Code Style. C#
On this page of JetBrains Rider settings, you can configure various aspects of code style in C#. Code style preferences are grouped in tabs, which are listed in this topic.
Auto-Detect Code Style Rules
Click this button to start analysis of formatting and syntax styles used in the current solution and detect rules that differ from your current settings. You will then be able to review the detected rules, change them as required, and save them to the desired settings layer or to a configuration file in the .editorconfig or .clang-format format.
Tabs, Indents, Alignment
This tab helps you specify how JetBrains Rider should format indents in your code when you type or when you reformat existing code.
Indentation-settings source
If there are .editorconfig files that affect your solution, preferences on this and other tabs with code style settings could be overridden by EditorConfig styles. You will see a yellow warning if at least one preference on the page is overridden by EditorConfig or Clang-Format styles for the current file, each overridden preference will also be highlighted with yellow. For example:
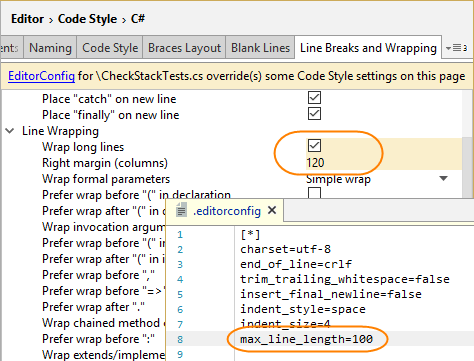
Combine indentation and alignment in multiline constructs
If your preferences say that whitespaces should be used for indents, JetBrains Rider will also use whitespaces to align multiple constructs — for example, when function parameters on multiple lines are aligned by the first parameter:
However, when you choose to use tabs for indents, there could be different ways for aligning multi-line constructs. JetBrains Rider lets you choose which of these ways to use when creating new and reformatting existing code.
You can configure preferences for aligning multi-line constructs with the How to align when tabs are used for indents option .
- Use spaces (recommended, looks aligned on any tab size)
When this option is selected, JetBrains Rider uses tabs for indents and spaces for alignment:
This is recommended option because code aligned with second and third options may lose alignment when viewed in an editor with a different tab size.
- Only use tabs (inaccurate)
When this option is selected, JetBrains Rider uses tabs for both indents and alignment, which may not result in precise alignment:
- Mix tabs and spaces for optimal fill
When this option is selected, JetBrains Rider uses tabs for both indents and alignment adds necessary spaces for precise alignment.
Naming
On this tab, you can configure symbol naming rules for C#.
JetBrains Rider helps you define, control, and apply desired naming styles for symbols in your code. There is a set of rules, each of which targets specific identifiers with the set of constraints (for example, a rule can target static private readonly fields) . Each rule can have one or more associated styles that define capitalization of compound words, underscores, suffixes, prefixes, and so on.
These rules are taken into account when JetBrains Rider produces new code with code completion and code generation features, applies code templates and performs refactorings. JetBrains Rider also helps you detect and fix violations of naming rules. If necessary, the automatic checkup of naming rules can be configured or disabled.
Syntax Style
Preferences configurable on this tab help you enforce code syntax style — how to use interchangeable language syntax constructions. These preferences are taken into account when JetBrains Rider produces new code with code completion and code generation features, applies code templates and performs refactorings. They can also be applied to the existing code by using code cleanup with the corresponding settings.
The preferences with the Notify with selector have corresponding code inspections that notify you if this aspect of the syntax style in the inspected scope differs from the preferred style. Using the selectors, you can configure severity levels of the inspections.
'var' usage in declarations | Preferences in this section define how the implicitly typed local variables (also known as You can set different preferences of using 'var' or explicit type for different types:
For each of these preferences you can opt for using 'var', explicit type, or 'var' when evident. For more information, refer to Code Syntax Style: Implicit/Explicit Typing ('var' Keyword). |
Prefer Roslyn (Visual Studio) logic for type evidence | When configuring preferences of using 'var' keyword vs. explicit type, you can opt for Use 'var' when evident. This option seems self-explanatory but in some cases it might be unclear what is considered 'evident' and what is not. Apart from that, there are some differences between what is considered evident (apparent) by JetBrains Rider and by Visual Studio when both products suggest using 'var' keyword or explicit type. Use this checkbox to apply Visual Studio logic for suggesting 'var' or explicit type when Use 'var' when evident is chosen in any options above. To learn the differences between JetBrains Rider and Visual Studio logic, refer to Use 'var' when evident: what is considered evident?. This checkbox is enabled automatically when the |
Prefer separate declarations for deconstructed variables | By default, JetBrains Rider will suggest joined notation for multiple |
Use 'var' keyword for discards | By default, JetBrains Rider suggests using standalone |
Instance members qualification | Preferences in this section define how to use 'this' qualifier. For more information, refer to Code Syntax Style: Optional Member Qualifiers. |
Static members qualification | Preferences in this section define how to qualify static members. For more information, refer to Code Syntax Style: Optional Member Qualifiers. |
Built-in types | Preferences in this section define how to reference C# built-in types: you can either use C# keywords or CLR type names. For more information, refer to Code Syntax Style: Built-In Type References. |
Reference qualification and 'using' directives | Preferences in this section define the style of namespace imports:
A number of other options related to namespace imports can be configured on the page of JetBrains Rider options. |
Modifiers | Preferences in this section define how to arrange modifiers of types and members. For more information, refer to Code Syntax Style: Modifiers. |
Arguments | Preferences in this section let you define how named or positional arguments should be enforced for specific types of parameters. For more information, refer to Code Syntax Style: Named/Positional Arguments. |
Parentheses | Preferences in this section let you define when optional parentheses should be removed or added if they help you clarify precedence of operations. For more information, refer to Code Syntax Style: Optional Parentheses. |
Braces | Preferences in this section let you define which statements require braces for single nested statements. For more information, refer to Code Syntax Style: Braces for Single Nested Statements. |
Code body | Preferences in this section let you define which kinds of members should be declared with the expression body and which with the block body. For more information, refer to Configure preferences for expression-bodied functions. By default, JetBrains Rider applies heuristics to decide whether to convert block bodies of methods to expression bodies. Among other things, this means that JetBrains Rider will not suggest expression bodies for You can simplify this logic by clearing the Apply style heuristics checkbox. In this case, the logic is simple: if you choose to use expression body for some kind of members, JetBrains Rider will suggest expression body if the declaration of a member of this kind consists of a single statement. The Namespaces option lets you choose whether to use file-scoped namespaces in files containing a single namespace declaration. |
Attributes | Preference in this section defines how to arrange multiple attributes. For more information, refer to Code Syntax Style: Multiple Attributes. |
Trailing Comma | Preferences in this section define how to treat trailing commas in declarations with multiple items and similar constructs (object, array, and collection initializers, as well as enums and switch expressions) . For more information, refer to Code Syntax Style: Trailing Commas. |
Object Creation | Preferences in this section define whether to add optional type specification when creating new objects with the |
Default Value | Preferences in this section define whether to use optional type specification |
Patterns | The Null checking pattern style in this section defines a style for null-checking inside pattern-matching expressions: |
Braces Layout
Use this tab to adjust the way JetBrains Rider arranges braces when it generates new and reformats existing code; in particular, there are several ways to position braces after if
or for
operators.
For every item, there is a preview pane in the bottom part of the page where you can observe changes after tweaking specific preferences.
Blank Lines
This tab lets you configure whether JetBrains Rider should increase or decrease the number of blank lines around namespaces, members, regions and groups of import directives. You can adjust the values and check the preview pane at the bottom of the page to see how your preferences affect the code.
Options in the Preserve Existing Formatting section are only applied when JetBrains Rider reformats existing code whereas the Blank Lines section contains options that also take effect when you type new code.
Line Breaks and Wrapping
Use this tab to configure how JetBrains Rider should add or remove line breaks before/after specific language constructs, and whether to wrap long lines exceeding the length specified by the Hard wrap at preference. You can adjust the values and check the preview pane at the bottom of the page to see how your preferences affect the code.
Note that preferences with names starting with Keep existing... allow you to keep existing formatting for other preferences in the same group.
Spaces
Use this tab to configure how to insert or remove spaces in different code constructs. You can adjust the values and check the preview pane at the bottom of the page to see how your preferences affect the code.
Null Checking
Use this tab to customize generating null-checking routines for exceptions and assertions.
Null checks for exceptions and assertions
There are situations when encountering an object that is a null
reference is critical in your program and should be either logged or signalled by throwing an exception. A typical example here is throwing an ArgumentNullException
in a function that is not designed to accept null
objects.
Generate null checks for exceptions and assertions
You can generate these kinds of null checks in the following ways:
Press Alt+Enter on a parameter or expression and choose the corresponding context action:
If a parameter is marked with the [NotNull] attribute, you can place the caret directly after the parameter name or parameter type and press !:
private void Foo([NotNull] object/*!*/ arg/*!*/)When generating a constructor (Alt+Insert ), select Check parameters for null in the dialog.
To generate an assertion for
null
for any nullable expression, JetBrains Rider provides the Assert expression for null action on Alt+Enter. Depending on the nullability analysis settings, it appears as a quick-fix or a context action.This action becomes unavailable if JetBrains Rider infers that the expression can never be
null
.
If you use code annotations in your project, JetBrains Rider will decorate parameters, which you check for null, with the [NotNull] attribute. This will let JetBrains Rider notify you when null objects are passed to the decorated parameters.
You can disable adding [NotNull]
by clearing the Automatically propagate annotations checkbox on the page of JetBrains Rider settings Ctrl+Alt+S.
Configuring null checks for exceptions and assertions
This kind of null checks can be written in different ways, so they are configurable on the
page of JetBrains Rider settings Ctrl+Alt+S, which is also accessible from the Alt+Enter menu on the corresponding actions: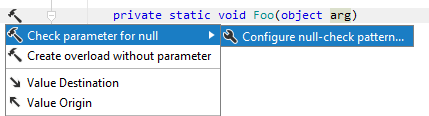
This settings page lists all predefined null-checking patterns in the priority order with higher priority patterns shown on the top. When JetBrains Rider generates a null check, it will take the highest-priority pattern which semantically suits the context, taking into account the current C# version.
By default, JetBrains Rider automatically detects C# version based on the associated compiler. However, you can specify the target C# version explicitly for a project — press Alt+Enter on the project in the Solution Explorer and use the Language version selector on the Application page of the Project Properties dialog .
To set the C# version for all projects in your solution, specify it in a Directory.Build.props file in your solution directory as described here.
For example, with the default configuration the 'throw expression' pattern $EXPR$ ?? new System.ArgumentNullException($NAME$);
has higher priority than the 'classic' throw statement if ($EXPR$ == null) throw new System.ArgumentNullException($NAME$);
. But if expressions are not allowed in the current context, JetBrains Rider will skip the first and use the second pattern:
The same applies to generating assertions: JetBrains Rider will use the first pattern that is marked with Can use for assertion.
If you have any preferences for generating null checks, use the Move up Alt+U/Move down Alt+D buttons on the settings page to raise the priority of patterns that you prefer.
Creating custom null checks for exceptions and assertions
If your codebase provides dedicated helper methods for handling null checks, you may want to create your own null-checking patterns by editing the two custom patterns that are highlighted in bold on the settings page — Custom (statement) and Custom (expression):
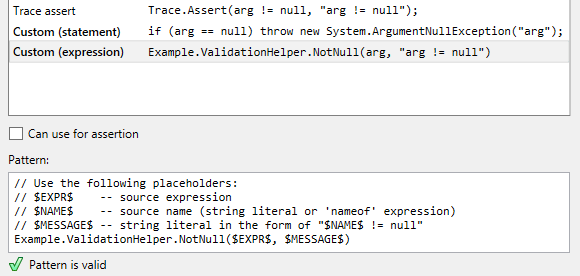
By default, these two patterns have the lowest priority, meaning that they will never be used for generation. So if you are going to use them, move them up to raise their priority.
When a custom pattern is selected in the list, you can edit it in the text field at the bottom of the page using the $EXPR$
, $NAME$
, $MESSAGE$
placeholders. As long as the pattern is valid, JetBrains Rider will display the corresponding icon below the text field.
You can also tick the Can use for assertion checkbox to make the pattern work with the Assert expression for null action.
XML Documentation
This tab helps you configure a number of settings that control how JetBrains Rider generates new XML Doc Comments code and how it reformats existing code. You can adjust the values and check the preview pane at the bottom of the page to see how your preferences affect the code.
File Layout
This tab contains the pattern that defines how to reorder type members when cleaning up your code. You can use one of the default patterns or create your own pattern. For more information, refer to Rearrange members with file and type layout patterns.
Other
This tab helps configure a number of additional settings that control how JetBrains Rider treats new code and reformats existing code. You can adjust the values and check the preview pane at the bottom of the page to see how your preferences affect the code.