Code Style. C++
On this page of JetBrains Rider settings, you can configure various aspects of code style in C++. Code style preferences are grouped in tabs, which are listed in this topic.
Auto-Detect Code Style Rules
Click this button to start analysis of formatting and syntax styles used in the current solution and detect rules that differ from your current settings. You will then be able to review the detected rules, change them as required, and save them to the desired settings layer or to a configuration file in the .editorconfig or .clang-format format.
General
C++ Formatting Engine | Use these radio buttons to choose which formatting engine should be used when JetBrains Rider formats and reformats your C++ code.
|
Write current style to .clang-format | Click this button to export code styles from JetBrains Rider settings to a .clang-format file. |
Tabs and Indents
This tab helps you specify how JetBrains Rider should format indents in your code when you type or when you reformat existing code.
Indentation-settings source
If there are .editorconfig files that affect your solution, preferences on this and other tabs with code style settings could be overridden by EditorConfig styles. You will see a yellow warning if at least one preference on the page is overridden by EditorConfig or Clang-Format styles for the current file, each overridden preference will also be highlighted with yellow. For example:
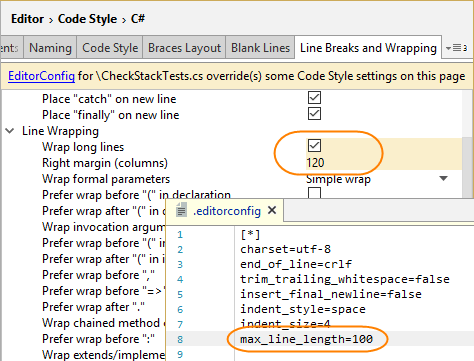
Combine indentation and alignment in multiline constructs
If your preferences say that whitespaces should be used for indents, JetBrains Rider will also use whitespaces to align multiple constructs — for example, when function parameters on multiple lines are aligned by the first parameter:
However, when you choose to use tabs for indents, there could be different ways for aligning multi-line constructs. JetBrains Rider lets you choose which of these ways to use when creating new and reformatting existing code.
You can configure preferences for aligning multi-line constructs with the How to align when tabs are used for indents option .
- Use spaces (recommended, looks aligned on any tab size)
When this option is selected, JetBrains Rider uses tabs for indents and spaces for alignment:
This is recommended option because code aligned with second and third options may lose alignment when viewed in an editor with a different tab size.
- Only use tabs (inaccurate)
When this option is selected, JetBrains Rider uses tabs for both indents and alignment, which may not result in precise alignment:
- Mix tabs and spaces for optimal fill
When this option is selected, JetBrains Rider uses tabs for both indents and alignment adds necessary spaces for precise alignment.
Naming
On this tab, you can configure symbol naming rules for C++.
If you're working with a UE4 project, JetBrains Rider will automatically apply a naming ruleset corresponding to the Unreal Engine coding standard, which is not configurable. This means that the rules configured on the page of JetBrains Rider settings Ctrl+Alt+S will be ignored when you're working on a UE4 project. If you still want to use your configured ruleset instead of the Unreal Engine coding standard, you can disable the corresponding inspection.
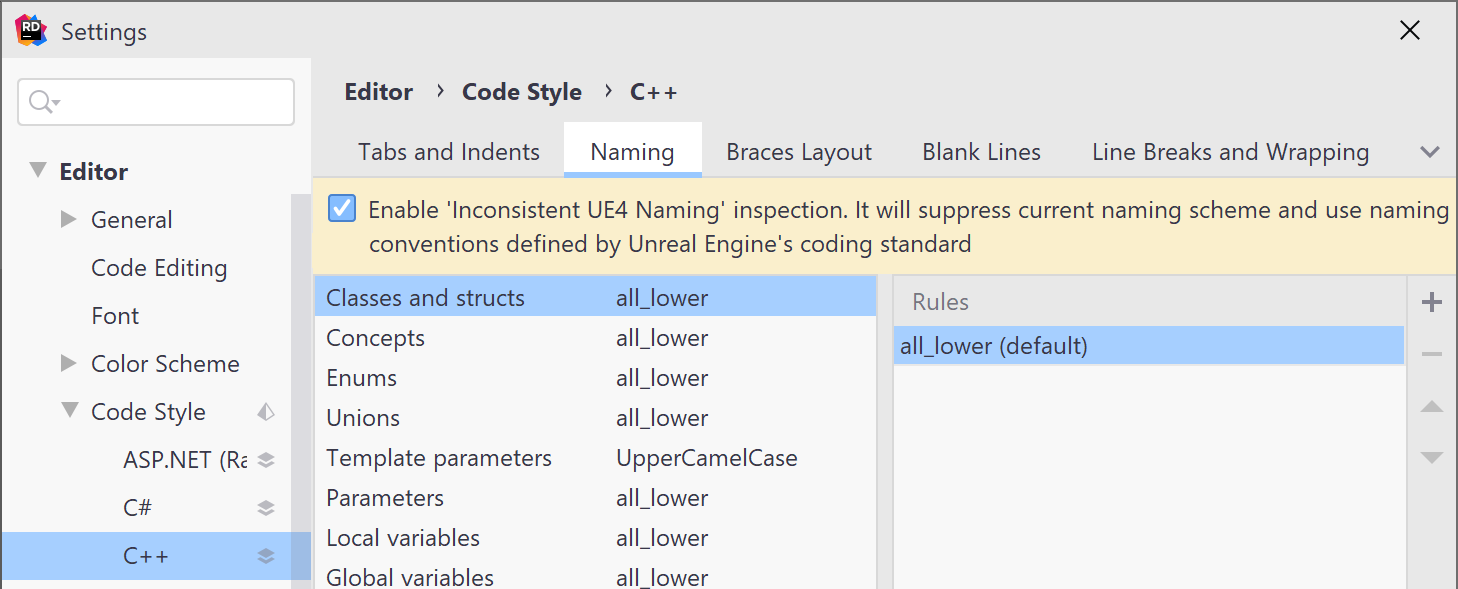
Syntax Style
Preferences configurable on this tab help you enforce code syntax style — how to use interchangeable language syntax constructions. These preferences are taken into account when JetBrains Rider produces new code with code completion and code generation features, applies code templates and performs refactorings. They can also be applied to the existing code by using code cleanup with the corresponding settings.
The preferences with the Notify with selector have corresponding code inspections that notify you if this aspect of the syntax style in the inspected scope differs from the preferred style. Using the selectors, you can configure severity levels of the inspections.
Sort include directives | Select this option to highlight any |
Type of slashes to use in include directives | Use this checkbox to choose the preferred path separator for include directives: forward slash or backward slash. |
Prefer to use forward declarations if possible | If this option is selected, auto-import puts the option to add a forward declaration before the option to insert an include directive. |
Use paths relative to the source file | You can use this selector to configure when JetBrains Rider may use relative paths in generated include directives. |
Use angle brackets instead of quotes | By default, generated include directives are added in quotes, if necessary, you can use this selector to specify cases where generated include directives are added in angle brackets. |
Default pointer initializer | You can specify |
Use uniform initialization in member initializers | By default, JetBrains Rider uses C++03 style when generating initializers (for example when you generate constructors or apply a quick-fix that inserts initializer into an existing constructor). You can select this checkbox to enable uniform initialization in the above-mentioned cases. |
Prefer uniform initialization in non-static data member initializers | This option lets you configure how JetBrains Rider generates non-static data member initializers (NSDMIs) when applying its features. For example, when you apply a quick-fix Alt+Enter on the uninitialized
class test {
int field;
};
JetBrains Rider will generate a uniform initialization |
Sort member initializers by the order of initialization | This option lets you enforce the order of member initializers in constructor initializer lists. Class members are initialized in the order they are declared in their containing class, so to minimize confusion and errors the common practice is to keep member initializers in the same order. |
'auto' usage in variable types | Preferences in this section let you configure the usage of |
Position of cv-qualifiers | Preferences in this section let you configure whether the |
Function declaration syntax | Use this option to configure whether to use trailing or regular return types in function declarations. |
Prefer typedefs or type aliases | Use this option to specify the preferred way to define type synonyms: in the form of alias templates or type aliases. It also affects which syntax is used by the Introduce Typedef refactoring. |
Nested namespaces | Use this option to enforce the C++17 syntax for nested namespaces, which allows you to declare multi-level namespaces in a more concise manner. |
Overriding functions | Preferences in this section let you configure which specifiers should be required on overriding functions and destructors. |
Braces | Preferences in this section let you define which braces can be omitted around single nested statements under the |
Remove redundant | Use this option to configure whether JetBrains Rider should remove optional braces that do not comply with the chosen preference. |
Braces Layout
Use this tab to adjust the way JetBrains Rider arranges braces when it generates new and reformats existing code; in particular, there are several ways to position braces after if
or for
operators.
For every item, there is a preview pane in the bottom part of the page where you can observe changes after tweaking specific preferences.
Blank Lines
This tab lets you configure whether JetBrains Rider should increase or decrease the number of blank lines around namespaces, members, regions and groups of import directives. You can adjust the values and check the preview pane at the bottom of the page to see how your preferences affect the code.
Options in the Preserve Existing Formatting section are only applied when JetBrains Rider reformats existing code whereas the Blank Lines section contains options that also take effect when you type new code.
The options that you configure on this tab apply in the following cases:
When code is automatically formatted on editing or after pasting (you can toggle auto-formatting on the page of JetBrains Rider settings Ctrl+Alt+S.
When JetBrains Rider produces new code with code completion and code generation features, applies code templates and performs refactorings.
When you reformat existing code.
Line Breaks and Wrapping
This page is used to configure line breaks in certain positions of source code. Options in this page are applied when JetBrains Rider reformats existing code or when you type new code. You can adjust the values and check the preview pane at the bottom of the page to see how your preferences affect the code.
Spaces
Use this tab to configure how to insert or remove spaces in different code constructs. You can adjust the values and check the preview pane at the bottom of the page to see how your preferences affect the code.
The options that you configure on this tab apply in the following cases:
When code is automatically formatted on editing or after pasting (you can toggle auto-formatting on the page of JetBrains Rider settings Ctrl+Alt+S.
When JetBrains Rider produces new code with code completion and code generation features, applies code templates and performs refactorings.
When you reformat existing code.
Indentation and Alignment
You can use preferences on this page to specify how JetBrains Rider indents and aligns your code in specific cases. In the Indentation group, you can define how tabs and indents (that you can configure on the options page) are applied in specific cases. In the Align multiple constructs group, you can define how code constructs that span multiple lines are aligned (you can specify the rules for breaking long lines on the options page).
The options that you configure on this tab apply in the following cases:
When code is automatically formatted on editing or after pasting (you can toggle auto-formatting on the page of JetBrains Rider settings Ctrl+Alt+S.
When JetBrains Rider produces new code with code completion and code generation features, applies code templates and performs refactorings.
When you reformat existing code.
Unreal Engine
On this tab, you can adjust formatting preferences that affect C++ code in Unreal Engine projects.
The options that you configure on this tab apply in the following cases:
When code is automatically formatted on editing or after pasting (you can toggle auto-formatting on the page of JetBrains Rider settings Ctrl+Alt+S.
When JetBrains Rider produces new code with code completion and code generation features, applies code templates and performs refactorings.
When you reformat existing code.