Running and debugging TypeScript
With WebStorm, you can run and debug client-side TypeScript code and TypeScript code running in Node.js.
Before running or debugging an application, you need to compile your TypeScript code into JavaScript. You can do that using the built-in TypeScript compiler and other tools, including ts-node for running TypeScript with Node.js, used separately or as part of build process.
During compilation, there can also be generated source maps that set correspondence between your TypeScript code and the JavaScript code that is actually executed. As a result, you can set breakpoints in your TypeScript code, launch the application with the run/debug configuration of the type JavaScript Debug (for client-side code) or Node.js, and then step through your original TypeScript code, thanks to generated sourcemaps.
Run a client-side TypeScript application
You can write a client-side application in TypeScript, compile the code as described in Compiling TypeScript into JavaScript, and then run and debug your application exactly in the same way as client-side applications written in JavaScript. The only difference is that you can set breakpoints right in the TypeScript code.
In the editor, open the HTML file with a reference to the generated JavaScript file. This HTML file does not necessarily have to be the one that implements the starting page of the application.
Do one of the following:
Choose
from the main menu or press Alt+F2. Then select the desired browser from the list.Hover over the code to show the browser icons bar:
. Click the icon that indicates the desired browser.
Debug a client-side TypeScript application
If your application is running on the built-in WebStorm server, refer to Running a client-side TypeScript application above, you can also debug it in the same way as JavaScript running on the built-in server.
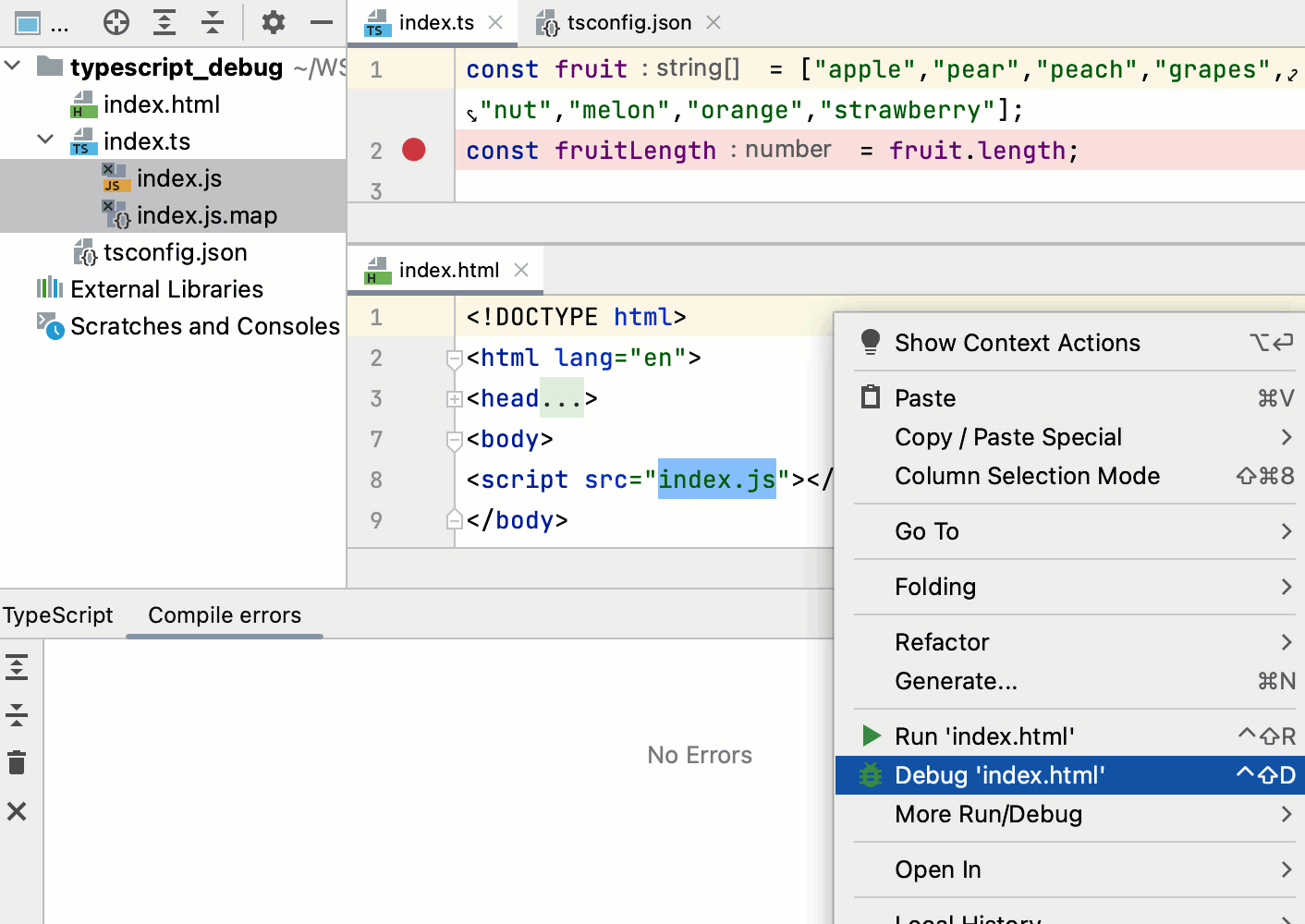
Debug a TypeScript application running on an external web server
Most often, you may want to debug a client-side application running on an external development web server, for example, powered by Node.js.
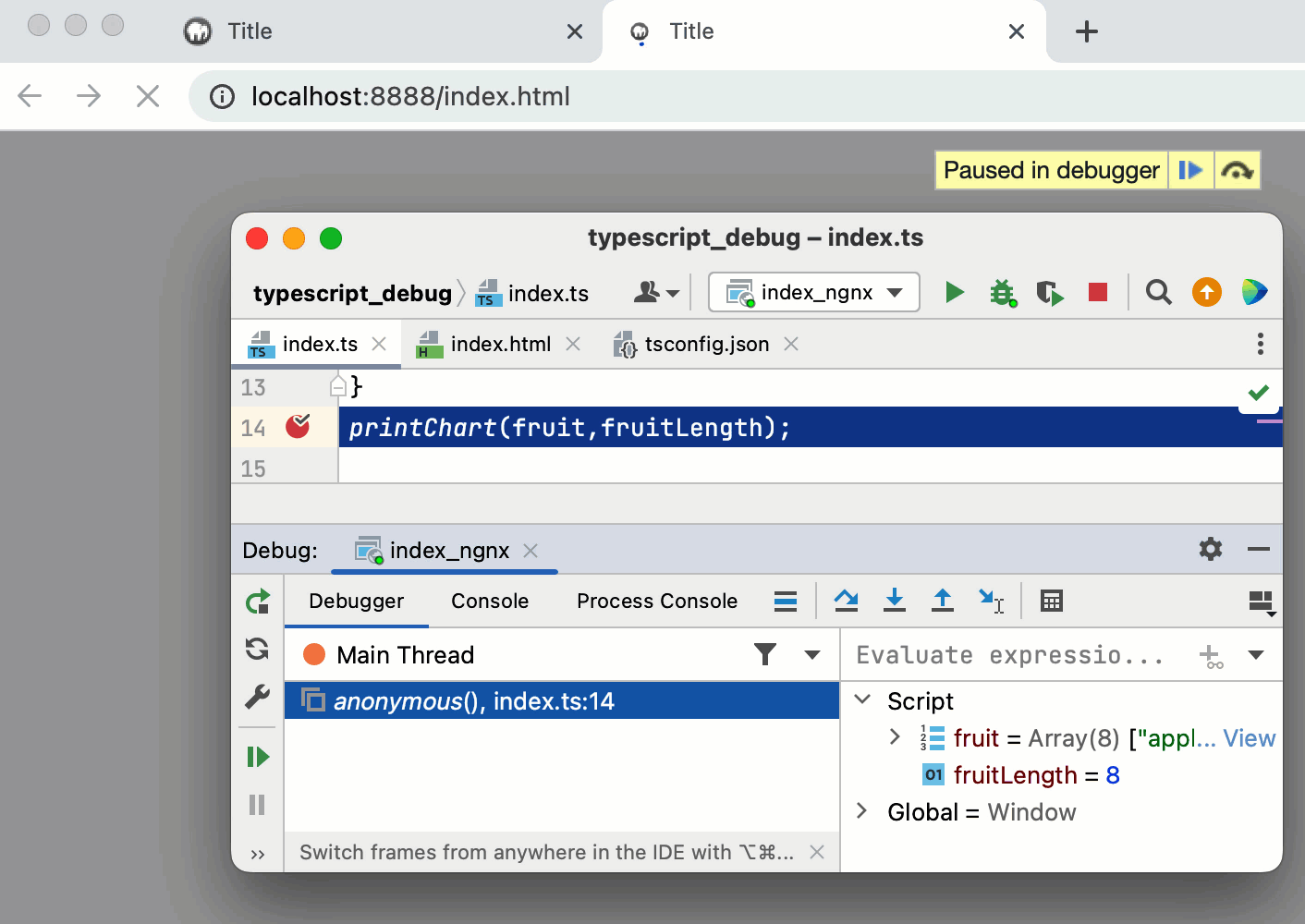
Configure the built-in debugger as described in Configuring JavaScript debugger.
To enable source maps generation, open your tsconfig.json and set the
sourceMap
property totrue
, as described in Create a tsconfig.json file.Configure and set breakpoints in the TypeScript code.
Run the application in the development mode. Often you need to run
npm start
for that.Most often, at this stage TypeScript is compiled into JavaScript and source maps are generated. For more information, refer to Compiling TypeScript into JavaScript.
When the development server is ready, copy the URL address at which the application is running in the browser - you will need to specify this URL address in the run/debug configuration.
Go to Edit Configurations from the list on the toolbar.
. Alternatively, selectIn the Edit Configurations dialog that opens, click the Add button (
) on the toolbar and select JavaScript Debug from the list. In the Run/Debug Configuration: JavaScript Debug dialog that opens, specify the URL address at which the application is running. This URL can be copied from the address bar of your browser as described in Step 3 above.
From the Select run/debug configuration list on the toolbar, select the newly created configuration and click
next to it. The URL address specified in the run configuration opens in the browser and the Debug tool window appears.
You may need to refresh the page in the browser to get the controls in the Debug tool window available.
In the Debug tool window, proceed as usual: step through the program, stop and resume the program execution, examine it when suspended, view actual HTML DOM, run JavaScript code snippets in the Console, and so on.
Run and debug a server-side TypeScript application with Node.js
With WebStorm, you can launch server-side TypeScript code on Node.js via the Node.js run configuration. Before running or debugging, your TypeScript code has to be compiled into JavaScript, as described in Compiling TypeScript into JavaScript.
For debugging, also make sure that compilation produces source maps that set correspondence between your TypeScript code and the JavaScript code that is actually executed. For more information, refer to Create a tsconfig.json file.
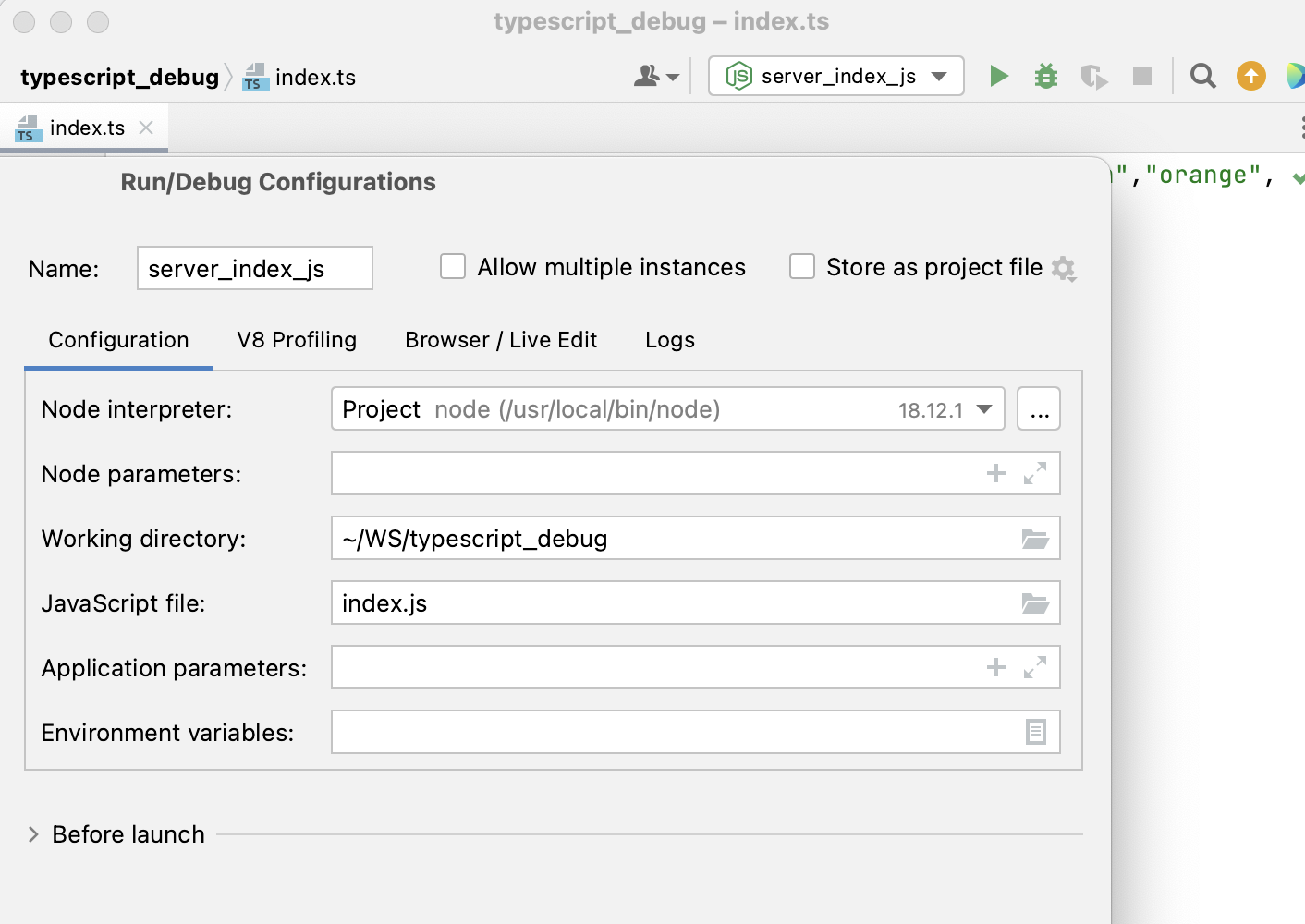
Before you start
Make sure you have Node.js on your computer.
Make sure the Node.js plugin is enabled in the settings. Press Ctrl+Alt+S to open settings and then select . Click the Installed tab. In the search field, type Node.js. For more information about plugins, refer to Managing plugins.
To enable source maps generation, open your tsconfig.json and set the
sourceMap
property totrue
, as described in Create a tsconfig.json file.
Create a Node.js run/debug configuration
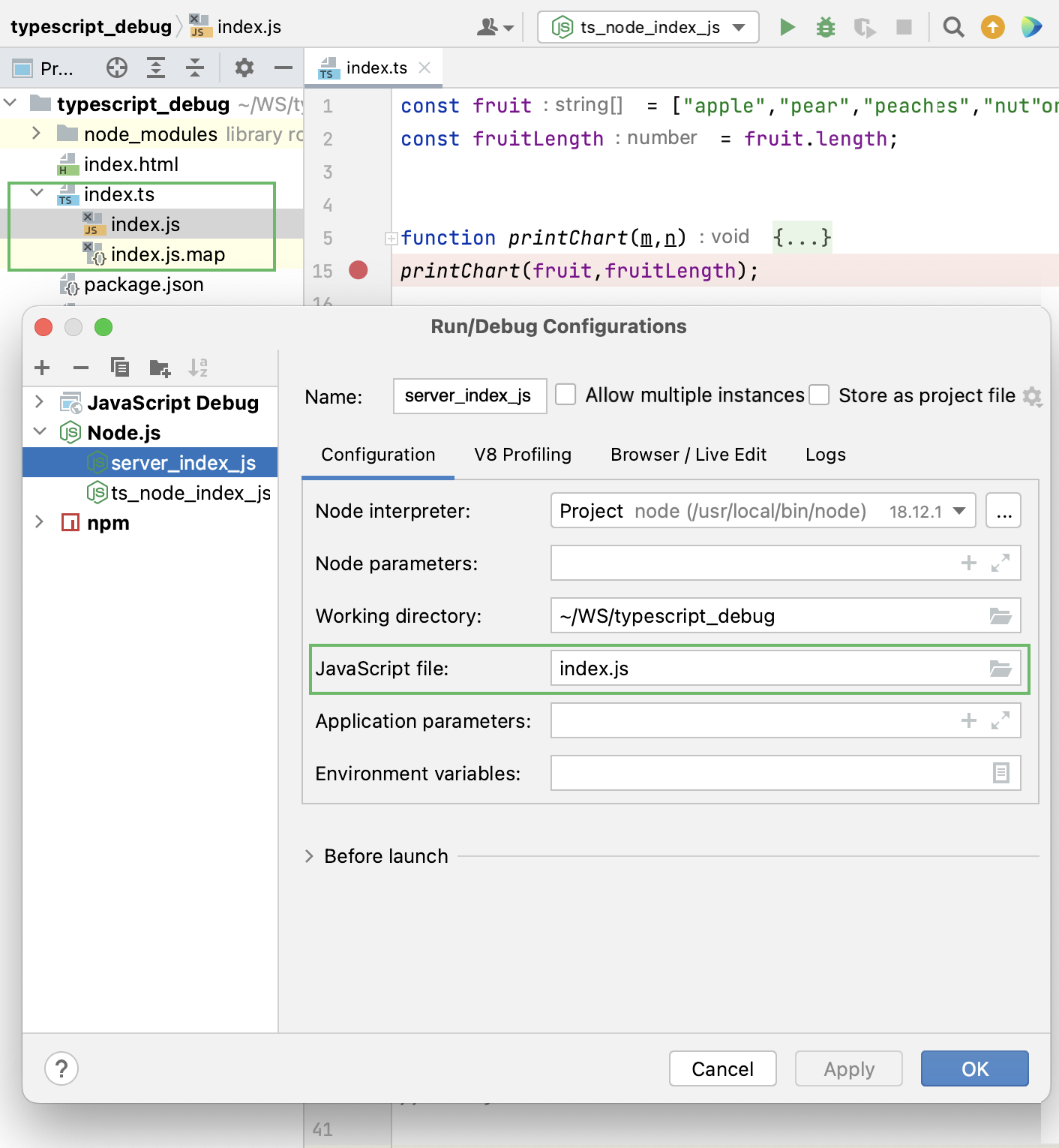
Go to Edit Configurations from the list on the toolbar.
. Alternatively, selectIn the Edit Configurations dialog that opens, click the Add button (
) on the toolbar and select Node.js from the list.
In the Run/Debug Configuration: Node.js dialog that opens, specify the Node.js interpreter to use.
In the JavaScript File field, specify the compiled file generated from the main file of the application that starts it.
Learn more from Creating a Node.js run/debug configuration.
Run server-side TypeScript with Node.js
Compile your TypeScript code into JavaScript. For more information, refer to Compiling TypeScript into JavaScript.
Create a Node.js run/debug configuration as described above.
From the Run/Debug Configurations list on the toolbar, select the newly created Node.js configuration and click
next to it.
Debug server-side TypeScript with Node.js
Compile your TypeScript code into JavaScript. For more information, refer to Compiling TypeScript into JavaScript.
Set the breakpoints in the TypeScript code where necessary.
Create a Node.js run/debug configuration as described above.
From the Select run/debug configuration list on the toolbar, select the newly created Node.js configuration and click
next to it. The Debug tool window opens.
Perform the steps that will trigger the execution of the code with the breakpoints.
Switch to WebStorm, where the controls of the Debug tool window are now enabled.
Use ts-node
If you need to run or debug single TypeScript files with Node.js, you can use ts-node instead of compiling your code as described in Compiling TypeScript into JavaScript.
Install ts-node
In the embedded Terminal (Alt+F12) , type:
npm install --save-dev ts-node
Create a custom Node.js run/debug configuration for ts-node
Go to Edit Configurations from the list on the toolbar.
. Alternatively, selectIn the Edit Configurations dialog that opens, click the Add button (
) on the toolbar and select Node.js from the list. The Run/Debug Configuration: Node.js dialog opens.
In the Node Parameters field, add
--require ts-node/register
.Specify the Node.js interpreter to use.
If you choose the Project alias, WebStorm will automatically use the project default interpreter from the Node interpreter field on the Node.js page . In most cases, WebStorm detects the project default interpreter and fills in the field itself.
You can also choose another configured local or remote interpreter or click
and configure a new one.
For more information, refer to Configuring remote Node.js interpreters, Configuring a local Node.js interpreter, and Using Node.js on Windows Subsystem for Linux.
In the JavaScript File field, specify the TypeScript file to run or debug. Depending on your workflow, you can do that explicitly or using a macro.
If you are going to always launch the same TypeScript file, click
and select this file in the dialog that opens. By default, the run/debug configuration gets the name of the selected file.
If you need to launch different files, type
$FilePathRelativeToProjectRoot$
. With this macro, WebStorm will always launch the file in the active editor tab.
If necessary, specify any additional parameters for
ts-node
(for example,--project tsconfig.json
) in the Application parameters field.Save the configuration.
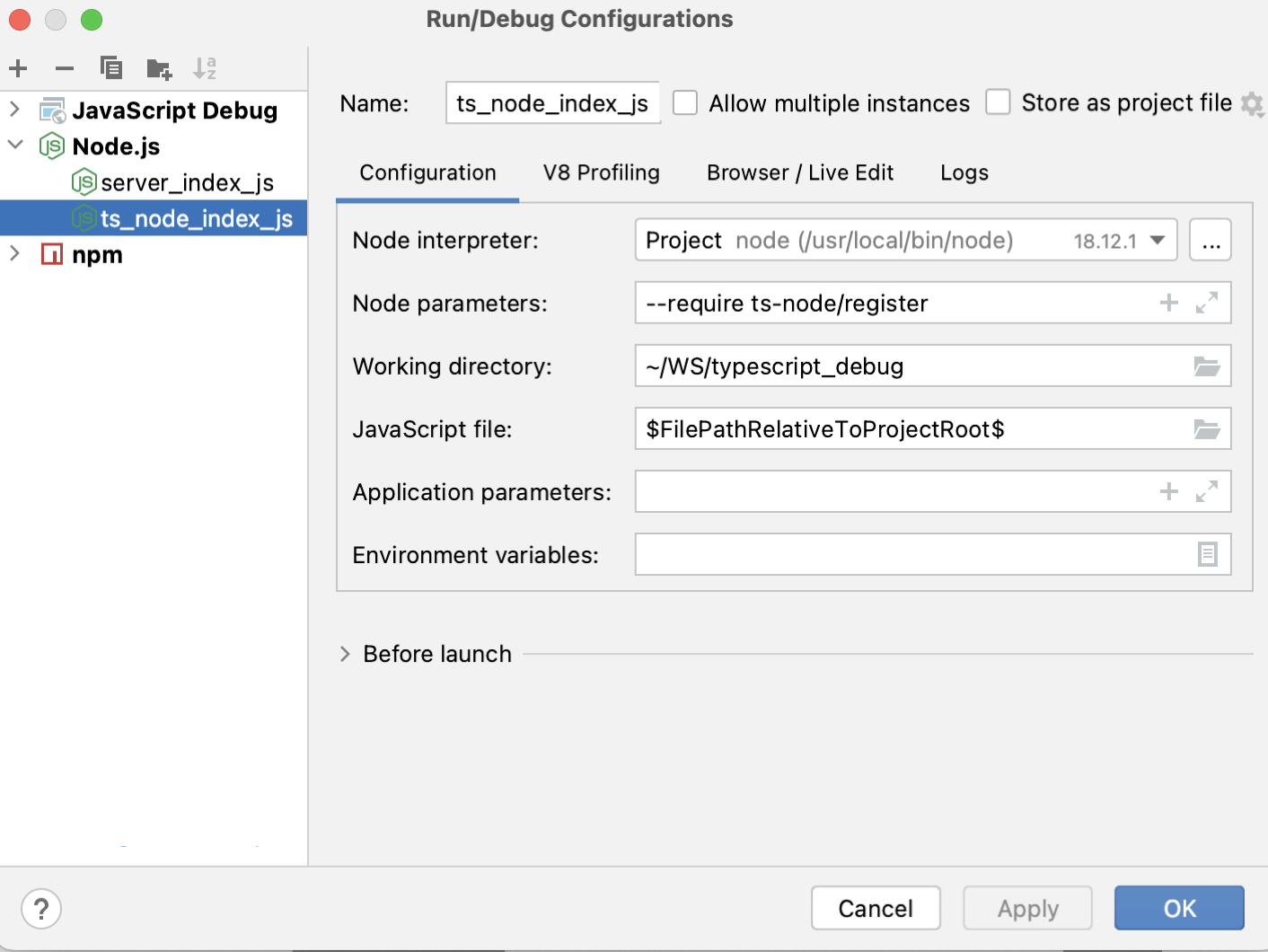
Run a TypeScript file with ts-node
Depending on the way you specified your TypeScript file in the run/debug configuration, do one of the following:
If you typed the filename explicitly, select the required configuration from the list on the toolbar and click
next to the list or press Shift+F10.
If you specified a macro, open the TypeScript file to run in the editor, select the newly created configuration from the list on the toolbar, and click
or press Shift+F10.
WebStorm shows the output in the Run tool window.
Debug a TypeScript file with ts-node
In the TypeScript file to debug, set the breakpoints as necessary.
Depending on the way you specified your TypeScript file in the run/debug configuration, do one of the following:
If you typed the filename explicitly, select the required configuration from the list on the toolbar and click
next to the list or press Shift+F9.
If you specified a macro, open the TypeScript file to debug in the editor, select the newly created configuration from the list on the toolbar, and click
next to the list or press Shift+F9.