---|---|
| Enabled by default. Run a job after a user pushes a ref (a branch, tag, commit, or any other Git reference) to the project repository. You can limit the scope of |
| Run a job after a particular branch is deleted from the repository. Works only in the project's default branch ( |
| Run a job after a code review or merge request is created in the project. |
| Run a job after a code review or merge request is closed in the project. |
| Run a job regularly at the specified time (UTC). Works only in the project's default branch ( |
Alternatively, you can run a job:
Manually on the Jobs page. Learn more
Using the API call
POST /api/http/projects/{project}/automation/jobs/{jobId}/start
.
Set job triggers
To set triggers, use the job's startOn
block:
If there's no
startOn
block in a job, the defaultgitPush
trigger is set.If there's a
startOn
block in a job, only specified triggers are set.If there's an empty
startOn
block, no triggers are set.
For example:
Disable job triggers in a particular repository
In some cases, you might need to disable automatic job triggers for a particular repository. For example, by default, if you mirror a repository in another project, 'git push' will run Automation jobs not only in the main repository but in its mirror as well.
Open the Jobs page and click Settings.
In Job Triggers, disable triggers for the required repository.
Git push trigger
Track refs, branches, tags, and file paths
gitPush
filters let you trigger jobs on specific conditions.
Filter | Description |
---|---|
| Run a job on pushing any branch, or branches matching specific patterns. Learn more |
| Run a job on pushing any tag, or tags matching specific patterns. Learn more |
| Run a job on pushing any Git ref (including branches and tags), or refs matching specific patterns. Learn more |
| Run a job on pushing changes in the specified directories or files. Learn more |
Default gitPush behavior
By default, if a gitPush
trigger or its filters are not set, the job will run on a push to any branch but not on tag or other ref pushes. So, the following scripts are equivalent:
job("Run on gitpush") {
container("ubuntu")
} | job("Run on gitpush") {
startOn {
gitPush()
}
container("ubuntu")
} | job("Run on gitpush") {
startOn {
gitPush {
anyBranch()
}
}
container("ubuntu")
} |
Filter by branch
By default, when a commit is pushed to the repository, Automation tries to run a job from the .space.kts
file in the same branch where the changes were committed. For example, if you push changes to the cool-feature
branch, Automation will try running a job from the .space.kts
file in this revision in the cool-feature
branch.
anyBranchMatching
filter lets you specify the list of branches where Automation should run the script. For example, the following job will run only on changes in the my-feature
branch:
If a change is pushed to the my-feature
branch, this job will start in the my-feature
but not in the main
or any other branch. If a change is pushed to the main
branch, none of the jobs will start – Automation will try to run the script in the main
branch, but it has the anyBranchMatching
filter that prevents this.
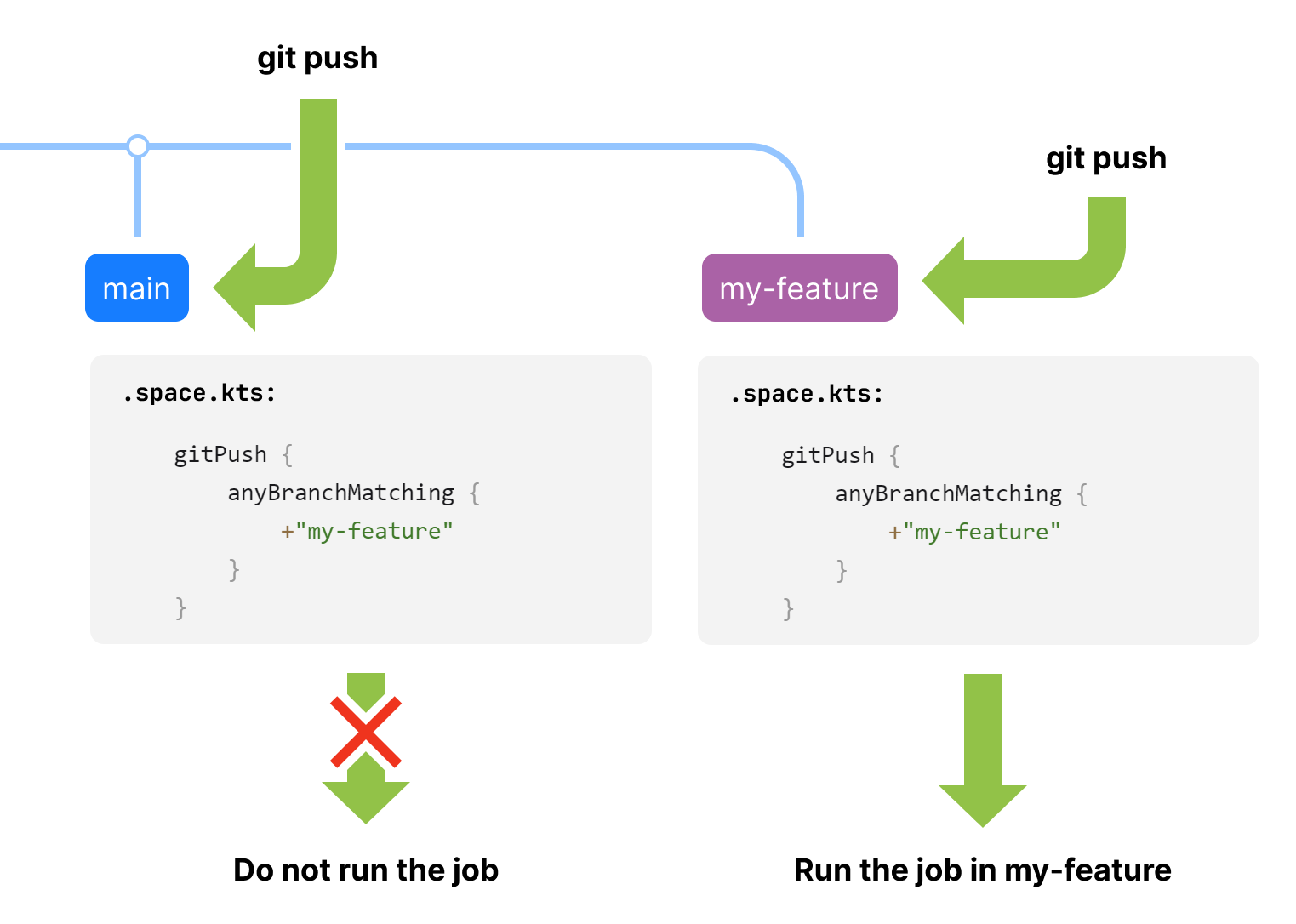
Branch filtering supports the following rules:
The filter supports include (
+
) and exclude (-
) rules.Exclude rules have priority over include rules.
You can use asterisk (
*
) wildcards, e.g.+"release-*"
.If only exclude rules are specified, it is assumed so that all non-excluded branches are included with
+"*"
.If you want to trigger a job on Git push to any branch, use
anyBranch
.For example:
job("Run on git push to specific branches") { startOn { gitPush { anyBranchMatching { // add 'main' +"main" // add all branches containing 'feature' +"*feature*" // exclude 'test-feature' -"test-feature" } } } // here go job steps... } job("Run on git push to any branch") { startOn { gitPush { anyBranch() } } // here go job steps... }
Filter by tag
anyTagMatching
lets you specify certain Git tags. The anyTagMatching
filter supports the same filtering rules as anyBranchMatching
. The anyTag
filter triggers a job on commits with any Git tag.
For example:
Filter by refs
Filter by refs is needed for fine-tuning when filtering by branch or tag is insufficient. anyRefMatching
lets you specify any Git refs, be it branches, tags, or references to particular commits. So, the following filters are basically the same:
gitPush {
anyBranchMatching {
+"main"
}
} | gitPush {
anyRefMatching {
+"refs/heads/main"
}
} |
The anyRefMatching
filter supports the same filtering rules as anyBranchMatching
or anyTagMatching
, but in addition:
The filter supports Kotlin regular expressions (
Regex
), e.g.+Regex("""refs/heads/release-\d+\.\d+""")
. Regex rules are applied to the full reference name, including therefs/.../
prefix.The filter supports all reference types including Space-specific like
refs/merge
. For example, with+"refs/merge/*/head"
you can run a job every time a merge request has updates.For example:
job("Run on git push on specific refs") { startOn { gitPush { anyRefMatching { +"refs/heads/release-*" +"refs/tags/v2.*" +Regex("""refs/heads/dev-v\d+\.\d+\.\d+""") } } } // here go job steps... } job("Run on git push on any ref") { startOn { gitPush { // all refs, including branches, tags, and merge requests anyRef() } } // here go job steps... }
Filter by path
pathFilter
lets you specify paths to certain directories and files. For example, the job below will run only if there is a change in the Main.kt
file:
You can use pathFilter
to fine-tune anyBranchMatching
, anyTagMatching
, or anyRefMatching
: Automation first checks if there's a change in a particular branch or if a particular tag is applied and only then it applies a pathFilter
. If other filters are not specified, pathFilter
works only within the current branch (the one with the committed changes).
pathFilter
supports the following filtering rules:
The job will run if at least one file matches the specified filter.
The filter supports the include (
+
) and exclude (-
) rules.If a single
+
path rule is specified, all other paths are excluded. Vice versa, if a single-
path rule is specified, all other paths are included.You should specify path relative to the working directory.
You can use asterisk (
*
) wildcards for matching the files only inside the current directory and double-asterisk (**
) for a recursive match. For example,src/tests/*
matches all files inside thetests
directory. Thesrc/tests/**
matches all files insidetests
and all its subdirectories.A more specific path has priority over less specific paths, e.g.
+"src/tests/MyTests.kt"
has priority over-"src/tests/**"
.For example:
job("Run on git push") { startOn { gitPush { // run only on changes in 'main' anyBranchMatching { +"refs/heads/main" } pathFilter { // include all from 'targets' dir +"targets/**" // exclude 'targets/main' dir -"targets/main/**" // include all 'Main.kt' files in the project // As this rule is more specific, // 'Main.kt' will be included even if // it's located in 'targets/main' +"**/Main.kt" // include all '.java' files from the 'common' dir +"common/**/*.java" } } } // here go job steps... }Automation will ignore the
pathFilter
and run the job in the following cases:the push contains more than 250 commits,
more than 10000 files were changed,
the push contains commits with more than 1000 changed files.
Combine gitPush filters
The gitPush
filters can be combined. The resulting filter follows the rule (anyBranch[Matching] OR anyTag[Matching] OR anyRef[Matching]) AND pathFilter
:
anyBranch[Matching]
,anyTag[Matching]
, andanyRef[Matching]
filters are combined with theOR
rule: a job will run in case any of the specified filters match.pathFilter
is combined with theAND
rule: a job will run if any of the specified branch/tag/ref filters match but only if the change was made in the specified paths.
For example:
Track changes in other project repositories
Sometimes, you might need to configure complex builds that use source code from several project repositories. For example, you can even create a separate repository that will contain all build scripts while other repositories will have no .space.kts
files at all.
The repository
property of the gitPush
trigger lets you specify the repository where a job
should track changes. For example, you have a project with two repositories: first-repository
and second-repository
. The .space.kts
script is located in first-repository
and you want Automation to trigger the script on changes in second-repository
:
But in which branch of first-repository
will the .space.kts
script run when a commit is pushed to some branch of second-repository
? In this case, Automation will try to match branches – Automation will search first-repository
for the branch that has the same name as the one where the commit was pushed. For example, the second-repository
gets a commit to the my-feature
branch. If first-repository
has the my-feature
branch with the .space.kts
script in it, this script will be started.
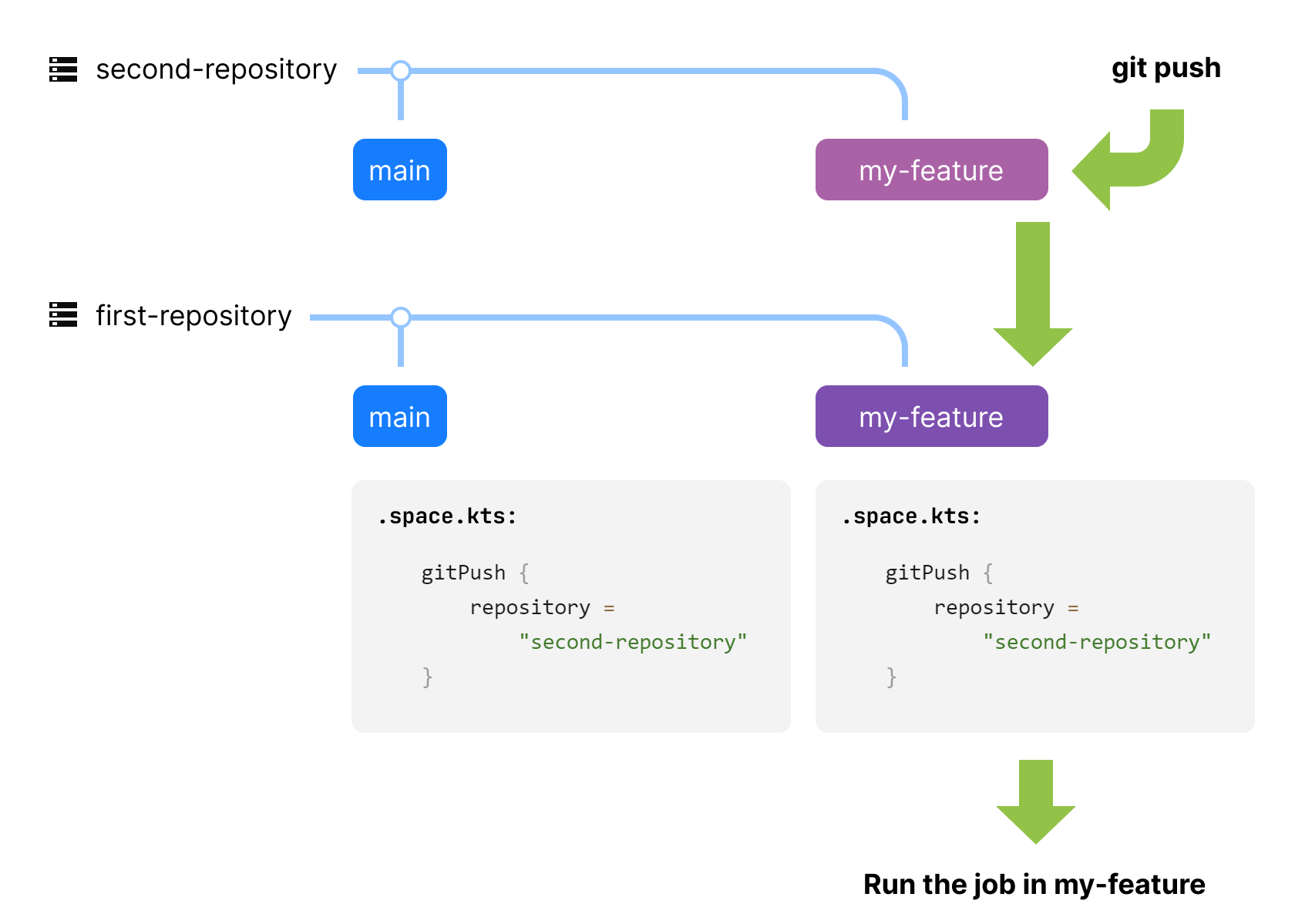
Similarly, if second-repository
gets a commit to the main
branch, the script from the first-repository/main
branch will be started.
If second-repository
gets a new branch that does not exist in the first-repository
, Automation will try running the script from the first-repository/main
branch. So, the main
branch works as some kind of a fall-back branch.
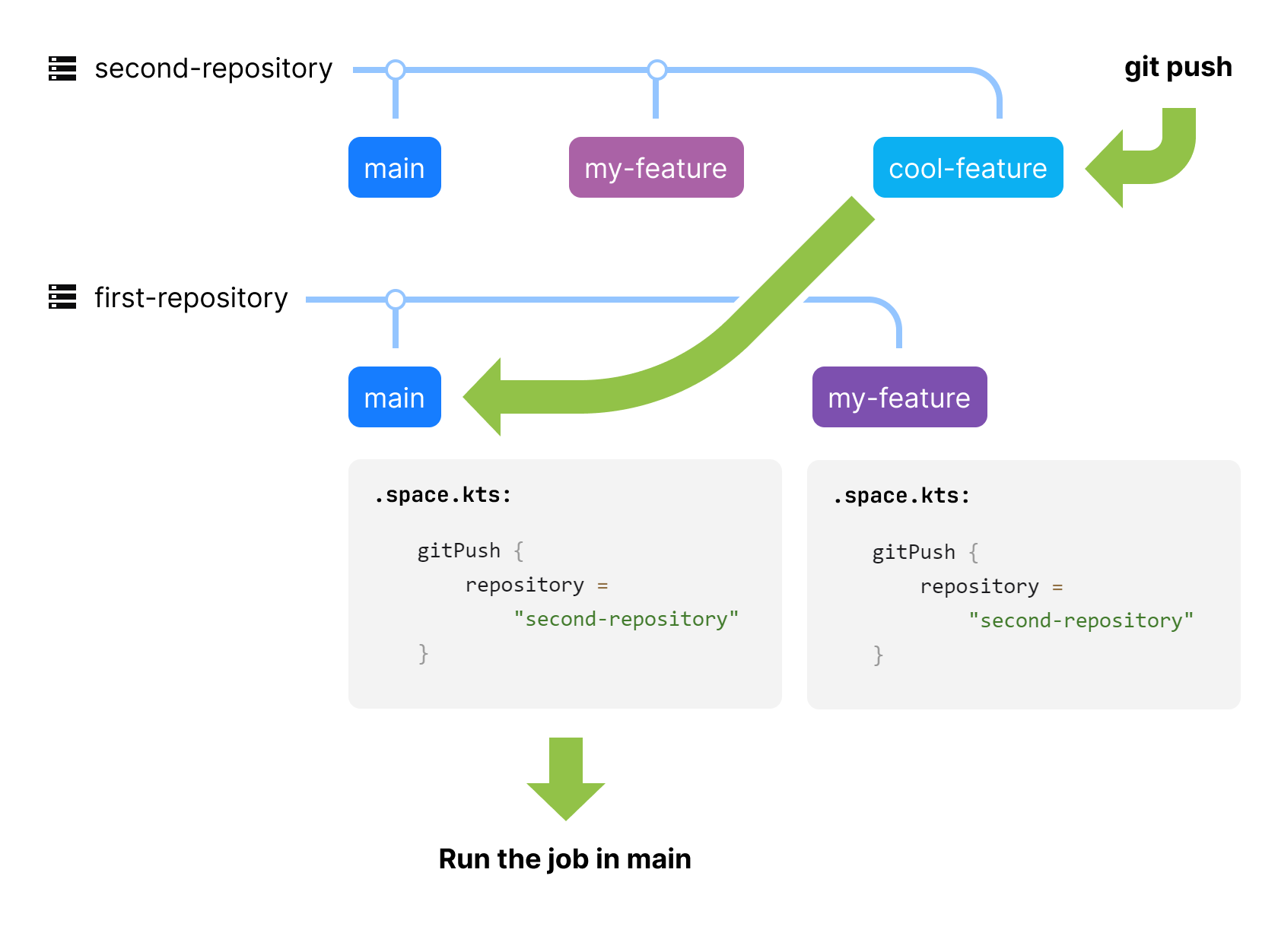
If you don't want the main
branch to work as a fall-back branch or want Automation to run scripts only in particular branches, you can add a anyBranchMatching
filter (or a pathFilter
for tracking changes by particular path). For example, to run jobs only on commits to the main
repository:
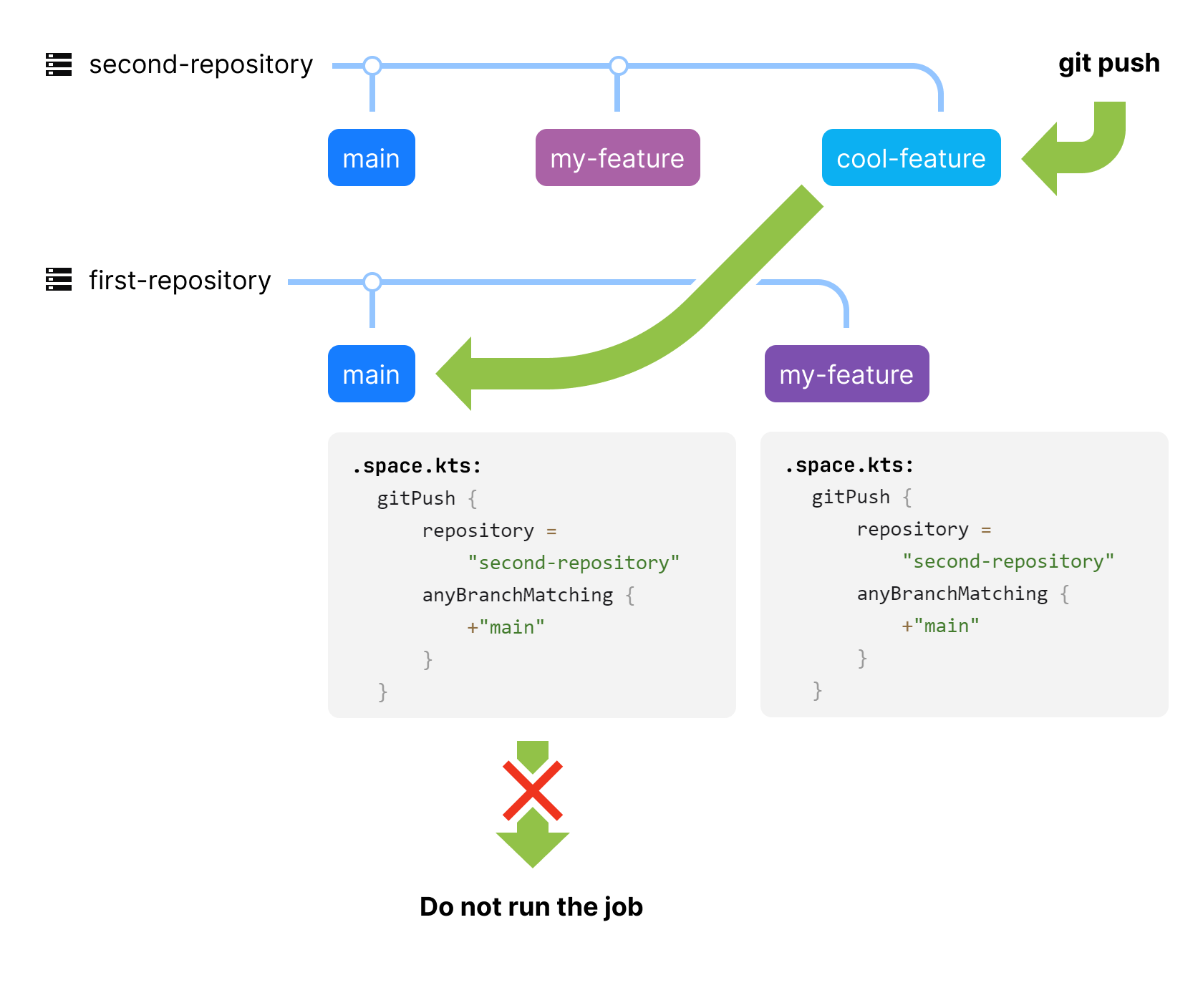
What if a commit is pushed to the second-repository/my-feature
branch but the first-repository/my-feature
branch doesn't have the .space.kts
script (or the script doesn't have the repository="second-repository"
trigger)? Then, Automation will not trigger any job runs as there is nothing to run.
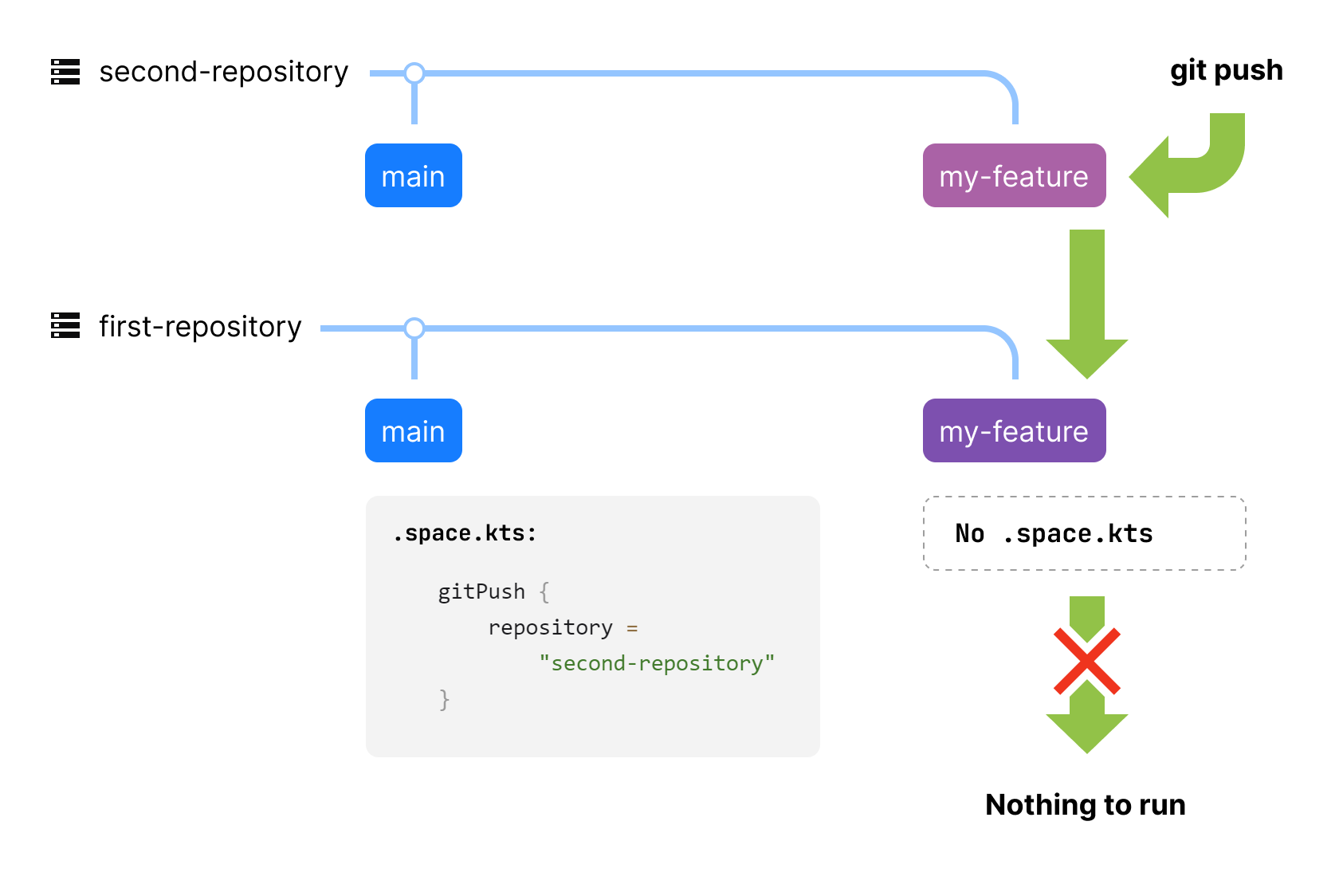
View multi-repository builds in the Jobs page
The jobs that reference other repositories are called cross-referenced jobs. If you open the Jobs page for the repository that contains cross-referenced jobs, you will see no difference between cross-referenced and "regular" jobs.

But if you switch the Jobs to the repository that was referenced by a job from other repository, you will see the list of Cross-referenced jobs.
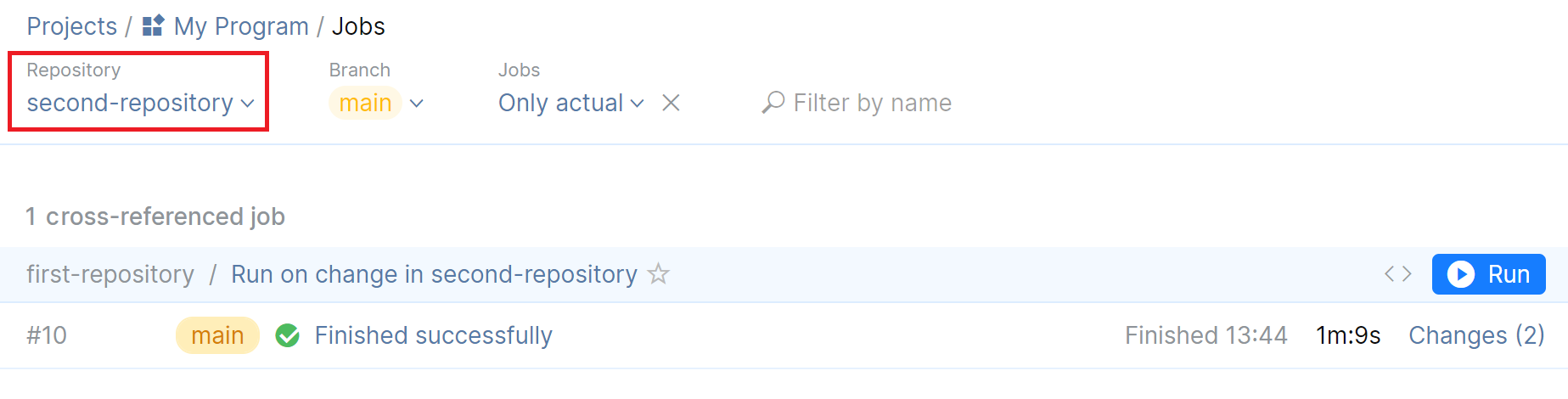
Skip job run
A job with the enabled gitPush
trigger won't run if the commit message contains any of the following strings (case-insensitive):
[ci skip]
[skip ci]
This way, you can skip job runs for specific commits, for example:
If you want to skip the job run for a git push with multiple commits, each commit message must contain the [ci skip]
or [skip ci]
string.
Code review and merge request triggers
codeReviewOpened
and codeReviewClosed
let you run a job when a code review or a merge request is opened or closed in the project. For example, this can be useful if you want to use the results of a job as a requirement for a merge request.
Using the branchToCheckout
parameter, you can specify which branch the job will check out. The branchToCheckout
parameter can take the following values:
branchToCheckout = CodeReviewBranch.MERGE_REQUEST_SOURCE
– check out the source branch if this code review is a merge request, or the default branch of the repository (typically,main
) if it's a code review for a set of commits.branchToCheckout = CodeReviewBranch.MERGE_REQUEST_TARGET
– check out the target branch if this code review is a merge request, or the default branch of the repository (typically,main
) if it's a code review for a set of commits.If
branchToCheckout
is not specified orbranchToCheckout = CodeReviewBranch.REPOSITORY_DEFAULT
, the job will check out the default repository branch (typically,main
).
For example:
If you want to run a job on any change in a merge request, use the gitPush
trigger: