Interact with other Space Modules
The kotlinScript
block provides the space()
API that lets you work with other Space modules like Chats, Issues, Documents, and so on.
For example, this is how you can send a message to a channel from a job:
The space()
API is a Space HTTP API client that works by sending HTTP requests to Space.
How the API client authorizes in Space
To authorize in Space, the space()
API client uses an Automation service account. When you create a project, Space creates this account based on the Automation Service role.
The service account exists only in the project scope and doesn't have any global permissions. This means that the space()
client can work only with Space modules within the current project (for example, Issues, Documents, Checklists, and so on) or with Space modules that don't check permissions (for example, Chats).
To change permissions of the Automation service account
Open the required project, then Settings.
In the Access tab, find the Automation Service role and change the permissions.
How to make the work with the API client easier
As the space()
API client uses the Space HTTP API, the best place to look for particular code snippets is the Space API Playground. Here you can find the required API request, check the required permissions, and get a Kotlin code snippet (switch the code snippet panel to Kotlin SDK):
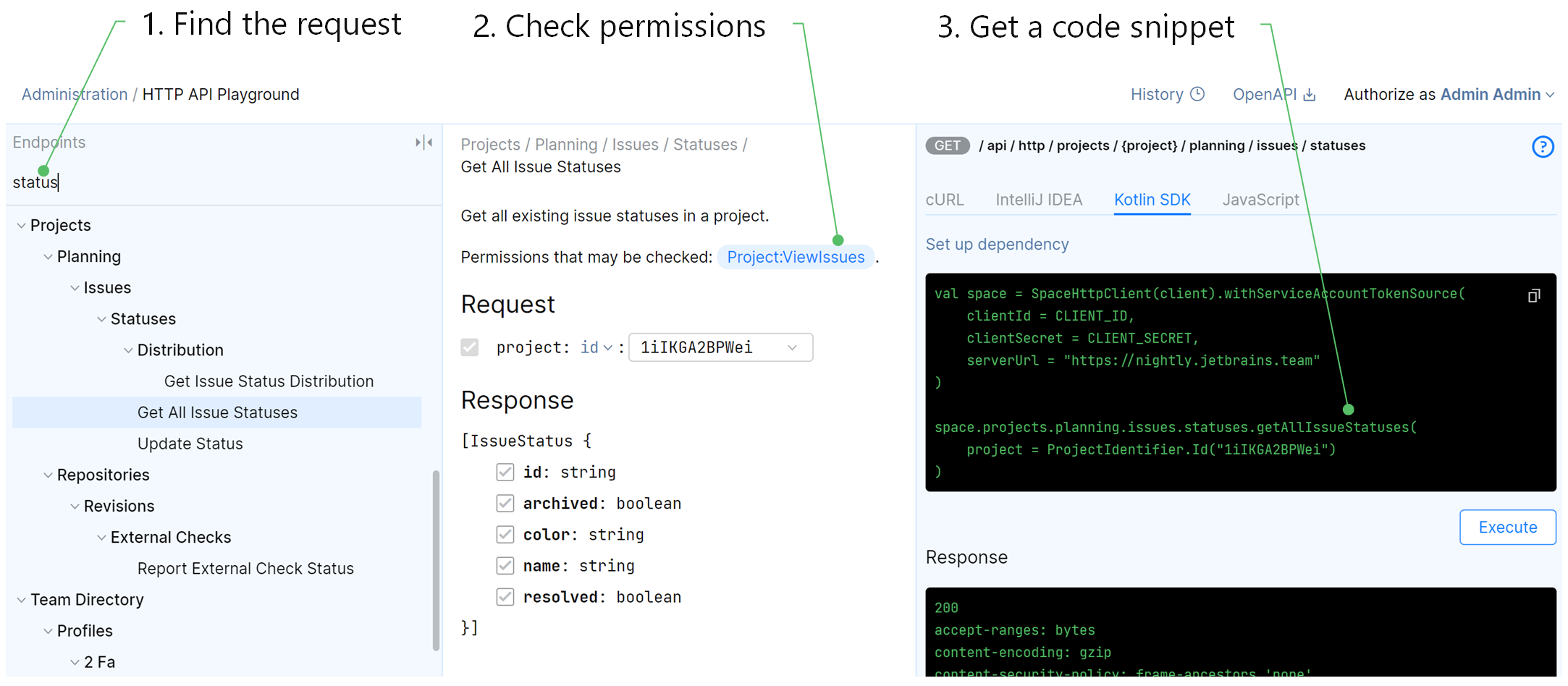
One more way to ease working with the client is to edit your .space.kts
file in IntelliJ IDEA with the installed Space plugin (starting IntelliJ IDEA 2021.1, the plugin is bundled with the IDE). The plugin provides code completion for the Automation DSL and for the space()
client APIs:
Example. Create an issue
The following job builds a Gradle project and if the build fails, creates an issue. In order for this job to work, the Automation Service role in your project must have the Create Issues permission, which you can enable for this role in .