CMake profiles
Settings required for building a CMake project are incorporated into a CMake profile. It includes toolchain and build type, as well as CMake options such as generators and environment variables.
You can configure multiple profiles for your project in order to, for example, use different compilers or to build targets with differing settings.
Access profile settings
Do one of the following to open the CMake profile settings.
Go to
.Press Ctrl+Shift+A to open the Find Action dialog and search for CMake settings:
Go to assign a shortcut for CMake settings. Use this shortcut to quickly open the CMake settings page.
and
Add a new profile
Go to
.Click
, and CLion will add a new profile to the list.
If required, change the profile name, build type, and other settings.
Build with a profile
The enabled profiles are listed in the profile switcher.
Select the desired profile before building, running, or debugging your application.
Click
or call one of the available Build actions.
Also, build is performed by default before run
or debug
(you can change this in the configuration settings).
Configure default profiles for new projects
Go to
.Configure the list of profiles to be used for all new projects by default.
Share profiles
You can share CMake profiles in VCS along with the project. The profiles' settings are stored in cmake.xml in the .idea directory.
Select the profile you want to share and set the Share checkbox:
Shared profiles are automatically moved to the bottom of the list.
Make sure to have different names for shared and local profiles. If a shared and a local profile have the same name, the local one takes precedence and you will not see the shared one in the settings.
Disable/enable profiles
You can disable the profiles that are not currently in use to save time on loading and avoid potential errors (for example, when you have a remote profile you don't use regularly and the machine is shut down).
Use one of the following options:
Clear or set the Enable profile checkbox in . Disabled profiles are grayed out in the list.
To disable a successfully loaded profile, select Disable This Profile from the configuration menu in the CMake tool window:
From this menu, you can also enable any of the disabled profiles:
CMake options
Compiler flags
In CLion, you can specify compiler flags in the CMake options field of a profile or in the CMakeLists.txt script.
Using CMake options:
Select the profile in CMake options field.
and edit theUse
-D
with theCMAKE_CXX_FLAGS
variable (orCMAKE_C_FLAGS
for C projects). For example,-DCMAKE_CXX_FLAGS="-Wall -Wextra"
.CLion also works with the
--preset
argument passed to CMake options. The data from the specified preset is loaded into build type, toolchain, and build directory settings.Alternatively, you can set compiler flags in CMakeLists.txt.
Add the following command with the required flags:
set(CMAKE_CXX_FLAGS "${CMAKE_CXX_FLAGS} -Wall -Wextra")
CMake toolchain files
In CMake options, you can specify a CMake toolchain file using the CMAKE_TOOLCHAIN_FILE variable.
CMake cache variables
You can view and edit the CMake cache variables in the Cache variables table:
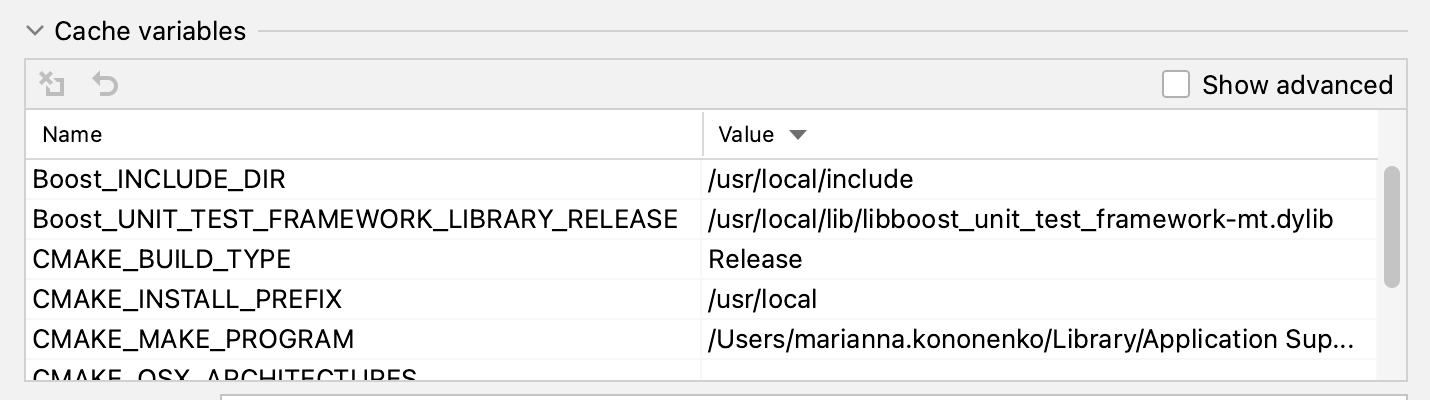
You can add new variables either in the CMake options field or in the CMakeLists.txt file. User-defined variables are placed on the top of the table. Values of the user-defined variables are highlighted in bold, while the changed values of the other variables are shown in italics:
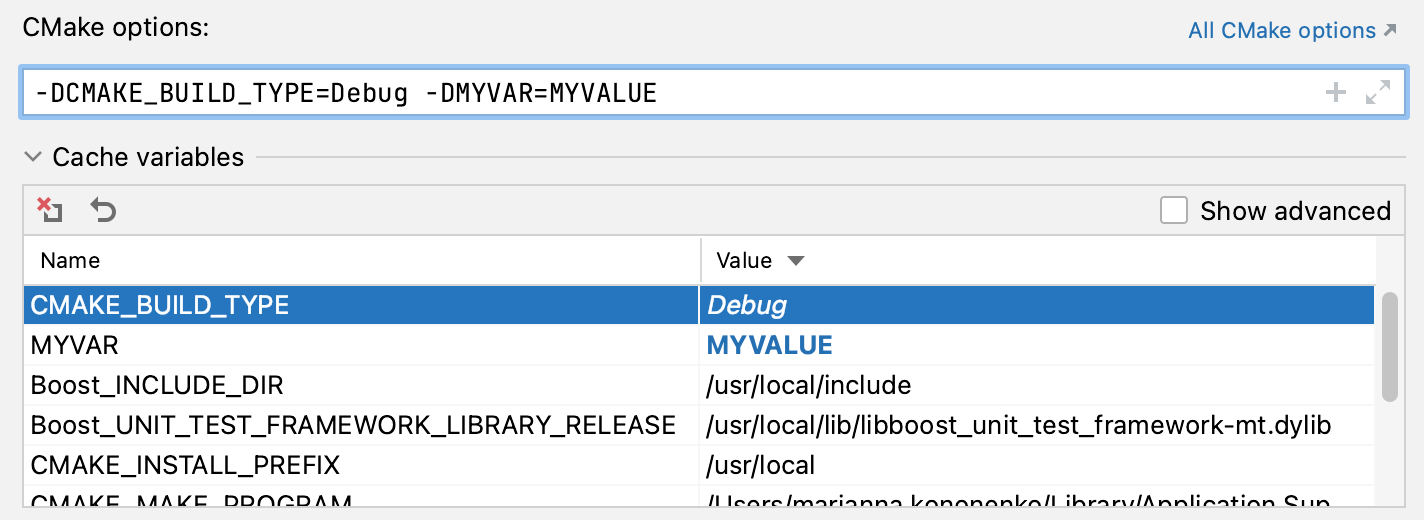
To remove a variable from the CMake options field, select it in the table and click or press Alt+Delete. The variable will be removed from the table or its value will be set to default after resetting the CMake cache.
To discard changes made in the current session, select a variable and click or press Ctrl+Z.
Advanced CMake variables are hidden by default, but you can show them by selecting the Show advanced checkbox:
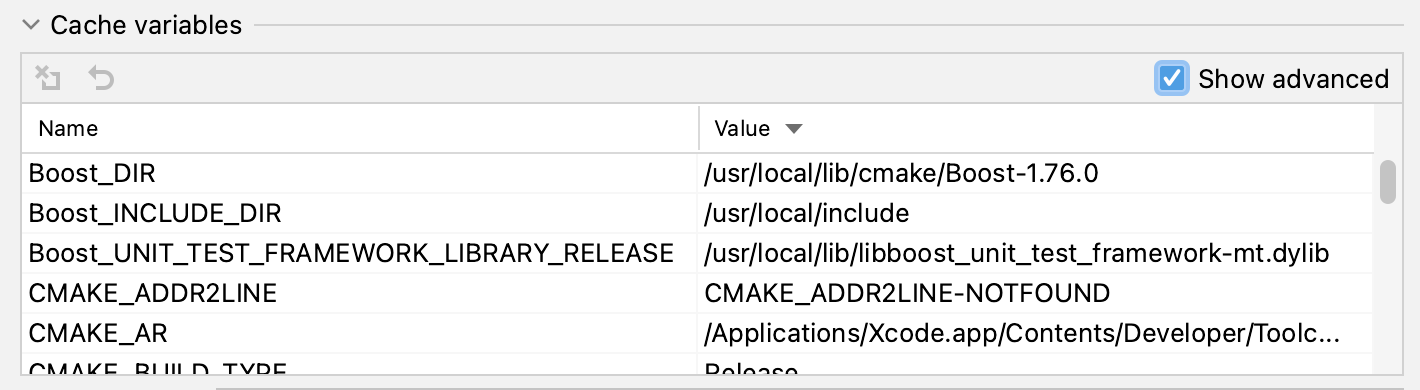
CLion also shows the short description in tooltips for CMake cache variables:
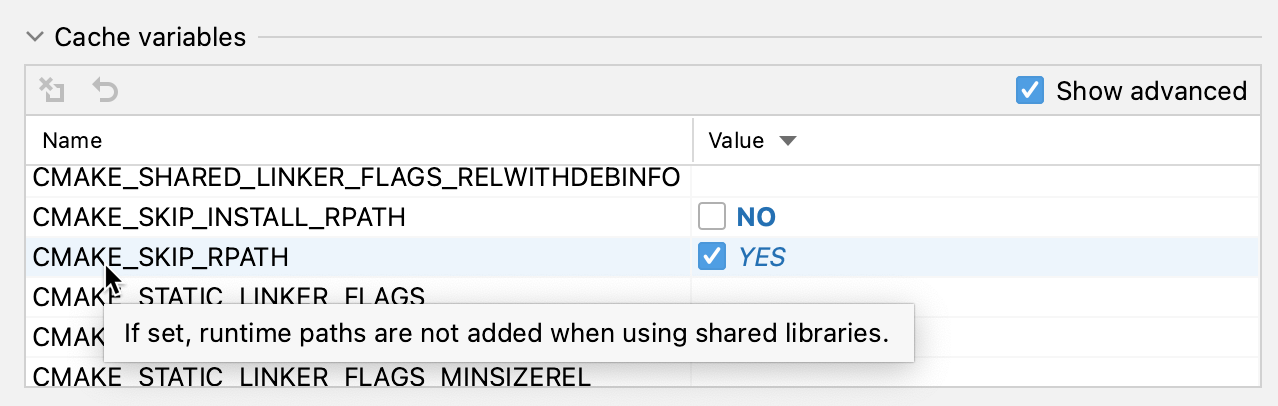
The IDE shows Boolean values as checkboxes. Next to the checkboxes, you can see the actiual values behind the Boolean variables. The following values are treated as False
: 0
, OFF
, NO
, N
, IGNORE
, NOTFOUND
, empty strings, and values that end in the suffix -NOTFOUND
. In all other cases, the value is treated as True
.
When you check or uncheck the checkbox in the CLion user interface, the following pairs of values are used to configure option values: 0-1
, OFF-ON
, NO-YES
, FALSE-TRUE
, and N-Y
. For any other values, the IDE converts the checkbox state to OFF-ON
.
Generators
In the Generator field, you can switch between CMake generators. Use the default value for the selected toolchain or set another generator from the predefined list:
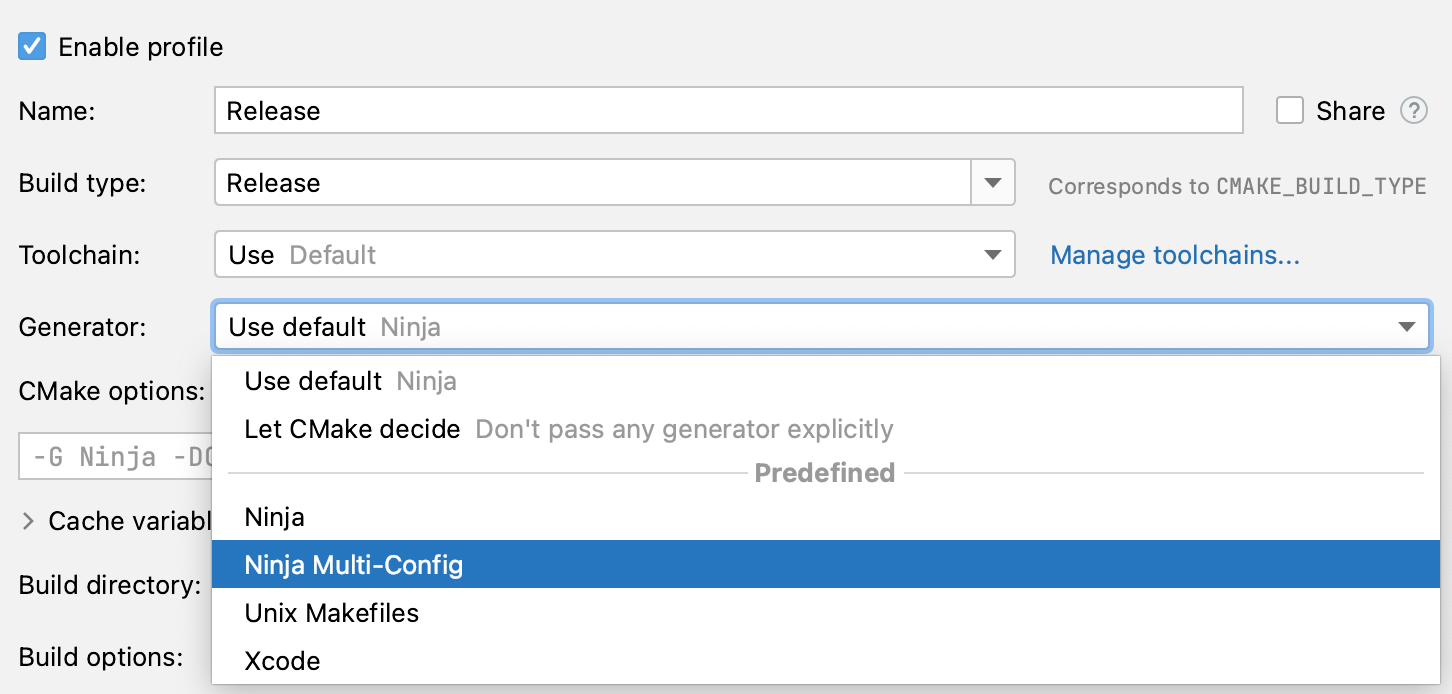
Use default | CLion uses either Ninja or Makefiles as the default generator. Ninja is used when all of the following is true:
In all other cases, the Makefiles generator is used. |
Let CMake decide | If you choose this option, CLion will not force any generator explicitly, and CMake will decide which generator to use. By default, this is controlled by the CMAKE_GENERATOR environment variable. |
Alternatively, you can set the generator in CMake options via -G
. When the Generator field is used, CLion automatically updates CMake options and vice versa:

Current limitations
CLion uses CMake File API, which first appeared in CMake v3.14. However, CLion supports it starting from the update introduced in CMake v3.15, so if you decide to switch from the bundled CMake, make sure to use version 3.15.x or later.
For multi-config generators like Ninja Multi-Config, Xcode, or Visual Studio, CLion uses only the configuration that corresponds to the build type specified in the CMake profile (CPP-20890).
Build types
Use the Build type field to set one of the following CMake build types:
Default (corresponds to the empty value of CMAKE_BUILD_TYPE).
Debug (the default build type)
Release
RelWithDebInfo (Release with debugging information)
MinSizeRel (Release optimized for size)
To refer to the build type in CMakeLists.txt, use the CMAKE_BUILD_TYPE
variable. For example:
You can also create conditional statements in your code based on the current build type:
Custom build types
The list of the available build types is defined in the CMAKE_CONFIGURATION_TYPES
command. The default value of this command is the four build types given above, but you can extend it to have other build types. For example:
After reloading the project, custom types will be available from the CMake settings:
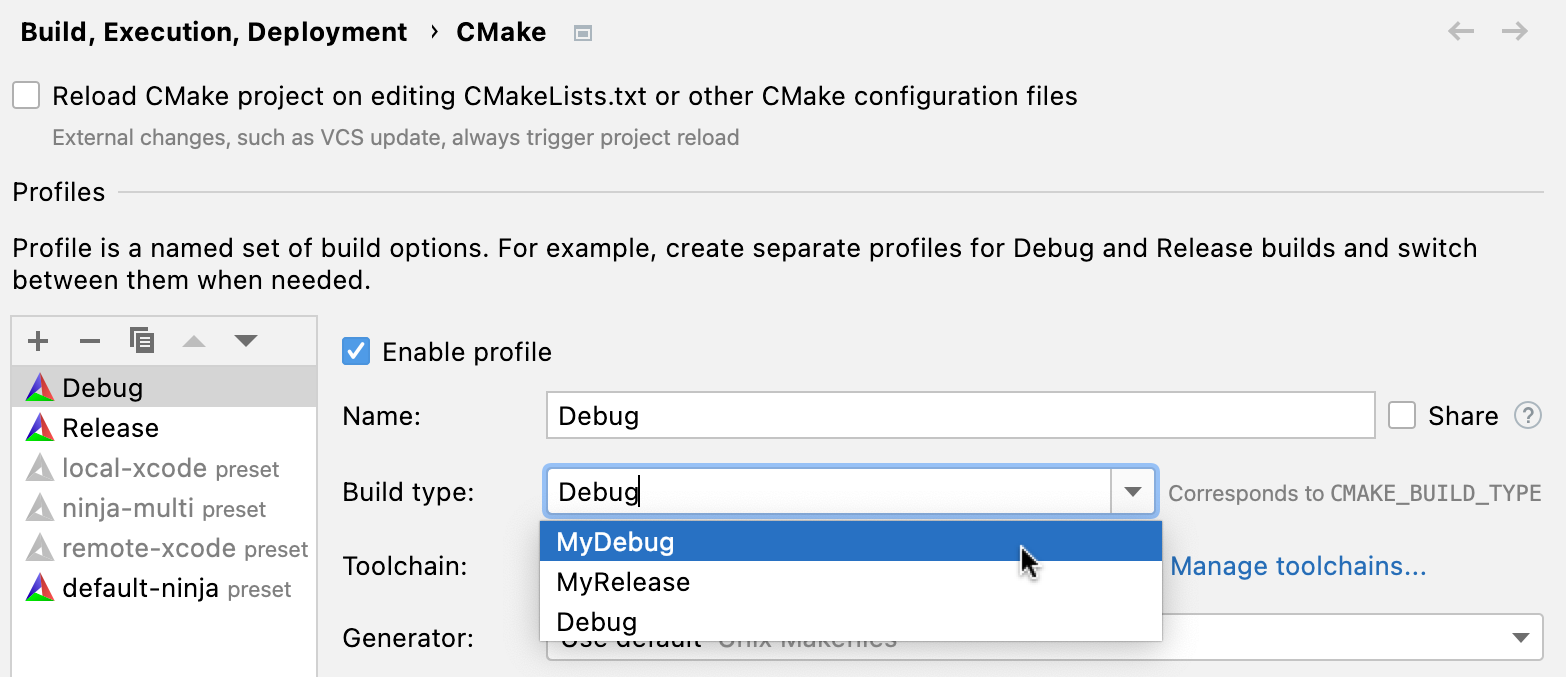
Note that the custom types were added to the value Debug which was cached in CMakeCache.txt. So for example, if you add a new CMake profile, it will have its own CMakeCache.txt, and for this profile, the list of the available build types will contain your custom types only:
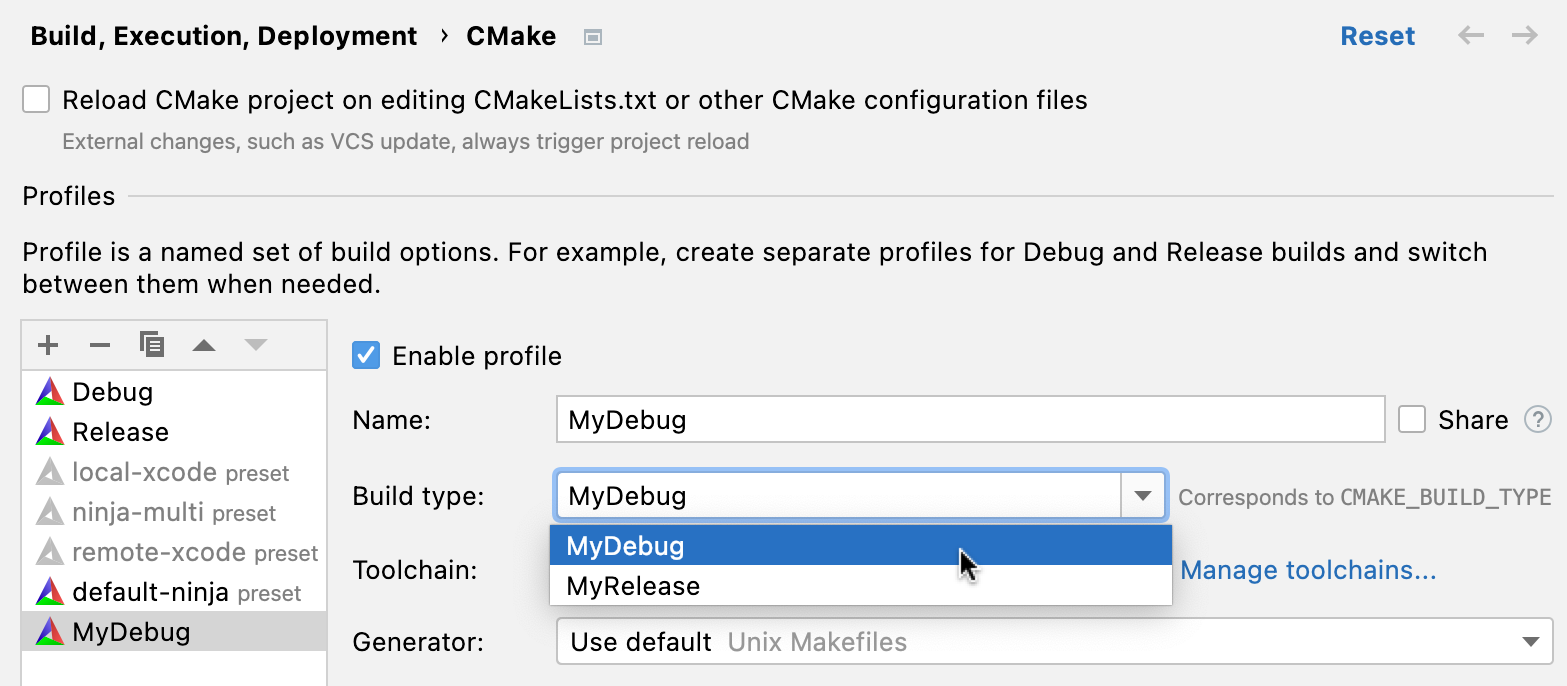
Build options
In the Build options field, you can set the options to be passed either to the build tool used by CMake or as command line parameters to CMake itself. These options will be used during the build phase.
Arguments for the underlying build tool (make, Ninja, or another one) should be preceded with --
. For example, if you specify -j 5 --clean-first -- -d -p
, then -j 5 --clean-first
will be processed by CMake, while -d -p
will be passed to the build tool.
When nothing is specified in this field, CLion uses default settings which depend on the selected environment. For example, the default setting for build processes running in parallel is -- -j max(cpucount * 0.8, cpucount - 2)
for make and Ninja, while for Microsoft Visual C++ this option is not set and the field is empty.
Environment variables
You can pass additional environment variables to CMake generation and build via the Environment field of the dialog (navigate to ).
The overall impacting environment for CMake generation and build consists of:
Parent environment
To include parent environment, open the Environment Variables dialog by clicking
or pressing Shift+Enter, and set the Include system environment variables checkbox. The values you specify additionally will be appended to system variables. Otherwise, when the checkbox is cleared, your custom values will overwrite the system ones.
Toolchain environment
These are the variables defined by the selected toolset (for example, vcvarsall.bat for MSVC or path variables like
mingw/bin
), or the variables from an environment initialization script.CMake profile environment
Your custom variables specified in the Environment field.
You can reference existing variables, including the parent environment variables, using the $VAR$
syntax. Note that such references are case-sensitive: for example, PATH=xxx:$PATH$
for Linux/macOS and Path=xxx;$Path$
for Windows. Referencing existing variables is currently not available for remote toolchains (CPP-15693).