Unity
This topic explains how to add and set up the Unity step to automate building Unity projects under all supported platforms.
Unity Support in Pipelines
Unity support in TeamCity Pipelines includes two key components:
Unity software installed on JetBrains-hosted cloud agents (except for Linux agents). You can communicate with this tool via command-line arguments sent from regular Script steps.
A Unity plugin installed on the Pipelines server. This plugin extends Pipelines with the following elements:
New Unity Step — facilitates communication with Unity installed on agents and allows you to build Unity projects using an intuitive UI solution instead of terminal commands sent from the generic Script step.
New Unity integration — a quick and reliable way to supply agent-hosted Unity with your licence data.
This plugin is installed upon a request and is not bundled with default Pipelines instances. Contact us if you want to enable the aforementioned UI elements on your server.
Unity License
JetBrains-hosted cloud agents have Unity installed on them. However, to use this tool you first need to activate it using your Unity license.
To do so, navigate to the Integrations section and add the Unity License integration.
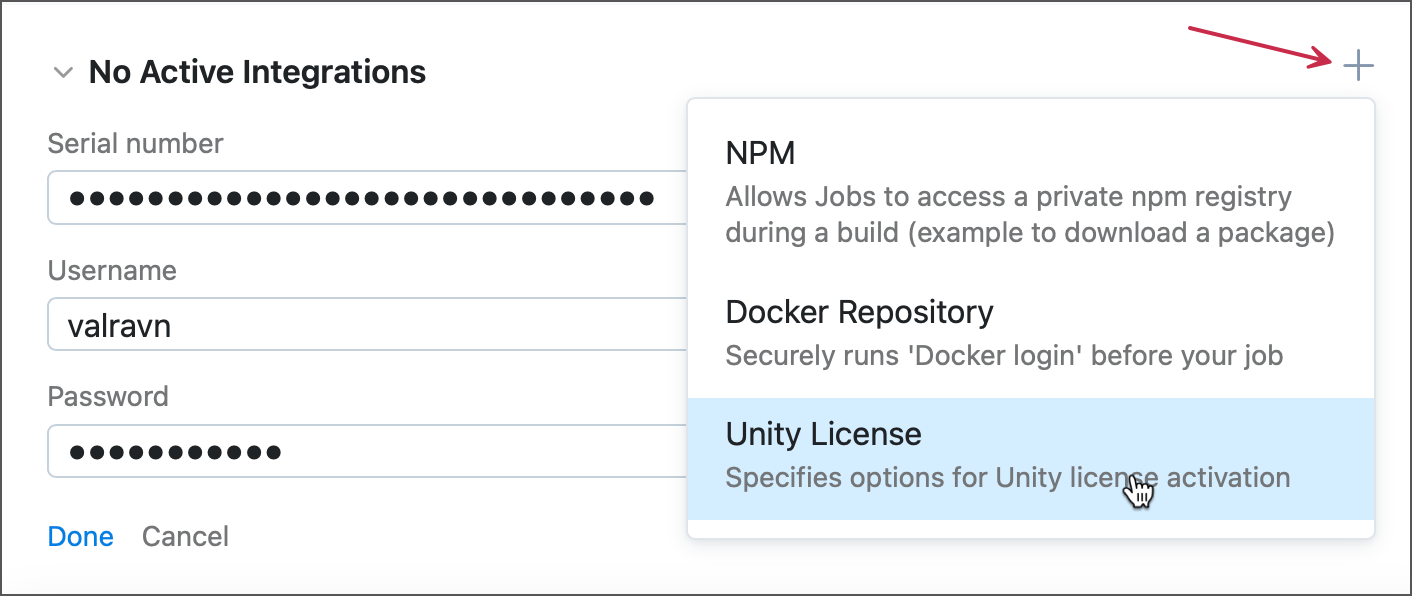
Unity Step Settings
The runner sends a command to launch Unity installed on an agent. By default, this command is supplied with a few basic arguments:
Depending on which runner options you set up, additional command-line arguments can be added to the Unity
command. For example:
Main Settings
To build a Unity project, you need to point the runner to the required project and (optionally) provide a build script.
To specify a path to your Unity project, use the following runner settings:
Working Directory — limits the checkout process to the specific repository subfolder. Use this option for large monorepositories with multiple projects, or leave it empty to use the root repository directory.
Project path — the path to the project to open in Unity. If left blank, TeamCity will look for the project in the working directory. This value is passed to the
-projectPath
argument of theUnity
command.
An optional build script is a public class that allows you to define a custom build process, run unit tests, prepare data, and so on. Typically, this class initializes a new instance of the BuildPlayerOptions class, specifies its properties, and passes it to the standard BuildPipeline.BuildPlayer method.
The following sample illustrates two methods that build a Unity game for different platforms.
Using this build script allows you to govern the building process without setting up some of the additional runner settings. For example, since the BuildPlayerOptions
class provides the target
property, you do not need to specify a value for the Build target setting in TeamCity UI.
To tell the TeamCity Unity runner to use your custom script, type the name of your static build method in the Execute method field (in either the NamespaceName.ClassName.MethodName
or ClassName.MethodName
format).
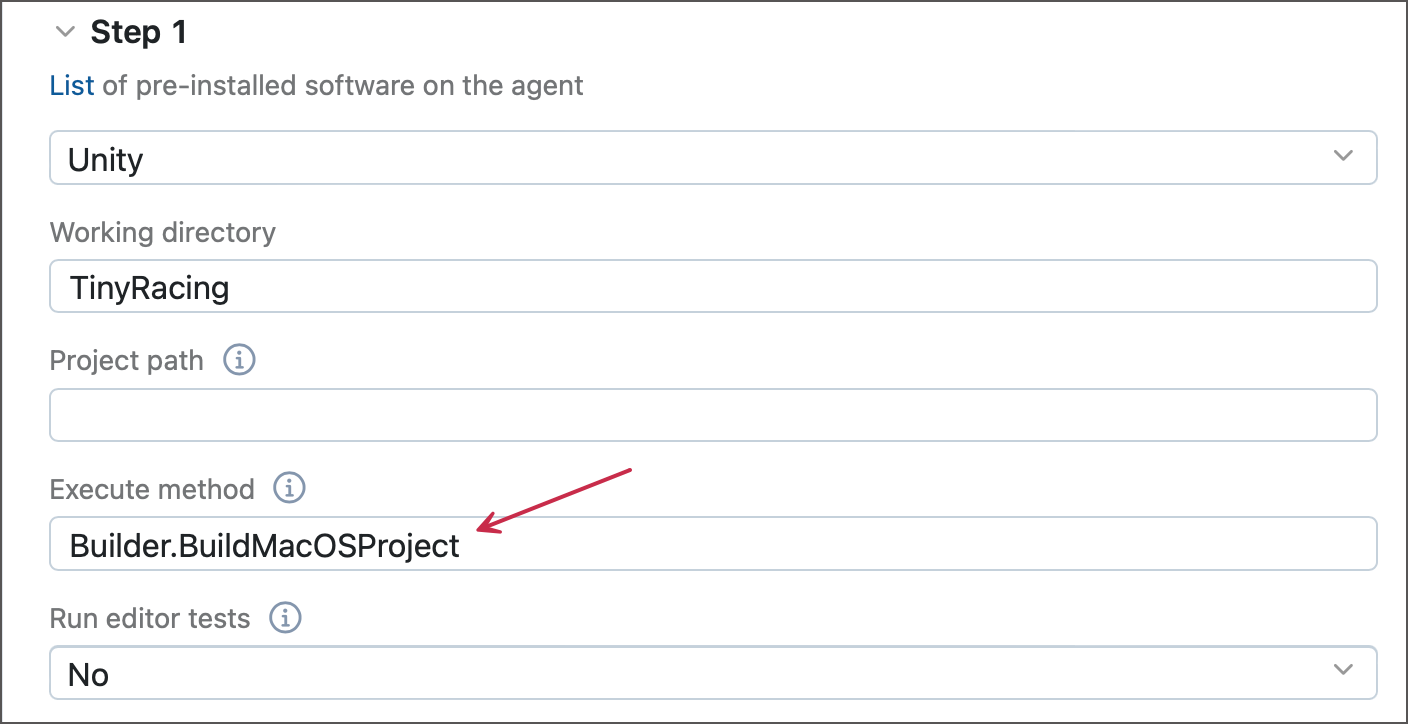
This value is passed to the -executeMethod
argument of the Unity
command.
Additional Settings
The following options allow you to set up additional settings for your building process.
Run editor tests — enable this option to access additional settings that allow you to run Unity test framework tests in both Edit and Play modes.
Build target — allows you to choose the required BuildTarget enumeration value and pass it to the
-buildTarget
argument of theUnity
command.Standalone player and Player output path — build arguments for the
Unity
command. For example, to add the-buildLinux64Player <pathname>
argument, choose "Linux 64-bit" in Standalone player and enter the required path to your build in the Player output path field.No graphics — toggle this setting on to add the
-nographics
argument. This argument prevents Unity from initializing the graphics device.No quit — toggle this setting on to remove the
-quit
argument. This argument terminates Unity once all other commands finish.Silent crashes — toggle this setting on to add the
-silent-crashes
argument. This argument prevents Unity from displaying the dialog that appears when a Standalone Player crashes.Command line arguments — allows you to add additional arguments that cannot be specified using the runner's UI (for example,
-noUpm
or-disable-gpu-skinning
).Detection mode — specifies whether TeamCity should automatically look for the Unity versions installed on the agent machine. If Auto is selected, use the Unity version to choose a pre-installed Unity. Otherwise, use the Custom path to Unity field.
Logging
Line statuses file — the path to an XML file with rules that allow you to override the default logging settings. The file contents should adhere to the following format:
<?xml version="1.0" encoding="UTF-8"?> <lines> <line level="warning" message="warning CS\d+" /> <line level="error" message="error CS\d+" /> </lines>In each particular rule, the
message
parameter is a regular expression. Log messages that match this regex are labeled as errors or warnings according to thelevel
parameter. You can then use the Logging verbosity setting to toggle required log messages on or off depending on their severity.Log file path — allows you to specify the location where Unity should write its editor or standalone log file. This value is passed to the
-logFile
argument of theUnity
command.Logging verbosity — allows you to choose which events should be written to the build log.