Quick start with Go
This quick start guide will introduce you to the key concepts and help you with your first steps in IntelliJ IDEA.
Step 1. Open or create a Go project in IntelliJ IDEA
Create a Go file
A new project has no Go files. To create a Go file, perform one of the following actions:
Right-click the parent folder of a project, and select
.Click the parent folder of a project, press Alt+Insert, and select Go File.
Click the parent folder of a project, navigate to
.
In the New Go File dialog, type a name of the file and select whether you want to create an empty Go file (Empty file) or a Go file with the defined
main
function (Simple application).
Step 2. Explore the user interface
When you launch IntelliJ IDEA for the very first time, you see the Welcome to IntelliJ IDEA dialog. From the Welcome to IntelliJ IDEA dialog, you can create and open projects, check out a project from a version control system, view documentation, and configure the IDE.
When a project is opened, you see the main window divided into several logical areas.
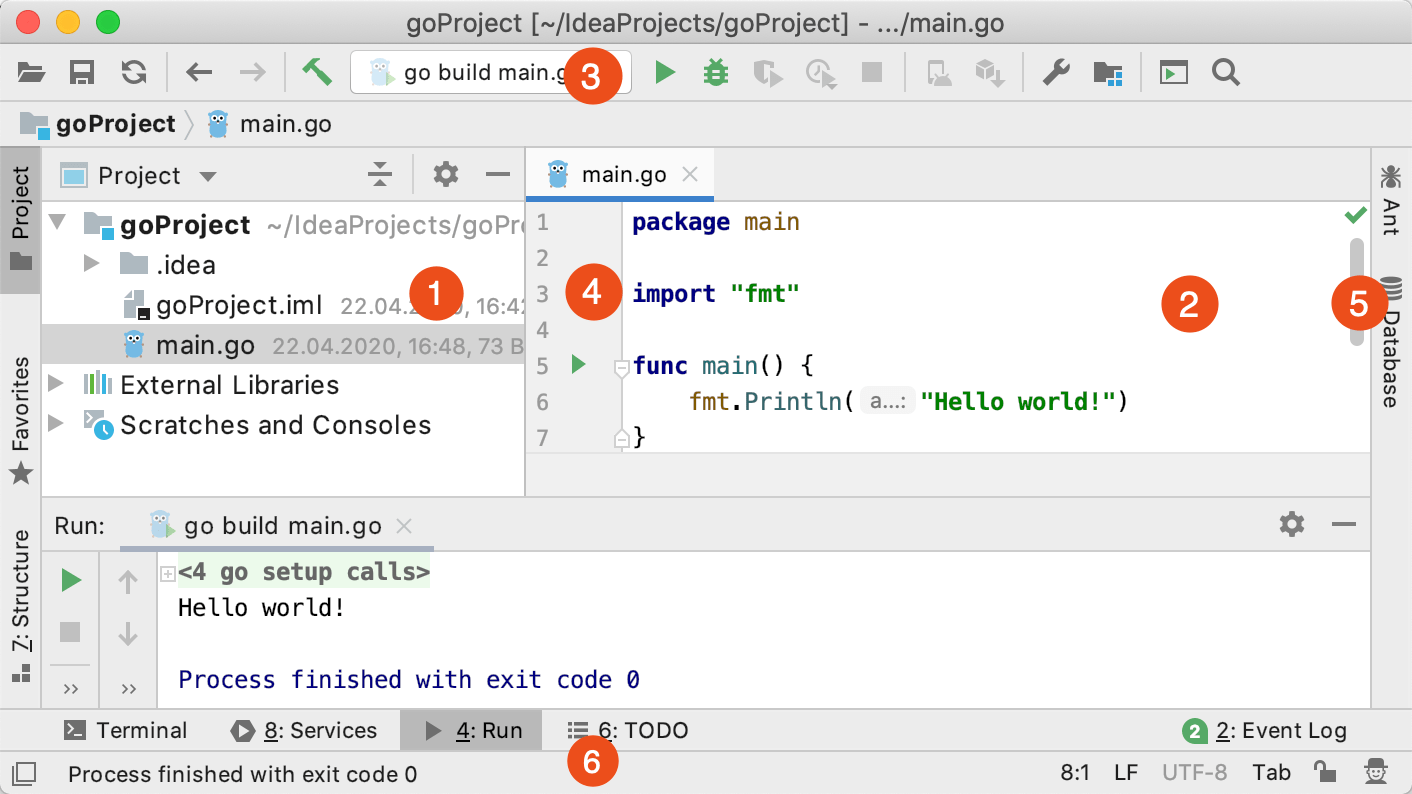
The Project tool window, which is on the left side, displays your project files. Tool windows are windows that provide access to project management, search, running and debugging, integration with version control systems, and other tasks. To see a list of all tool windows, navigate to .
The Editor pane is on the right side, where you write your code. It has tabs for easy navigation between open files.
The navigation bar is above the editor additionally allows you to quickly run and debug your application as well as do basic VCS actions.
The Gutter is the vertical stripe next to the editor. It shows you the breakpoints and provides a convenient way to navigate to a definition or a declaration. Also, you can use the Run Application icon (
) to run or to debug your application.
The Scrollbar, on the right side of the editor. IntelliJ IDEA monitors the quality of your code and shows the results of code inspections: errors, warnings, typos, and other issues. The indicator at the top of the gutter shows the overall status of code inspections for the entire file.
The status bar indicates the status of your project and the entire IDE and shows you information about a file encoding, line separators, inspection profiles, and other warnings and information messages.
In the lower left part of the IDE, in the Status bar, you can see or
buttons. These buttons toggle the display of the tool window popup. If you hover over this button, the list of the currently available tool windows shows up.
Step 3. Write your code
As you work in the editor, IntelliJ IDEA analyzes your code, searches for ways to optimize it, and detects potential and actual problems. The following list includes basic features and tools that might be useful for your code writing and increase your productivity:
Refactorings
Refactoring is a process of improving your source code without creating a new functionality. Refactoring helps you keep your code solid , dry, and easy to maintain.
IntelliJ IDEA suggests you the following refactoring options:
Change signature changes the method or function name; adds, removes, and reorders parameters; assigns default values to new non-variadic parameters.
Extract refactorings extracts code fragments that can be grouped. You can extract a constant, a variable, a method, and an interface.
Inline refactoring moves an extracted code fragment to the calling code. An opposite to the Extract refactoring.
Copy refactoring copies a file, a directory, or a package to a different directory or a package.
Move refactoring moves source code sections to another package or a file. For example, you can use the Move refactoring to move methods to another package.
Code completion
IntelliJ IDEA has two types of code completion:
Basic code completion Ctrl+Space helps you complete names of types, interfaces, methods, and keywords within the visibility scope. When you invoke code completion, IntelliJ IDEA analyzes the context and suggests the choices that are reachable from the current caret position. By default, IntelliJ IDEA displays the code completion popup automatically as you type.
Smart code completion Ctrl+Shift+Space filters the suggestion list and shows only the types applicable to the current context.
The following animation shows the difference between basic and smart type-matching completion. Notice the number of variants that are suggested for different completion types
Generating code
IntelliJ IDEA provides multiple ways to generate common code constructs and recurring elements, which helps you increase productivity. These can be either file templates used when creating a new file, custom or predefined live templates that are applied differently based on the context, various wrappers, or automatic pairing of characters.
Additionally, IntelliJ IDEA provides code completion and Emmet support.
You can generate getters, setters, constructors, missing methods, and test files.
Live templates
Use live templates to insert common constructs into your code, such as loops, conditions, declarations, or print statements.
To expand a code snippet, type the corresponding template abbreviation and press Tab. Keep pressing Tab to jump from one variable in the template to the next one. Press Shift+Tab to move to the previous variable.
To see the list of live templates, open settings Ctrl+Alt+S and navigate to
.Consider the following example of the
Hello World
program that is coded with Live Templates.
Inspections
In IntelliJ IDEA, there is a set of code inspections that detect and correct abnormal code in your project before you compile it. The IDE can find and highlight various problems, locate dead code, find probable bugs, spelling problems, and improve the overall code structure.
Inspections can scan your code in all project files or only in specific scopes (for example, only in production code or in modified files).
Every inspection has a severity level — the extent to which a problem can affect your code. Severities are highlighted differently in the editor so that you can quickly distinguish between critical problems and less important things. IntelliJ IDEA comes with a set of predefined severity levels and enables you to create your own.
To see the list of inspections, open settings Ctrl+Alt+S and navigate to
. Disable some of them, or enable others, plus adjust the severity of each inspection. You decide whether it should be considered an error or just a warning.For example, the Unreachable code inspection detects parts of code that cannot be executed.
Intention actions
As you work in the editor, IntelliJ IDEA analyzes your code, searches for ways to optimize it, and detects potential and actual problems.
As soon as the IDE finds a way to alter your code, it displays a yellow bulb icon in the editor next to the current line. By clicking this icon, you can view the intention actions available in the current context. Intention actions cover a wide range of situations from warnings to optimization suggestions. You can view the full list of intentions and customize them in the Settings dialog Ctrl+Alt+S.
To see the list of intention actions, open settings Ctrl+Alt+S and navigate to
.To apply an intention action, click the bulb icon (or press Alt+Enter) to open the list of suggestions.
Select an action from the list and press Enter.
For example, you can use an intention action to inject another language in your code:
Step 4. Run and debug your code
You can run and debug your code in IntelliJ IDEA from a keyboard, from the context menu, from the gutter menu, or by using the Run/Debug configuration.
Run your code
To run your application Shift+F10, click the Run Application icon
in the gutter and select Run <application_name>.
Debug your code
Debugging starts with placing breakpoints at which program execution will be suspended so that you can explore program data. Just click the line in the gutter where you want to place a breakpoint.
To debug your application Shift+F9, click the Run Application icon in the gutter
and select Debug <application_name>. Then go through the program execution step by step (see the available options in the menu or the Debug tool window).
For more information, refer to Debugging.